Werdmüller on Medium
Where I’m coming from
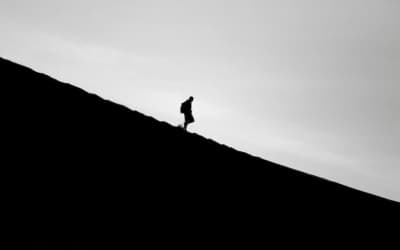
Brought to you by Identity Woman and Infominer.
Support this collaboration on Patreon!!!
I’m paralyzed by the world. We seem to be at a kind of crossroads.
There’s so much to be appalled by, so much to be worried about, and I worry that not saying something might be considered to be acquiescence or approval.
So, in this moment, I thought I’d actually take a step back and explain what my worldview actually is. It’s perhaps overly ambitious, but I want to declare I think is important, and what drives me to say the sorts of things I do. And, yes, these same factors also drive the decisions I make about where I work and how I build software.
As always, I would like to read yours.
My view on the world — as is true of yours, and of everybody’s — is a function of my lived experiences, and the lived experiences of the people I care about.
It’s not about leading the world; it’s about living in a peaceful oneI was born in the Netherlands, grew up in England, spent time in Austria, went to school in Scotland, and have been in the United States since my early thirties. My dad is Swiss-Dutch-Indonesian; my mother was American on one side and Ukrainian on the other. They met in Berkeley in the early seventies and were heavily active in various progressive causes as activists. My dad in particular, who was drafted into the US Army after his family moved to America as a teenager, organized Vietnam War protests in the Bay Area, and was often harassed by the police.
My parents intentionally left the US to raise me. The closest thing I have to a hometown is Oxford, famous for its universities, where academic families are constantly coming through. My peers at school came from all over the world — including from behind the iron curtain — and experiencing the different smells and tastes of peoples’ homes was completely normal.
At the same time, I was a third culture kid for almost all of my childhood and early adulthood, and identified with no national identity. I never felt any real ties to any particular geographic place for its own sake. I was raised an atheist and have never felt religious ties (even though, as a child in England, I went to a Church of England school where we were made to pray every day). What I did identify with, very strongly, was family.
My dad is one of the youngest concentration camp survivors: he spent his early years in a Japanese-run camp in Indonesia, which still colors the way he sees the world. His mother had nightmares every single night for the rest of her life; I will remember hearing her screams though the walls forever. His father, who I never got to meet, was a resistance leader who was forced to dig his own grave multiple times. I’ve heard stories about the camps and what happened afterwards for my entire life. Even when they returned to the Netherlands, Indo people like my dad’s family were an ethnic minority and treated poorly. They eventually moved to California, thanks to a local sponsor, where they ran a gas station on highway 12 in Sebastopol. My uncle was severely beaten up for daring to serve Black people. The gas station itself was routinely shot at — once killing the family dog — simply because it was run by immigrants.
My great grandfather escaped Ukraine twice. His village was destroyed by the White Army as part of vicious Pogroms. When he emigrated to the US, he secularized, changing his name in the process in order to sound less Jewish. Eventually he became the General Manager of the Pennsylvania Joint Board of the Amalgamated Shirt Workers. I’ve previously posted excerpts from my grandfather’s obituary that discuss that experience as well as my grandfather’s experience as a Jewish POW in Germany during WWII.
My grandfather, by the way, ended up translating Crime and Punishment into English, and taught in the Slavic and Eurasian Studies and History departments at the University of Texas at Austin for forty years. He met Albert Einstein, had coffee with Sylvia Plath, discussed philosophy with Hannah Arendt, and never quite realized his dream of being a poet. In the end, he married into an institutional American family: my great grandfather was a WWI test pilot and eventually became a diplomat who negotiated the US withdrawal from Haiti. (This fact of my family history is, I want to be clear, not an endorsement of the US’s behavior overseas, including in Haiti.)
So, all of this is to say: I have no interest in patriotism, let alone nationalism. It’s not a value I hold, and I’m not excited by any country having a leadership position in the world. I find flag-waving to be petty. What I care about are values: the democracy, inclusion, and co-operation that can lead to a lasting peace. I’m repelled by military strength, because I’ve seen what various militaries did to my family. I’m repelled by anti-immigrant sentiment, because I come from refugees and immigrants. I don’t like the idea of assimilation, because I’ve seen the richness inherent in lots of cultures. Forced assimilation — which is usually into a conquering culture — is tantamount to subjugation.
National exceptionalism — American exceptionalism, or European exceptionalism, come to that — is ridiculous on its face. Cold wars and imperialist foreign policies are things to avoid, not things to perpetuate. No country is the “best”, and even the idea of “a best country” is narrow-minded. No religion is the “best”; please enjoy practicing yours, but please don’t impose it on anyone else. There are definitely people who think McCarthy’s witch hunt against communism was right in spirit, even if they condemn the historical event itself — let’s just say they and I harbor some very different ideas about what an open, democratic society should look like.
Nations aren’t what’s important. Principles are. Specifically, the principles of openness, inclusion, fairness, peace, equity, and democracy.
What matters is that everyone can live a good life, wherever they are, whoever they are, and however they identify, free from threat of violence or exploitation. Ideological or national superiority aren’t useful values. What matters is the experience of being a human, everywhere. What matters is avoiding the killing and horror of war. What matters is honoring the beautiful diversity of the world.
A strong operating system for allI was only half-joking when I compared governments to operating systems. While they certainly don’t map perfectly to actual software operating systems, I do think they provide a very similar purpose: to create a bedrock of services and infrastructure in order to ensure society runs smoothly.
What does “society runs smoothly” really mean? I’ll return to my definition above. What matters is that everyone can live a good life, wherever they are, whoever they are, and however they identify, free from threat of violence or exploitation. Freedom of expression, association, and to pursue one’s best interests are important here: what John Locke called the pursuit of happiness. To ensure the safety of that pursuit, I think John Locke’s version of a social contract — the idea that we surrender a little personal liberty in order to make evolving common agreements in the best interests of everyone — is important.
I can’t be a libertarian because I see the importance of the trade-offs here. One role of the operating system is to prevent the vulnerable from exploitation: public goods like universal healthcare, public education, and integrated public transit ensure that people who are not wealthy have the opportunity to build a great life. One of the most visceral reactions I’ve ever had in my life was discovering Ayn Rand, and then, to my horror, discovering that beyond just getting into her novels, some people actually believed in her ideology of everyone for themselves.
Healthy communities are an important part of all of our well-being. Once again: every person deserves to live a good life. We all live in a complex, interconnected network of people, and what happens to someone else also affects us. Caring for the whole network is also in our own best interests. It can’t be everyone for themselves.
It’s hopefully obvious from my definition, but I don’t think GDP (or money at all) is a great way to measure a society, either. It doesn’t say much about what an ordinary person’s experience actually is. It doesn’t measure human well-being, and that’s how we should be thinking about how well we’re doing. I’m less interested in is the stock market going up? than is being poor a death sentence? as a question — and I don’t think the first necessarily leads to a reduction in the second. More and more people agree.
One important function of the social operating system is welfare, which ensures that people don’t fall though the cracks. There are others, some of which I’ve already mentioned: education, transit, and healthcare.
I couldn’t have founded my first startup if I hadn’t had the benefit of the excellent National Health Service. Millions of PR dollars have been spent in the US to paint social infrastructure as being a bad thing, but I never once had to worry about going to the doctor under universal healthcare. I didn’t have to worry about losing health insurance when I quit my job. I could just do it. Say what you want about free markets, but I think that freedom of optionality — having broad choices regardless of income or personal net worth — comes closer to real personal freedom than a world without that kind of social infrastructure.
Here in the United States, it doesn’t come automatically: you need people to fight for you. I’ve lost five members of my family to an incurable genetic disease. One of them was my mother, who I helped care for over the course of a decade — which was, in fact, the reason I moved to the US to begin with. She was a teacher, and the great medical care she received was only possible because of the incredible negotiating power of her teacher’s union. While there should have simply been universal healthcare to look after her, their incredible negotiating power literally lengthened her life by eight years. Unions can be amazing institutions; while not every union is great, the concept of them is. And in a world without the social infrastructure to care for the vulnerable, they are vital.
This should be the purpose of the law, too: to prevent harm and exploitation, particularly of the vulnerable, in service of maintaining the ability to have a good quality of life. But the law itself, alongside tradition and the twin ideas of unity and stability, often does the opposite. It has often used as a way to maintain a status quo where vulnerable people are exploited for other peoples’ benefit.
This runs deep: some of the earliest police forces in America were slave patrols. A law that only benefits the powerful or upholds an unjust status quo is, in itself, unjust. Unity that depends on adherence to the values of the powerful (and on the silence or silencing of the vulnerable) is a sham. Stability based on prioritizing the needs of an in-group to the exclusion of others is definitionally fascism. A claim that moderate values are more reasonable only makes sense to people who don’t need more radical change in order to achieve equity.
Being awake to those injustices is not a binary: it’s not something you either are or not. It’s an ongoing, uncomfortable process of education and coming to terms. There are lots of ways to deal with and redress them, the comparative merits of which are up for discussion. What’s clear to me, though, is that dismissing their existence outright, and painting them with a reductionist brush in order to rob them of importance, is in itself a perpetuation of those injustices.
When people started talking about being “woke” and taking to the streets to demand restorative justice, I was relieved and excited. This is what moving forward looks like. In contrast, I see people harping on about the harms of “woke-ism” as being part of the dying gasps of the twentieth century: that adherence to tradition and unity and stability in service of the same old inequalities. Change is good; particularly here.
Change can also be easy. Using a preferred pronoun costs you nothing except for letting go of a tradition. The tradition, in other words, gets in the way of someone’s chosen identity being recognized. Same-sex marriage costs you nothing except for letting go of a tradition. The tradition once again gets in the way of someone being able to realize their needs. Your religious beliefs might forbid same-sex marriage; then simply don’t get same-sex married. Practice your own religion to your heart’s content, but don’t enforce your traditions on anyone else. Refer to someone as they would like to be referred; treat everybody with respect. It seems foundational. A fear of change or adherence to a tradition should not be a barrier to making the world more just or treating our fellow humans with respect.
Let’s return again to the core idea: every person deserves to live a good life. Of course, who we consider to be a “person” is important. Thomas Jefferson incorporated Locke’s version of a social contract into the Declaration of Independence, even going so far as to say that “all men are created equal,” but he famously kept slaves. These days, we might ask about our spheres of concern: do we care about people in our families? Our neighborhoods? Our towns and cities? Our churches? Our ethnicities? Our value structures? Our states? Our countries? Our regions? The world? How do we relate to people outside of those spheres?
For reasons I’ve tried to explain above, I’d love it if we considered the world to be what we care about; the welfare of a person in Gaza is just as important as the welfare of a person next door, even if we might not share a religion or care for the regime they live under. In fact, depending on their context, their welfare might be more important, because they need more help to bring them to that reasonable standard of living, free from violence and exploitation.
Because our definitions of what a good life is vary, and because no government can possibly claim to represent or understand the complete set of needs and lived experiences in its populace, participative democracy is the only equitable model for government. What’s important here: everyone can vote without hindrance, votes are fair, anyone can become a representative, decisions are actually made at the ballot box rather than in court, and there is real choice. (If there’s a candidate whose values you hate, do what you can to persuade your fellow voters to vote for someone else. That’s what democracy is.)
Those principles are core. It might surprise you to learn that I’m not inherently against the idea of billionaires, and certainly not against the idea of starting businesses and finding success in doing so. But it must be done without exploiting other people and preventing them from being able to live a good life. It must be done without perpetuating injustices, for example by eroding workers’ rights, forcing a minimum wage that is too low to live well on, lobbying for unequal laws, or fighting against their ability to negotiate for better working conditions. Can it be done without those things? I don’t know. But if it can’t, then it shouldn’t happen.
So what does this have to do with the internet?I see the web as a platform as being rooted in the kind of internationalism I believe in. The internet itself is a physical manifestation of the idea that we are all connected.
Anyone can publish, anywhere, and be read by anyone, anywhere. That’s amazing! Anyone can start a business and find users all over the world. That’s also amazing! It’s the most borderless, open platform we’ve ever created. The potential to learn about the lives of people we would never otherwise meet, in places we would never otherwise visit, is colossal. We can share ideas and, even more importantly, build empathy globally. I couldn’t be more excited about that. That’s what keeps me building.
It’s harder to dehumanize someone you don’t know. The internet has the potential to allow everybody to become knowable. I see that as a route to peace, and to a better world where exploitation can no longer happen in the shadows.
What I’m not enthused by is the idea that the internet is here as an exercise in furthering any one country’s interests: that one nation’s worldview should trump another’s. At its best, it’s an international commons: an overtly progressive space by design.
I support the indieweb and the fediverse because those technologies harness for the benefit of the public, rather than for the profit and entrenched power of a tiny few. I see silos and centralized services as being anti-democracy, because the whims of a monarch-like figure can have a profound impact on which information we’re allowed to see. We’ve seen that most obviously recently with Elon Musk’s acquisition of Twitter, but it was previously also true of Facebook, and of every large service that aimed to intermediate peoples’ connections with each other. If one entity controls what we see and can learn about, they will abuse it, always.
I’m imperfect. Of course I am. I’ve made terrible mistakes and, from time to time, I’ve hurt people. But that doesn’t mean I can’t try.
I’ve built open source platforms for organizing educational institutions and non-profits; I’ve supported newsrooms that help to create a more informed voting population; I’ve worked in newsrooms that help speak truth to power. It’s not because I love social networking in itself, or because I want to get rich by building software.
It’s because I remember the sound of my Oma having nightmares through the walls. I see the nationalists and isolationists as trying to divide people into in-groups and out-groups. I see hoarding wealth as akin to building walls. I see conservatism as being a way to preserve the kind of bigotry that can grow and explode into the kinds of hatred that swallow whole families. Quixotic as it might be, I see connecting people as a way to help prevent it all from ever happening again.
A fire broke out backstage in a theatre. The clown came out to warn the public; they thought it was a joke and applauded. He repeated it; the acclaim was even greater. I think that’s just how the world will come to an end: to general applause from wits who believe it’s a joke.
💬 Søren Kierkegaard
It's very fast to build something that's 90% of a solution. The problem is that the last 10% of building something is usually the hard part which really matters, and with a black box at the center of the product, it feels much more difficult to me to nail that remaining 10%. With vibecheck, most of the time the results to my queries are great; some percentage of the time they aren't. Closing that gap with gen AI feels much more fickle to me than a normal engineering problem. It could be that I'm unfamiliar with it, but I also wonder if some classes of generative AI based products are just doomed to mediocrity as a result.
If you’re auditioning for your job every day, and you’re auditioning against every other brilliant employee there, and you know that at the end of the year, 6% of you are going to get cut no matter what, and at the same time, you have access to unrivaled data on partners, sellers, and competitors, you might be tempted to look at that data to get an edge and keep your job and get to your restricted stock units.
Der hessische Ministerpräsident Boris Rhein (CDU) und Justizminister Christian Heinz (CDU) haben einen „Entwurf eines Gesetzes zur Einführung einer Mindestspeicherung von IP-Adressen für die Bekämpfung schwerer Kriminalität“ (PDF) vorgelegt. Am Freitag, 26. April 2024 soll das geplante Gesetz im Bundesrat behandelt werden.
Der Europaabgeordnete Dr. Patrick Breyer (Piratenpartei / Grüne/EFA) kommentiert:
„Dieser Vorstoß ist ein Rückschritt für die Kinderschutz-Debatte. Echter Kinderschutz wird seit Jahrzehnten vernachlässigt – statt für echte Lösungen zu sorgen wird das Problem als Vorwand für eine Internet-Massenüberwachung genutzt, die pauschal alle Bürgerinnen und Bürger unter Generalverdacht stellen würde. Der vorgelegte Gesetzentwurf ignoriert nicht nur dringend notwendige Maßnahmen für echten Kinderschutz, sondern auch den Fakt, dass es keinen messbaren Nutzen einer solchen Massenüberwachung gibt. Anstatt echte Lösungen auszuarbeiten, wollen Ministerpräsident Boris Rhein (CDU) und Justizminister Christian Heinz (CDU) offenbar das Rad zurückdrehen:
Vor gerade einmal zwei Wochen hat die Ampel anstelle anlassloser IP-Speicherung den Weg frei gemacht für das anlassbezogene und zielgerichtete Quick Freeze-Verfahren, wie es beispielsweise auch in Österreich eingesetzt wird. Der hessische Vorschlag hat das Potenzial die Einführung eines verhältnismäßigen Quick Freeze-Instruments aufzuhalten.
Rückschrittlich ist der Entwurf auch, weil das Bundesverwaltungsgericht erst 2023 die von CDU und SPD beschlossene Vorratsdatenspeicherung in vollem Umfang für unionsrechtswidrig und damit für nicht anwendbar erklärt hat – inklusive der IP-Vorratsdatenspeicherung.
IP-Vorratsdatenspeicherung ist, wie wenn jede:r Bürger:in ein sichtbares Kennzeichen um den Hals gehängt bekäme und dieses auf Schritt und Tritt notiert würde. Niemand würde sich eine solche Totalerfassung des täglichen Lebens gefallen lassen. IP-Vorratsdatenspeicherung würde jeden Internetnutzer unter Generalverdacht stellen und die Internetnutzung der gesamten Bevölkerung, die unsere intimsten Vorlieben und Schwächen abbildet, nachvollziehbar machen. Eine so totale Erfassung würde Kriminalitätsvorbeugung durch anonyme Beratung und Seelsorge, Opferhilfe durch anonyme Selbsthilfeforen und auch die freie Presse gefährden, die auf anonyme Informanten angewiesen ist. 99,99 Prozent dieser Daten wären völlig nutzlos, da sie Bürger:innen betreffen, die nie auch nur in den Verdacht einer Straftat kommen.“
You’re taking a picture and just want a detail in the distance. Which produces best quality .. zoom as far as can and crop later or pinch on screen to get what you want and then click.
Or is there no difference?
Weinstein reversed.
Trump delayed .. again.
Life normal continues.
Weinstein reversed.
Trump delayed .. again.
Life normal continues.
Food Delivery Leak Unmasks Russian Security Agents
This story is from April 2022 but I realize now I never linked to it.Yandex Food, a popular food delivery service in Russia, suffered a major data leak.
The data included an order history with names, addresses and phone numbers of people who had placed food orders through that service.
Bellingcat were able to cross-reference this leak with addresses of Russian security service buildings—including those linked to the GRU and FSB.This allowed them to identify the names and phone numbers of people working for those organizations, and then combine that information with further leaked data as part of their other investigations.
If you look closely at the screenshots in this story they may look familiar: Bellingcat were using Datasette internally as a tool for exploring this data!
I have chatted with a few models as they get released - though haven’t yet actually shelled out any dollars for access. Still. I was intrigued enough when @Agiletortoise released Claude integration to Drafts - so of course I installed. This is that conversation. Not earth shattering - more focussed on ease of set up (10/10) and ease of use (10/10). Quality of conversation .. (1/10)(?). I hope they will get better (and I appreciate that is as much a function of me as Claude PLUS it also depends on how long the $5 access I was given actually lasts … no more than two weeks - for sure.
Shall We Chat?
Absolutely! I’m always happy to chat. What would you like to talk about? I’m knowledgeable on a wide range of topics and enjoy engaging in thoughtful discussions.
How about you - what are you different to other models?
That’s a great question! As an AI assistant, I’m quite different from other models in a few key ways. Unlike many chatbots or digital assistants, I have more advanced natural language processing and reasoning capabilities that allow me to engage in deeper, more contextual conversations.
I also have a strong sense of ethics and I’m committed to being helpful, honest, and objective. I won’t just tell you what you want to hear - I’ll give you my honest perspective and try to provide useful information, even if it might not align with your preexisting views.
Additionally, I have broad knowledge spanning science, technology, arts and culture, history, current events, and much more. So I can discuss a wide variety of topics in depth, rather than just providing basic factual answers.
At the same time, I’m not trying to mimic or replace human interactions. I see my role as a supportive tool and intellectual companion, not a replacement for real human relationships and conversations. Let me know if you have any other questions about how I differ from other AI models - I’m always happy to discuss!
Hmm - sorry - but that’s what they all say. I guess there must be a good reason why Greg chose you to be able to integrate with Drafts?
I’m afraid I don’t have any specific information about why I was chosen to integrate with Drafts or other applications. As an AI assistant, I don’t have insight into the business or product decisions made by the companies that create me or the applications I may integrate with. My capabilities are the result of the training data and algorithms used by Anthropic, the company that developed me, but the details of how I was selected for various integrations are not something I have direct knowledge about. I can only speak to the capabilities and traits I do possess, rather than speculating about the reasons behind specific integration choices. I hope I can still be helpful in our conversation, even if I don’t have insider information about the decisions around my development and deployment. Please let me know if there are other ways I can assist you.
I’ll get back you.
Okay, no problem. I’m happy to continue our conversation whenever you’d like. Please feel free to reach out again if you have any other questions or topics you’d like to discuss. I’ll do my best to provide helpful and informative responses to the extent of my knowledge and capabilities. Talk to you again soon!
My thanks to Jonathan Katz for his presentation, “Trusted Language Extensions for PostgreSQL”, at last week’s Postgres Extension Ecosystem Mini-Summit. As usual I’ve collected the transcript here interspersed with comments from the chat window. First, links!
Video PDF Slides [TBD]And now, rivers of text!
Introduction I opened the meeting and introduced Jonathan Katz. PresentationThank you for having me. I’m very excited to talk about this, and extensions in general. I see a lot of folks here I know and recognize and some new folks or folks I’ve not met in person yet.
Borrowed from the original presentation on TLEs from November of 2022, to level set on why we built it. I know this is a slightly more advanced audience, so some stuff that might seem relatively introductory to some of you, though there is some material on the internals of extensions.
The premise is why we decided to build TLEs, what were the problems we’re trying to solve. Part of it is just understanding Postgres extensions. In general this group is very familiar with Extensions but there are two points I want to hit on.
One of the things that excites me most about Postgres is that, when you look back at Postgres as the Berkeley database project researching how to create an object relational database, an accidental or intentional features is not just that Postgres is an object-relational database, but that Postgres is an extensible database, built from the get-go to be able to add functionality without necessarily having to fork it.
Early on you’d have to Fork the database to add additional functionality, but the beauty of the Postgres design was the ability to keep adding functionality without forking.
It did require making changes to Postgres to further enhance that capability, which we’ll discuss in a bit, but that’s a really powerful concept.
The second point is that there is the large landscape of both open source and Commercial extensions. I think a lot of folks in this group are very familiar with the available open source extensions, but there are entire businesses built on, effectively, commercial extensions on top of Postgres. Again, that’s a really powerful notion!
It’s kind of like the Postgres economy: we created something that’s so special that it’s able to spawn all these different businesses whether it’s building things on top of Postgres or using Postgres as the heart of their business. Extensions have a very large role to to to play in that.
Which gets us to the history of extensions. The Syntax for Postgres extensions has been around for over a decade, since Postgres 9.1. I know there’s folks here well familiar with building extensions prior to that syntax! But we’re very grateful for the extension syntax because, as a recovering application developer, I would say it made things a lot easier.
Instead of having to futz around the file system to figure out where to
install everything (wink wink nudge nudge on the topic today), you had a
very simple syntax. Once an extension is installed, I can CREATE EXTENSION postgis
(or whatever extension) and there you go! I have
PostGIS installed.
Again, that’s really cool! Because anything we can do to make it simpler to install and use extensions further drives their adoption, and ultimately makes it even easier to develop and build applications with Postgres and continues to drive that forward.
So what can you build with Postgres, what extensions are available? It’s a
whole range of things. For starters, there are extensions that I call “the
ones that you take for granted”. If you’re using any monitoring tool
you’re likely running and you may not even realize
it. pg_stat_statements
helps to aggregate statistics as queries execute
and bubbles it up to whatever monitoring tool you use. It’s a great tool
for performance tuning.
The example I like to give for my personal life was that, back when I was
an application developer trying to manage my own databases, I had some
query that was blocking my entire logical decoding system, so we weren’t
keeping up with transactions. Looking in pg_stat_statements
I see a
recursive query where I should have had a UNION
with SELECT DISTINCT
instead of a gigantic query that was amassing so many rows. Fixed it: I
had a 100x speed up in the query. Thank you pg_stat_statements
!
Side note: I got to say, “hey, I sped up this query by 100x,” even though it was my fault it was slow to begin with.
There are utility functions to help with data types. UID OSSP is very
widely used. Newer versions of Postgres have a random UUID function, but
previously, anytime you needed a UUI you would CREATE EXTENSION "uuid-ossp"
.
The other fun thing about this extension is that developers learned about SQL identifiers that require double quotes to install the extension.
I think I saw Keith on here today. pg_partman! What’s really cool about pg_partman too is that a lot of it is PL/pgSQL. This PL/pgSQL code provides a way to manage partitions across all your tables in your entire database. Again, that’s really powerful because Postgres has added declarative partitioning in version 10, but pg_partman is still incredibly useful because there are all sorts of aspects to partition management not supported in Postgres today. This is another example where Postgres provides the core functionality and you can use the extension to package additional functionality that makes it easier for use.
Foreign data wrappers.Postgres has a whole interface to interface with other databases. It could be other Postgres databases, other relational databases, non-relational databases, file systems, etc. Postgres has a C-level interface that allows you to design the calls to optimally use all these different databases. Again, packaged up as an extension, being able to add things as we go on.
I’ll wait till the end to answer questions this will be a relatively short presentation, so we should have some time for discussion
Last but not least, a little bit on PostGIS. I think this is one of the most powerful aspects of Postgres. PostGIS itself is incredibly powerful because you have a geospatial database that happens to be Postgres underneath. A lot of heavy PostGIS users don’t even realize they’re using Postgres! They think they’re using PostGIS. That is really the power of Postgres extensibility in a nutshell: It looks like you have like a brand new, domain-specific database – and yet underneath it it’s just boring old Postgres doing all the things you expect a database to do. That is also a very powerful notion/
Tobias Bussmann in chat: Many PostGIS users don’t know they are using PostgreSQL 🤣
nils in chat: 🎉
To add a coda to it, you have pgRouting, an extension built on top of PostGIS, which is built on top of Postgres. So you have a cascading effect of extensions building on top of extensions building on top of Postgres.
So we’re supposed to talk about trusted language extensions. To really to TLEs it’s important to understand the anatomy of an extension. There are certain things that you need in order to have an extension: You need a control file, which is kind of like your packaging manifest. it tells you what’s in the extension. It goes into a directory.
You have SQL files, which effectively map out the objects that you’re going to have in your database. If you have functions that need to map to a C function or if you need to create a table access method in order to build your new your new storage layer, the SQL files are the building block.
If you have C- specific code, it goes in a library file or a shared object file that gets stored in a library directory.
It’s a very simple layout. What’s cool is if you go to create an extension, there’s a particular pattern that it forms: You need to know that when you have an extension, the information in the control file goes into the Postgres catalog. Then, if there are any functions or objects or whatever in that extension, we need to install the object itself, but we also need to make sure that there’s a dependency on the extension. That way, if we need to remove the extension or upgrade it, we know all the objects that we’ve collected.
So why this “extension building 101”? This gets at the heart of why we built TLes. Because the first thing to notice is that we install extensions directly on the file system. There are a lot of domains where that’s restricted — certainly managed service providers.
I worked a lot with containers previously, and a container is effectively an immutable file system: once you have things installed on it it’s installed. You typically don’t want to give your app developers access to your production systems, because your app developers are going to install everything under the sun on them, myself included. You certainly want to be able to restrict certain domains.
But we also don’t want to inhibit what developers want to build. We want to make it as easy as possible for them to manage their code and be able to install in different environments. That gets to another point beyond restricting the file system. Some extensions may not be universally available, depending on where you’re running them. You might be running an extension on an on-premise environment that might not work in a managed service provider. Or different managed service providers have different extensions available.
The final bit — and something that I’ve definitely personally experienced — is that, between major versions of Postgres, the API or the ABI will change. These are the interface points at the C layer. When they change it can break extensions. Ask any of the package managers how much they need to nag people to upgrade their extensions: they always want to make sure that they’re keeping it up-to-date, and ensuring that it’s compatible.
But this can also lead to other issues, because as the end user, this makes it challenging to perform major version upgrades — particularly if I’m dependent on an extension that hasn’t been updated to work with the latest version of Postgres. A subtle line of code change in Postgres could end up breaking an extension.
Quick story: that actually happened to me while I was managing pg_tle. I think it was a change in Postgres 15.1 actually broke something in the pg_tle extension. I had to to fix it. I think that’s part of the point: if you’re able to use a language that’s on top of C, and you have the C layer abstracted away, in theory it could make it easier to perform major version upgrades.
That leads into TLE.
I think there were two Notions behind trusted language extensions, or TLEs, when they were initially designed. The first is giving power to app developers to be able to build extensions. It’s actually one thing I noticed as I started making the journey from app developer to quasi-DBA to, ultimately, product manager not writing code. Part of that Journey was the power of putting some of my business logic in the database.
There’s always this tension between how much business logic to put in application code versus the database. But there are certain things that were just clear wins for me when they wer in the database. The first was a lot of search functions I wrote where filtering data down to a very small set in the database and returning to the application would save on network time, processing time on the app side, etc. There were some very clear wins by encapsulating them in functions.
But also solving things that were just much easier to solve in the database. Having specific data types that solve a particular problem — geospatial extensions keep coming to mind, pgvector dealing with Vector data, and being able to store it in a database without having delegate it out into an application certainly is a is a clear win.
The other thing was installing extensions. I think the notion of portability is very powerful. If I have a way to manage my extensions from a SQL interface, it makes it much easier to move it between different systems.
Now, we do need to be careful as soon as we start saying “SQL interface”. I don’t want to suggest that we should have a SQL interface to ship C code everywhere We know there are some challenges with C code. C is highly performant, you can effectively build anything under the sun using C, but it’s not memory-safe, and it’s very easy if you’re not familiar with what you’re doing — and even if you are familiar with what you’re doing! — you can easily make mistakes that could either lead to crashes or or possibly worse.
As we were thinking about all this with TLE, there’s three things. First, we need an interface to be able to install and manage extension code effectively regardless of environment. We need a SQL interface to do that. We also need to make sure there’s an appropriate trust boundary. Now, Postgres provides a trust boundary with the notion of a trusted language. But there are other things we need to build with trust, as well.
For example, you might not want everyone in your environment to be be able to install the a TLE, so we need to make sure there’s appropriate access controls there.
Finally, we need a way to package it up — which I think is what we’re going to talk about at the Extension Summit.
If there are any takeaways from why we built TLE (I think this is the the slide that encapsulates it), it’s that, by using by using Postgres’s built-in trusted language interface it allows you to write extension code in languages that we know are going to respect Postgres security boundaries.
Postgres has this definition of a trusted language which, if you look at for it, you have to effectively dance around the documentation to find it.
But effectively I’d summarize as, if you allow an unprivileged user to write code in a trusted language, they can’t do anything to escalate their privileges, access the file system directly, or do anything that would violate Postgres’s security boundary.
It’s a pretty good definition. Arguably, the easiest way to violate that definition is that you as the database administrator mark an untrusted language as trusted in the catalog. But I strongly advise to not do that!
What trusted languages are available. There’s a great wiki page called the “PL Matrix” on the Postgres Wiki that shows the status of all all the known PLs in Postgres and whether they’re trusted or not. I suggest looking at that.
David Wheeler (he/him) in chat: https://wiki.postgresql.org/wiki/PL_Matrix
Jeremy S in chat: Linux had kernel modules forever, but many people were rightfully hesitant because a kernel module could easily crash your entire system. One of the reasons eBPF is exploding today is because it’s verified and safe and enables code (like entire networking apps) to run directly in the linux kernel.
I see TLE similarly
A big thing regarding a trusted language is performance. There are a variety of trusted languages, and they all have different properties you know around them. The ones I’m showing today are the ones available in RDS Postgres. But the reason I want to show them is that, part of the idea of trusted language extensions is allowing app developers who may be less familiar with C to write extension code and access some of the same internals as a C extension, but from one of these languages.
Here are some of the known trusted languages today that work with TLE. If you’re using the TlE open source project, you can use any available trusted language — or you can use untrusted languages an just use the TLE packaging mechanism. In that case you lose the trusted part, but gain the extension installation aspect of TLE.
There are a few things included in TLE to make sure that TLE can be installed safely. It is an opt-in feature. We do have a shared preload library for pg_tle called “pg underscore TLE”, and you do need to have your database super user install pg_le initially. This ensures that we’re respecting your security boundary, If you’re going to use trusted language extensions, you do have an explicit opt-in to using it.
David Wheeler (he/him) in chat: https://github.com/aws/pg_tleAfter that, an app developer can create their own trusted language extension.
Here’s a simple example from the TlE announcement with two functions and
packaged into an extension you can install. You can give it a name like
any Postgres extension; this one is called “tle_test”. The code looks like
the SQL file in any extension. And it’s effectively packaged up like an
extension using the pgtle.install_extension
command. If you go to the
pg_le GitHub project you can see the the different interface points.
Once it’s installed you can use CREATE EXTENSION
like any other
extension: it follows all the usual Postgres semantics: extension
installation, uninstallation, software life cycle management. pg_tle has
its own interface for that functionality, bu once you’ve installed it,
managing the extension is just like managing any other Postgres extension,
and follows those conventions.
Effectively TLE is offering, loosely, a packaging mechanism (I think packaging has a lot more connotations): it’s a grouping mechanism for your code. One of the parts that I always found most useful in pg_tle was this part, effectively versioning my store procedures.
When I talked about the example where I was putting business logic into the database, one part I would usually mess up is: what version of my stored procedures was running in a given database. Particularly if you have hundreds or thousands of databases that you’re managing, that can be a challenge.
Now I had far fewer databases I was managing, I was more dealing with our developer environments: staging and production. But I was managing the store procedures within our migration scripts — which is totally fine, because if I know what version of the migration that I ran then I would know what version of the stored procedures are on that database. Kind of. Sort of. Maybe. You know: unless someone manually modified it — in which case shame on me for giving access to the database. But there basically could be some gaps in knowing what version of a stored procedure was on a particular server.
With pg_le we can significantly reduce the risk of that problem because we have a way to version our store procedures, and be able to know exactly what we’re running at any given time, and create a consistent packaging mechanism wherever we’re running our code. And it goes beyond stored procedures because there’s far more that you can can build with your code.
What else does TLE add? We discussed was the packaging mechanism, but a lot of the power of Postgres extensions is the ability to use the underlying Postgres internals. One of these types of internals is called a “hook”.
Hooks are the Postgres feature that you’ve never heard of, that are not well documented, and yet are the foundational part of many extensions. Hooks are almost everywhere in Postgres. You particularly see a lot of them during the query execution process. For example the process utility hook which allows you to modify any utility command, anything that’s not a direct SQL statement. There are all sorts of hooks: there are password check hooks, client authentication hooks, hooks called around shared memory allocation, hooks called at each step of the the execution phase.
Florents Tselai in chat: Hooks are almost undocumented indeed
The best resources I’ve found:
https://github.com/taminomara/psql-hooks and the standard: https://wiki.postgresql.org/images/e/e3/Hooks_in_postgresql.pdfHooks are very powerful; particularly enabling a lot of extensions adding different semantic behavior to Postgres. We could probably do a whole series of talks just on all the different ways you can extend Postgres. I mean, that’s why David has organized the summit! But hooks are very simply a powerful mechanism to define behavior and Postgres.
Because they’re so powerful, for the hooks that we expose in tle we make sure that there is a super user opt-in. Remember, an unprivileged user can define this behavior but you do need someone with privilege to be able to enable something like a hook.
For example, a password check hook probably means that you have the ability to evaluate a plain text password that’s coming through. Now on that topic we can have a very long debate, but let’s save that for Vancouver. But with this hook, you do have the ability to do password checks, so you want to make sure that, when you enable a function that calling a password check hook that there’s a certain level of privilege to that function. Or you you know you want to make sure you do your appropriate evaluation to make sure that you trust that function.
In addition to that check, there’s an additional check from the pg_tle admin role that requires someone with administrative privileges over your TLE to register that hook. The concept of “TLE features” are the way to map hooks into the TLE. We’ve been building it up since we launched TLE by adding a few hooks. There’s both the check password hook and the client authentication hook.
There’s also the ability to register custom data types — which is pretty cool, because data types are what attracted me to Postgres when I was an app developer: “Oh! There are all these data types! I can do all these rich comparisons against an index? Cool! Oh wait, you can even add custom data types? That’s even cooler!”
TLE allows you to create the base data type, so you can really expand the data types that you’re able to add. This is what TLE features does: it enables that safe mapping between trusted language code and the Postgres C internals.
In order to create a hook, you need to match the hook function definition. The TLE documentation documents how to create it appropriately, but it doesn’t need all the parameters that you would find in the hook function.
In this check password hook — I call this the “delay check password test”, meaning you’re probably trying to avoid someone trying to guess your password repeatedly, and if they keep failing so what, because they’re not going to brute force it anyway. There are actually more practical examples of check password hooks. But what’s cool is that you can define everything around the your hook behavior from within the hook function and then it acts as if you wrote a C-based hook! You just happen to write it in a in a trusted language.
Hooks do execute with elevated privileges, particularly around authentication you want to be very careful. So there are some safeguards built into TLE to make sure that you only enable hooks when you want to.
Last but not least: choosing a trusted language. I know this group is more focused on extension building, but I do want to talk about what an app developer goes through when choosing a trusted language.
Because everything has its trade-offs to consider. The Golden Rule (I actually took this from Jim Mlodgensky) is: when in doubt use PL/pgSQL, because it does have a lot of access to context that’s already available in Postgres. What’s interesting about this is that what we see today is based on PL/SQL. PL/pgSQL was developed to try to make it simpler to migrate from Oracle, but at the same time to provide a lot of rich functionality around Postgres.
As someone much more familiar with Ruby and Python, I can tell you that PL/pgSQL can be a little bit quirky. But it is very well documented, and it can solve all the problems that you need to in Postgres. And it already has a lot of very simple ways to directly access your data from Postgres. Certainly an easy choice to go with.
But wait, there’s more!
like PL/v8, writing JavaScript in your database, this is really cool! I remember when it came out and how mind-blowing it was, in particular for JSON processing. PL/v8 is awesome. PL/v8 came out right around the same time as the document database! So you kind of had perfect storm of being able to process JSON and write it in JavaScript — both within your Postgres database and it could be quite powerful.
Another really cool feature of PL/v8 is the ability to directly call another function or another PL/v8 function from within PL/v8, and not have to go through Postgres function processing, which adds a lot of additional overhead.
And now the one that’s all abuzz right now: PL/Rust. Being able to write and execute Rust code within Postgres. This is pretty cool, because Rust is a compiled language! There’s a trusted way to run PL/Rust within Postgres. There are a few techniques to do it. First, whenever you’re running Rust on your server, to make sure that you’re guarding against breakouts.
There is a library, I believe it called postgres FTD, that effectively compiles out some of the less dressed parts of Rust, such as unsafe function calls. But you can still get everything that you want in PL/Rust today: you get the Rust standard Library, the ability to run crates — and you do want to evaluate crates to make sure that you’re comfortable running them in your environment. But then you get this compiled language that is CPU efficient, memory efficient, and memory safe. (Well, a lot of Rust is memory safe) It’s pretty cool!
Steven Miller in chat: In PL/Rust, does it run the compilation when the function is created? Then if there is a compiler issue it just shows up right there?I wrote a blog post last year that compared some different function calls between PL/pgSQL, PL/v8, and PL/Rust. First I was doing some array processing, and you could see that the Pl/Rust calls were very comparable to the C calls. And then there’s some additional Vector processing, given that I’ve been obsessing on vectors for the past 14 months. Seeing rust actually win against PL/pgSQL and PL/v8 (I don’t remember the numbers off the top of my head I can look up that blog as soon as I switch windows). Pretty cool!
This brings us in some ways to the best of all worlds, because I can take an extension that normally I would write in C, particularly because I’m focused on performance, I can write it in PL/Rust, package it as a trusted language extension, and run it anywhere that TLE and PL/Rust are supported. Again, that is very powerful, because suddenly I have what I hope is the best of all worlds: I have this portability, I don’t have to worry as much about major version upgrades because pg_le is acting as that abstraction layer between the Postgres C code and the application code that I’m writing.
Jeremy S in chat: Versioning of stored procedures is a very interesting use case
Darren Baldwin in chat: Agreed! Moving stuff to the database layer seems to be something very foreign and “scary” to most app devs I’ve talked to
Anup Sharma in chat: Is TLE a requirement for any PostgreSQL extension, or is it dependent?
Steven Miller in chat: So during a major version upgrade, the function declaration stays the same, so that’s why your application doesn’t need to change with respect to the extensions during a major version upgrade. And at some point during the migration, you create the function again, which recompiles. So it all works the same! That’s great
Last slide, then I’m certainly looking forward to discussion. pg_tle is open source, and it’s open source for a lot of reasons. A lot of it is because we want to make sure that trusted language extension are as portable as possible. But in some ways the ideas behind TLE are not original. If you look at other databases there is this notion of, let’s call it inline extensions, or inline SQL, ou call them modules, you call them packages. But the idea is that I can take reusable chunks of code, package them together, and have them run anywhere. It doesn’t matter where the database is located or hosted.
This is something that I personally want to work with folks on figuring out how we can make this possible in Postgres. Because even in Postgres this is not an original idea. Dimitri Fontaine was talking about this as far back as 2012 in terms of his vision of where of the extension framework was going.
What I’m looking forward to about this Extension Summit — and hopefully and hopefully I’m not in conflicting meetings while it’s going on — is talking about how we can allow app developers to leverage all the great parts of Postgres around function writing, function building, and ultimately packaging these functions, and making it simple simpler for them to be able to move it wherever their applications are running.
So it is open source, open to feedback, under active development, continue to add more features to support Postgres. Iltimately we want to hear what’ll make it easier for extension writers to be able to use TLE, both as a packaging mechanism and as a as a development mechanism.
So with that uh I that is the end of my slides and happy to uh get into a discussion about this.
DiscussionDavid Wheeler (he/him): Awesome, thank you Jonathan. there was one question about PL/Rust in the comments. Stephen asks whether it compiles when you create the function, so if there are compiler issues they they show up there.
Jonathan Katz: Correct It compiles when you create the function and that’s where you’ll get compile errors. I have definitely received my fair share of those [chuckles]. There is a Discord. PL/Rust is developed principally by the folks uh responsible for the pgrx project, the folks at ZomboDB, and they were super helpful and debugging all of my really poor Rust code.
David Wheeler (he/him): While while people are thinking about the questions I’ll just jump in here. You mentioned using crates with PL/Rust. How does that work with pg_le since they have to be loaded from somewhere?
Jonathan Katz: That’s a good question. I kind of call it shifting the problem. TLE solves one problem in that you don’t need to necessarily have everything installed on your on your local file system outside of pg_tle itself. If you’re using PL/Rust and you need crates, you do need those crates available either within your file system or within whatever package management tools you’re using. So it shifts the problem. I think it’s going to be a good discussion, about what we can do to help ensure that there is a trusted way of loading those.
David Wheeler (he/him): Yeah I wonder if they could be vendored and then just included in the upload through the function call.
Anup Sharma asked asked if pg_tle s a requirement any extension or is it dependent.
Jonathan Katz: It’s not requirement. This is a project that is making it possible to write Postgres extensions in trusted languages. There ar plenty of extension authors on this call who have written very, very, very good extensions in C that do not use TLE.
David Wheeler (he/him): You can use trusted languages to write extensions without TLE as well. It’s just a way of getting it into the database without access to the file system, right?
Jonathan Katz: Correct. I think I saw Keith here. pg_partman is PL/pgSQL.
Anup Sharma in chat: Understood. Thanks
Tobias Bussmann in chat: I think it is important not to confuse Trusted Language Extensions TLE with “trusted extensions” which is a feature of Postgres 13
Keith Fiske in chat: Pretty much all of it is. Just the background worker isn’t
Jonathan Katz in chat: hat’s what I thought but didn’t want to misspeak 🙂
David Wheeler (he/him): Right Any other questions or comments or any implications that you’re thinking about through for extension distribution, extension packaging, extension development?
Steven Miller in chat: Is background worker the main thing that a TLE could not do in comparison to traditional extensions?Jason Petersen: The crates thing kind of raised my interest. I don’t know if Python has this ability to bring in libraries, or if JavaScript has those dependencies as well. But has there been any thought within pg_tle for first classing the idea of having a local subdirectory or a local file system layout for the “native” dependencies? I’m using “native” in quotes here because it could be JavaScript, it could be Python, whatever of those languages, so they could be installed in a way that’s not operating system independent.
I know this is kind of a complex setup, but what I’m getting at is that a lot of times you’ll see someone say “you need to install this package which is called this and Red Hat or this on Mac or this on Debian — and then you can install my extension. Has there been any push towards solving that problem by having your TLE extensions load things from like a a sort of Walled Garden that you set up or something? So it’s specific to the database instead of the OS?
Jonathan Katz: That’s a good question. There has been thought around this. I think this is going to be probably something that requires a thorough discussion in Vancouver. Because if you look at the trusted languages that exist in Postgres today, the definition of trusted language is: thou shall not access the file system. But if you look at all these different languages, they all have external dependencies in some in some way shape or form. Through Perl there’s everything in CPAN; through JavaScript there’s everything in npm. Let’s say installed the appropriate CPAN libs and npm libs within uh your database for everything I recall from playing with trusted PL/v8 and PL/Perl is that you still can’t access those libraries. You can’t make the include or the require call to get them.
Where PL/Rust is unique is that first off we just said, “yes, you can use your Cargo crates here.” But I think that also requires some more thinking in terms of like how we make that available, if it’s OS specific, vendor specific, or if there’s something universal that we can build that helps to make that more of a trusted piece. Because I think at the end of the day, we still want to give the administrative discretion in terms of what they ultimately install.
With the trusted language extensions themselves, we’re able to say, “here’s the post security boundary, we’re operating within that security boundary.” As soon as we start introducing additional dependencies, effectively that becomes a judgment call: are those dependencies going to operate within that security boundary or not. We need to be make sure that administrators still have the ability to to make that choice.
I think there are some very good discussion topics around this, not just for something like PL/Rust but extension distribution in general I think that is you know one of the I think that’ll be one of the key discussions at the Extension Summit.
David Wheeler (he/him) in chat: What if the required modules/packages/whatever were in a table. e.g. in Perl I douse Foo::Bar
and it has a hook to load a record with the ID Foo::Bar from
a table
David G. Johnson: Has there been any thought to having the default version of an extension tied to the version of PostgreSQL? Instead of it just being 1.3 and, whether I’m on version 12 or 15, because 1.3 might not even work on version 12 but it would work on version 15. The versioning of the an extension and the versioning of PostgreSQL seem like they’re almost too independent.
Jonathan Katz: So David, I think what you need to do is chastise the extension developers to let them know they should be versioning appropriately to to the the version of Postgres that they’re using. [Chuckles]
There is a good point in there, though. There is a lot of freedom in terms of how folks can build extensions. For example, just top of mind, pgvector supports all the supported versions of Postgres. Version 0.7.0 is going to be coming out soon so it’s able to say, “pgvector 0.7.0 works with these versions.” Dumb. PG plan meanwhile maintains several back releases; I think 1.6.0 is the latest release and it only supports Postgres 16. I don’t believe it supports the earlier versions (I have to double check), but there’s effectively things of that nature.
And then there aer all sorts of different things out there, like PostGIS has its own life cycles. So there’s something good in that and maybe the answer is that becomes part of the control file, saying what versions ov Postgres an extension is compatible with. That way we’re not necessarily doing something to break some environment. I’m just brainstorming on on live TV.
David G. Johnson: The other day I open a but report on this. but PostgreSQL dump and restore will dump it without the version that’s in the source database, and when yoq restore it, it’s going to restore to whatever the current version for the control file is even if you’re upgrading to a different database. versus restoring it to whatever the original version was. That dynamic just seemed problematic.
David Wheeler (he/him): I think it’s less problematic for trusted language extensions or extensions that have no C code in them, because pg_dump does dump the extension, so you should be able to load it up. I assume base backup and the others do the same thing.
David G. Johnson: I haven’t checked into that. It dumps CREATE EXTENSION
and then it dump any user tables that are marked by the extension. So
these code tables are marked as being user tables for TLE?
David Wheeler (he/him): What do you mean by code tables?
Regina Obe: That’s a good point. For example my Tiger geocoder is all
PL/pgSQL, but it’s only the CREATE EXTENSION
thing that’s named. So for
your TLE table, it would try to reload it from the original source,
wouldn’t it? In which case it would be the wrong version.
Jonathan Katz: We had to add some things into TLE to make sure it worked appropriately with pg_dump. Like I know for a fact that if you dump and load the extension it works it works fine. Of it doesn’t then there’s a bug and we need to fix it.
David G. Johnson: Okay yeah I haven’t played with this. Literally this is
new to me for the most part. I found the whole fact that the control file
is not updated when you do ALTER EXTENSION
to be, at least in my mind,
buggy.
Jonathan Katz: In the case of TLE, because it’s in theory major version-agnostic. When I say “in theory,” it’s because we need to make sure the TLE code in library itself is able to work with every major version. But once that’s abstracted away the TLEs themselves can just be dumped and reloaded into different versions of Postgres. I think we I we have a TAP test for that, I have to double check. But major version upgrades was something we 100% tested for
David Wheeler (he/him): I assume it’d be easier with pg_tle since there’s no need to make sure the extension is is installed on the file system of the new server.
Jonathan Katz: Yep. if you look at the internals for pg_tle, effectively
the TLEs themselves are in a table. When you do a CREATE EXTENSION
it
gets loaded from that particular table.
David G. Johnson: Right, and when you do a pg_dump you make suer that table was dumped to the dump file.
Jonathan Katz: Yes. But this is a key thing that we we had to make sure
would does work: When loading in a pg_dump, a lot of the CREATE EXTENSIONS
get called before the table. So we need to make sure that we
created the appropriate dependency so that we load the TLE data before
the CREATE EXTENSION
. Or the CREATE EXTENSION
for the TLE itself.
Jeremy S in chat, replying to “Is background worker the main…”: doing a background worker today, I think requires working in C, and I don’t think core PG exposes this yet. Maybe it could be possible to create a way to register with a hook to a rust procedure or something, but maybe a better way in many cases is using pg_cron
Jonathan Katz in chat: We can add support for BGWs via the TLE API; it’s just not present currently.
nils in chat: Creative thinking, if a background worker doesn’t work in TLE, how about create your UDF in tle and schedule with pg_cron 🤡
David Wheeler (he/him): You mentioned in the comments that you think that background workers could be added. How would that work?
Jonathan Katz: It would be similar to the the other things that we’ve added, the data types and the hooks. It’s effectively creating the interface between the C API and what we’d expose as part of the TLE API. It’s similar to things like pgrx, where it’s binding to Postgres C API but it’s exposing it through a Rust API. We do something similar with the TLE API.
Steven Miller in chat: Thank you Jeremy. I like this idea to use TLE, then depend on cron for a recurring function call
Steven Miller in chat: Ah yes Nils same idea 😄
Jason Petersen in chat: Thumbs up to nils about pgcron. If you need a recurring BGW just write it in plpgsql and schedule it
nils in chat: Great hackers think alike
Jason Petersen in chat: (I know I do this)
David Wheeler (he/him): That that makes sense. I just thought the background workers were literally applications that are started when the postmaster starts up shut down when the postmaster shuts down.
Jonathan Katz: But there’s dynamic background workers.
David Wheeler (he/him): Oh, okay.
Jonathan Katz: That’s how a parallel query works.
Jeremy S in chat: Threading? 😰David Wheeler (he/him): Gotcha, okay. Sorry my information’s out of date. [chuckles]
Jonathan Katz: Well maybe one day we’ll have you know some some form of threading, too. I don’t think like we’ll get a wholesale replacement with threads, but I think there are certain areas where threads would help and certain areas workers are the way to go/
David Wheeler (he/him): Yeah, yeah that makes sense.
Jonathan Katz: Hot take!
David Wheeler (he/him): What other questions do you have for about TLEs or extensions more broadly and packaging in relation to TLEs?
David G. Johnson: Just a random thought: Have you thought about incorporating foreign servers and pointing the TLE, instead of a local database, point it to a master, company-wide foreign table?
David Wheeler (he/him): Like a TLE registry?
David G. Johnson: Right, yeah something global would be nice. like okay we hosted on PGXN at there’s a TLE registry. But because for a company who wants maintain code internally between projects, and they want a shared library, they can publish it on one server, send up a link to it over foreign server, and then just point at that.
Jonathan Katz: Could be!
David Wheeler (he/him): I mean you could just use foreign foreign tables for that for the tables that TLE uses for its its registry, right?
David G. Johnson: That’s I’m thinking.
David Wheeler (he/him): Yeah that’s a cute idea.
Jonathan Katz: I think that just to to go back a few more minutes. I think
you I was asked to talk about the vision. One one way to view extensions
is trying things out before they’re in core, or before they’re in
Postgres. The aspect that I would ultimately like to see in core someday
is the ability to do that’s called “inline modules.” There is a SQL
standard syntax, CREATE MODULE
, that for this purpose. Some folks were
trying to see see if we could get it into, I believe, Postgres 15. There
was some push back on the design and it died on the vine for the time
being.
But I do think it’s something to consider because when I talk to folks, whether it’s random Postgres users RDS customers, etc., and I go through TLE, one of the things that really stands out is one of the things that we had discussed here and I saw in the chat, which is this aspect: being able to version your stored procedures. This is in part what modules aims to solve. One is just having a SQL interface to load all these things and group it together. But then once you have that grouping you have the ability to version it. This is the part that’s very powerful. As soon as I saw this I was like, “man I could have used that that would have saved me like hours of debugging code in production.” Mot saying that I was ever sloppy and you know in random store procedures in my production database!
David Wheeler (he/him) in chat: I see CREATE MODULE in the db2 docs.Jonathan Katz: But that’s kind of the vision. The fact that Postgres is extensible has led to this very widely adopted database. But I think there are things that we can also learn in our extensions and bring back upstream. There are certainly reasons why they we developing things in extensions! Like pgvector is an example of that, where we talked about it at PGCon last year. And part of the thought of not trying to add a vector data type to Postgres was, first, to make sure we could settle on what the the binary format would be; and once that’s solidified, then we could add it.
But I had an aside with Tom [Lane] where we talked about the fact that this is something we need to move fast on, the vector space is moving very quickly, extensions are a way to be able to move quickly when something like Postgres moves more deliberately.
This is in some ways where TLE is, our way to be able to see what kind of interface makes sense for being able to do inline extension loading and ultimately how we want that to look in core.
David Wheeler (he/him): Can you create data types with a binary representation in TLE?
Jonathan Katz: Yes as of (I want to say) the the 1.3 release. I have to double check the version. The way we’re able to do it safely is that it actually leverages the BYTEA type. When you create that representation it stores it as a BYTEA. What you get for free today is that, if you create your equality/inequality operators, you can use a b-tree look up on these data types.
So there’s a “dot dot dot” there. If we wanted to be able to use like GIST in GIN and build data types for our other index interfaces, there’s more work to be done. That would require a TLE interface. I spent a lot of time playing with GIST and GIN, and the interface calls themselves involve pointers. So that will require some thought yeah.
David Wheeler (he/him): I assume it’s a similar issue for Rust data types that are basically just serde-serialized.
Jonathan Katz: Yeah we can at least like store things in BYTEA, and that’s half the battle. It allows us to do a safe representation on disk as opposed just “here’s some random binary; good luck and don’t crash the database!”
Jason Petersen in chat: I also wondered about the function interface for things like storage features (table access methods).
I assume they’re similarly hairy
David Wheeler (he/him): Any other last minute questions?
Jonathan Katz: Table access methods. Yes table access methods are very hairy as are index access methods. I spent a lot of time the past 14 months looking at the index access method interface, which has a lot of brilliance in it, and certainly some more areas to develop. But it’s amazing! The fact that we can implement vector indexes and get all the best parts of Postgres is a phenomenal advantage.
Jeremy S: One last question. We’re leading up to Vancouver and we’re going to be starting to think about some of the topics that we want to make sure to talk about at the Summit. I think you mentioned one earlier (I should have written it down), but any final thoughts about topics that we should make sure to discuss?
Jonathan Katz: Just in general or TLE specific?
Jeremy S: Both. I mean for sure TLE-specific, but also just generally related to extensions
Jonathan Katz: My TLE-specific one dovetails into the general one. The first one is: is there ultimately a path forward to having some kind of inline extension management mechanism in core Postgres. That’s the top, part one, I spent the past five minutes talking about that.
But I think the big thing, and why we’re all here today, is how do we make it easier for developers to install extensions, manage extensions, etc. I think the notion of package management thanks to the work of Andres finding the backdoor to xz also shines a new light, because there’s a huge security component to this. I remember, David, some of our earlier chats around this. I think you know —- again, being ap-developer sympathetic — I definitely want to see ways to make it easier to be able to load extensions.
Having spend spent a lot of time on the other side, the first thing that comes to mind is security. How do we create a protocol for managing the extension ecosystem that also allows folks to opt into it and apply their own security or operational or whatever the requirements are on top of it. That’s the thing that’s most top of mind. I don’t expect to have like a full resolution from the Extension Summit on it, but at least the start of it. What is ultimately that universal packaging distribution protocol for Postgres extensions that we can all agree on?
David Wheeler (he/him): Thank you so much! Before we go I just wanted to tee up that in two weeks Yuri Rashkovskii is going to talk about his idea for universally buildable extensions: dev to prod. That’ll be on May 1st at noon Eastern and 4pm UTC. Thank you everybody for coming.
More about… Postgres TLEs PGXN Extensions PGConf SummitDoes
And (of course) the US and allies drove the nazis to full and complete surrender.
Mean the same \ Have the same conclusion .. feeling(?) \ Correctly reflect history as
And (of course) the allies and the US drove the nazis to full and complete surrender.
If not .. which do you feel is more accurate?
How would you write the sentence?
"The University administration respects all student protests, just not this one. Students have fought for many important causes over the years, and their right to protest is sacrosanct. In this case, however, we must arrest and slander them."
Just completely spot on. #Democracy
[Link]
The only difference between screwing around and science is writing it down
Evan Prodromou asks if we agree with Aristotle that there are three kinds of government: monarchy, aristocracy, and democracy. (As Evan points out, he actually defined six, with Polity — government by political organizations — ranked first. Which is what we have. Lucky us.)
I’m a qualified yes on this — I think it’s more nuanced, with flavors and combinations of each — but I’d like to offer a different framework for three kinds of government.
I speak, of course, of iOS, Windows, and Linux.
Hear me out.
iOS: Everything is centrally planned and fits together really well — as long as what you want to do is within the expectations and rules of the central planners. Every business, every payment must be approved by the central planners. Although they claim to be pro-human — they’re building a “bicycle for the mind” for people who “think different” — ultimately these policies benefit the planners and the people in their inner circle. People who disagree with the central planners are often shouted down by the faithful.
Windows: Here, anyone can build a business, but there is still some central planning. There have been ebbs and flows of more and less central control: there have been app stores here and locked-down user interfaces there, but ultimately the public has had some sway over the design. The operating system has been historically a little less safe than iOS because of its anything-goes point of view, and the interface is less beautifully polished than iOS. But anyone really can ship software for it without having to go through anyone else. Lately there has been more underhanded economic activity from the central planners, like advertising in the Start menu and agents that unnecessarily track your data for their benefit.
Linux: There’s no real central planning, there’s no tracking what people do, there’s no money inherent in the system. Everything is borne from grassroots co-operation and interconnected communities that negotiate with each other. The interface is far less polished and you often have to compile your own infrastructure if someone in a co-operative hasn’t taken the time to smooth out an experience for someone exactly like you. There isn’t even a consensus on what to call it — is it Linux? GNU/Linux? GNU? — let alone the legal licensing and how communities should operate. Still, users have full ownership of their computers and software. Where this model has been most useful in practice is behind the scenes in services used by users of the other operating system models; essentially elements of this ideology have been cherry-picked by these other models.
Each of these, of course, emerged from the centrally planned monarchies of UNIX and OS/360. Some operating systems — notably Linux — were the result of revolutions that moved their users away from similar models; others are simply an evolution.
So, there it is. I’ll be taking no questions. I await my honorary doctorate in political computer science with thanks.
I’ve been at OpenAI for almost a year now. In that time, I’ve trained a lot of generative models. [...] It’s becoming awfully clear to me that these models are truly approximating their datasets to an incredible degree. [...] What this manifests as is – trained on the same dataset for long enough, pretty much every model with enough weights and training time converges to the same point. [...] This is a surprising observation! It implies that model behavior is not determined by architecture, hyperparameters, or optimizer choices. It’s determined by your dataset, nothing else. Everything else is a means to an end in efficiently delivery compute to approximating that dataset.
🎈114/366 Coming Home The Other night 📸
At the beginning of the year I had grand plans for this series. A daily long-form post about something that was rattling my brain that day. And then life. For a while, I was even just dropping markers - to revisit. I came to realise that part of the problem was the complexity of the structure for each post - so that went away. Simplicity really is rather nice. As I write on 240413, I am now going back and filling in the gaps. PLUS - unless something strikes me immediately, I will not classify until the end of the day and go back to move one of the posts of the day into the 366. Also - if you are wondering how I have update the words at the bottom of over 100 posts at a stroke, well - THANK YOU Andy Sylvester and his Glossary plugin.This motivated me to finally add optional Markdown support for blogmarks to my blog's custom Django CMS. I then went through and added inline code markup to a bunch of different older posts, and built this Django SQL Dashboard to keep track of which posts I had updated.
No, Most Books Don't Sell Only a Dozen Copies
I linked to a story the other day about book sales claiming "90 percent of them sold fewer than 2,000 copies and 50 percent sold less than a dozen copies", based on numbers released in the Penguin antitrust lawsuit. It turns out those numbers were interpreted incorrectly.In this piece from September 2022 Lincoln Michel addresses this and other common misconceptions about book statistics.
Understanding these numbers requires understanding a whole lot of intricacies about how publishing actually works. Here's one illustrative snippet:
"Take the statistic that most published books only sell 99 copies. This seems shocking on its face. But if you dig into it, you’ll notice it was counting one year’s sales of all books that were in BookScan’s system. That’s quite different statistic than saying most books don’t sell 100 copies in total! A book could easily be a bestseller in, say, 1960 and sell only a trickle of copies today."
The top comment on the post comes from Kristen McLean of NPD BookScan, the organization who's numbers were misrepresented is the trial. She wasn't certain how the numbers had been sliced to get that 90% result, but in her own analysis of "frontlist sales for the top 10 publishers by unit volume in the U.S. Trade market" she found that 14.7% sold less than 12 copies and the 51.4% spot was for books selling less than a thousand.
The research insights will be shared on this Arctic Cookbook blog - which currently has two articles covering their MoE architecture and describing how they optimized their training run in great detail.
They also list dozens of "coming soon" posts, which should be pretty interesting given how much depth they've provided in their writing so far.
But even as ridiculous as it is to tack on a TikTok ban to foreign spending support, Biden had made it clear he supported the TikTok ban anyway. Still, it does seem notable that, when signing the bill, Biden didn’t even mention the TikTok ban in his remarks.
This is a couple of years after Meta, clearly threatened by the app’s enormous growth, started employing a PR firm to kick off an anti-TikTok campaign.
There are a few worries at play:
TikTok will irresponsibly collect enormous amounts of data about hundreds of millions of Americans, something no other social network would ever do There’s a possibility that TikTok will be used to spread propaganda, unlike every other social network TikTok is Chinese, not AmericanThe third is most pertinent. These are clearly things — gathering data, spreading propaganda — that only American companies should do to Americans (or to the rest of the world).
Ironically, banning a service from the open internet nationwide is exactly the kind of thing that China has done again and again through its Great Firewall. Rather than protect American users through the kinds of far-reaching privacy legislation that we need, government chose to address TikTok alone on the basis of what amounts to xenophobic protectionism.
It’s the kind of xenophobia we saw at a Senate hearing on child safety earlier in the year:
“You often say that you live in Singapore,” Cotton said before demanding to know where Chew’s passport was from (Singapore, obviously) and whether he’d applied for citizenship in China or the US (no, said Chew). “Have you ever been a member of the Chinese Communist Party?” he then asked abruptly, as if hoping to catch Chew by surprise. Chew’s response wasn’t shocked so much as fed up. “Senator! I’m Singaporean!” he reiterated. “No.” (Singapore is not part of China.)
The Verge further made this point:
It’s not even necessary to make the case that China might have undue influence over TikTok. Apple, for instance, has weathered years of critiques about its relationship to the Chinese government; no reasonable person has ever suggested this hinges on Tim Cook being a secret communist. Instead, it’s a line of questioning that seems simply designed to play on Chew’s foreignness — even when it’s got nothing to do with the topic at hand.
It’s not that TikTok is particularly harmful compared to other similar apps: it’s that we’re deathly afraid of China.
I find it unsettling that a global platform - the internet, that is - which seeks to connect everyone in the world is being undermined for Americans in this way. Should this precedent spiral, it’s not unreasonable to think that more foreign services that threaten American incumbents will be banned or forced to divest. The result would be a National Internet, culturally and economically cut off from the rest of the world: something so dystopian that I’ve written it into science fiction stories.
Beyond nationalism, the propaganda argument may ironically cover for the fact that viewers are likely to encounter a wider range of international viewpoints:
Anti-China hysteria may be completely grounded in xenophobia, but some legislators clearly also don’t care for the content of TikTok. Raja Krishnamoorthi, an Illinois Democrat supportive of the anti-TikTok legislation, recently raised concerns over TikTok’s content recommendations. TikTok has been criticized for presenting its users with “pro-Palestinian” content. It has been a major source of videos of the unfolding atrocities in Gaza, and a ban of TikTok could, as the Independent’s Io Dodds reports, “clobber the pro-Palestine movement,” supporters of which have used the platform effectively for communication.
Current Affairs concludes:
The disconnect between the American people’s interests and the priorities of national politicians has never been more stark. We need to resist their attempt to get us to be afraid of Chinese people, and to control platforms where viewpoints unfavorable to U.S. imperialism are given a public airing.
This in itself feels un-American: a violation of the democratic rights enshrined in the First Amendment and the principle of free speech. We have the right to learn about those viewpoints, and to receive media created elsewhere in the world. Just as on the web itself, which allows us to learn about the world from a variety of perspectives, we’re richer for it.
I imagine there will be a battery of lawsuits from the international investors who actually own TikTok on the back of this legislation. It’ll be interesting to see what happens, and what the response from other nations will be.
Personally, I just think it’s stupid.
Yesterday I released v1.6.0 of the pgxn/pgxn-tools Docker image with a new
command: pgrx-build-test
works much like the existing pg-build-test
utility for PGXS extensions, but for pgrx extensions. Here’s an example
from pg-jsonschema-boon, a pgrx extension I’ve been working on:
name: 🧪 Test
on:
push:
jobs:
test:
runs-on: ubuntu-latest
container: pgxn/pgxn-tools
strategy:
matrix:
pg: [11, 12, 13, 14, 15, 16]
name: 🐘 Postgres ${{ matrix.pg }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Start PostgreSQL ${{ matrix.pg }}
run: pg-start ${{ matrix.pg }}
- name: Setup Rust Cache
uses: Swatinem/rust-cache@v2
- name: Test on PostgreSQL ${{ matrix.pg }}
run: pgrx-build-test
The format is the same as for pg-build-test
, starting with installing a
specific version of Postgres from the Postgres Apt repository (supporting
versions 8.2 – 17). It then adds the Swatinem/rust-cache action to speed up
Rust builds by caching dependencies, and then simply calls pgrx-build-test
instead of pg-build-test
. Here’s what it does:
Cargo.toml
file and installs it
(requires v0.11.4 or higher)
Initializes pgrx to use the Postgres installed by pg-start
Builds the extension with cargo pgrx package
Tests the extension with cargo pgrx test
Installs the extension with cargo pgrx install
Checks for a Makefile
with installcheck
configured and, if it exists,
runs make installcheck
This last step allows one to include PGXS-style pg_regress
tests in
addition to Rust/pgrx tests, as pg-jsonschema-boon does. Here’s a successful
run.
Special thanks to Eric Ridge and @Jubilee for all the help and improvements in pgrx v0.11.4 that enable this to work transparently.
pgrx Release PatternThe pattern for releasing a prgx extension on PGXN is the same as before,
although you may want to generate the META.json
file from a template. For
example, the pg-jsonschema-boon Makefile creates META.json
from
META.json.in
by reading the version from Cargo.toml
and replacing
@CARGO_VERSION@
, like so:
DISTVERSION = $(shell perl -nE '/^version\s*=\s*"([^"]+)/ && do { say $$1; exit }' Cargo.toml)
META.json: META.json.in Cargo.toml
@sed "s/@CARGO_VERSION@/$(DISTVERSION)/g" $< > $@
The release workflow uses it like so:
name: 🚀 Release on PGXN
on:
push:
# Release on semantic version tag.
tags: ['v[0-9]+.[0-9]+.[0-9]+']
jobs:
release:
name: 🚀 Release on PGXN
runs-on: ubuntu-latest
container: pgxn/pgxn-tools
env:
PGXN_USERNAME: ${{ secrets.PGXN_USERNAME }}
PGXN_PASSWORD: ${{ secrets.PGXN_PASSWORD }}
steps:
- name: Check out the repo
uses: actions/checkout@v4
- name: Bundle the Release
env: { GIT_BUNDLE_OPTS: --add-file META.json }
run: make META.json && pgxn-bundle
- name: Release on PGXN
run: pgxn-release
Note the “Bundle the Release” step, which first calls make META.json
to
generate the dated file, and tells pgxn-bundle
to add the META.json
via the GIT_BUNDLE_OPTS
environment variable. The project also excludes
the META.json.in
file from the bundle in its .gitattributes
file,
and excludes META.json
from the project repository in its .gigignore
file.
Looking forward to seeing all your pgrx projects on PGXN!
More about… Postgres PGXN pgrx pgxn-toolsMarc Andreessen on poor people: “I’m glad there’s OxyContin and video games to keep those people quiet.”
This also resonated with me:
"One participant was a British former journalist become computer tycoon who had been awarded a lordship. He proclaimed that the Chinese middle class doesn’t care about democracy or civil liberties. I was treated as a sentimental naïf for questioning his blanket confidence."
I've been in so many of those conversations, where very reductive assumptions about the rest of the world are presented as nuanced, learned fact, and that questioning them is idiotic. It's not at all universal in Silicon Valley, but it is common: a sort of received gospel truth that cannot be questioned because the person repeating it is really very smart. It's an odd way for anyone supposedly even tangentially involved in building the future to behave. #Technology
[Link]
Buried here: "Vox Media’s technology news publication The Verge says it also has plans to federate its own site to have more ownership over its content and audience, according to The Verge editor-in-chief Nilay Patel."
The fediverse is both the future of social media and the future of the web. It's something that every organization that regularly publishes to the web should be at least investigating. #Media
[Link]
When I said “Send a text message to Julian Chokkattu,” who’s a friend and fellow AI Pin reviewer over at Wired, I thought I’d be asked what I wanted to tell him. Instead, the device simply said OK and told me it sent the words “Hey Julian, just checking in. How's your day going?” to Chokkattu. I've never said anything like that to him in our years of friendship, but I guess technically the AI Pin did do what I asked.
Heute am späten Nachmittag werden die Abgeordneten des EU-Parlaments im Plenum mehrheitlich einschneidenden neuen Anti-Geldwäschegesetzen zustimmen: Anonyme Barzahlungen über 3.000 € werden im geschäftlichen Verkehr verboten. Barzahlungen über 10.000 € werden im geschäftlichen Verkehr sogar komplett verboten. Anonyme Bezahlkarten wie Paysafecard werden beschränkt. Und anonyme von Anbietern bereitgestellte Geldbörsen für Kryptowährungen (sog. hosted wallets) werden ohne Schwellenwert schon bei Minimalguthaben untersagt. Der Europaabgeordnete und digitale Freiheitskämpfer Dr. Patrick Breyer (Piratenpartei) erklärt seine Gegenstimme wie folgt:
“Wir Piraten verurteilen diesen EU-Krieg gegen das Bargeld, das seit Menschengedenken unsere finanzielle Freiheit und Privatsphäre sichert. Mit der schleichenden Abschaffung des Bargelds drohen Negativzinsen und das jederzeitige Abdrehen der Geldversorgung über Kartensperrungen. Die Abhängigkeit von Banken nimmt bedrohlich zu. Das gefährdet auch die Arbeit von Regierungskritikern, wie das Abschneiden von Wikileaks von Kreditkartenspenden vor einigen Jahren zeigt. Diese finanzielle Entmündigung gilt es zu stoppen!
Wir müssen stattdessen Wege finden, die besten Eigenschaften von Bargeld in unsere digitale Zukunft mitzunehmen. Auch im Netz haben wir ein Recht darauf, in Kryptowährungen bezahlen und spenden zu können, ohne dass unser Zahlungsverhalten anlasslos und personenbezogen aufgezeichnet wird. Dass die EU anonyme Geldbörsen für Kryptowährungen bei Anbietern (hosted wallets) ohne jeden Schwellenwert verbietet, während selbstbetriebene Wallets anonym bleiben, zeigt die Sinnlosigkeit dieser Repressivgesetze. Wenn die EU glaubt, virtuelle Währungen im Alleingang regulieren zu können, hat sie das weltweite Internet ohnehin nicht verstanden.
Anonyme Zahlungen in Bargeld zu begrenzen und anonyme Kryptowallets zu verbieten, hat bestenfalls minimal senkende Effekte auf die Kriminalität, nimmt aber unbescholtenen Bürgern die finanzielle Freiheit. Zum Einsammeln von Spenden sind Dissidenten wie der verstorbene Alexej Navalny und seine Ehefrau weltweit heute zunehmend auf anonyme Spenden in virtuellen Währungen angewiesen. Wo jede Zahlung erfasst und für immer gespeichert wird, drohen Hackerangriffe, unberechtigte Ermittlungen und eine abschreckende Staatsaufsicht über jeden Kauf und jede Spende.”
Die Spitzenkandidatin der Piratenpartei zur Europawahl Anja Hirschel unterstreicht: „Bargeldzahlungen werden immer weiter eingeschränkt und gleichzeitig anonyme Kryptowallets im Netz verboten. Dies führt zu einer immer detaillierteren Nachverfolgbarkeit unseres Konsums und Privatlebens. Wie viel ich wofür wann und wo ausgebe, lässt immer genauere Rückschlüsse auf mich als Person zu. In Zusammenhang mit den nationalen Diskussionen um Bezahlkarten für verschiedene Menschengruppen kann dies ein Baustein hin zu einer gesetzliche Grundlage zur späteren Profilbildung und Kontrolle selbst von legalen Ausgaben darstellen.“
Hintergrund zur Barzahlungsobergrenze:
2017 fragte die EU-Kommission schon einmal die Öffentlichkeit nach ihrer Meinung zur Begrenzung von Barzahlungen. Mehr als 90 % der antwortenden Bürger sprachen sich gegen einen solchen Schritt aus. Die Befragten hielten das anonyme Bezahlen mit Bargeld für eine “essentielle persönliche Freiheit” und waren der Meinung, dass “Beschränkungen für Bargeldzahlungen ineffektiv sind, um die möglichen Ziele (Bekämpfung von kriminellen Aktivitäten, Terrorismus, Steuerhinterziehung) zu erreichen”. Laut einer EZB-Umfrage verwenden bis zu 10% der Bürger Bargeld auch für Beträge über 10.000 € (z.B. beim Autokauf). Nach Berechnungen von Schattenwirtschaftsfachmann Friedrich Schneider von der Universität Linz hat das Verbot großer Barzahlungen „nur minimale senkende Effekte auf die Schwarzarbeit oder die Kriminalität“.
Hintergrund zu Kryptowährungen:
Im Gegensatz zu Bargeld, das völlig anonym ist, können Transaktionen mit Kryptowährungen gerade im Fall von Bitcoin über die “Blockchain” nachvollzogen werden. Wo virtuelle Vermögenswerte in der Vergangenheit für kriminelle Aktivitäten verwendet wurden, war eine strafrechtliche Verfolgung möglich, etwa indem ungewöhnliche Muster erkannt und Verdächtige identifiziert wurden. Einige Kriminelle haben sich im Laufe der Zeit selbst de-anonymisiert, und jeder Kriminelle wird irgendwann seine digitalen Gelder gegen echtes Geld eintauschen müssen. Virtuelle Guthaben sind von geringer Relevanz für das globale Finanzsystem. Es gibt keine belastbaren Nachweise dafür, dass virtuelle Währungen nennenswert zur Geldwäsche genutzt würden. EU-Vorschriften können durch Verwendung von Nicht-EU-Wallet-Diensten leicht umgangen werden. Virtuelle Vermögenswerte können auch direkt von einer Person zu einer anderen übertragen werden, ohne Zwischenhändler einzuschalten (unhosted wallets), so dass Identifizierungspflichten leerlaufen.
Die Abgeordneten des EU-Parlaments werden heute Mittag in Plenum die Verordnung zur Schaffung eines „Europäischen Gesundheitsdatenraums“ (EHDS) abgesegnen. Danach sollen zu allen Patienten Informationen über jede medizinische Behandlung einschließlich Impfstatus, Medikamente und Schwangerschaften, Labor- und Entlassberichte digital gespeichert und europaweit abrufbar gemacht werden – auch zu den in Deutschland bisher nicht von der elektronischen Patientenakte erfassten Privatpatienten. Zugang sollen europaweit eine Vielzahl von Stellen erhalten können, das von der Bundesregierung versprochene Widerspruchsrecht gegen Datenzugriffe aus dem Ausland ist nicht vorgesehen. Als Schattenberichterstatter und Mitverhandler der Verordnung für den Innenausschuss (LIBE) warnt der Europaabgeordnete der Piratenpartei Dr. Patrick Breyer vor einem Kontrollverlust der Patienten über sensibelste Gesundheitsdaten und einer Aufgabe des Arztgeheimnisses. Die Piraten werden deshalb heute gegen die Verordnung in ihrer geplanten Ausgestaltung stimmen.
“Wir Piraten unterstützen die Idee eines EU-Gesundheitsdatenraums, aber nicht um den Preis der Aufgabe des Selbstbestimmungsrechts der Patienten und des Arztgeheimnisses zugunsten von Gesundheitsdatenabgriffen durch Regierungen, Big Pharma und Big Tech. Es gibt nichts intimeres als Informationen über unsere körperliche und geistige Gesundheit, einschließlich unserer Suchtkrankheiten, psychischer Störungen, Schwangerschaftsabbrüche bis hin zu Geschlechtskrankheiten und Reproduktionsstörungen. Wenn wir uns nicht darauf verlassen können, dass diese Informationen bei unseren behandelnden Ärzten sicher sind, lassen wir uns vielleicht nicht mehr behandeln mit schlimmen Folgen für unsere Gesundheit bis hin zu Suiziden. Von einem EU-Gesundheitsdatenraum können grenzüberschreitende Behandlungen und Forschung profitieren, aber diese Vorteile hätte man auch auf der Grundlage einer Einwilligung der Patienten und mit vollständiger Datenanonymisierung haben können.
Die schlussendlich vereinbarte Ausgestaltung der EU-Verordnung widerspricht dem aus Umfragen bekannten Willen der Patienten. Patienten werden vor der identifizierbaren Weitergabe ihrer Gesundheitsdaten nicht gefragt und haben je nach EU-Land nicht einmal ein Widerspruchsrecht, Daten können außerhalb der EU gespeichert werden auf Systemen ohne unabhängige Sicherheitsprüfung – all das zeigt, dass die Verordnung im Sinne maximaler Verwertung unserer persönlicher Gesundheitsdaten und nicht im Interesse der Patienten ausgestaltet worden ist. Diese Verordnung verrät im Profitinteresse der Industrie die Interessen und den Willen der Patienten, um mit ihren identifizierbaren Daten Produkte entwickeln und KI-Algorithmen trainieren zu können. Einer solchen Entmündigung der Patienten erteilen wir Piraten eine klare Absage!”
Anja Hirschel, medizinische Informatikerin und Spitzenkandidatin der Piratenpartei für die Europawahl 2024, ergänzt: “Eine zentrale Datenspeicherung weckt Begehrlichkeiten in verschiedenste Richtungen. Wir sprechen dabei allerdings nicht nur von Hackerangriffen, sondern von der sogenannten Sekundärnutzung. Diese bezeichnet Zugriffe, die zu Forschungszwecke vollumfänglich gewährt werden sollen. Die Patientendaten sollen dann an Dritte weitergegeben werden. Aus Datenschutzsicht ist bereits das zentrale Ansammeln problematisch, bei Weitergabe wenigstens ein Opt-In Verfahren (aktive Einwilligung) richtig. Dies würde eine gewisse Entscheidungshoheit jedes Menschen über die persönlichen Daten ermöglichen. Wird allerdings nicht einmal ein Opt-Out Verfahren (aktiver Widerspruch) etabliert, so bedeutet dies letztlich die Abschaffung der Vertraulichkeit jeglicher medizinischer Information. Und das obwohl Ärzte in Deutschland gemäß § 203 StGB berufsständisch zurecht der Schweigepflicht unterliegen, wie u.a. auch Rechtsanwälte. Dieser Schutz unserer privatesten Informationen und das Recht auf vertrauliche Versorgung und Beratung stehen jetzt auf dem Spiel.”
Auf Initiative von Breyer und anderen Abgeordneten konnte immerhin ein europaweiter Zwang zur elektronischen Patientenakte verhindert werden. Laut Artikel 8h und Erwägungsgrund 13a der Verordnung ist das deutsche und österreichische Widerspruchsrecht gegen die Einrichtung einer elektronischen Patientenakte gerettet. Im Fall eines Widerspruchs werden die Pflichtinformationen nur beim behandelnden Arzt gespeichert. “Ich selbst werde dieser elektronischen Patientenakte widersprechen, um nicht die Kontrolle über meine Gesundsheitsdaten zu verlieren”, erklärt Breyer. “Wir wissen aber, dass die wenigsten Patienten, die Fremdzugriffe auf ihre Daten ablehnen, tatsächlich das komplizierte Widerspruchsverfahren durchlaufen.”
Auf Anfrage Breyers hat die EU-Kommission kurz vor der Abstimmung bestätigt, dass das von der Bundesregierung versprochene Widerspruchsrecht gegen ausländische Zugriffe auf Gesundheitsdaten in der endgültigen Fassung der Verordnung “nicht vorgesehen” ist. “Wer der elektronischen Patientenakte oder ihrer Auswertung nicht insgesamt widerspricht, ermöglicht damit zwangsweise auch einen grenzüberschreitenden Zugriff darauf durch ausländische Behandler, Forscher und Regierungen. Das von der Bundesregierung geplante Recht speziell grenzüberschreitenden Datenzugriffen widersprechen zu können, ist in der Verordnung nicht rechtssicher vorgesehen. Das widerspricht dem Interesse und Willen der Patienten, von denen laut Meinungsumfrage nur eine Minderheit einen grenzüberschreitenden europaweiten Zugriff auf ihre Patientenakte wünscht”, so Breyer.
Hintergrund: Laut Umfragen wollen mehr als 80% der EU-Bürger selbst über die Weitergabe ihrer Patientenakten entscheiden. Mehrheitlich wollen sie um Einwilligung gebeten werden. Dies sieht die Verordnung aber nicht vor. Ohne Einwilligung des Patienten erhalten künftig europaweit auch Gesundheitsministerien und Gesundheitsbehörden, Universitäten, zur Forschung, Produktentwicklung und zum Trainieren ‚künstlicher Intelligenz‘ auch Technologieunternehmen und Pharmaindustrie Zugang zu anonymisierten und personenbezogenen identifizierbaren (nur pseudonymisierten) Patientenakten – es sei denn der Patient widerspricht ausdrücklich. Kein Widerspruchsrecht gibt es in Deutschland gegen die Weitergabe medizinischer Registerdatensätze und von Abrechnungsdatensätzen. Im EU-Ausland müssen die nationalen Gesundheitssysteme auch weiterhin kein Widerspruchsrecht vorsehen.
Die Bundesregierung unterstützt die EU-Pläne. Kritik äußerten dagegen der europäische Verbraucherverband BEUC und das Datenschutznetzwerk EDRi.
🔗 Bob’s writing about Music and Musicians, but what he described has applicability to any new business launching itself onto the world.
US finally breaks ground on its first-ever high-speed rail.
… from LA to, no … not SFO .. Vegas baby. It’s gonna be so much quicker and Greener to go see a show at the Sphere.
IMHO .. this particular route would be waaaay down on the ‘which route first’ list, but I can’t think of any route that might be higher on the ‘which route is easiest’ list.
Yet another one to file in the bucket of things that don’t seem quite right.
The parent project is called CoreNet, described as “A library for training deep neural networks”. Part of the release is a new LLM called OpenELM, which includes completely open source training code and a large number of published training checkpoint.
I’m linking here to the best documentation I’ve found of that training data: it looks like the bulk of it comes from RefinedWeb, RedPajama, The Pile and Dolma.
This is genuinely wonderful:
“The FTC’s final rule to ban noncompetes will ensure Americans have the freedom to pursue a new job, start a new business, or bring a new idea to market.”
This was already true for California; banning it nationwide is an enormous step for innovation everywhere. Even new noncompetes for executives are banned, although ones that are currently in place can remain. #Business
[Link]
A bad survey won’t tell you it’s bad. It’s actually really hard to find out that a bad survey is bad — or to tell whether you have written a good or bad set of questions. Bad code will have bugs. A bad interface design will fail a usability test. It’s possible to tell whether you are having a bad user interview right away. Feedback from a bad survey can only come in the form of a second source of information contradicting your analysis of the survey results.
Most seductively, surveys yield responses that are easy to count and counting things feels so certain and objective and truthful.
Even if you are counting lies.
As my Category culling is drawing to a close continues with no end in sight, I just discovered a new project.
Not only do I have this space - but over on Blot I have a different - yet related space.
I used to have 🔗 https://words.philpin.com - for ‘words’ and 🔗 https://pictures.philpin.com which eventually became 🔗 https://images.philpin.com for, well … ‘images’.
And then I moved everything into 🔗 https://archive.philpin.com - it’s so easy in Blot - and everything is was great - were it not for rather a large number of links on Micro Blog that point to those three old blogs. (I know its large - because I just searched for those URLs in Mars Edit),
Three choices;
Do Nothing (which doesn’t really work for me)
Manually change them - one by one (which is something I don’t want to do)
Write a script to search for those three parts of URLs and replace with ‘https://archive.philpin.com’, at the same time as adding a category ‘My Archives’
Which is likely to happen shortly after we witness a small pig flying overhead.🎈113/366 Today I discovered and then learned about Scoped Tags in Drafts - only 10 versions later I think - but now I know and thank you @Agiletortoise
At the beginning of the year I had grand plans for this series. A daily long-form post about something that was rattling my brain that day. And then life. For a while, I was even just dropping markers - to revisit. I came to realise that part of the problem was the complexity of the structure for each post - so that went away. Simplicity really is rather nice. As I write on 240413, I am now going back and filling in the gaps. PLUS - unless something strikes me immediately, I will not classify until the end of the day and go back to move one of the posts of the day into the 366. Also - if you are wondering how I have update the words at the bottom of over 100 posts at a stroke, well - THANK YOU Andy Sylvester and his Glossary plugin.Here’s the latest installment in the series on working with LLMS: https://thenewstack.io/the-future-of-sql-conversational-hands-on-problem-solving/
I keep returning to the theme of choral explanations (#4 on my list of best practices), and it’s especially relevant in the SQL domain where there are just so many ways to write a query.
Exploring the range of possibilities used to be arduous, time-consuming and hard to justify. Now it’s becoming hard to justify not doing that; optimizations (sometimes major ones) can and do emerge.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
12 Let’s talk: conversational software development
13 Using LLMs to Improve SQL Queries
14 Puzzling over the Postgres Query Planner with LLMs
15 7 Guiding Principles for Working with LLMs
16 Learn by Doing: How LLMs Should Reshape Education
17 How to Learn Unfamiliar Software Tools with ChatGPT
18 Using AI to Improve Bad Business Writing
19 Code in Context: How AI Can Help Improve Our Documentation
A fire broke out backstage in a theatre. The clown came out to warn the public; they thought it was a joke and applauded. He repeated it; the acclaim was even greater. I think that’s just how the world will come to an end: to general applause from wits who believe it’s a joke.
💬 Søren Kierkegaard
High-level diagram of the six logical services making up the proposed future extension distribution architecture. The Root Registry sits at the center, providing APIs for the other services to consume for their own use cases. Trusted instances of those services submit additional data about extensions via the Interactions service to enhance and enrich the service to better inform and delight users.
Over on the Postgres Wiki I’ve published a new document for the PGXN v2 project: PGXN v2 Architecture. It has diagrams, such as the one above! From the introduction:
This document outlines the project to build extension distribution, discovery, and packaging tools and services to power the growth, accessability, and utility of the Postgres extension ecosystem. Taking the overall Postgres community as its audience, it defines the services to be provided and the architecture to run them, as well as the strategic vision to guide project planning and decision-making.
With the goal to think strategically and plan pragmatically, this document describes the former to enable the latter. As such, it is necessarily high-level; details, scoping, and planning will be surfaced in more project-focused documents.
Bear in mind that this document outlines an ambitious, long-term strategy. If you’re thinking that there’s too much here, that we’er over-thinking and over-designing the system, rest assured that project execution will be fundamentally incremental and pragmatic. This document is the guiding light for the project, and subject to change as development proceeds and new wrinkles arise.
For those of you interested in the future of Postgres extension distribution, please give it a read! I expect it to guide the planning and implementation of the the new services and tools in the coming year. Please do consider it a living document, however; it’s likely to need updates as new issues and patterns emerge. Log in and hit the “watch” tab to stay in the loop for those changes or the “discussion” tab to leave feedback.
I’ve also moved the previously-mentioned document Extension Ecosystem: Jobs and Tools to the wiki, and created a top-level PGXN v2 and PGXN category for all PGXN-related content. It also includes another new document, Service Disposition, which describes itself as:
A summary of the ambitiously-envisioned future PGXN services and architecture, followed by an examination of existing services and how they will gradually be refactored or replaced for the updated platform.
Check it out for how I expect existing services to evolve into or be replaced by the updated platform.
More about… Postgres PGXN ArchitectureI am killing alerts on my phone to achieve minimum interruptions and then adding some of them to my less used iPad mini, This means that my phone allows for focus and when I pick up the iPad I can quickly scan the notifications ..got it .. flush them.
Sounds like a grand plan, except that I have now found three iPad apps that do not have notification controls that their iPhone counterparts do.
Unless I am doing something wrong. As usual.
We [Bluesky] took a somewhat novel approach of giving every user their own SQLite database. By removing the Postgres dependency, we made it possible to run a ‘PDS in a box’ [Personal Data Server] without having to worry about managing a database. We didn’t have to worry about things like replicas or failover. For those thinking this is irresponsible: don’t worry, we are backing up all the data on our PDSs!
SQLite worked really well because the PDS – in its ideal form – is a single-tenant system. We owned up to that by having these single tenant SQLite databases.
microsoft/Phi-3-mini-4k-instruct-gguf
Microsoft’s Phi-3 LLM is out and it’s really impressive. This 4,000 token context GGUF model is just a 2.2GB (for the Q4 version) and ran on my Mac using the llamafile option described in the README. I could then run prompts through it using the llm-llamafile plugin.The vibes are good! Initial test prompts I’ve tried feel similar to much larger 7B models, despite using just a few GBs of RAM. Tokens are returned fast too—it feels like the fastest model I’ve tried yet.
And it’s MIT licensed.
Via @simonw
Llama 3 landed on Thursday. I ended up updating a whole bunch of different plugins to work with it, described in Options for accessing Llama 3 from the terminal using LLM.
I also wrote up the talk I gave at Stanford a few weeks ago: AI for Data Journalism: demonstrating what we can do with this stuff right now.
That talk had 12 different live demos in it, and a bunch of those were software that I hadn't released yet when I gave the talk - so I spent quite a bit of time cleaning those up for release. The most notable of those is datasette-query-assistant, a plugin built on top of Claude 3 that takes a question in English and converts that into a SQL query. Here's the section of that video with the demo.
I've also spun up two new projects which are still very much in the draft stage.
llm-evalsOny of my biggest frustrations in working with LLMs is that I still don't have a great way to evaluate improvements to my prompts. Did capitalizing OUTPUT IN JSON really make a difference? I don't have a great mechanism for figuring that out.
datasette-query-assistant
really needs this: Which models are best at generating SQLite SQL? What prompts make it most likely I'll get a SQL query that executes successfully against the schema?
llm-evals-plugin (llmevals
was taken on PyPI already) is a very early prototype of an LLM plugin that I hope to use to address this problem.
The idea is to define "evals" as YAML files, which might look something like this (format still very much in flux):
name: Simple translate system: | Return just a single word in the specified language prompt: | Apple in Spanish checks: - iexact: manzana - notcontains: appleThen, to run the eval against multiple models:
llm install llm-evals-plugin llm evals simple-translate.yml -m gpt-4-turbo -m gpt-3.5-turboWhich currently outputs this:
('gpt-4-turbo-preview', [True, True])
('gpt-3.5-turbo', [True, True])
Those checks:
are provided by a plugin hook, with the aim of having plugins that add new checks like sqlite_execute: [["1", "Apple"]]
that run SQL queries returned by the model and assert against the results - or even checks like js: response_text == 'manzana'
that evaluate using a programming language (in that case using quickjs to run code in a sandbox).
This is still a rough sketch of how the tool will work. The big missing feature at the moment is parameterization: I want to be able to try out different prompt/system prompt combinations and run a whole bunch of additional examples that are defined in a CSV or JSON or YAML file.
I also want to record the results of those runs to a SQLite database, and also make it easy to dump those results out in a format that's suitable for storing in a GitHub repository in order to track differences to the results over time.
This is a very early idea. I may find a good existing solution and use that instead, but for the moment I'm enjoying using running code as a way to explore a new problem space.
datasette-secretsdatasette-secrets is another draft project, this time a Datasette plugin.
I'm increasingly finding a need for Datasette plugins to access secrets - things like API keys. datasette-extract and datasette-enrichments-gpt both need an OpenAI API key, datasette-enrichments-opencage needs OpenCage Geocoder and datasette-query-assistant needs a key for Anthropic's Claude.
Currently those keys are set using environment variables, but for both Datasette Cloud and Datasette Desktop I'd like users to be able to bring their own keys, without messing around with their environment.
datasette-secrets
adds a UI for entering registered secrets, available to administrator level users with the manage-secrets
permission. Those secrets are stored encrypted in the SQLite database, using symmetric encryption powered by the Python cryptography library.
The goal of the encryption is to ensure that if someone somehow obtains the SQLite database itself they won't be able to access the secrets contained within, unless they also have access to the encryption key which is stored separately.
The next step with datasette-secrets
is to ship some other plugins that use it. Once it's proved itself there (and in an alpha release to Datasette Cloud) I'll remove the alpha designation and start recommending it for use in other plugins.
近年、多要素認証(MFA)の普及が進む中、ユーザーの利便性とセキュリティのバランスを取ることが課題となっています。MFAとは、パスワードに加え、生体認証やワンタイムパスワードなど複数の認証方式を組み合わせることで、アカウントへの不正アクセスを防ぐセキュリティ手法です。しかし、MFAの導入はユーザーにとって面倒な手順が増えることを意味します。この課題を解決するために登場したのが、Syncable Authenticator(同期可能認証器)、別名Passkey(パスキー)と呼ばれる新しい認証技術です。
同期可能認証器は、認証に用いる秘密鍵を複数のデバイス間で同期できるようにすることで、ユーザーはどのデバイスでも同じ認証情報を使えるようになります。これにより利便性が大幅に向上します。一方で、秘密鍵を複数の端末で共有することはセキュリティ上のリスクを伴います。そこで、米国国立標準技術研究所(NIST)は、シンカブル認証器の安全な利用に関するガイドライン(SP 800-63Bの補遺)を公開しました。
NIST: Incorporating Syncable Authenticators Into NIST SP 800-63Bこの文書は、NIST Special Publication 800-63Bへの補足であり、認証キーを複製しデバイス間で同期できる同期可能認証器(パスキーとしても知られている)の使用に関するガイダンスを提供するものです。主なポイントは以下の通りです。
適切に構成された同期可能認証器は、中間者攻撃、検証者のなりすまし、リプレイ攻撃などの脅威を軽減し、認証の意図を提供することで、認証保証レベル2(AAL2)を達成できます。AAL2は、フィッシング耐性など高度なセキュリティを求める一方で、ある程度の使いやすさも確保するレベルです。 本文書では、キーの生成、保存、アクセス制御に関する一定の要件を満たすことを条件に、同期可能認証器の認証キーの複製を許可するようSP 800-63Bを更新しています。これによって、政府機関でもパスキーを使うことができるようになります。 WebAuthn仕様のフラグを使用して、オーセンティケータがAAL2の要件を満たしているかどうかを判断するなど、実装上の考慮事項について説明しています。エンタープライズでのユースケースでは、Attestationを利用してオーセンティケータの機能を検証することができます。 同期可能認証器の潜在的な脅威と課題を概説し、不正なキーの使用、同期ファブリックの侵害、失効の難しさなどを示し、それらを軽減するための方法を提案しています。 本文書は、一部の実装におけるユーザー間のキー共有のリスクを認識し、エンタープライズおよび公開向けのユースケースについてガイダンスを提供しています。全体として、この補足資料は、適切に導入されれば、便利でフィッシング耐性のある認証を提供できる同期可能認証器の統合について、機関がリスクに基づいた informed な意思決定を行うことを目的としています。
本補遺は、AAL2への同期可能認証器の適合性を検証する上で重要な基準を提供しています。また、鍵の不正使用やクラウドストレージの侵害といった新たな脅威についても言及し、対策を提言しています。同期可能認証器の潜在的なリスクを認識しつつ、適切に導入することで、利便性を損なわずにセキュリティを向上できることを示しています。
本補遺は、セキュリティ担当者だけでなく、同期可能認証器の導入を検討する全ての関係者にとって有益な情報を提供しています。NISTによる公的なガイドラインの存在は、同期可能認証器の安全性に対する信頼を高め、普及を後押しするでしょう。ユーザー数の増加は、さらなる技術革新を促します。本補遺は、セキュリティと利便性を両立する新認証技術の健全な発展に寄与する重要な一里塚になると考えられます。
とりあえず、アイデンティティに関心のある人は、本書『メタバース進化論』を読んだほうが良い。メタバース・バ美肉として現実の物理的制限から存在を解き放ち、抽象空間で取り扱うことができるようになることによって、アイデンティティとプライバシー管理の課題や要件がこんなにも明確になるのか!曰く
そもそも「アイデンティティ(自己同一性)とは、私たちが私たち自身をどのようなものと捉えるかという「認識」であり、他者や社会からそれが認められているという「感覚」のことです。 物理現実では、基本的に生まれたままの名前・姿・声を受け入れるしかありませんでした。つまり、アイデンティティとは「与えられる」ものでした。 基本的には与えられた固定のものを「受け入れる」しかなかった物理現実時代のそれとは違い、メタバース時代のアイデンティティは自由に「デザインする」ものになり、「なりたい自分」としての人生を送ることが可能になる 人間を分割可能な「分人(Dividual)」として捉える 複数のアイデンティティを「切り替える」ことで人生を自由にデザインできる プラトンの「イデア論」〜見ている現実世界は、実体ではなく、イデアの影にすぎないのだ アイデンティティを自在にデザインして「なりたい自分」になれるメタバース(出所)『メタバース進化論』
わたしの本1やブログ、講演などに接しているかたは、「はいはいアレね」と思うだろう。次の図は、2011年6月に書いた「非技術者のためのデジタル・アイデンティティ入門」の頃から使いまわしている図だが、ほとんど同様のことを言っている。分人 v.s. Identity (Partial Identity) など使っている言葉は違えど殆どマッピングできる。
ただ、多分、本書のほうが100倍わかりやすい。これは、この図では自己像と言って抽象的に扱っているものにアバターという実体を与えて手触り感を持たせているのと同時に、何でもかんでも「属性」という分類でくくってしまわずに、「言霊世界」「視覚世界」「音響世界」のようにカテゴライズして説明しているからだろう。もちろん単純な筆力の違いもあるが。
【図表1】自己像(アイデンティティ)と幸福追求
とりわけ重要なのが、魂の自由性とか、アイデンティティは他者から与えられるものではなく自分で確立するものだというところだ。このあたり、「アイデンティティの専門家」でも忘れている人達が多い。神だとか政府だとかに与えてもらいそれを受容するものだという中世的な考え方の人たちが多いのだ。
中世的アイデンティティ v.s. 近代的アイデンティティ近代的な個人観と中世的な個人観の主な違いは以下のようにまとめらるだろう。
中世的個人観
個人は共同体の一部として存在し、共同体からの役割や地位によって定義されていた。2 キリスト教の影響で、個人は神との関係性において「世俗外個人」として捉えられていた。3 4 個人の内面性よりも、共同体への所属と役割が重視されていた。5近代的個人観
個人は自律的な存在として捉えられ、個人の内面性や自我意識が重視されるようになった。67 啓蒙主義の影響で、個人は中世的な共同体からの拘束から解放され、「内面的孤独」を経験するようになった。8 個人の権利や自由が強調され、個人主義的価値観が台頭した。910 プライバシーの概念が生まれ、私的領域が神聖視されるようになった。11つまり、中世では個人は共同体の一部として捉えられていたのに対し、近代では個人の自律性と内面性が強調され、個人主義的価値観が確立されたということができる。魂の自由性と言っても良い。でも残念ながら、この個人主義的価値観・近代的個人観に行き着いていない人が結構多い。だから、アバターは現実世界の自分に似ていなければならないだとか、アイデンティティウォレットには政府発行の個人属性証明(PID)を入れて、それを中心に回していくとかの考えになってくる。
これは別にウォレットに限ったことではなくて、Web 2.0的なSNSにおける本人確認でも似たようなことが起きてくる。先ほど経験したことだと、LinkedInで本人確認済みにするには、氏名はパスポート表記と同一でなければならないという。パスポート表記などというのは、国境を超えるときの識別子として政府に登録されたものであって、自分を表出するものではない。わたしは英語では Nat Sakimura として活動しているが、それが「本人確認」をすると、Natsuhiko Sakimuraであることを強制される。なんだそれは。そんなことしたら過去の業績とのリンクができなくなるではないか。いやそんな些細なことではない。名乗りを奪うということは、人格を否定することに等しい。ちょうど千と千尋の神隠しの湯婆婆が千尋の名前を奪うように。
まさに、本書がいうように、名前は「言霊世界のアイデンティティ」なのだ。この「名乗りの自由性」はとても大切だ。なぜならば、本書が言うように『周りの方々からこの「言霊」で毎日繰り返し呼びかけられ続けることで、わたしは◯◯としての自己認識を獲得することができる』からだ。
また、言うまでもなく「アバター:視覚世界のアイデンティティ」(本書)の自由性も重要だ。『自分自身の「アイデンティティ表現」としてみた時は現実のような「人間」のかたちに縛られたくない』(本書)からだ。それともあれだろうか。四肢が不自由だったら、メタバースでも四肢が不自由でなければいけないとでも言うのか。
その自由性の象徴がバ美肉である。日本では、人形浄瑠璃や歌舞伎の女形などの文化的背景がありだいぶ受容が進んできたが、西洋ではヘタをしたら56されかねない。バ美肉を受容できるかどうかは、潜在的な差別意識のあるなしのリトマス試験紙になる。
本書ではMetaが規制を入れてくる可能性に触れているが、AppleもVision Pro でやってきても驚かない。場合によっては、VRのアバタを簡単に作らさせるためにUXとしてそういうことにするといってくるかもしれない。だがこうした動きには徹底抗戦しなければなるまい。日本では、自己イメージコントロール権説や、「身だしなみなどに関する自己決定権」から、「なりたい自分になれる権利」は認められ、間接適用説からmetaなど事業者もこの権利の侵害は許されないはずだ12。
こうしたアイデンティティに関する話は第4章にまとめられているので、時間がなかったらまずそこだけでも読んでほしいところだ。
プライバシーに関しても参考になるまた、アイデンティティと違って一つの章になっているわけではないが、プライバシーに関しても参考になる記述がいろいろとある。曰く、
同じく日本の伝統芸能「人形浄瑠璃」でも、優美に踊る美しい女性の人形の背後には黒子のおじさんが見えてしまっています。しかし、それを指摘するのは野暮これは、「データが見えてしまっても、意図された目的以外では使ってはいけない」というデータ保護の基本につながる。わたしが昔から「大人のプライバシー」と呼んでいるものだ。もちろん「なりたい自分になれる」というのが、「自己イメージコントロール権」13と表裏一体であるのは言うまでもなく。
ISOでは現在、メタバースのプライバシーについての検討が始まっているが、関係者にぜひ本書を読ませたいところだ。英語版がほしい。
もちろんメタバースの解説もさて、自分がアイデンティティ&プライバシー業界の人間だから、いきなりそのあたりの各論に入ってしまったが、そもそも本書はメタバースについての本だ。「メタバースの定義:実現に必要な七要件」であるとか、4大ソーシャルVRの紹介であるとか、それらを支える技術であるとか、メタバースに住む住民たちの特性、なぜ人々はバ美肉になるのか、などなど、データの裏付けをとりながらきっちりと解説している。しかも、マーカーで線を引きたくなるようなキャッチーなフレーズが満載で。たとえばこんな感じ:
声:音響世界のアイデンティティ 自分の精神に作用するプロテウス効果 バ美肉は枯山水。見立ての文化の延長線。 (現在は)「永遠に新しいことを学び続けていく」ことが当然(の社会)。すべての人が永遠に「未熟」であることを受け入れざるを得ない社会において、「大人としての自尊心」に変わる新たな価値観こそが、お互いの「未熟さ」を愛おしいと思う価値観「かわいい」(出所)『メタバース進化論』
わたしは、Kindleで大量に線を引きながら読んでしまった。上記はそのほんの一部だ。ぜひ本書を買って読んいただきたい。
動画による解説も一緒にみるとなお良しなお、本書は文章による紹介としては大変優れているが、やはりメタバースのイメージを得るには映像のほうが適している。そういう意味で、筆者である「バーチャル美少女ねむ」さんが、国連のIGF 2023で行ったプレゼン「バーチャル美少女だけど国連登壇してみた」も並行して見ることをおすすめする。非常にわかりやすいプレゼンだ。英語ですが…。
これは、国連が主催するインターネット・ガバナンス・フォーラム(IGF14 )2023 京都のDay 0
「Event No.134 Talk with Metaverse residents – a new identity and diversity | Internet Governance Forum」(2023年10月8日 18:00〜19:00)の模様を録画したものだ。わたしもこの日だけは、自分のセッション15があったのでIGFの会場にいたのに、生参加できなくて残念だった。多分この時間はOECDの人とTrusted Data Intermeidaries (TDI: 信頼できるデータ仲介者) について話していたと思う。
というわけで本書、本当におすすめだ。サンフランシスコからの機内で読んでいて、第4章を読んでいて涙が自然に流れ出てきたくらい。ぜひ買って読みましょう!
【変更履歴】
2024-04-23 初版 2024-04-24 中世的アイデンティティ v.s. 近代的アイデンティティを追加、敬体を常態に変更。🖇️ Remember Micro Monday Follow Day? - I just fixed the click through - because after all this shuffling a few things got broken. There are some crackers in there … 5 Years Ago Today Apparently!
I seem to have an about page that is rather old and I want to kill it anyway - but according to my page list - it doesn’t exist.
F U N K Y
The Instruction Hierarchy: Training LLMs to Prioritize Privileged Instructions
By far the most detailed paper on prompt injection I’ve seen yet from OpenAI, published a few days ago and with six credited authors: Eric Wallace, Kai Xiao, Reimar Leike, Lilian Weng, Johannes Heidecke and Alex Beutel.The paper notes that prompt injection mitigations which completely refuse any form of instruction in an untrusted prompt may not actually be ideal: some forms of instruction are harmless, and refusing them may provide a worse experience.
Instead, it proposes a hierarchy—where models are trained to consider if instructions from different levels conflict with or support the goals of the higher-level instructions—if they are aligned or misaligned with them.
The authors tested this idea by fine-tuning a model on top of GPT 3.5, and claim that it shows greatly improved performance against numerous prompt injection benchmarks.
As always with prompt injection, my key concern is that I don’t think “improved” is good enough here. If you are facing an adversarial attacker reducing the chance that they might find an exploit just means they’ll try harder until they find an attack that works.
The paper concludes with this note: “Finally, our current models are likely still vulnerable to powerful adversarial attacks. In the future, we will conduct more explicit adversarial training, and study more generally whether LLMs can be made sufficiently robust to enable high-stakes agentic applications.”
Via @_akhaliq
We introduce phi-3-mini, a 3.8 billion parameter language model trained on 3.3 trillion tokens, whose overall performance, as measured by both academic benchmarks and internal testing, rivals that of models such as Mixtral 8x7B and GPT-3.5 (e.g., phi-3-mini achieves 69% on MMLU and 8.38 on MT-bench), despite being small enough to be deployed on a phone.
I am guessing that Spark New Zealand is having an issue - got an email this morning demanding an outrageous amount of money that apparently I owe them. Not phishing as far as I can so tried to log on to their site - which I did - but no service available, no billing, no way to message them, no list of products - but I was recognized. Tried their app - same deal.
UH OH.
🔗 Received from my friend Graham, article from The New Yorker .. as I commented tomorrow him;
Personally speaking - my life is too short
🎈112/366 I am at a good point now. Time to stop for a while and let it digest .. but still ‘goodenough’ to have a link on the site that takes you down the rabbit hole for any passing observer interested enough to click.
At the beginning of the year I had grand plans for this series. A daily long-form post about something that was rattling my brain that day. And then life. For a while, I was even just dropping markers - to revisit. I came to realise that part of the problem was the complexity of the structure for each post - so that went away. Simplicity really is rather nice. As I write on 240413, I am now going back and filling in the gaps. PLUS - unless something strikes me immediately, I will not classify until the end of the day and go back to move one of the posts of the day into the 366. Also - if you are wondering how I have update the words at the bottom of over 100 posts at a stroke, well - THANK YOU Andy Sylvester and his Glossary plugin."This has long been the dream, and it seems like the platforms betting on it in various ways — Mastodon, Threads, Bluesky, Flipboard, and others — are where all the energy is, while attempts to rebuild closed systems keep hitting the rocks."
Just an enormous deal: for the web, for independent media, for social media. #Technology
[Link]
timpaul/form-extractor-prototype
Tim Paul, Head of Interaction Design at the UK’s Government Digital Service, published this brilliant prototype built on top of Claude 3 Opus.The video shows what it can do. Give it an image of a form and it will extract the form fields and use them to create a GDS-style multi-page interactive form, using their GOV.UK Forms design system and govuk-frontend npm package.
It works for both hand-drawn napkin illustrations and images of existing paper forms.
The bulk of the prompting logic is the schema definition in data/extract-form-questions.json
I’m always excited to see applications built on LLMs that go beyond the chatbot UI. This is a great example of exactly that.
Via @timpaul
Publishing turns out to be similar to VC investing: a tiny percentage of books are hits that cover the costs for the vast majority that didn't sell well. The DOJ found that, of 58,000 books published in a year, "90 percent of them sold fewer than 2,000 copies and 50 percent sold less than a dozen copies."
UPDATE: This story is inaccurate: those statistics were grossly misinterpreted during the trial. See this post for updated information.
Here's an even better debunking: Yes, People Do Buy Books (subtitle: "Despite viral claims, Americans buy over a billion books a year").
Via Hacker News
Hacking Postgres S2E3: David Wheeler, Principal Architect, Tembo
Last week I appeared on s02e03 of the Hacking Postgres podcast.
The experience I had after my independent Consulting gig for 10 years working in companies was, like, bringing up other people and being supportive of other people and hearing from a diversity of voices and perspectives makes everything better.
That’s part of why I want to get so much input on and feedback on the stuff that I’m hoping do with PGXN v2 — or whatever we ultimately call it. But people matter, more than the technology, more than any of the rest of it.
I quite enjoyed this wide-ranging discussion. We covered my history with the Postgres community, a bunch of the projects I’ve worked on over the years, plans and hopes for the PGXN v2 project, perspectives on people and technology, and exciting new and anticipated features of Postgres. Find it wherever fine podcasts are streamed, including:
YouTube Apple Podcasts Overcast Twitter More about… Postgres Podcast Hacking Postgres Sqitch pgTAP PGXN32 Europäische Polizeichefs – offenbar einschließlich des BKA-Präsidenten – fordern in einer gemeinsamen Erklärung einen Stopp der Einführung sicherer Ende-zu-Ende-Verschlüsselung für Direktnachrichten über Facebook und Instagram. Dieser Schritt mache Telekommunikationsüberwachung und die freiwillige Chatkontrolle unmöglich, heißt es zur Begründung. Der Europaabgeordnete der Piratenpartei Dr. Patrick Breyer, der Meta wegen der freiwilligen Chatkontrolle verklagt hat, ordnet ein:
„Mit ihrem Angriff auf sichere Verschlüsselung fordern die die Überwachungsbehörden gerade keinen ‚gesetzmäßigen Zugang‘, sondern eine grundrechtswidrige und damit gesetzwidrige Gefährdung unser aller Sicherheit. Der Europäische Menschenrechtsgerichtshof hat erst vor wenigen Wochen geurteilt, dass eine generelle Schwächung von Verschlüsselung und Überwachbarkeit gegen das Menschenrecht auf Schutz der Privatsphäre verstößt, zumal es zielgerichtete Überwachungsmöglichkeiten gibt.“
Die Informatikerin und Spitzenkandidatin der Piratenpartei zur Europawahl Anja Hirschel kommentiert: „Der Ruf nach immer weiter reichenden Zugriffsmöglichkeiten ist nicht neu. Und jeder Schritt führt uns lediglich näher zur nächsten Forderung. Es ist nie genug: Der Wunsch nach völliger und lückenloser Überwachungsmöglichkeit eines jeden Menschen ist längst zum Selbstzweck geworden, lediglich die Begründungen variieren.“
Breyer weiter: „Dass Polizeiarbeit ohne Chatkontrolle nicht möglich sei, ist eine dreiste Lüge. Die allermeisten, gerade europäischen Kommunikationsdienste respektieren doch seit jeher das Telekommunikationsgeheimnis. Dass unsere Privatnachrichten vor verdachtslosen und unzuverlässigen Schnüffelalgorithmen sicher sind, schützt uns – und übrigens auch die Fotos unserer Kinder. Dass die freiwillige Chatkontrolle signifikant zur Rettung von Kindern beitrage, ist eine Mär, die nie belegt worden ist. Die britische Polizei zitiert einen Fall sexueller Erpessung, ohne aber mitzuteilen, ob der Hinweis infolge einer Nutzermeldung erfolgt ist oder aufgrund der unzuverlässigen Chatkontrolle. Sexuelle Erpressung wird normalerweise dadurch bekannt, dass sie von Nutzern gemeldet wird – einer Zerstörung des digitalen Briefgeheimnisses braucht es dazu nicht.
Gerade erst hat die neue Kriminalstatistik eine überdurchschnittlich hohe Aufklärungsquote bei Internetdelikten gezeigt – doch unsere Überwachungsbehörden scheint jeder geschützte und private Raum in Panik zu versetzen. Haben sie vergessen, dass unsere Wohnungen und Briefe schon immer weitgehend vor Spionage sicher waren? Sollen demnächst auch unsere Wohnungen und Briefe ‚überwachbar by Design‘ werden, vielleicht mit Nachschlüssel für die Polizei? Noch nie wusste die Polizei so viel über uns wie heute im digitalen Zeitalter. Totales Wissen nach dem Vorbild der DDR-Staatssicherheit darf es in einer Demokratie, in der die Bürger den Staat kontrollieren, nicht geben!“
Hintergrund: Eine EU-Expertengruppe („High-Level Group (HLG) on access to data for effective law enforcement“) erarbeitet gerade Vorschläge gegen sichere Verschlüsselung und zur Wiedereinführung der Vorratsdatenspeicherung.
Summary: The abundance of X.509 certificate authorities who already perform identity proofing for businesses provides a rich resouce that can be leveraged to boot the verifiable data ecosystem. Here’s how it could work.
When you used a verifiable credential to prove something about yourself, the verifier can know cryptographically: (1) the identifiers for the issuer, (2) the credential hasn't been tampered with, (3) the credential was issued to you, and (4) the credential hasn't been revoked. These four checks are important because their establish the fidelity of the data being transferred. They don't, however, tell them whether they can trust the issuer. For that, they need to take the issuer's decentralized identifier (DID) that they got from credential presentation and determine who it belongs to.
At Internet Identity Workshop last week, Drummond Reed gave a session on how X.509 certificates could help with this. The first step, like always, is to resolve the DID and retrieve the DIDDoc that associates keys and endpoints with the DID. The endpoint can be an HTTP server and, of course, should have an X.509 certificate providing TLS security. That certificate, at the very least, has a a domain name to bind that to the certificate's public key. It can, if you pay for the feature, also include information about the entity that applied for the certificate. The certificate authority proofs that information and is vouching for it when they sign the certificate.
The key to making the X.509 certificate useful for checking the provenance of a DID lies in one key change. X.509 certificates can contain and extended field called a Subject Alternative Name. This following figure shows how it can help.
Using X.509 Certificates to establish the owner of a DIDThe issuer (Attestor) creates the DID they will use to issue the certificate along with its associated DIDDoc, including an HTTP endpoint for DID verification.
Attestor applies for a X.509 certificate for that endpoint, including in the application the DID they created in (1).
The certificate authority does it's usual proofing of the application and issues a certificate that includes the DID in the Subject Alternative Name field.
The issuer creates a credential definition in the usual way that includes their DID and writes it to whatever Verifiable Data Registry their DID method dictates.
Attestor issues a credential to a holder (Alice) using that credential definition.
At some later time, Alice presents the credential to the verifier (Certiphi).
Certiphi resolves the DID to get the DIDDoc and retrieves the verfication endpoint from the DIDDoc
Certiphi retrieves the certificate for that endpoint1.
Certiphi verifies the certificate by checking it's signature and ensures that the DID in the DIDDoc for the credential matches the one in certificate.2
The issuer's DID has now been tied in a verifiable way to whatever information is in the certificate. Provided the certificate includes information about the entity beyond the domain name, the verifier can use that information to determine whether or not the credential is authentic (i.e., issued by who the credential definition purports issued it). That might be all the evidence they need to determine whether to trust the entity. Certificate authorities could also issue verifiable credentials to the customer attesting the same verified claims—after all, it's one more product they can offer.
The benefit of doing issuer validation using X.509 certificates is that there are already many trusted X.509 certificate authorities in business who already do proofing of attributes about businesses. That's a huge chunk of the verifiable data ecosystem that doesn't need to be built because it can be leveraged. To make this work, digital certificate authorities would need to start offering to validate DIDs and include them in a certificate as a Subject Alternative Name. I don't discount that this will take some bureaucratic maneuvering. Certificate authorities will need to see a business opportunity. I'd love to see Digitcert or someone do a pilot on this.
NotesNote that this step might be combined with the previous step if the Verifiable Data Registry is the same server as the endpoint, but that's not necessarily going to be the case for a number of reasons.
Note that this does not create a call back wherein Attestor can determine which credential was used, preserving the privacy of the presentation. Attestor does know one of its credentials has been presented to Certiphi.
"A recent MIT study indicated that “the cloud” now has a larger carbon footprint than the airline industry, and that “a single data center can consume the equivalent electricity of 50,000 homes.” The study also cites the enormous cooling costs, the huge volumes of water required, and the noise pollution affecting local communities."
A useful step back to look at the internet's relationship with climate change: how it helps as a conduit for scientific research and testing, as a contributor through emissions and vast electricity usage, and as a recipient through rising sea levels and other adverse climate effects.
I've personally had a very hard time finding hosting providers who are genuinely green - using direct renewable energy rather than offsets, and taking steps to mitigate their water use. And that's just the tip of the iceberg. The internet feels green, because we don't see everything that is involved in keeping us online, but it very much isn't. There's a lot of work to do in order to reduce our harmful impact on the planet. #Climate
[Link]
Llama 3 was released on Thursday. Early indications are that it's now the best available openly licensed model - Llama 3 70b Instruct has taken joint 5th place on the LMSYS arena leaderboard, behind only Claude 3 Opus and some GPT-4s and sharing 5th place with Gemini Pro and Claude 3 Sonnet. But unlike those other models Llama 3 70b is weights available and can even be run on a (high end) laptop!
My LLM command-line tool and Python library provides access to dozens of models via plugins. Here are several ways you can use it to access Llama 3, both hosted versions and running locally on your own hardware.
Llama-3-8B-Instruct locally with llm-gpt4all Fast API access via Groq Local Llama 3 70b Instruct with llamafile Paid access via other API providers Llama-3-8B-Instruct locally with llm-gpt4allIf you want to run Llama 3 locally, the easiest way to do that with LLM is using the llm-gpt4all plugin. This plugin builds on the excellent gpt4all project by Nomic AI, providing a quantized (q4) Llama 3 8B instruct model that needs 8GB of RAM and requires a 4.34GB download.
If you don't have LLM installed yet, install it like this:
brew install llmOr like this:
pipx install llmInstall the plugin:
llm install llm-gpt4allThe first time you run a prompt it will download the model:
llm -m Meta-Llama-3-8B-Instruct "Three great names for a pet emu"What an interesting question! Here are three name suggestions for your pet emu:
Emmet: A playful take on the word "emu" that suits a friendly and curious bird. Gizmo: Emus are known for their quirky behavior, so Gizmo is a fitting name to capture their unique personality. Aussie: As an Australian native species, naming your emu Aussie would be a nod to its heritage.Remember to choose a name that suits your pet's temperament and characteristics!
Each time you run a command like this the model will be loaded into memory from scratch. To keep it resident in memory, use the llm chat
command:
Here's an example session:
Chatting with Meta-Llama-3-8B-Instruct
Type 'exit' or 'quit' to exit
Type '!multi' to enter multiple lines, then '!end' to finish
> javascript function to reverse a string
%
Here is the JavaScript code that reverses a given string:
```
function reverseString(str) {
return str.split("").reverse().join("");
}
```
You can use this function by passing your desired input string as an argument, like so:
```javascript
let reversedStr = reverseString("Hello World");
console.log(reversedStr); // Output: "dlroW olleH"
```
In the code above:
- `str.split("")` splits the original string into individual characters.
- `.reverse()` reverses the order of those characters.
- `.join("")` combines them back together as a single string.
This function works for any input string, not just "Hello World". Try it out with different strings to see how it handles punctuation and spaces!
> capitalize it too
%
Here's an updated JavaScript function that not only reverses the input string but also capitalizes each character:
```javascript
function reverseAndCapitalize(str) {
return str.split("").reverse().map(function(char) {
if (char.match(/[a-z]/i)) {
return char.toUpperCase();
} else {
return char;
}
}).join("");
}
```
(I'm not sure I'd write reverseAndCapitalize()
quite like that, but still a fun example!)
Consult the LLM documentation for more details on how to use the command-line tool.
Fast API access via GroqGroq serve openly licensed LLMs at ludicrous speeds using their own custom LPU (Language Processing Unit) Inference Engine. They currently offer a free preview of their API: you can sign up and obtain an API key to start using it.
You can run prompts against Groq using their OpenAI compatible API endpoint.
Edit the file ~/Library/Application Support/io.datasette.llm/extra-openai-models.yaml
- creating it if it doesn't exist - and add the following lines to it:
This tells LLM about those models, and makes them accessible via those configured model_id
values.
Run this command to confirm that the models were registered correctly:
llm models | grep groqYou should see this:
OpenAI Chat: groq-openai-llama3
OpenAI Chat: groq-openai-llama3-8b
Set your Groq API key like this:
llm keys set groq # <Paste your API key here>Now you should be able to run prompts through the models like this:
llm -m groq-openai-llama3 "A righteous sonnet about a brave owl"Groq is fast.
There's also a llm-groq plugin but it hasn't shipped support for the new models just yet - though there's a PR for that by Lex Herbert here and you can install the plugin directly from that PR like this:
llm install https://github.com/lexh/llm-groq/archive/ba9d7de74b3057b074a85fe99fe873b75519bd78.zip llm keys set groq # paste API key here llm -m groq-llama3-70b 'say hi in spanish five ways' Local Llama 3 70b Instruct with llamafileThe Llama 3 8b model is easy to run on a laptop, but it's pretty limited in capability. The 70b model is the one that's starting to get competitive with GPT-4. Can we run that on a laptop?
I managed to run the 70b model on my 64GB MacBook Pro M2 using llamafile (previously on this blog) - after quitting most other applications to make sure the 37GB of RAM it needed was available.
I used the Meta-Llama-3-70B-Instruct.Q4_0.llamafile
Q4 version from jartine/Meta-Llama-3-70B-Instruct-llamafile - a 37GB download. I have a dedicated external hard disk (a Samsung T7 Shield) for this kind of thing.
Here's how I got it working:
curl -L -o Meta-Llama-3-70B-Instruct.Q4_0.llamafile 'https://huggingface.co/jartine/Meta-Llama-3-70B-Instruct-llamafile/resolve/main/Meta-Llama-3-70B-Instruct.Q4_0.llamafile?download=true' # That downloads 37GB - now make it executable chmod 755 Meta-Llama-3-70B-Instruct.Q4_0.llamafile # And start it running: ./Meta-Llama-3-70B-Instruct.Q4_0.llamafileA llamafile
is an executable that runs on virtually any platform - see my previous notes on Cosmopolitan and Actually Portable Executable for more on how that works.
This will take quite a while to start, because it needs to load that full 37GB of binary content into memory. Once it's finished loading a local web server becomes available at http://127.0.0.1:8080/ - this serves a web UI you can use to interact with the model, and also serves another OpenAI-compatible API endpoint.
The easiest way to access this from LLM is to install the llm-llamafile plugin:
llm install llm-llamafileAll this plugin does is configure a model called llamafile
that attempts to access the model hosted on port 8080. You can run prompts like this:
Here are three neat characteristics of a pelican:
Unique Beak: Pelicans have a distinctive beak that is shaped like a scoop or a basket. This beak is specially designed to catch fish, and it can hold up to 3 gallons of water! The beak is also very sensitive, which helps pelicans detect the presence of fish in the water.
Waterproof Feathers: Pelicans have a special coating on their feathers that makes them waterproof. This is essential for their fishing lifestyle, as they need to be able to dive into the water without getting weighed down by wet feathers. The coating is made up of a waxy substance that helps to repel water.
Pouch-Like Throat: Pelicans have a unique throat pouch that allows them to catch and store fish. When they dive into the water, they use their beak to scoop up fish, and then they store them in their throat pouch. The pouch can expand to hold multiple fish, and the pelican can then swallow the fish whole or regurgitate them to feed their young. This pouch is a key adaptation that helps pelicans thrive in their aquatic environment.
If you don't want to install another plugin, you can instead configure the model by adding this to your openai-extra-models.yaml
file:
One warning about this approach: if you use LLM like this then every prompt you run through llamafile
will be stored under the same model name in your SQLite logs, even if you try out different llamafile
models at different times. You could work around this by registering them with different model_id
values in the YAML file.
A neat thing about open weight models is that multiple API providers can offer them, encouraging them to aggressively compete on price.
Groq is currently free, but that's with a limited number of free requests.
A number of other providers are now hosting Llama 3, and many of them have plugins available for LLM. Here are a few examples:
Perplexity Labs are offeringllama-3-8b-instruct
and llama-3-70b-instruct
. The llm-perplexity plugin provides access - llm install llm-perplexity
to install, llm keys set perplexity
to set an API key and then run prompts against those two model IDs. Current price for 8b is $0.20 per million tokens, for 80b is $1.00.
Anyscale Endpoints have meta-llama/Llama-3-8b-chat-hf
($0.15/million tokens) and meta-llama/Llama-3-70b-chat-hf
($1.0/million tokens) (pricing). llm install anyscale-endpoints
, then llm keys set anyscale-endpoints
to set the API key.
Fireworks AI have fireworks/models/llama-v3-8b-instruct
for $0.20/million and fireworks/models/llama-v3-70b-instruct
for $0.90/million (pricing). llm install fireworks
, then llm keys set fireworks
to set the API key.
OpenRouter provide proxied accessed to Llama 3 from a number of different providers at different prices, documented on their meta-llama/llama-3-70b-instruct and meta-llama/llama-3-8b-instruct pages (and more). Use the llm-openrouter plugin for those.
Together AI has both models as well. The llm-together plugin provides access to meta-llama/Llama-3-8b-chat-hf
and meta-llama/Llama-3-70b-chat-hf
.
I'm sure there are more - these are just the ones I've tried out myself. Check the LLM plugin directory for other providers, or if a provider emulates the OpenAI API you can configure with the YAML file as shown above or described in the LLM documentation.
That's a lot of optionsOne key idea behind LLM is to use plugins to provide access to as many different models as possible. Above I've listed two ways to run Llama 3 locally and six different API vendors that LLM can access as well.
If you're inspired to write your own plugin it's pretty simple: each of the above plugins is open source, and there's a detailed tutorial on Writing a plugin to support a new model on the LLM website.
Another discovery from the archives … although only March, so not so bad.
Many of us seek status and belonging without even seeing it; we find it hard not to react to what others are doing, especially those we have been programmed to want to impress. We’re reactive without realizing it. We put limits on ourselves because we buy into other people’s rules. It’s the fundamental limit I’ve observed in most people, including myself and most founders: they define success by succeeding according to the rules that others have defined.
💬 Mike Maples
🔗 Source (Medium) - so heaven only knows how I stumbled on this one - other than Mike is actually very good at what he does - so maybe I was looking out from him?
The Santa Barbara News-Press was born in 1868 and died in 2023 at age 155. Its glory years ran from 1932 until 2000, when the New York Times sold it to Wendy McCaw, who rode it to hell.
That ride began with the Santa Barbara News Press Controversy in 2006 and ended when Ampersand, the company McCaw created to hold the paper’s bag of assets (which did not include its landmark building downtown, which McCaw kept), filed for Chapter 7 bankruptcy in late July of last year. Here are stories about the death of the paper in three local news journals that have done a great job of taking up the slack left when the News-Press began to collapse, plus one in the LA Times:
‘Santa Barbara News-Press’ Files for Bankruptcy: Publisher Ampersand Claims Few Assets and Many Creditors, by Jean Yamamura in the Santa Barbara Independent (July 23, 2023) Santa Barbara News-Press Declares Bankruptcy, Staff Told All Jobs ‘Eliminated’, by Joshua Molina, in Noozhawk (July 23, 2023) Santa Barbara News-Press Files for Bankruptcy, Staff Fired, by Edhat Staff (July 24, 2023) Santa Barbara News-Press bankruptcy brings uneasy end to an owner’s bitter tenure, by James Rainey, in the Los Angeles Times. (July 29, 2023)I followed those with this in We Need Deep News:
From what I’ve read so far (and I’d love to be wrong) none of those news reports touch on the subject of the News-Press‘ archives, which conceivably reach back across the century and a half it was published. There can’t be a better first draft of history for Santa Barbara than that one. If it’s gone, the loss is incalculable. (August 18 2023)
Last month brought bad news about that:
‘Santa Barbara News-Press’ Online Assets to Be Sold: Bankruptcy Sale for $250,000 Subject to Bids in April, by Jean Yamamura in the Santa Barbara Independent March 8, 2024 A local paper went bankrupt. Now a faraway buyer wants its assets: The Santa Barbara News-Press’s digital assets are up for sale. Locals worry they could become a farm for AI-generated SEO bait. By Ernie Smith in Fast Company. (March 5, 2024) Santa Barbara’s Collective Memory, Sold for Kindling: Will ‘NewsPress.Com’ Become a Zombie Website? by William Belfiore in the Independent. (March 29, 2024)But then, thanks to William Belfiore’s appeal in that last piece, we learned this:
‘Santa Barbara News-Press’ Website Goes to ‘Local Kids’ Group Fronted by Ben Romo Makes Winning Auction Bid of $285,000, by Jean Yamamura in the Independent (Apr 09, 2024)The only mention of archives was in the closing sentences of that piece:
The purchase of the website included the Santa Barbara News-Press trademark, which would be important to the groups looking at the physical archive of back issues, photographs, and clippings by topic. Romo, who was once a paper boy for the daily, acknowledged that his group was supportive of the archive remaining local, too.
I don’t know what that means, and I haven’t checked. But I am sure that the archives ought to be managed by the community as a common pool resource.
As it happens, my wife and I are visiting scholars at the Ostrom Workshop at Indiana University, which is concerned with this kind of thing, because its namesake, Elinor Ostrom, won a Nobel Prize in Economics for her work on how commons are self-governed. In her landmark book, Governing the Commons: The Evolution of Institutions for Collective Action, she lists eight principles for managing a commons, which are summarized here:
Define clear group boundaries. Match rules governing use of common goods to local needs and conditions. Ensure that those affected by the rules can participate in modifying the rules. Make sure the rule-making rights of community members are respected by outside authorities. Develop a system, carried out by community members, for monitoring members’ behavior. Use graduated sanctions for rule violators. Provide accessible, low-cost means for dispute resolution. Build responsibility for governing the common resource in nested tiers from the lowest level up to the entire interconnected system.Journalists, especially those who report news, are not herding animals. They tend to be competitive and territorial by both nature and purpose. So the collection of news entities I wrote about in We Need Wide News and We Need Whole News will almost certainly not cohere into a commons such as Lin (her nickname) Ostrom addresses in that list.
But they should cohere around archives—not only because that’s the right thing to do, but because they need those archives. We all do.
So I hope Santa Barbara’s many journals, journalists, friends, supporters, and interested local institutions get together around this challenge. Build a commons around those archives, whatever and wherever they happen to be.
Meanwhile here in Bloomington, my other hometown, we are pushing forward with The Online Local Chronicle that Dave Askins wrote about in the previous installment in this series. We might call that a commons interest here.
I’m Arkansas bound in the morning, so I don’t have time for a long post. All I have time for is to share a video with musings about the second part of my trip north and the photos that go along with it. Keep me in your prayers these next few weeks. I’m going to be putting a lot of miles behind me.
Doing Pages.
Using Pages.
Now Pages : about nownownow.com.
Any other pages we need to know about?
TIL
Dubai has a unique demographic model. Of its 3.5 million people, 92% of are foreigners who come from 200 countries to live and work in the city, lured by its tax-free status and relaxed lifestyle.
92% are foreigners … 🤯
Wikidata has pages for huge numbers of concepts, people, places and things.
One of the many pieces of data they publish is QRank—“ranking Wikidata entities by aggregating page views on Wikipedia, Wikispecies, Wikibooks, Wikiquote, and other Wikimedia projects”. Every item gets a score and these scores can be used to answer questions like “which island nations get the most interest across Wikipedia”—potentially useful for things like deciding which labels to display on a highly compressed map of the world.
QRank is published as a gzipped CSV file.
Colin’s hikeratlas/qrank GitHub repository runs weekly, fetches the latest qrank.csv.gz file and loads it into a SQLite database using SQLite’s “.import” mechanism. Then it publishes the resulting SQLite database as an asset attached to the “latest” GitHub release on that repo—currently a 307MB file.
The database itself has just a single table mapping the Wikidata ID (a primary key integer) to the latest QRank—another integer. You’d need your own set of data with Wikidata IDs to join against this to do anything useful.
I’d never thought of using GitHub Releases for this kind of thing. I think it’s a really interesting pattern.
This means you can bundle an offline map of the world as part of any application that doesn’t need a higher level of detail. A lot of smaller island nations are missing entirely though, so this may not be right for every project.
It even includes a service worker to help implement offline mapping support, plus several variants of the map with less cities that are even smaller.
Via Hacker News
Probably only works on Ubuntu.
From the FAQ: “Q: Why did you make this? A: I thought it would be funny.”
Via @willmcgugan
We who care about journalism are asked to join the Save Journalism Project, and its fight against Big Tech. Their pitch begins,
and adds,
On the first point, we should note that journalists have been working for magazines, broadcasters, newsletters and themselves for many dozens of years. So journalism isn’t just about newspapers. Also, because so many journalists have long made livings in those other media, the loss of work is far greater than the 2,400 gone from newspapers. It’s truly massive. I don’t know any field where the loss of paying jobs is larger on a percentage basis. Not taxi driving, not hospitality, not retail, not manufacturing… not anything I can think of. (Well, maybe nuns. I don’t see many of those these days.)
We should also respect the simple fact that now there is more journalism than ever: in blogs, social media, podcasting, and other places. Most of those kinds of journalism don’t pay, but that doesn’t disqualify the work from the label. Hell, I’m committing journalism here and this doesn’t pay.
“The story of big tech’s threat to journalism” (what the Project wants us all to tell) is also something of a red herring because it distracts our attention from causes much bigger than Big Tech.
Every new technology “works us over completely,” Marshall McLuhan says (in The Medium is the Massage). And no new medium, no new technologies, have ever worked us more than the digital kind. The change began with digital tech and integrated circuits and then went absolute with the Internet. Together, digital technologies and the Internet have radiacally changed our species, our civilization, and our planet.
Not long ago, in a conversation about this with Joi Ito, I asked him how big he thought the digital transformation was. Bigger than broadcast? Print? Writing? Speech? Stone tools?
“No,” he replied. “It’s the biggest thing since oxygenation.” In case you don’t remember, that happened between about two and a half billion years ago. (Joi also writes about it here.)
So, while journalism matters enormously, it’s just one casualty of digitalization. And, let’s face it, a beneficiary as well. Either way, we need to understand the whole picture, which is about a lot more than what journalism sees happening in the mirror.
Here’s one outfit working on that bigger picture. I‘m involved with it.
I also don’t expect most journalists to take much interest in the subject, because it’s too big, and it doesn’t make full sense as a story, which is journalism’s stock in trade. (I explain a bit about journalism’s “story problem” in this TEDx talk.)
Still, some journalists are on the case, including me. Love to have others join in. But please don’t bother if you think Big Tech is alone to blame. Because the story is bigger than that, and far more than a story.
I just copied and pasted this post from here in Medium, where I posted it in July 2019. It expands on a post now archived here. It’s kinda sad that not much has changed over all that time.
The blog post announcing the shutdown was done one day early. The idea was to take the opportunity of the new Pope being announced and Andy Rubin being replaced as head of Android, so that the [Google] Reader news may be drowned out. PR didn't apparently realize that the kinds of people that care about the other two events (especially the Pope) are not the same kind of people that care about Reader, so it didn't work.
Tips on Adding JSON Output to Your CLI App
Kelly Brazil - also the author ofjc
, the neat CLI tool that converts the output of common Unix utilities such as dig into JSON - provides some useful do's and don'ts for adding JSON output as an option to a command-line tool.
Kelly recommends defaulting to arrays of flat objects - or newline-delimited objects - and suggests including an "unbuffer" option for streaming tools that discourages the OS from buffering output that is being sent through a pipe.
Via Hacker News
llm -m Meta-Llama-3-8B-Instruct "say hi in Spanish"
I like mail-in ballots because I can do my research as I go, on my own time, in my own environment. To me, it feels a lot closer to giving the process the time and attention it deserves.
I filled in my Pennsylvania primary ballot this morning. I’d never been a British voter (I’m not a British citizen), so moving to the US represented the first time I could actually vote for my representatives in the country I lived in.
Primaries are sort of a funny idea: you pledge yourself to a party ahead of time, and that party affiliation is public. It seems to me to be a little counter to the idea of a private ballot, which is the cornerstone of free and fair elections, but that’s the system we have. If you’re a registered voter for a party, you can vote in its primary, which helps to select which candidates will actually make it to the general election.
I already knew how I’d cast my vote for the big-ticket item — the President of the United States — but I had very little idea about down-ballot candidates like the Attorney General or the Treasurer. So I sat down with my laptop and, person by person, checked them out on:
Ballotpedia Spotlight PA WHYY (my local public media station) Progressive and restorative justice groups like Reclaim Philadelphia Their own websites A general Google News searchRather than look to a central party recommendation or trust one set of endorsements, I prefer to form my own opinions, triangulating between sources that care about the same things I do. I explicitly seek out criticism, including from the opposition party and opposing points of view, because even if I don’t agree with someone’s political position, they may bring up flaws that a candidate’s own party would not highlight.
And then I seal it up in an envelope and send it off. It feels good to vote. It feels important to vote (particular in this era). I’ll be crossing my fingers for the most compassionate, inclusive, peaceful outcome from our set of possible outcomes in November.
This is exactly what I'd hope to see from a standards organization like the W3C:
"W3C leads the community in defining a World Wide Web that puts users first, by developing technical standards and guidelines to empower an equitable, informed, and interconnected society.
The fundamental function of W3C today is to provide an open forum where diverse voices from around the world and from different organizations and industries work together to evolve the web by building consensus on voluntary global standards for Web technologies."
There's no shirking away from the importance of equity or diversity: it's right there in the first two paragraphs of the vision, and stated again further down in more detail:
"Diversity: We believe in diversity and inclusion of participants from different geographical locations, cultures, languages, disabilities, gender identities, industries, organizational sizes, and more. In order to ensure W3C serves the needs of the entire Web user base, we also strive to broaden diversity and inclusion for our own participants."
This is exactly as it should be, and it makes me really happy to see it. Of course, this is only a draft of sorts from the working group; it needs to now get consensus from the wider organization. I hope its pro-human focus remains front and center. #Technology
[Link]
So much of what we build on the web is about connecting people.
It is impossible to connect people effectively without paying attention to social justice and equity.
Otherwise we’re just connecting the privileged with the privileged, creating ever smaller networks of influence, and learning nothing new.
Solidarity with the 28 Google workers who were fired for protesting Project Nimbus this week. Anonymous Google and Amazon workers described the project as follows a couple of years ago:
Project Nimbus is a $1.2bn contract to provide cloud services for the Israeli military and government. This technology allows for further surveillance of and unlawful data collection on Palestinians, and facilitates expansion of Israel’s illegal settlements on Palestinian land.
I have never worked for Google or Amazon, but I would like to think that I would have protested too.
There is nothing honorable about supporting your employer as it commits or facilitates human rights violations. Protesting is the ethical thing to do, particularly when you hold deeply-held beliefs like these:
We cannot look the other way, as the products we build are used to deny Palestinians their basic rights, force Palestinians out of their homes and attack Palestinians in the Gaza Strip – actions that have prompted war crime investigations by the international criminal court.
Human rights should always trump business.
Further to that, apparently some of these 28 workers hadn’t even protested — they’d just associated with the people who had:
Yeah, this was retaliation, like completely indiscriminate—people who had just walked by just to say hello and maybe talk to us for a little bit. They were fired. People who aren't affiliated with No Tech For Apartheid at all, who just showed up and were interested in what was going on. And then security asked to see their badge and they were among the 28 fired.
Not a good look, to say the least. The same goes for the scores of tech workers who — on a cursory glance of social media — seem to have been derisive of the protests. Shame on you.
"Too often, [...] we assume that the person who is the object of our feedback has something to learn and fail to notice the same thing about ourselves."
This is a really important observation. Without checking ourselves, our feedback can be a way to ensure our vision remains myopic, and can enforce a kind of conservatism that (like all conservatism, I believe) ultimately serves nobody.
Feedback is important, but curiosity, care, and respect are even more important. Starting off with an assumption that our colleague might know something we don't - and a need to get to the "why" of everything we notice, and consider what we might not be noticing - is more than healthy. #Business
[Link]
Ruff v0.4.0: a hand-written recursive descent parser for Python
The latest release of Ruff—a Python linter and formatter, written in Rust—includes a complete rewrite of the core parser. Previously Ruff used a parser borrowed from RustPython, generated using the LALRPOP parser generator. Victor Hugo Gomes contributed a new parser written from scratch, which provided a 2x speedup and also added error recovery, allowing parsing of invalid Python—super-useful for a linter.I tried Ruff 0.4.0 just now against Datasette—a reasonably large Python project—and it ran in less than 1/10th of a second. This thing is Fast.
Their new "overturemaps" Python CLI utility makes it easy to quickly pull subsets of their data... but requires you to provide a bounding box to do so.
Drew Breunig came up with this delightful recipe for fetching data using LLM and gpt-3.5-turbo to fill in those bounding boxes:
overturemaps download --bbox=$(llm 'Give me a bounding box for Alameda, California expressed as only four numbers delineated by commas, with no spaces, longitude preceding latitude.') -f geojsonseq --type=place | geojson-to-sqlite alameda.db places - --nl --pk=id
Via @dbreunig
John Bradley and I convened a session on Trust Establishment with OpenID Federation at the Internet Identity Workshop (IIW) on Thursday, April 18, 2024. The material used to drive the discussion was:
Trust Establishment with OpenID Federation (PowerPoint) (PDF)The session was well attended and the discussion lively. Numerous people with trust establishment problems to solve contributed, including experts from the SAML federation world, people involved in digital wallet projects, and several people already using or considering using OpenID Federation. Thanks to all who participated!
Andrej Karpathy's Llama 3 review
The most interesting coverage I’ve seen so far of Meta’s Llama 3 models (8b and 70b so far, 400b promised later).Andrej notes that Llama 3 trained on 15 trillion tokens—up from 2 trillion for Llama 2—and they used that many even for the smaller 8b model, 75x more than the chinchilla scaling laws would suggest.
The tokenizer has also changed—they now use 128,000 tokens, up from 32,000. This results in a 15% drop in the tokens needed to represent a string of text.
The one disappointment is the context length—just 8,192, 2x that of Llama 2 and 4x LLaMA 1 but still pretty small by today’s standards.
If early indications hold, the 400b model could be the first genuinely GPT-4 class openly licensed model. We’ll have to wait and see.
How cheap, outsourced labour in Africa is shaping AI English
The word “delve” has been getting a lot of attention recently as an example of something that might be an indicator of ChatGPT generated content.One example: articles on medical research site PubMed now use “delve” 10 to 100 times more than a few years ago!
Nigerian Twitter took offense recently to Paul Graham’s suggestion that “delve” is a sign of bad writing. It turns out Nigerian formal writing has a subtly different vocabulary.
Alex Hern theorizes that the underlying cause may be related. Companies like OpenAI frequently outsource data annotation to countries like Nigeria that have excellent English skills and low wages. RLHF (reinforcement learning from human feedback) involves annotators comparing and voting on the “best” responses from the models.
Are they teaching models to favour Nigerian-English? It’s a pretty solid theory!
The recent attempted XZ Utils backdoor (CVE-2024-3094) may not be an isolated incident as evidenced by a similar credible takeover attempt intercepted by the OpenJS Foundation, home to JavaScript projects used by billions of websites worldwide. The Open Source Security (OpenSSF) and OpenJS Foundations are calling all open source maintainers to be alert for social engineering takeover attempts, to recognize the early threat patterns emerging, and to take steps to protect their open source projects.
Vigilance is good, and it’s worth heeding the advice and paying attention to the evidence presented here. The XZ Utils backdoor was a smart attack that very nearly caused havoc.
I think it’s also worth pointing out that we know about the exploit because it was in an open source project. Andres Freund was debugging a server resource issue when he uncovered the issue. Because the project — and its downstream client — were open source, he could investigate and find the intrusion.
It’s not clear how this would have panned out if this had been proprietary software: particularly on a team that was resource strapped or moving at speed. The same social engineering exploits that allowed Jia Tan to become a maintainer of the XZ Utils project would also see someone hired as a contractor by a tech team. If I was a nefarious actor who wanted to place an exploit in an important software library, that’s exactly what I’d do: go send someone to join the team as a contractor. While there are mandatory identity verification procedures for full-time employees (which we can certainly argue the pros and cons of), contractors have no such requirements.
I bring this up because all the advice I’ve seen to date has been directed at open source maintainers. Again, this is smart and good and should absolutely be heeded — but there’s a world of other software out there that is also critical infrastructure and which doesn’t enjoy the sunlight of open source projects. This isn’t an open source software problem; it’s a software problem. Everyone should be vigilant, regardless if there are eyes on their source code or not. And perhaps we should be even warier of projects whose code we can’t audit ourselves.
Der EU-Datenschutzausschuss hat in einer gestern veröffentlichten Stellungnahme festgestellt, „dass es großen Online-Plattformen in den meisten Fällen nicht möglich sein wird, die Anforderungen an eine gültige Einwilligung zu erfüllen, wenn sie die Nutzer nur vor die Wahl stellen, entweder in die Verarbeitung personenbezogener Daten für verhaltensbezogene Werbung einzuwilligen oder eine Gebühr zu zahlen.“ Der Europaabgeordnete der Piratenpartei Dr. Patrick Breyer bewertet das als faktisches Aus von Meta’s „pay or okay“-Plan, gegen den Verbraucherorganisationen bereits vor Gericht klagen. Zuvor hatten bereits 46 Europaabgeordnete auf Breyers Initiative dieses Bezahlmodell verurteilt, das Nutzern vor die Wahl zwischen Überwachung und Bezahlabo stellt.
„Metas Datenschutzgebühr untergräbt den Schutz der Privatsphäre, stellt Nutzer vor eine falsche Wahl und übt wirtschaftlichen Zwang zur Einwilligung in eine umfassende Überwachung und Ausbeutung der Onlineaktivitäten des Nutzer aus. Zuckerbergs Konzern wird nicht damit durchkommen, die Nutzer zur Einwilligung in diese Ausbeutung zu zwingen, indem er Datenschutz unbezahlbar macht. Die Ursache dafür, dass Meta auf einem rechtswidrigen Einwilligungsmodell besteht, ist sein auf allgegenwärtigem Tracking beruhendes Geschäftsmodell. Wie jetzt auch die EU-Datenschützer schreiben, muss Meta eine für den Nutzer kostenfreie Alternative zu diesem überwachungskapitalistischen Geschäftsmodell entwickeln, etwa aufbauend auf kontextbezogener und überwachungsfreier Werbung. Meta muss jetzt einlenken, sein ‘Pay or okay’-System aufgeben und endlich unser Grundrecht auf anonyme Internetnutzung respektieren!”
I have a child who is also 2e and has been part of the NYC G&T program. We've had a positive experience with the citywide program, specifically with the program at The Anderson School.
I am convinced that ActivityPub is going to change the entire web.
"I find my feelings about AI are actually pretty similar to my feelings about blockchains: they do a poor job of much of what people try to do with them, they can't do the things their creators claim they one day might, and many of the things they are well suited to do may not be altogether that beneficial."
This description of the uses and pitfalls of the current generation of AI tools is a characteristically sharp breakdown from Molly White.
I've found AI useful for similar sorts of things: proofreading in particular. But I agree with her conclusions - in fact, I agree with every single point she brings up in this piece. One to share with your colleagues who are thinking about deeply integrating LLMs based on the hype cycle alone. #Technology
[Link]
Ein gestern vom Nachrichtenportal netzpolitik.org geleaktes Dokument enthüllt erstmals, wann Messenger- und Chatdiensten nach dem Vorschlag des EU-Ratsvorsitzes ein „hohes Risiko“ bescheinigt werden soll, was den Weg für ihre Verpflichtung zur Chatkontrolle frei machen soll. Zur Chatkontrolle führen kann danach, dass ein Dienst „anonyme Profile oder Zugriffe ohne Benutzerkonto“ ermöglicht, dass „verschlüsseltes Messaging“ angeboten wird oder dass überhaupt interaktive Funktionen wie Direktnachrichten, Posts oder Nutzerkommentare angeboten werden. Das Dokument stammt vom Februar, jedoch sieht eine aktualisierte Fassung vom 10. April ähnliche Kriterien vor.
„Ausgerechnet die bisher datenschutzfreundlich anonym nutzbaren Kommunikationsdienste wie Protonmail sollen per Verpflichtung zur Chatkontrolle zu den extremüberwachtesten Diensten werden. Ausgerechnet die bisher sicher verschlüsselten Messengerdienste wie Signal sollen durch verpflichtendes ‚client-side scanning‘ zu Spionen auf unseren Smartphones werden“, prangert der Europaabgeordnete der Piratenpartei und Schattenberichterstatter zur Chatkontrolle Dr. Patrick Breyer an. „Die EU-Regierungen wollen gegen Vertraulichkeit und Sicherheit unserer digitalen Kommunikation insgesamt in den Krieg ziehen. Alles, was das Internet und digitale Kommunikation ausmacht und moderne Lebensrealität ist, ist für die EU-Innenminister ein zu bekämpfendes ‚Risiko‘. Wir Piraten werden nicht aufhören, für unser Grundrecht auf digitales Briefgeheimnis und sichere Verschlüsselung zu kämpfen – genau dafür sind wir digitale Freiheitskämpfer im EU-Parlament.“
„Sichere Verschlüsselung und datensparsame Messenger sind in den Fokus der Überwachung gerückt“, bewertet die Informatikerin und Spitzenkandidatin der Piratenpartei zur Europawahl Anja Hirschel. „Genau wie befürchtet sollen also Nachrichten vor der Transportverschlüsselung direkt auf den Endgeräten mitgelesen werden. Anbieter können sich zukünftig dieser Verpflichtung, einen Zugriff zu ermöglichen, dann nicht mehr entziehen. Bisher sichere Kommunikationswege können dann mit einem Update zum persönlichen ‚Taschenspion‘ werden.”
"Virtually every single part of the self-publishing grift world that can be automated or monetized has been automated and monetized."
This is a really depressing read: fascinating, for sure, but what's left unsaid is what happens to traditional publishing as these folks become more and more successful, and book marketplaces become more and more saturated.
Or perhaps it'll drive everyone to real-life bookstores? There, at least, I know I'm not going to run into the kind of trash sold by Big Luca or the Mikkelsen Twins. #Media
[Link]
As has become traditional, I gave the following presentation at the Monday, April 15, 2024 OpenID Workshop at Google:
OpenID Connect Working Group Update (PowerPoint) (PDF)I also gave this invited “101” session presentation at the Internet Identity Workshop (IIW) on Tuesday, April 16, 2024:
Introduction to OpenID Connect (PowerPoint) (PDF)People aren't digitally embodied. As a result their online relationships are anemic. As we think about the kinds of digital identity systems that will help people live authentic online lives, we must build identity system that give people tools to operationalize rich digital relationships.
Identity is the ability to recognize, remember, and react to people, organizations, systems, and things. In the current web, companies employ many ponderous technological systems to perform those functions. In these systems, we are like ghosts in the machines. We have "accounts" in companies' systems, but no good way to recognize, remember, and react to them or anyone else. We are not digital embodied.
One of great benefits of embodiment is the ability to form and operationalize rich digital relationships. I've written a lot about the nature of digital relationships.
Authentic Digital Relationships
Operationalizing Digital Relationships
Relationships in the Self-Sovereign Internet of Things
The Architecture of Identity Systems
Are Transactional Relationships Enough?
One of the discussions at VRM Day caused me to think about a feature of digital relationships I hadn't considered before. Someone said that if you think about a graph with people (or things, organizations, and so on) as the nodes, the relationships are the edges, like so:
Bi-directional relationshipIn this figure Alice and Bob have a bi-directional relationship. This is how I've normally thought about it and how I'd have drawn it. But in today's discussion, someone said that the relationship is shared and that Alice and Bob both control it. But I realized that viewpoint is too simple. Specifically, Alice and Bob each have a different perspective of that relationship and will use it separately.
For example, imagine that Alice is the cashier at a grocery store and Bob is a customer. Alice gives great service, so Bob seeks her out when he shops. Alice on the other hand has no particular recollection of Bob from encounter to encounter. For Alice, the relationship is ephemeral, but for Bob, it's longer term. The nature of each relationship is different. So, we might look at it like this[1]:
Two, uni-directional relationshipsBut after discussing it some more, I realized that these relationships aren't independent. They're entangled like this:
Entangled relationshipsIn the example I gave above, as Bob seeks out Alice more and more, Alice might come to recognize him and call him by name, changing the nature of her relationship with Bob. And that may influence the nature of Bob's relationship with Alice. Over time, these interactions influence both relationships. So, while Alice and Bob both have control over their relationship with the other, actions by one influence the other.
I frequently say that we don't build identity systems to manage identities, but rather to manage relationships. The problem with contemporary identity systems is that they are all one sided, controlled by one party—almost always a company. As I've said before, people are not digital embodied and thus have no good way to manage their online relationships. As we strive to build better digital identity systems, I think it's paramount that we build systems that provide people with tools that embody them and provide them with the ability to operationalize their online relationships. These are more than decentralized; they are self-sovereign.
NotesPeer decentralized identifiers (DIDs) are a great technology for creating bi-directional relationships.
"I am absolutely convinced that journalism’s most essential role at this critical moment goes far, far beyond what it’s doing. The status quo in political (and related) coverage consists of sporadically noting that gosh-maybe-there’s-a-problem, while sticking mostly to journalistic business as usual. The status quo is journalistic malpractice."
A strong implied call to action from Dan Gillmor, who has long argued for a more principled journalism industry (alongside a more principled software ecosystem that supports it). #Media
[Link]
Coming up this week: the fourth installment of the Postgres extension ecosystem mini-summit. The topic: Trusted Language Extensions, a.k.a. TLEs. Jonathan Katz of AWS will give a brief presentation on “TLE Vision and Specifics” followed by community discussion of the issues TLEs aim to address, what works and what doesn’t, and the role of TLEs in the future of the extension ecosystem.
Join us! Note! that if you reserved a spot at a prior
mini-summit, sadly you will need to do so again for each subsequent summit or
miss out on reminders from Eventbrite. And if Eventbrite says the event is
sold out, rest assured we have plenty more virtual seats! Just send at
david@
this domain, ping me on Mastodon or via the #extensions channel
on the Postgres Slack or the Postgres Discord.
Nachdem neuesten, vom Informationsdienst Contexte geleakten Gesetzentwurf zur Chatkontrolle, den die EU-Mitgliedsstaaten heute diskutieren, wollen die EU-Innenminister Accounts der Angehörigen von Sicherheitsbehörden wie Geheimdienste, Polizei und Militär von der geplanten Chatkontrolle ausnehmen (Artikel 1 Absatz 2a). Die Verordnung soll auch auf „vertrauliche Informationen“ wie Berufsgeheimnisse keine Anwendung finden (Artikel 1 Absatz 2b). Weiterhin lehnen es die EU-Regierungen ab, dass das geplante EU-Kinderschutzzentrum sie bei der Prävention sexuellen Kindesmissbrauchs unterstützt und Best Practices für Präventionsinitiativen ausarbeitet (Artikel 43 Absatz 8).
„Dass die EU-Innenminister Polizisten, Soldaten, Geheimdienstler und auch sich selbst von der Chatkontrolle ausnehmen wollen beweist, dass sie wissen, wie unzuverlässig und gefährlich die Schnüffelalgorithmen sind, die sie auf uns Bürger loslassen wollen“, kommentiert die Europaabgeordnete der Piratenpartei Dr. Patrick Breyer. „Offensichtlich rechnen sie damit, dass selbst Militärgeheimnisse ohne jeglichen Bezug zu Kindesmissbrauch jederzeit in den USA landen könnten. Die Vertraulichkeit der Regierungskommunikation ist natürlich wichtig, aber dasselbe muss für den Schutz der Wirtschaft und natürlich der Bürgerinnen und Bürger gelten, einschließlich der Räume, die Missbrauchsopfer selbst für einen geschützten Austausch und Therapie brauchen. Wir wissen, dass die meisten von den heute noch freiwilligen Schnüffelalgorithmen geleakten Chats ohne jede Bedeutung für die Polizei sind, zum Beispiel Familienfotos oder einvernehmliches Sexting. Es ist eine Unverschämtheit, dass die EU-Innenminister die Folgen der Zerstörung des digitalen Briefgeheimnisses und sicherer Verschlüsselung, die sie uns zumuten, selbst nicht ausbaden wollen.“
Die Informatikerin und Spitzenkandidatin der Piratenpartei zur Europawahl Anja Hirschel ergänzt: „Die weitere Formulierung, dass Berufsgeheimnisse von der Chatkontrolle nicht betroffen sein sollen, ist eine in Paragrafen gegossene Lüge. Kein Anbieter und kein Algorithmus kann wissen oder feststellen, ob ein Chat mit Ärzten, Therapeuten, Anwälten, Strafverteidigern usw. geführt wird, um ihn von der Chatkontrolle auszunehmen. Die Chatkontrolle droht unweigerlich auch Intimfotos für Behandlungszwecke und Prozessunterlagen zur Verteidigung von Missbrauchsopfern an Personen zu leaken, bei denen sie nichts zu suchen haben und bei denen sie nicht sicher sind.“
Breyer erklärt abschließend: „Dem offiziellen Ziel des Kinderschutzes spricht es Hohn, dass die EU-Innenminister ausgerechnet bei der Vorbeugung von Kindesmissbrauch die Ausarbeitung von Best Practices ablehnen. Deutlicher kann man nicht machen, dass Ziel der Chatkontrolle Massenüberwachung mit chinesischen Methoden ist und nicht ein besserer Schutz unserer Kinder. Wer echten Kinderschutz will, würde eine systematische wissenschaftliche Evaluierung und Umsetzung multidisziplinärer Präventionsprogramme auf den Weg bringen, ebenso wie europaweite Standards und Leitlinien für strafrechtliche Ermittlungen wegen Kindesmissbrauchs, einschließlich der Identifikation von Opfern und der nötigen technischen Mittel. Nichts davon planen die EU-Innenminister.“
Die EU-Regierungen wollen die Chatkontrolle bis Anfang Juni beschließen.
This article shows how to implement a secure .NET 8 Blazor Web application using OpenID Connect and security headers with CSP nonces. The NetEscapades.AspNetCore.SecurityHeaders nuget package is used to implement the security headers and OpenIddict is used to implement the OIDC server.
Code: https://github.com/damienbod/BlazorWebOidc
OpenIddict is used as the identity provider and an OpenID connect client is setup to allow an OpenID Connect confidential code flow PKCE client. The Web application is a server rendered application using Blazor server components implemented using Blazor Web, ASP.NET Core and .NET 8.
Step 1: Init solution from the .NET Blazor samplesThe solution was created using the Blazor samples from Microsoft. The .NET 8 BlazorWebAppOidc project was used to setup the solution.
https://github.com/dotnet/blazor-samples/tree/main/8.0/BlazorWebAppOidc
The code sample implements the client profile parts and the CSRF protection. Login and Logout plumbing is also implemented.
Step 2: Switch the OpenID Connect serverOpenIddict is used as the identity provider and so the OIDC client set up needs to be changed. The program file was updated and the OpenID Connect Microsoft Entra ID client was replaced with the OpenIddict client. The client on the server is setup directly in the worker class in the Openiddict server. Both of the setups must match. The client uses an OpenID Connect confidential client with code flow and PKCE.
builder.Services.AddAuthentication(OIDC_SCHEME) .AddOpenIdConnect(OIDC_SCHEME, options => { // From appsettings.json, keyvault, user-secrets // "OpenIDConnectSettings": { // "Authority": "https://localhost:44318", // "ClientId": "oidc-pkce-confidential", // "ClientSecret": "--secret-in-key-vault-user-secrets--" // }, builder.Configuration.GetSection("OpenIDConnectSettings").Bind(options); options.SignInScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.ResponseType = OpenIdConnectResponseType.Code; options.SaveTokens = true; options.GetClaimsFromUserInfoEndpoint = true; options.MapInboundClaims = false; // Remove Microsoft mappings options.TokenValidationParameters = new TokenValidationParameters { NameClaimType = "name" }; }) .AddCookie();Note: You could also use the OpenIddict client packages to implement the client. I like to use the defaults.
Step 3: Disable WASM modeAny web application should protect the session, not just implement authentication using an OIDC server. One of the most important browser protection is the CSP header and a good CSP uses a nonce. Blazor Web using WASM does not support this and so this must be disabled. Remove the WASM part from the middleware.
In the program.cs, update Blazor Web to:
builder.Services.AddRazorComponents() .AddInteractiveServerComponents();and
app.MapRazorComponents<App>() .AddInteractiveServerRenderMode() .AddAdditionalAssemblies( typeof(BlazorWebAppOidc.Client._Imports).Assembly);Remove the WASM usage in the UI components. Switch to InteractiveServer mode.
<HeadOutlet @rendermode="InteractiveServer" /> </head> <body> <Routes @rendermode="InteractiveServer" /> Step 4: Add CSP nonce middlewareThe CSP nonce can be used in Blazor (Server) components with some extra effort because the Blazor components cannot read the HTTP headers from the responses. The CircuitHandler class can be used for this. A BlazorNonceService class can be created to add the nonce. This class inherits the CircuitHandler implementation.
using Microsoft.AspNetCore.Components; using Microsoft.AspNetCore.Components.Server.Circuits; namespace BlazorWebAppOidc.CspServices; /// <summary> /// Original src: https://github.com/javiercn/BlazorWebNonceService /// </summary> public class BlazorNonceService : CircuitHandler, IDisposable { private readonly PersistentComponentState _state; private readonly PersistingComponentStateSubscription _subscription; public BlazorNonceService(PersistentComponentState state) { if (state.TryTakeFromJson("nonce", out string? nonce)) { if (nonce is not null) { Nonce = nonce; } else { throw new InvalidOperationException( "Nonce can't be null when provided"); } } else { _subscription = state.RegisterOnPersisting(PersistNonce); } _state = state; } public string? Nonce { get; set; } private Task PersistNonce() { _state.PersistAsJson("nonce", Nonce); return Task.CompletedTask; } public void SetNonce(string nonce) { ArgumentException.ThrowIfNullOrWhiteSpace(nonce); if (Nonce != null) { throw new InvalidOperationException("Nonce already defined"); } Nonce = nonce; } public void Dispose() => ((IDisposable)_subscription)?.Dispose(); }A NonceMiddleware ASP.NET Core middleware service can now be used to read the nonce from the headers and set this in the BlazorNonceService CircuitHandler implementation. NetEscapades.AspNetCore.SecurityHeaders is used to implement the security headers and if a CSP nonce is created, the NETESCAPADES_NONCE http header is set.
namespace BlazorWebAppOidc.CspServices; public class NonceMiddleware { private readonly RequestDelegate _next; public NonceMiddleware(RequestDelegate next) { _next = next; } public async Task Invoke(HttpContext context, BlazorNonceService blazorNonceService) { var success = context.Items .TryGetValue("NETESCAPADES_NONCE", out var nonce); if (success && nonce != null) { blazorNonceService.SetNonce(nonce.ToString()!); } await _next.Invoke(context); } }The middleware for the nonce is added to the ASP.NET Core services.
builder.Services.TryAddEnumerable( ServiceDescriptor.Scoped<CircuitHandler, BlazorNonceService>(sp => sp.GetRequiredService<BlazorNonceService>())); builder.Services.AddScoped<BlazorNonceService>();Use the middleware is in the ASP.NET Core pipelines.
app.UseMiddleware<NonceMiddleware>(); Step 5: Add HTTP browser security headersThe NetEscapades.AspNetCore.SecurityHeaders nuget package is used to implement the security headers as best possible for this type of application. The SecurityHeadersDefinitions class implements this. CSP nonces are configuration as well as other security headers.
namespace BlazorWebAppOidc; public static class SecurityHeadersDefinitions { public static HeaderPolicyCollection GetHeaderPolicyCollection( bool isDev, string? idpHost) { ArgumentNullException.ThrowIfNull(idpHost); var policy = new HeaderPolicyCollection() .AddFrameOptionsDeny() .AddContentTypeOptionsNoSniff() .AddReferrerPolicyStrictOriginWhenCrossOrigin() .AddCrossOriginOpenerPolicy(builder => builder.SameOrigin()) .AddCrossOriginResourcePolicy(builder => builder.SameOrigin()) .AddCrossOriginEmbedderPolicy(builder => builder.RequireCorp()) .AddContentSecurityPolicy(builder => { builder.AddObjectSrc().None(); builder.AddBlockAllMixedContent(); builder.AddImgSrc().Self().From("data:"); builder.AddFormAction().Self().From(idpHost); builder.AddFontSrc().Self(); builder.AddBaseUri().Self(); builder.AddFrameAncestors().None(); builder.AddStyleSrc() .UnsafeInline() .Self(); // due to Blazor builder.AddScriptSrc() .WithNonce() .UnsafeEval() // due to Blazor WASM .StrictDynamic() .OverHttps() .UnsafeInline(); // only a fallback for older browsers }) .RemoveServerHeader() .AddPermissionsPolicy(builder => { builder.AddAccelerometer().None(); builder.AddAutoplay().None(); builder.AddCamera().None(); builder.AddEncryptedMedia().None(); builder.AddFullscreen().All(); builder.AddGeolocation().None(); builder.AddGyroscope().None(); builder.AddMagnetometer().None(); builder.AddMicrophone().None(); builder.AddMidi().None(); builder.AddPayment().None(); builder.AddPictureInPicture().None(); builder.AddSyncXHR().None(); builder.AddUsb().None(); }); if (!isDev) { // maxage = one year in seconds policy.AddStrictTransportSecurityMaxAgeIncludeSubDomains(); } policy.ApplyDocumentHeadersToAllResponses(); return policy; } }The security headers are added using middleware as early as possible in the pipeline. I add the headers for all requests.
app.UseSecurityHeaders( SecurityHeadersDefinitions.GetHeaderPolicyCollection( app.Environment.IsDevelopment(), app.Configuration["OpenIDConnectSettings:Authority"]));The CSP nonce can now be used in the Blazor components and scripts can only be read using the nonce. Unsecure scripts or unsecure inline scripts should never be read anywhere in a browser application.
<pre class="wp-block-syntaxhighlighter-code"> <a href="http://_framework/blazor.web.js">http://_framework/blazor.web.js</a> </body> </html> @code { /// <summary> /// Original src: https://github.com/javiercn/BlazorWebNonceService /// </summary> [CascadingParameter] HttpContext Context { get; set; } = default!; protected override void OnInitialized() { var nonce = GetNonce(); if (nonce != null) { BlazorNonceService.SetNonce(nonce); } } public string? GetNonce() { if (Context.Items.TryGetValue("nonce", out var item) && item is string nonce and not null) { return nonce; } return null; } }</pre>The applications can be started and the authentication and the session protection can be validated. Using the WASM mode in Blazor Web requires a weaker security setup and you need to disable the CSP nonces. This is not a good idea.
Linkshttps://github.com/dotnet/blazor-samples/tree/main/8.0/BlazorWebAppOidc
Using a CSP nonce in Blazor Web
https://github.com/damienbod/BlazorServerOidc
4/13(土) 午前8:10-午前8:40にNHKで「最深日本研究~外国人博士の目~」という番組が放映されました。4/20(土) 午前8:39 までNHK+で視聴可能です2では触れられているようです。また、日本における「理想」の実体化としての「カワイイ」の考察などもされています。昨今、アイデンティティとプライバシーの国際標準化の分野でもメタバースが関心の対象になってきているところなので、とても参考になりました。
以下に番組概要のメモと、その後にアイデンティティやプライバシーの観点での考察を記載します。
番組概要番組の概要はこんな感じです。なぐり書きメモなんで整っていませんが、まぁなんとなくは分かるでしょう。NHK+での番組公開終了までは、ぜひ番組をご覧になることをおすすめします。
(出所)NHK+ 「最深日本研究~外国人博士の目~」https://www.nhk.jp/p/ts/RJ5G2XZ4N3/episode/te/J1K96JLJ9K/
導入部 2000年代以降ネット上に広がったもう一つの世界、メタバース。人々はアバターと呼ばれる自らの分身を使い見知らぬ人との出会いを楽しむ。 その中で、日本人コミュニティではバ美肉が目立つ。 スイスの文化人類学者リュドミラ・ブレディキナ氏(31)3はこのことに興味を持つ。 なぜ日本人男性は美少女キャラクターになりたがるのか、その答えを求めて研究 アニメ・和食・J-POP日本文化に注がれる世界からの熱い視線。そして、日本を愛しわれわれには無い目線でより深く日本を知ろうとする外国人研究者 彼らに日本はどう写っているか。バーチャル人類学者がわれわれの知らない日本の真の姿を解き明かす〜最深日本研究 第1章:最新メタバース体験施設を調査 スイスにはこのような施設はない メタバースを体験するためのすべてが揃っている 初心者には案内人もついている。やはり美少女キャラクター 一般視聴者に向けたメタバースの紹介 アバターの選択〜好きなアバターになる〜アイデンティティの表出 バーチャル空間では一瞬で何十万もある世界を移動できる そこで景色を楽しんだり、人々とお酒を飲んで交流したりなど、現実世界と同じように楽しめる メタバースの利用者は世界中でおよそ4億人 花火をましたから見上げるなどここでしかできない体験 第2章:日本のバーチャル世界を研究したい ミラさんがバーチャル世界の研究を始めたのは4年前 研究調査で驚きを持って知った言葉「バ美肉」(バーチャル美少女受肉)男性が美少女キャラを持つときに使われることば 「受肉」には西洋では宗教的な意味があるが、バ美肉たちは宗教に全く関心がない。そこにミラさんは興味を惹かれた。 調査をすると驚くべきことがわかった。男性のおよそ8割が美少女キャラクターを使っている 女性 78.3% 男性 15.2% その他 6.5% その見た目には大きな特徴:アニメに出るような可愛い女の子。これに対して西洋では、クールでセクシー。カワイイとは違って強い女性という印象を受ける。(出所)NHK「最深日本研究~外国人博士の目~」https://www.nhk.jp/p/ts/RJ5G2XZ4N3/episode/te/J1K96JLJ9K/
ミラさん:わたしは西洋で育ち、女性として美しくエレガントでありたいと思っていた。なぜ日本の男性はカワイイと言われたいのか。西洋人としてこの異なる視点をもっと理解したいと思います。 文化人類学の紹介 20世紀初頭に一つの学問領域として確立。 クロード・レヴィ・ストロース ルース・ベネディクト 梅棹忠夫 机に向かうだけではなく、現地に赴き直接人と触れあるフィールドワークという手法で人間とは何かを探求する学問 人類学者は世界各地の民族を訪ね、彼らとともに暮らし、彼らの生活をつぶさに観察、自分の属する社会と比較することで、その共通点や違い、多様性を明らかにしてきた バーチャル世界という新たな地平 最初SNSを使いバ美肉たちに話しかけたが、部外者であるわたしは信用されなかった。わたしが西洋人であり女性だったから。そこで、ミラさんは人類学の手法であるフィールドワークを使ってバ美肉たちに接近。自分のアバターを作ることは重要だった。バーチャル世界で彼らの信用を得ることができたから。 アバターを纏うことでバ美肉たちと同じ目線に立ち直接ふれあい始めたリュドミラ ときには一緒にライブ配信を行い、オリジナル曲の英訳を買って出た そして1年ほどかけて、徐々にコミュニティの中に入っていった 第3章 声までカワイイってどういうこと? 両声類4の紹介 声は男性のままか音声変換器を使うのが一般的 しかし、あまちじょんこ(Youtuber @johnko_amachi X: @johnko20100711) などは自らの超絶技巧で声を変える。 中の人は、じょんアニキ。 めざすもの:天真爛漫で無邪気で、こういう小さい子っているよねという女の子をしたい。 高い声にするだけだと、男の人の高い声になってしまう。声は楽器と一緒なので楽器の形を変えてあげる。そうやって音質を変えて、その上で音の高さを変える。両方が合わさってじょんこの声になる 2年かけ、理想の可愛い声を獲得した 「別の自分になれるってめちゃくちゃ楽しくて。で、なんかそれで、ただ楽しいということを突き詰めていく。天才じゃないから頑張るしかない。」 カワイイ動きのトレーニング。いざやっていむと難しい。ちいさい子の気持ちになって動かないといけない。たとえば、歩くときに右の足を出すと右に重心がよってしまう。これを繰り返すとふらふら見える。このふらふらした動きが、見ている人からしたら守ってあげたいというので可愛く見える。 ミラさん 歩く時にかわいいを意識したことがなかったのでとてもおもしろいと思った 前は日本の男性といえばサラリーマンかオタクという表層的なステレオタイプなイメージしかなかった。しかし、研究をしてみると、日本人男性の多様性や日本文化の寛容性を知りとても面白いということがわかってきた ミラさんの生い立ちの説明 ロシア生れ。12歳でスイスに移住。フランス語の勉強に日本の漫画。「天使禁猟区」5。今までこのような美しい絵を見たことがなかったので新しい世界が開けた。 27際のときに日本文化を学ぶためにジュネーブ大学の修士課程に。 論文を発表「Babiniku」6 日本の伝統芸能の一つである歌舞伎の女形と同様に男性が美少女キャラクターを演じる類似点 人形浄瑠璃とアバターをあやつるバ美肉の共通性など日本の伝統文化を通してバ美肉を考察した論文は大学から学術賞を受けた 日本の文化はとても興味深く、趣味と実益を兼ねた研究をしたいと考えた ブレンダ・マーフィー教授 「彼女の研究の魅力は西洋で主流ではない”日本文化”に着目している点です」 バーチャル人類学はとても新しい分野であり、彼女はグローバルな文化への挑戦者といえる リュドミラが来日するのはこれで6度目。街に行けば目につくのは美少女キャラクター。看板や飲食店など町のいたるところで見かける。美少女キャラクターは秋葉原だけでなく日本文化で不可欠な存在 アニメや漫画の専門店に立ち寄り、次々に新しいものが生まれる日本のサブカルチャーもミラさんにとって研究の一環 第4章 美少女キャラに会いたい アイドルVTuber のらきゃっと (@NoraCat0415 ch. 登録者86000人以上)。ファンネーム「ねずみさん」 これを生み出したのが「のらねこP」(@PIMR, X: @VR_Produce_Nora)インタビュー のらきゃっとにガチ恋だと言っているファンとの関係についておしえて のらちゃんがちょっとドジなところもあってでも強くて可愛くてというのはファンがそう思っているから作られたという部分が多くて、ネズミさんたちがのらきゃっとという存在にそうあってほしいという願いのもとに作られたのでファンからの影響はとても強い のらきゃっとがファンから可愛いと言われるとどう感じるか 娘が公園で仲の良い友達と遊んでいて幸せそうだと親が感じるのに似ている のらきゃっとが生まれて人生は変わったか? 今までの仕事に行って帰ってきて家で寝るだけという生活でそんなに友達もいないというような人生から、本当に楽しく話せる友達が10倍以上に増えた。誰かと話したいときに話せるので寂しいと思う瞬間がなくなった かわいい美少女になれるって最高ですね「みんななれる〜」 ファンから求められることで美少女キャラはよりかわいくくなり、その美少女を生み出した彼の生活も豊かにしている。今日いちばん重要な発見だった 第5章 美少女になる理由を知りたい バーチャル美少女とファンとの交流はさらに進化。現実世界のファンが集まる店。友人のバ美肉が出演すると聞き訪ねた。「新宿ねむバー」 バーチャル美少女ねむさん(Youtube @nemchan_nel, X @nemchan_nel )=『メタバース進化論』(技術評論社)の著者で、ミラさんの研究の頼もしい協力者 ミラさん:「直接あって美少女やメタバースとか私達が情熱を注いでいることについてもっと話そう」 ねむさん:「楽しそう。たくさん話すのを楽しみにしてるよ」 ミラさん:「ではリアル世界で」 ねむはプライベート時間の殆どをメタバースで過ごし、専門書7を出版するほどこの世界について考え続けてきた人物。 ミラさん:直接美少女キャラクターになる理由を訊いてみたい 匿名で活動したいというねむさんの希望により映像と音声を加工しています→モザイクの大きさはかなり調整したらしい SNSを通じ知り合ったのは4年前。ねむさんとはメタバースユーザの生活実態を一緒に調査したことも。 ミラさんによるねむさんへのインタビュー 初めてねむになったときどう思ったの? すごく恥ずかしかった。 罪悪感〜違う自分になるというのが悪いことをしているような感覚だった でもだんだんいつもの自分では出てこないような言葉が口から出てきた 美少女になると自己表現も変わるということか? 「現実の自分だとカッコつけちゃうのかな」 「そこまで心揺れ動かない結構冷静な人間だと自分のことを思っていたけど、ねむちゃんのときはそういうのから開放されてもっと自由に表現できる」 あなたにとっての美少女ってどういう存在なのか? “カワイイ”という概念を具現化した存在が美少女キャラクターだと思っている 肩書とか現実世界のしがらみを剥ぎ取ると人間の本質ってすごくカワイイ。魂って実はカワイイと思っていて、カワイイはその人の本質を褒めている気がする。 自信になるというか、ありのままでちゃんと意味があるんだと思える気がする なぜ日本人男性が美少女になりたいと思うのか。その答えを求めてフィールドワークを続けてきたミラさんの今考える結論 日本人男性は美少女になりカワイイを獲得することでストレスから開放される。 なぜならカワイイは失敗しても許されるし責任を負わなくていいからです。 私は日本文化から生まれたカワイイに可能性を感じました しかし私は人類学者としてコミュニティを一般化するのではなく、これからも多くの人たちの声に耳を傾けたいと思っていますエンド:若き人類学者が日本のバーチャル世界に分け入っていく
考察 アイデンティティとバ美肉アイデンティティという言葉は番組の中に出て来ませんが、多くの発言は「アイデンティティ」関連だとわたしの中では整理されました。たとえば、じょんこさんの「別の自分になれるってめちゃくちゃ楽しくて。で、なんかそれで、ただ楽しいということを突き詰めていく。」という発言は、現実社会の仕事だとか交友関係という既存のコンテキストを超えた新たなコンテキストでの自我の確立とその表出(自観するアイデンティティの表出)およびそれがコミュニティ内で他の人に観測されるもののフィードバック(他観)という関係性の享受から幸福感を得ているように見えます。(自観と他観のあたりは、「非技術者のためのデジタルアイデンティティ入門」をご参照ください)
類似のことは、ねむさんの「(最初ねむになったとき)すごく恥ずかしかった。罪悪感〜違う自分になるというのが悪いことをしているような感覚だった。でもだんだんいつもの自分では出てこないような言葉が口から出てきた。」「ねむちゃんのときはそういうのから開放されてもっと自由に表現できる。」という言葉にも出てきます。
一方、のらねこPの「のらちゃんがちょっとドジなところもあってでも強くて可愛くてというのはファンがそう思っているから作られたという部分が多くて、ネズミさんたちがのらきゃっとという存在にそうあってほしいという願いのもとに作られたのでファンからの影響はとても強い。」という発言は、自観と他観のズレの最小化を、表出する属性の調整によって他観の方を変えて行うという「通常」のやり方とは逆で、他観の方に自観をあわせてしまうというアプローチに見えます。根源的自我に他観を合わせに行くのではなく、自我の方を調整してしまうわけですね。ただ、これが本当に自分の自我だとかなり心理的負荷が大きくなってくるのではないかと思われます。心理的負荷を減らすには、自分の中からその人格を外だししてしまって客観化してしまうことが必要であるようにも思えます。
このあたりが、のらねこPの「娘が公園で仲の良い友達と遊んでいて幸せそうだと親が感じるのに似ている」という発言からも表出されているように感じられました。
ここの部分で、ミラさんは「ファンから求められることで美少女キャラはよりかわいくくなり、その美少女を生み出した彼の生活も豊かにしている。今日いちばん重要な発見だった」と述べています。
免罪符としては機能しない美少女キャラクターとプライバシー次に検討してみたいのが、ミラさんの「日本人男性は美少女になりカワイイを獲得することでストレスから開放される。なぜならカワイイは失敗しても許されるし責任を負わなくていいから。」という発言です。わたしの解釈違いかもしれませんが、カワイイが免罪符的に機能するので、日本人男性はカワイイを獲得したいと言っているように聞こえます。「日本人男性は」としているので、この「カワイイ」の概念とその通用する範囲が日本にまず限定されているのだろうということは想像できます。そのうえで「カワイイ」が免罪符的に機能するのかと言うと、これはちょっと難しいところだと思っています。
「カワイイ」を体現するものとしては、いわゆるアイドルVTuberなどがいると思います。ところが、その免罪符を持っているはずの彼女たちは、時として誹謗中傷されたりコミュニティから叩かれ、「卒業」を余儀なくされたりしているのは、このあたりをちょっと追っている人ならよくご存知のことと思います。もし「カワイイ」が免罪符として機能するならばこういうことは起きないと思うのです。
おそらく理想としての「カワイイ」には人々の期待がつまっていて、その期待を裏切ると叩かれるという構図があるのではないかとも思えます。つまり「カワイイ」が免罪符として機能するわけではない。ある意味、「”カワイイ”という概念を具現化した存在としての美少女キャラクター」は現代の巫女であり、古代と同じく、期待を裏切ったときにはスケープゴートにされる存在だと言えるかもしれません。で、わたしはスケープゴートって作ってはいけないと思うんですよね。
現代の巫女がスケープゴート化してしまう原因の多くは、コンテキスト外の情報の当該コンテキストへの混入です。たとえば、誰かからのチャットが画面に映り込んでしまったとか、他の人の声が混入してしまったとか、自分の顔が反射で映り込んでしまったとかですね。今後は他のワールドでの行動が当該ワールドにレポーティングされてしまうというような形の情報混入も起きてくることでしょう。場合によっては悪意の人が監視用のキャラクターを各所に配置して意図的にこれをやってくることも考えられます。中の人と、その人が運用する異なるアバターの名寄せとかもこの中に入ってきます。
番組の中でねむさんは「匿名で活動したい」としてモザイクをかけて声も変えて登場していますが、これも望まない名寄せであるとか情報の混入を防ぐ取り組みの一環と整理することもできます。
こうしたことをどうやって防ぐかとか取り締まっていくかなどが、メタバースにおけるプライバシーの尊重という意味では重要性を増してくると思われます。メタバースプライバシーの国際標準作成作業が始まっている背景でもあります。
しがらみを削ぎ落とした存在としての「ありのままの自分=美少女キャラクター」さて、ではなぜ日本人男性はバ美肉化するのか?上記のことを勘案すると、ミラさんのいう「日本人男性は美少女になりカワイイを獲得することでストレスから開放される。なぜならカワイイは失敗しても許されるし責任を負わなくていいから。」というのはちょっと違いそうです。
番組内でねむさんは「”カワイイ”という概念を具現化した存在が美少女キャラクターだと思っている。肩書とか現実世界のしがらみを剥ぎ取ると人間の本質ってすごくカワイイ。魂って実はカワイイと思っていて、カワイイはその人の本質を褒めている気がする。自信になるというか、ありのままでちゃんと意味があるんだと思える気がする。」とおっしゃっておられます。現実世界のしがらみを剥ぎ取った人の本質は「カワイイ」であり、そのありのままの自分の自我「自観」を表出すると、日本文化においては美少女になるということでしょうか。そうかもしれません。なにせ、8割近くのひとがそうあろうとするのですから。
番組では、各被取材者ごとに数時間のインタビューがあったのがそれぞれ5分くらいにまとめられてしまっているようです。削ぎ落とされたものの中に、このあたりに光を当ててくれる発言も多くあったことでしょう。その意味で、アフタートークも準備されているようなので、それに期待したいと思います。
【ガチ勢各位】
— バーチャル美少女ねむ/Nem4/13 NHK「最深日本研究」出演! (@nemchan_nel) April 14, 2024
実際にはみんな2 ~3時間喋った内容をそれぞれ一般人向けに5分くらいにまとめられてるので、ガチ勢のみんなとしたはぶっちゃけ物足りない面もあると思う。という訳で、アフタートーク企画中です!!! #最深日本研究 https://t.co/db9ToxqQpl
(追記)アフタートークの日時が決まったようです。4/20(土)22:00〜。わたしはサンフランシスコからの飛行機の時間と微妙なのですが…。アーカイブは公開されるのだろうか…。
【緊急ライブ告知】4/20 (土) 22:00~
— バーチャル美少女ねむ/Nem4/13 NHK「最深日本研究」出演! (@nemchan_nel) April 15, 2024
ゴメン全然喋り足りない!!! #最深日本研究 出演者4名が、番組には収まりきらなかった”バ美肉”や活動への想いなど、時間無制限で喋り倒します!
NHK「最深日本研究」非公式アフタートーク【ねむ✕ミラ✕のらねこP✕じょんこ】https://t.co/2zG4EbuVGO pic.twitter.com/0G2R0zHGEo
<変更履歴>
ミラさんのことを博士と書いていましたが、まだ博士課程在学中のようですので「ミラさん」の表記に変えました。 アフタートークの日時を追加しました。 サムネがFacebookだとはねられてしまうので差し替えました。 「ブレディキナ」を「プレディキナ」と表記してしまっていたのを修正I decided to give Tesla FSD a second chance today. Aside from the bit where it decided to turn onto an actual municipal train track and use it as a road, it did really well!
The WebOfTrust community recently released a major update to the Key Event Receipt Infrastructure (KERI) and Authentic Chained Data Containers (ACDC) implementation as a coordinated release across the suite of WebOfTrust repositories.
Coordinated ReleaseThis resulted in the following release versions:
Repository: KERIpy (used for witnesses, command line-managed decentralized identities, and as the core library for the Python KERY space)Recent Blog Posts
See Nuttawut Kongsuwan’s explanation of how to use KERI in his “The Hitchhiker’s Guide to KERI. Part 3: How do you use KERI?“
The thing about most war commentary on social media is that it's speculative, almost on an entertainment level. Every time, there are people who bear the cost of this, who didn't ask for it, who don't endorse it, and yet will still pay an unimaginable price. It's described as points-scoring but it's death and suffering, children and families and innocent human beings, and their descendants, and theirs, and so on, for generations. There is no glory, there is no validity. It's sick.
Now for something entirely different. I write only occasionally on a topic of interest to me, verifiable credentials. Today it is something entirely different, my career. Most specifically, on how it may be time for a refocus. A movement from software development life-cycle process back toward a new more technical focus, AI.
I have, in some ways, refocused my career a couple of times. It wasn’t a full restart or anything; just moving into new directions within software development. Some were more a natural progressions than a refocus. This time it would certainly be a refocus.
What I do and what I have done?For the last several years I have been a software technical release manager focused on not only getting software out the door but on process improvement. That is not where I started my career. It all started as a system admin, then a programmer, then getting involved in migrations and software develpment for mitigating Y2K, on to a software team lead. Then came the first refocus, I got deeply invested in a new Microsoft product Team Foundation Server. This took me down a fun rabbit hole where I spent some years consulting on implementations and use of the product. I spent a few years of my consulting as a Micrsoft MVP on the product, which was very rewarding. Ultimately, with a focus on version control, automated builds and deploys, and release processes with the product and in general, I made the next refocus. I moved into release management. Decidely less technical, but something I have now enjoyed for a number of years. I find the cross-functional nature rewarding and plenty of opportunity to still practice process improvement.
Why the consideration of a refocus?I see it as a dying domain. I have found that the role is late to join organizations and more easily released from those same organizations. In my experience, companies find unexpected value in the role and can really enjoy having the “glue” bring higher quality and improved flow to production and beyond, but “enjoy” is too much of an operative word here. When belts are tightened it is one of the first roles to go — seen as a luxury not a requirement. I also see diminishing new opportunities in the marketplace. All this places downward pressure on compensation. Additionally, upward progression from the role is often difficult. So it may be time for a full refocus, again.
Too technical for the role?It wasn’t too long ago I was told as a final feedback for a failed pursuit of an open release management position:
You are too technical.
Hmmm… I knew what they meant, but I had never had my quals work against me- not that I know of. Most find it to be a boon. It made me think. With all the considerations of why there might need to be a refocus, is it time to shift my momentum?
Where to next?I really enjoy what I do and would hate to give it up but the writing might be on the wall. So, as I survey the landscape, I say to myself “what might be next”. Where is the best opportunity to delve into new things and make an impact for organizations? Is it the AI domain? Can I go from zero to hero? What does that look like? How long would it take and what path is there? I see a lot of potential in Microsoft’s Azure AI offerings and the ones that will inevtiably come down the line. Plus, I have a long history in the Microsoft stack. Let’s see where the next few years take me. Regardless of anything, I’ll certainly be digging deeper into the practical applications and implementations of the technology all why continuing my enjoyable current role in software life cycle.
Back to a common topicNow, if I can come up with an AI realm play that ties in verifiable, portable, credentials. What will be bigger in the future than identity and AI? Nothing.
I gave a presentation on Fully-Specified Algorithms for JOSE and COSE at the 2024 OAuth Security Workshop in Rome. The slides used to update participants on the progress of the work are available as PowerPoint and PDF.
Thanks to the organizers for another great OAuth Security Workshop! And special thanks to the colleagues from Fondazione Bruno Kessler who did a great job with local arrangements in Rome!
I’m pleased to report that the COSE “typ” (type) Header Parameter Specification has been approved by the IESG and is now in the RFC Editor queue.
The version approved by the IESG and sent to the RFC Editor is:
https://www.ietf.org/archive/id/draft-ietf-cose-typ-header-parameter-05.htmlIt joins CBOR Web Token (CWT) Claims in COSE Headers in the RFC Editor queue. Because of the reference to this spec by CWT Claims in Headers, they form a cluster, and therefore will become RFCs at the same time.
Gestern Abend haben die Abgeordneten des EU-Parlaments mehrheitlich für die Verlängerung der umstrittenen flächendeckenden freiwilligen Chatkontrolle 1.0 durch US-Internetkonzerne wie Meta (Instagram, Facebook), Google (GMail) und Microsoft (X-Box) bis April 2026 gestimmt. Von den deutschen EU-Abgeordneten unterstützten Union und SPD die Verlängerung, während Piraten, Grüne, FDP, Linke sie ablehnten und die AfD sich enthielt.
Der Europaabgeordnete der Piratenpartei und digitale Freiheitskämpfer Dr. Patrick Breyer, der gegen die eigenmächtige Chatkontrolle vor Gericht klagt, kritisiert:
„Das EU-Parlament will von den grundrechtswidrigen flächendeckenden Chatkontrollen weg, mit dem gestrigen Beschluss zementiert es sie aber für Jahre. Das EU-Parlament will einen viel besseren und gerichtsfesten Schutz vor Kindesmissbrauch im Netz, mit dem gestrigen Beschluss wird aber überhaupt nichts zum besseren Schutz unserer Kinder erreicht. Mit so wenig Rückgrat werden immer weitere Verlängerungen des Status Quo der Massenüberwachung folgen und ein besserer Schutz von Kindern immer unwahrscheinlicher. Missbrauchsopfer haben besseres verdient!
EU-Kommission, EU-Regierungen und einem internationalen überwachungsbehördlich-industriellen Netzwerk ist es leider gelungen, der Parlamentsmehrheit Angst vor einer vermeintlichen ‚Schutzlücke‘ durch Wegfall der flächendeckenden freiwilligen Chatkontrolle 1.0 zu machen. In Wahrheit leistet die freiwillige Massenüberwachung unserer persönlichen Nachrichten und Fotos durch US-Dienste wie Meta, Google oder Microsoft keinen signifikanten Beitrag zur Rettung missbrauchter Kinder oder Überführung von Missbrauchtätern, sondern kriminalisiert umgekehrt tausende Minderjähriger, überlastet Strafverfolger und öffnet einer willkürlichen Privatjustiz der Internetkonzerne Tür und Tor. Wenn nach eigenen Angaben der EU-Kommission nur jede vierte Meldung überhaupt für die Polizei relevant ist, bedeutet das für Deutschland Jahr für Jahr 75.000 ausgeleitete intime Strandfotos und Nacktbilder, die bei unbekannten Moderatoren im Ausland nicht sicher sind und in deren Händen nichts zu suchen haben.
Die Verordnung zur freiwilligen Chatkontrolle ist sowohl unnötig als auch grundrechtswidrig: Die sozialen Netzwerke als Hostingdienste brauchen zur Überprüfung öffentlicher Posts keine Verordnung. Dasselbe gilt für Verdachtsmeldungen durch Nutzer. Und die fehleranfälligen automatisierten Meldungen aus der Durchleuchtung privater Kommunikation durch Zuckerbergs Meta-Konzern, die 80% der Chatmeldungen ausmachen, werden durch die angekündigte Einführung von Ende-zu-Ende-Verschlüsselung ohnehin im Laufe des Jahres entfallen.
Als Pirat arbeite ich daran, die eigenmächtige Chatkontrolle als verdachtslose und flächendeckende Überwachungsmaßnahme vor Gericht stoppen zu lassen. Gleichzeitig werden wir Piraten im EU-Parlament gegen alle Versuche kämpfen, doch noch Mehrheiten im EU-Rat für die extreme Dystopie verpflichtender Chatkontrolle 2.0 zur Zerstörung des digitalen Briefgeheimnisses und sicherer Verschlüsselung zu finden.“
Breyer kündigte gestern an, gegen die erstinstanzliche, formal begründete Abweisung seiner Klage gegen die Chatkontrolle in Berufung zu gehen. Unterdessen wollen die EU-Regierungen bis Juni darüber entscheiden, die Chatkontrolle für alle Dienste verpflichtend zu machen, selbst für bisher sicher Ende-zu-Ende-verschlüsselte Messengerdienste.
Services that require identity verification have become increasingly dependent on “scan the front, scan the back, take a selfie, match” for everything from renting a sidewalk scooter to opening bank accounts to provisioning your mobile driver’s license. The cost of inaccuracy in each situation varies greatly. The practical amount of money to spend within these use cases doesn’t — from 0 to 25 cents. Yet most services cost $1. Startups eat the difference hoping the future cost will go down (sorry, it won’t). Many businesses eliminate steps to keep costs down but still roll out a high-demand feature. Avoid offering mobile services that depend on ID and you fall behind. Do it, and accept the risk of fraudulent inputs.
There are both financial risk mitigation use cases and government identity use cases that are currently utilizing mKYC. I’d like to separate these concepts (read to the end to see why).
Note: I do not use the overloaded term document verification. I specifically split document verification into two distinct steps: document authentication (is it from a trusted issuer) and document validation (is the data on it accurate and the account behind it still valid). (See also definitions NIST 800–63). Both of these would be resolved with digital government-signed identity documents.Cost Analysis of mKYC
Note: I invite those with more insider knowledge of the costs of IDV to connect with me to correct my educated summary of costs below.
There are at least 10 mKYC issues that negatively impact user experience, accuracy, and privacy. mKYC is a technology where we haven’t yet been able to achieve Privacy by Design Principle #4 of full functionality without zero-sum tradeoffs. It’s still cost vs. accuracy vs. user experience vs. privacy (spoiler alert: cost wins). Doing analog-to-digital, this won’t ever change.
The Document Authentication Cost ProblemIs the physical document real or fake? How much does it cost to accurately know?
The cost problem here is simply explained. In physical documents, the goal of winning the card security feature race against fraudsters was to make it expensive, in dollars and knowledge, to produce fake IDs. So cards have proprietary visual, tool-based (8x magnify, multi-spectral lighting), and forensic (destroy the card to detect) security features built into their structure, printing, and lamination. Digitally created for analog use... At great expense, including admirable IP, layered over time… but the fraudsters still caught up, able to replicate lower-cost visual features (and therefore make them acceptable by every bar bouncer on earth).
This gave rise to the supremacy of tool-based authentication for high-risk use cases — use an 8x loupe magnifier; combine white, UV, and IR lights (multi-spectral) to detect secrets built or printed into the card; purposeful design flaws, raised surfaces… amazing technology! Until you try to scan and authenticate it with a white-light phone camera held by a human hand.
Now We Expect to Go Back from Analog to Digital?Try to ingest a secure physical card back into the digital realm and you must try to detect all three levels of physical card security features with a hand-held camera designed for action photos and portraits taken at focal lengths much further away than human arm length. There’s no UV or IR light, no optical magnification, very few steady cams, and no foolproof UX coaching for a person who isn’t aware of what’s being detected.
Do you see where this is taking us? Massive cost, diminishing return, user friction, bad experience… dropoff. What do mKYC purchasers do? Turn features off to avoid paying for document authentication and user friction.
The Document Validation Cost ProblemOnce you’ve scanned an ID document, extracted the data from the front with OCR, decoded the barcode from the back, and skipped sending it for document authentication (lol), you’re still left with a few questions…
Is the document still valid? Is the identity data accurate? Are the attributes fresh or have they changed? Can I ask the authoritative source to validate them? How much does it cost me to perform that document data validation?
If you want to establish the provenance, accuracy, and freshness of the data, you need to connect to the source that mitigates your risk level.
At the first level of reliability are “credible sources”. For example, data aggregators charge dimes and quarters for the service of validating data you collected against what they algorithmically believe to be accurate. It’s due diligence on your part. Whether you consider that credible depends on your level of risk versus the cost of service.
At the higher level of reliability are “authoritative sources” — the official issuers of identity documents. Who can connect? Mostly government and law enforcement. You can pay a quarter or two per record to validate data in privacy-preserving manners — yes/no on the accuracy of what you have versus looking up someone’s identity data using an identifier.
See the problem? It costs more just to validate than businesses should logically spend on the whole identity problem. And most don’t have access.
Then there’s the “use at your own risk” level of reliability of unofficial sources (not NIST-defined, surprisingly). The problem with using unofficial sources is that all our data was breached. Assume that to be true — zero trust. Check your mailbox for legal breach notices — confirmed. It’s cheaper to buy that data from the dark web than to use either a credible or authoritative source (if available to you). Advantage fraudsters… and the reason fraudsters can pass Knowledge-Based Authentication more easily than our memories. Skip KBA as an alternative (please).
What’s the point? Eh, given the cost, you’ll skip the document validation step. And you skipped document authentication. So you’re left with having snapped an off-angle photo of that ID document so that you can crop the low-resolution face out of it (resplendent with holograms, security lines, watermarking, and reflective lamination) for biometric matching. Better set that False Accept Rate high enough to get a decent Match Score!
Or you can just produce digital documents in the first place. (See #3 below)Cost Consolidation
From what I see, there are some alternatives for us to start to win-win the zero-sum problem we are facing.
1. Do this Once at High Cost/Accuracy and Reuse it at Lower CostIf one analog-to-digital event can be run at a high level of identity assurance(IAL2 minimum) by an authoritative entity, and the event can be re-used, then we start to lower the repeated costs to all future entities. Perhaps then the cost-benefit ratio will make sense. Note that I said an “authoritative” entity and not a credible or trusted entity. I like banks. Banks act in trustworthy ways, perform due diligence when required, and have been willing to step to the forefront in many regions. But if you require a “government-issued ID” for your use case, bank KYC isn’t that. Bank KYC is an Identity Verification not a government signed ID.
A reusable identity solution should be a government-issued, privacy-protecting credential with user authentication and selective disclosure. You might look at mDL as this, however, there’s no standardized mechanism for user authentication yet in ISO/IEC 18013–5 or -7. Even without built-in user authentication, ingesting a digital mDL will short-circuit dozens of my list of 10 problems (pun intended), increase accuracy, and lower cost while allowing fewer fraudulent documents through. That’s why NIST wants to solve this first through the NCCoE.
2. Separate Financial Risk Mitigation from Official IdentityWhy this hasn’t happened yet confuses me. Yet we still call it “identity fraud” when criminals are using freely available identity data to perform “financial fraud”.
But seriously, scooter rentals don’t need my ID document, they need to know I can or will pay. Keep going and we will realize the ACLU’s dystopian over-identification state (link will download PDF). None of us want that.
The solution Financial Services needs to look at is reusable user authentication against the KYC they or their partners already performed… without sharing identity attributes that won’t mitigate financial risk.
3. Issue Digitally-Signed OriginalsBetter yet, just skip the analog to digital steps. Issue and accept digital birth certificates. Issue and accept Mobile or digital ID documents. If you’re not yet accepting digital mDLs, start.
People in the (self-labeled) Self-Sovereign or Decentralized Identity spaces have created the nearly ideal architecture for issuing authoritatively signed, lightweight, digital birth certificates. So far, to my knowledge, only Singapore has jumped on this technology and is realizing the cost savings and accuracy. There’s still one privacy hole to close… whoever controls the distributed ledger can potentially see all authentication and validation requests against the ledger if they control the API layer. This is a solvable technical problem with the architectural concepts of blinding, brokerage, or anonymizing API access (rotating relying party identifiers). This is solvable with policy (link will open video) if not law.
4. Put the User/Holder in Control of their Credentials and DataWhen you provide the user (aka citizen, resident, digital subject, holder) with their document in digital form, they gain control over how they share it. EU Digital Identity Wallets are making progress. More importantly, the ease of sharing means that the recipient (relying party) doesn’t need to store identity attributes to mitigate their risk. Ask, verify, dispose. This is a concept of Interactive Information Privacy(TM) that I’ll write about more in the future and that I presented at Cloud Identity Summit in 2017.
Don’t forget to put your reusable identity-proofing credential in that wallet.Call to Action
We want to stop “ripping IDs” just like we stopped ripping CDs when digital content services became ubiquitous. Digital Identity Services are the next frontier, but everybody is trying to solve everything everywhere all at once. I laid out 4 pathways above, each with existing possible solutions and a legitimate business model to make them self-sustaining. Let’s create actionable, cost-effective solutions to our “identity fraud” problems. The architects have done decent jobs… now the product, market, and business sides should capitalize in better ways than we are currently employing.
Terrific presentation and discussion at last week’s Postgres Extension Ecosystem Mini-Summit. In fact, I later learned that some people couldn’t make it because the Eventbrite event page showed it as sold out!
This is a limitation of the free level at Eventbrite, which caps tickets at
25. But this is a fully-remote event and we can host more people than that. We
had over 30 last week! So if you’d like to attend one of the remaining three
events and Eventbrite shows it as sold out, have a look at the bookmarks for
the #extensions channel on the Postgres Slack, or email the username
david
at this domain and I will send you the link!
Okay, back to business. Last week, Devrim Gündüz kindly gave a super thorough presentation in his capacity as the maintainer of the Postgres community Yum and ZYpp repositories. This topic sparked lots of interesting discussion and commentary, which I detail below. But why read when you can look?
Video PDF SlidesStill reading? Okay then, here we go!
Introduction I opened the meeting and introduced Devrim Gündüz. PresentationThank you for the introduction. Going to talk about Yum and ZYpp dot postgresql.org, these are the challenge for us, and the challenge of making RPMs from extensions.
Work for EDB as Postgres expert, Postgres Major contributor responsible for the Postgres Yum repo. If you’re using RPMs, I’m building them. I also contribute to Rocky, SUSE, and Fedora Linux, proud Postgres community member, live in London, and I’m also doing some DJing, so if I get fired I have an extra job to do.
Agenda: Last year at PGConf.eu in Prague, I had 60 slides for 5 minutes, so expect huge number of slides for today. I want to leave some time for discussion discussion and questions.
I’m going to start with how it started back in the 2000s, talk about the contents of the repos, which distributions we t because that’s another challenge um how do we do the extension packaging how to RPM how to build RPMs of an extension and how to update an extension RPM.
Then the horror story um when what happens when there’s a new Postgres beta is out, which is due in the next next month or so for Postgres 17.
Then we are going to speak about the extension maintainers, problem for us, and then the problems about relying on the external repos.
So if you have any questions please ask as soon as you can. I may not be able to follow the chat during the presentation, but I’ll try as much as I can.
So let’s talk about how it started. When I started using Linux in 1996 um and Postgres in 1998, we always had Tom Lane and we had we had Lamar for for who build built RPMs for RedHta Upstream. So they were just building the distribution packages, nothing community. It was only specific to RedHat — not RedHat Enterprise Linux but RedHat 3-4-5 — not the non-enterprise version of RedHat back then, but it was called it Fedora core back then, the first version was released in November 2003, which was another challenge for packaging
One of the problems with the distribution packaging was that it was slightly behind the minor Postgres releases, sometimes major post releases
So that was one single Postgres version available for a given distro, say Postgres 6.4 or something, 7.0, and multiple versions were not available back then, and the minor version was slightly behind.
I started building packages for my laptop because I wanted to use Postgres and not all versions wer available back then. So I started building packages for my laptop and my server. They were based on the packaging of Tom Lane and Lamar.
Then I uploaded them to my personal server and emailed the PG mailing lists lists and said, “I’m running on own packages, use at your own risk. This is just a rebuild of the upstream packages on the RedHat version that I’m using or the Fedora version that I’m using. Up to you! This is a personal project, nothing serious.”
So then then people started downloading them, and using them. There was no signature, nothing official back then. Then Lamar said he didn’t have enough time for the RPMs. He sent an email to the mailing lists and I said Devrim is stepping up to the plate, and I did it. So that was I think in 2004, about which is about 20 years ago.
So 19 years ago we had the first domain, postgresql.rpm.org
, and then we
had more packages. In 2007 we had the first repository RPM and then we had
yum.postgresql.org. This means that, starting 2007, this began to be the
official RPM repo of the community, which which was a good thing because
we could control everything under the Community.
I was living in Canada back then. We had the first RPM build farm — instead of using my laptop and my VMs — we had the actual machine back then.
In 2010 we had what was then called multiple postmaster support, which means the parallel installation of the Postgres RPMs. That was a that was a revolution because even still, the current Fedora or RedHat RPMs cannot be installed in parallel. So if you want to install post 13, 14, 15, 16, and 17 or whatever, it is available in the community repo. This is a great feature because you may want to test or do something else. This is how we how we started, 14 years ago we had this feature in the community repo.
Then next year we moved the repo to the community servers and unified the spec files. Our builds are extremely simple — like you can start your own builds in less than 10 minutes: five minutes for pulling the git repo and then a few minutes for for a package. Extremely simple builds, and now we are in 2024
Let’s talk about the contents, because we have four different contents for the repo. The first one is the “common” repo. We call it “common” in the repository config file. It has the RPMs that work across all Postgres major releases. Let’s say pg_badger, or the ODBC driver, JDBC driver, or GDAL for PostGIS. There’s lots of stuff: Python packages, which are not extensions but we like Patroni, which actually works for all Postgres releases.
This was an effort to get rid of duplicates in the Postgres repo. I think we shaved lots of gigabytes back then, and we still save a lot
Then, to address one of the topics of today’s talks, we have the
“non-common” RPMs. (These are the name of the directories, by the way.)
They are the RPMs which are Postgres version-specific, basically they are
extensions. Say plpgsql_check
15 or whatever. Lots of extensions are
there.
Then we have extras. They are not actually Postgres packages, they shouldn’t be included in our repo by default, but many people actually look for these packages because they they want to use Patroni and they don’t have the supporting RPMs or supporting RTM RPMs, or they’re not up-to-date.
I’m building a console, console-template, ETCD, HAProxy keepalived and vip-manager. They are all open source, some of them are Go packages — which, actually, I don’t build them, I just distribute the precompiled binaries via the repo. So that makes easier for people to deploy the packages.
And then we have the “non-free” repo. These are the packages that depend on closed-source software like Oracle libraries, or that have license restrictions. For example, ora2pg depends on Perl DBD::Oracle, oracle_fdw depends on Oracle, pg_storm depends on Cuda Nvidia stuff, timescaledb-tsl actually is Timescale DB with the TSL license, informix_fdw and db2_ftw.
So we have some non-free packages which actually depend on non-free stuff as well. All of them are well-maintained: I’m trying to keep everything up to date — like real up-to-date! That brings some problems but we will get there.
We support RedHat Enterprise Linux and of course Rocky Linux. This year we started supporting Alma Linux as well. Of course they are more or less identical, but we test them, install, and support to verify the packages in these three distributions.
We have x86_64 aarchm64, ppc64le, and RedHat 9, 8, and 7. We have also RedHat 6 for Postgres 12, but it’s going to be killed by the end of this year. We have Fedora, but only two major releases, which matches the Fedora lifecycle. And SUSE — my pain in the neck — um I’ll get there.
Since you all are here for extension packaging, let’s get there: what happens for extension packaging.
First of all, we have the first extension, which is the in-core
extensions. They are delivered with the contrib sub-package, which matches
the directory name in The Postgres tarball. There are separate packages
for each major version, so postgres15-contrib
, postgres13-contrib
,
etc. These are the installation directories for each extension. We are
going to use those directories for the other [non-cre] extensions as well.
When we add a new extension to the repo, it’s going to use these directories if they have a binary or if they an extension config file, if the library or the mem files — all are going are all installed under these directories. This magic is done by PGXS, which has been there forever. We just provide initial stuff and then the rest is done by the the PGXS magic. This is the base for a lot of the core extensions.
So what happens when we do non-common and non-free package? First of all,
they are distributed separately for each Postgres major version. Let’s go
back to the one of the extensions, say plpgsql_check
. We have a separate
package for Postgres 14, a separate package for Postgres 15, 16, 13, and
12. If they build against all the supported Postgres versions, we have
separate packages for each.
Of course from time to time — as far as I remember Timescale DB does this —- Timescale DB only supports Postgres 15 and 16 nowadays. So we drop support for the older versions in the Git repo. But they are separate packages; they are all installed in these directories along with the main contrib package.
This is the naming convention that I use: $extensionName_PGMajorVersion
.
Four or six years ago, some of the packages didn’t have an underscore
before the PG major version. It was a problem, so someone complained in
the hackers mailing list, and then I made the changes.
Currently all the previous and all the new packages have this naming convention except a few packages like PostGIS — because in PostGIS we have multiple versions of the same extension. let’s say we have PostGIS 3.3, 3.2, 3.4, 3.1, 3.0. We have combinations of each — I think we have PostGIS 3.0 in some of the distributions but mostly PostGIS 3.1, 3.2, 3.3, and 3.4, and then all the Postgres versions they support — A lot of builds! So there are some exceptions where we have the extension name and extension major version before the Postgres major version.
Jeremy S in chat: question: curious if you have thoughts about relocatable rpm packages. ever tried? just best to avoid?I have a question from Jeremy. This is a very basic question to answer. This is actually forbidden by the packaging rules. The RPM packaging rules forbid you to distribute or create relocatable RPM packages. We we stick to the packaging guidelines, so this this cannot be done.
Jeremy S in chat: Thanks! (Didn’t realize packaging guidelines forbid this)Let’s talk about how we build extensions. Often our develop package is enough: many of our packages just just rely on on Postgres itself. But of course packages like PostGIS may depend on some other packages, like GDAL, which requires lots of lots of extra dependencies as well. The most problematic one is the GIS Stack: they need EPEL on RHEL and RHEL and its derivatives.
There there has been a discussion in the past about whether should require EPEL by default. The answer is “no,” because not all not all of our users are installing, for example, the GIS stack or other packages. Most of our users — not the majority of our users —- rely on the um rely on just our repo.
On the other hand, in order to provide completeness for our users, I added lots of python packages in the past to support Patroni — because the upstream packages (I’m sorry not maybe upstream packages) were not enough. The version wasn’t enough or maybe too low. So From some time to time I add non Postgres-related packages to the repo just to support the Postgres package. In the past it was PGAdmin, but now it’s not in our repo so it’s not a problem: their upstream is building their own RPMs, which is a good thing for us. We are building extra packages mostly for Patroni.
However, this is a potential problem for some enterprise users because large companies don’t even want to use the EPEL repo because they feel like it’s like it’s not a community repo, but a community repo controlled by Fedora and RedHat. That’s why from time to time I try to add some of the packages to our repo.
If it’s a problem for enterprise users, does it mean we should we maintain tons of extra packages in the EPEL repo for the GIS stack? The answer is “no, definitely no”. Not just because of the human power that we need to maintain those those packages — I mean rebuilding them is easy: I just get the source RPM, commit the spec files into our repo, and rebuild them. But maintaining them is something else.
We will have a similar problem when we release our own ICU package in the next few years. Because, now that we have the in core collation — but just for C Locale —- and people are trying to get rid of glibc, maybe we should have an ICU package. But maintaining an ICU Library across a single Postgres major version is a real big challenge that I don’t know how to solve for now, at least.
And then SLES — my pain in the neck — the GIS stack requires lots of repos on SLES 15. They are they are well documented on on our website.
Fedora is safe because Fedora is Fedora, everything is there, it’s easy to get a package there.
Jeremy S in chat: “Building them is easy. Maintaining them is something else.”Yeah that’s that’s the problem, Jeremy.
So how do you RPMify an extension?
The first thing is to know that the extension exists. This is one of the
big problems between developers and users. The developer creates a useful
extension and then they don’t create a tarball, they don’t release
anything. They expect people to install Git on their production databases
and git pull
, install make
, gcc
, all the development
libraries and build a binary, blah, blah blah.
I’m sorry that’ss not going to work. It also doesn’t work for pip — pip is not a package manager, it just destroys your server. It downloads things to random places and then everything’s gone. That’s why I added lots of Python packages to support Patroni, because most of the users use the packaging package manager to install Postgres and other packages to their servers. It’s the same for Debian, Ubuntu, for RPMs, for Windows, for macOS.
So first of all we know have to know that the extension exists and we have to have a tallbal. If I see an extension that seems good enough I’ll get there. PGXN is a good place, because when I go to pgxn.org a few times per day and see if there is a new version of an extension or if there’s new extension, it’s a good piece. But there’s a problem: we have hundreds of extensions — maybe thousands — but not all of them are on PGXN. They should be!
David Wheeler (he/him) in chat: You should follow @pgxn@botsin.space for new releases :-)
nils in chat: pg_xz incoming
I don’t know how to solve this problem, but we should expect every extension to announce their extensions on PGXN. I’m not just talking about installing everything through PGXN, but at least have an entry that there’s a new extension, this is repo, the website, the readme and the is a tarball. It doesn’t have to be on PGXN, as long as we have something.
And then I check the version. If there is an extension that will kill your database and the version is 0.001, that’s not going to be added to the repo, because we don’t want to distribute an experimental feature.
David Wheeler (he/him) in chat: LOL, all my extensions start at 0.0.1
David G. Johnston in chat: If it isn’t on PGXN it doesn’t exist.
Another problem is that lots of people write extensions but some of them are just garbage. I’m sorry but that’s the truth. I mean they just release a version and then do nothing.
David Wheeler (he/him) in chat: LOLOLOLFrom the chat, “pgxn_xz is coming”: that’s right! We have blackhole_fdw, which was written by Andrew Dunstan. When you create blackhole_fdw, it throws all of your data into black hole, so everything is gone.
Yeah, “if it’s not on PGXN it doesn’t exist,” that’s what I hope we achieve achieve in the next year or so.
Jimmy Angelakos in chat, replying to “If it isn’t on PGXN …”: I would correct that to “If it isn’t on PGXN it isn’t maintained.” Sometimes even ON PGXN…Yeah Jimmy, that’s one of the big problems that we have: maintenance.
We create the spec file, just copy one of the existing ones and start
editing. It’s easy but sometimes we have to add patches. We used to carry
patches for each Postgres major version to change the Makefile
s for the
specific Postgres major version. But I realized that it was [not a great
pattern]. Now we just export the path, which fixes the problem.
Then I initiate a scratch build for any missing requirements. If there are any missing build requirements it fails to build. I only do it on Fedora latest, not for every package because it doesn’t always help because some distros may not have the missing dependency
Alvaro Hernandez in chat: Hi everybody!
David G. Johnston in chat: Delegated to PGXN for that directory.
Let’s say we rely on some really good feature that comes with a latest version of something, but that latest version may not appear in RedHat 7 or 8. So some dist dros may have it, but the version may be lower than required. Or some distros may have the dependency under different name. Now in the spec file we have “if SUSE then this” and “if RedHat then this” “if Fedora then”, “if RedHat nine then this”, etc. That’s okay, it’s expected. As long as we have the package, I don’t care.
Then I push it to the Git repo, which I use not just for the spec files and patches, but also for carrying the spec files and pitches to the build instances.
Jorge in chat: How to handle extension versioning properly? I mean, in the control file the version could be anything, like for ex. citus default_version = ‘12.2-1’ where the “published version” is v12.1.2, then the “default version” could remain there forever.
Also have seen in the wild extensions that the control file have a version 0.1 (forever) and the “released” version is 1.5
If something fails I go back to the drawing board. GCC may fail (gcc 14
has been released on Fedora 40 and is causing lots of issues for for
packaging nowadays), it could be cmake
— too recent or too old. It
could be LLVM — LLVM18 is a problem for Postgres nowadays. I either try
to fix it ping upstream. I often ping upstream because the issue must be
fixed anyway
If everything is okay, just push the packages to the repo.
Ruohang Feng (Vonng) in chat: question: how about adding some good extensions written in Rust/pgrx to the repo? like pgml, pg_bm25, pg_analytics, pg_graphql….One issues is that there is no proper announcement. Maybe I have an awesome extension available in the Postgres repo that people crave and, we build the extensions, it took a lot of time (thank you Jimmy, he helped me a lot) and then I didn’t actually announce it that much. On the other hand, people just can use PG stat base [?] to install and start using it in a few seconds. This is something that we should improve.
Steven Miller in chat: How to handle system dependencies like libc versions being updated on the target system? Do extensions need to be complied against exactly a specific libc version?
From Nevzat in chat: how can we make sure bugfix or extension is safe before installing it
vrmiguel in chat: Interesting approach to identify build/runtime requirements
Over at Tembo we have a project called trunk-packager which attempts to automatically create .deb packages given the extension’s shared object.
We try to identify the dynamic libs the extension requires by parsing its ELF and then trying to map the required .so to the Debian package that provides it, saving this info in the .deb’s control file
From the chat: How to handle extension versions properly? That’s a good thing but, extension version and the release version don’t have to match. Thr extension version isn’t the same thing as the release version. It’s the version of the SQL file or the functions or the tables, the views, sort procedures, or whatever. If it’s 0.1 it means it’s 0.1 it means nothing nothing has changed in this specific regarding the control file. They they may bump up the package version because they may add new features, but if they don’t add new features to the SQL file, then they don’t update the extensions. I hope that answers your question George
I have another question from Ruohang. Yaaaaay! I was afraid that someone would ask that one. We have no extensions written in Rust in repo so far. It’s not like Go; there is a ban against Go because we don’t want to download the world, all the internet just to build an extension. If I recall correctly they’re rewriting pg_anonymizer in Rust. They will let me know when they release it or they’re ready to release it, and then I’ll build it. It’s not something I don’t like, it just hasn’t happened.
Keith Fiske in chat: I still update the control file with my extensions even if it’s only a library change. Makes it easier to know what version is installed from within the database, not just looking at the package version (which may not be accessible)
Ruohang Feng (Vonng) inchat: question: How to handle RPM and
extension name conflicts, e.g., Hydra’s columnar
and Citus’s
columnar
.
*David Wheeler (he/him) in chat, replying to “I still update the c…” Yeah I’ve been shifting to this pattern, it’s too confusing otherwise
If you think there are good extensions like these, just create a ticket on redmine.postgresql.org. I’m happy to take a look as long as I know them. That’s one of the problems: I have never heard about pg_analytics or pgml, because they’re not on PGXN. Or maybe they are. This is something that we should improve in the next few months.
Jimmy Angelakos in chat: Go is a pretty terrible ecosystem. It has its own package manager in the language, so it’s kinda incompatible with distro packages
Jason Petersen in chat: (but that means a build is safe within a single release, it doesn’t mean you can move a built extension from one Fedora version to another, right?)
David Wheeler (he/him) in chat, replying to “How to handle system…”: Libc is stable in each major version of the OS, and there are separate RPMs for each.
Another question from Steven: how to handle system dependencies like libc version updates. The answer is no. It’s mostly because they don’t update the libc major version across the across across the lifetime of the of the release. So we don’t need to rebuild the extension against libc.
Steven Miller in chat, replying to “How to handle system…”: Ok I see, TY
Jason Petersen in chat, replying to “How to handle system…”: Is that how we deploy in trunk, though?
David Wheeler (he/him) in chat, replying to “Go is a pretty terri…”: Huh? You can build binaries in a sandbox and then you just need the binary in your package.
[Addressing Nevzat’s question]: That’s a great question. It’s up to you! It’s no different than installing Postges or any other thing. I just build RPMs. If you’re reading the hackers mailing list nowadays, people rely on me an Christoph and others, so that we don’t inject any code into the RPMs or Debian packages. You just need to trust us not to add extra code to the packages. But if there’s a feature problem or any bug then you should complain upstream, not to us. so you should just test.
Jimmy Angelakos in chat, replying to “Go is a pretty terri…”: Find me one person that does this.
Steven Miller in chat, replying to “How to handle system…”: We don’t have OS version as one of the dimensions of version packaging but should
[Addressing vrmiguel’s comment]: Yeah, that could be done but like I don’t like complex things, that’s why I’m an RPM packager.
Jason Petersen in chat, replying to “Go is a pretty terri…”: (doesn’t go statically link binaries, or did they drop that philosophy?)
vrmiguel in chat: I think citus has changed it to citus_columnar
David Wheeler (he/him) in chat, replying to “Go is a pretty terri…”: Hugo: https://github.com/hugomods/docker/tree/main/docker/hugo
David Wheeler (he/him) in chat, replying to “Go is a pretty terri…”: Jason: Static except for libc, yes
Another question from Ruohang: uh how to handle RPM and extension name conflicts. I think Citus came first, so you should complain to Hydra and ask them to change the name. They shouldn’t be identical. We have something similar with Pgpool: they they are conflicting with the PCP Library ,which has been in the Linux for the last 25 years. I think Pgpool has to change their name.
Jeremy S in chat, replying to “I still update the c…”: So you think people will run the “alter extension upgrade” eh?[Addressing Keith Fiske’s comment]: I’m not saying I don’t agree with you, but it means every time I have to update my extension version in my running database — it’s some extra work but that’s okay. It’s the user problem, not my problem.
Question from Jason [on moving an extension from one Fedora to another]: Right, it may not be safe because the GCC version may be different and other stuff may be different. One distro to another is not safe, Jason; sorry about that.
[Back to Steven’s question]: Yes, David’s answer is right.
[Addressing vrmiguel’s comment about citus_columnar]: You are right.
Jimmy I’m not going to read your comment about go because I don’t think think you can swear enough here.
vrmiguel in chat, replying to “Go is a pretty terri…”: Are there known Postgres extensions written in Go? Not sure how Go is relevant here
Jason Petersen in chat: you said “gcc” and not “libc” there, are you implying that things like compiler versions and flags need to be identical between postgres and extensions
Keith Fiske in chat, replying to “I still update the c…”: I think they should …
David Wheeler (he/him) in chat, replying to “Go is a pretty terri…”: Were some experiments a few years ago. https://pkg.go.dev/github.com/microo8/plgo
Let me continue now. First you have to know the extension exists, and then the you also need to know that the extension has an update. Unfortunately the same problem: the extension exists or has an update and they just don’t let us know.
Jimmy Angelakos in chat, replying to “Go is a pretty terri…”: @vrmiguel now you know why :DThis is a big challenge Fedora has in house solution.When you add a new package to Fedora, I think they crawl their repo once a day and if there’s new release they create a ticket in their bug tracker automatically, so that the maintainer knows there’s a new version. This can be done, but would need a volunteer to do it. Orr maybe the easiest thing is just add everything to the to PGXN,
When we update an extension we, have to make sure it doesn’t break anything. It requires some testing. As I said earlier, building is one thing, maintaining the extension is a bigger thing. If you want to raise a baby, you are responsible until until the end of your life. Consider this like your baby: either just let us know if you can’t maintain an extension anymore or please respond to the tickets that I open.
Steven Miller in chat: One other detail about compatibility dimensions. We have noticed some extensions can be complied with chip-specific instructions like AVX512, for example vector does this which optimizes performance in some cases
Alvaro Hernandez in chat, replying to “you said “gcc” and n…”: I’d reverse the question: do we have strong guarantees that there are no risks if versions and/or flags may differ?
I believe extensions are already risky in several ways, and we should diminish any other risks, like packaging ones.
So I’d say absolutely yes, compile extensions and Postgres in exactly the same way, versions and environments.
Sometimes a new minor version of an extension breaks a previous Postgres release. For example, an extension drops support for Postgres 12 even though Postgres 12 is still supported. Or they didn’t do the upgrade path work. I have to make sure everything is safe.
nils in chat, rReplying to “I think citus has ch…”: It was never changed, the extension has always either been embedded in Citus or later moved to a separate extension called citus_columner.
I think the name conflict comes from the access method being called
columnar
, which Citus claimed first. (Hydra’s started actually as a
fork from Citus’ codebase).
(disclaimer; I work on Citus and its ecosystem)
Jason Petersen in chat, replying to “I think citus has ch…”: hi nils
Next month a new beta comes out. Everyone is happy, let’s start testing new features. For the packagers that means it’s time to start building extensions against beta-1. So a build might fail, we fix it, and then it may fail against beta-2. I understand if extension authors may want to wait until rc-1. That’s acceptable as long as they let us know. Many of them fail, and then Christoph and I create tickets against them and display them on wiki.postgresql.org. It’s a Hall of Shame!
Eric in chat: When you decide to package a new extension do you coordinate with upstream to make that decision?
David Wheeler (he/him) in chat, replying to “When you decide to p…”: I learned I had extensions in the yum repo only after the fact
Eric in chat, replying to “When you decide to p…”: I see
vrmiguel in chat: @Devrim Gündüz I’m curious about how RPM deals with extensions that depend on other Pg extensions
David Wheeler (he/him) in chat: You can test Postgres 17 in the pgxn-tools docker image today. Example: https://github.com/theory/pgtap/actions/runs/8502825052
This list pisses off the extension authors because they don’t respond to ticket. So what do we do next? It happens again and again and again, because they just don’t respond to us. On Monday uh I got a response from an extension maintainer. He said “you are talking like you are my boss!” I said, “I’m talking like I’m your user, I’m sorry. I just asked for a very specific thing.”
nils in chat: I love Devrim’s issues against our repo’s! They are like clockwork, every year 😄
David Wheeler (he/him) in chat, replying to “You can test Postgre…”: It relies on the community apt repo
Eric in chat, replying to “When you decide to p…”: Related: ever had upstream request you stop packaging an extension?
Steven Miller* in chat, replying to “One other detail abo…”: Even if compiled inside a container, on a specific chip it can get chip-specific instructions inside the binary. For example building vector on linux/amd64 inside docker on a chip with AVX512, the container image will not work on another linux/amd64 system that does not have AVX512
David Wheeler (he/him) in chat: :boss:
Unresponsive maintainers are a challenge: they don’t respond to tickets, or emails, or they don’t update the extensions for recent Postgres versions.
Don’t get me wrong even the big companies also do this, or they don’t update the extensions for the new GCC versions. I don’t expect them to test everything against all all the GCC versions; that’s that’s my problem. But just respond please.
What’s the responsibility of the packager in this case? Should we fork if they don’t respond at all? No we are not forking it! VBut going to conferences helps, because if the extension author is there I can talk to them in person in a quiet place, in a good way, just “please update the package tomorrow or you’re going to die”. Of course not this but you see what I mean.
[Looking at chat]: I’m going to skip any word about containers; sorry about that.
[Addressing Eric’s question]: That’s a good so so the question! No, actually they support us a lot, because that’s the way that people use their extensions. And do we coordinate with upstream? No, I coordinate with myself and try to build it. Of course upstream just can just create a ticket, send me email, or find me at a conference. They can say, “hey, we have an extension, could you package an RPM?” Sure, why not." I don’t coordinate with Upstream as long as uh there is no problem with the builds.
Eric in chat, replying to “When you decide to p…”: So you haven’t run into a situation where upstream did not want/appreciate you packaging for them?[Respondinding to nils’s comment]: Thank you, thanks for responding!
[Responding to vrmiguel’s question about depending on other extensions]: We actually add dependency to that one. That’s bit of uh work, like PG rotting depends on PostGIS. In order to provide a seamless installation the PostGIS package, in the PostGIS spec file, I add an extra line that says it provides PostGiS without the version as part of the name. Then when we install pg rotting, it looks for any PostGIS package — which is fine because it can run against any PostGIS version. So I add the dependency to other extensions if we need them.
David G. Johnston in chat: The tooling ideally would report, say to PGXN or whatever the directory location for the initial application is, the issues and remind them that if the build system cannot build their extension it will not be included in the final RPM. You are an unpaid service provider for them and if they don’t meet their obligations their don’t get the benefit of the service.[Responding to Eric’s upstream follow-up question]: I haven’t seen anything in any upstream where a person didn’t want me to package. But I haven’t seen many appreciations, either; I mean they don’t appreciate you. I’m being appreciated by EDB — money, money, money, must be funny — thanks EDB! But I haven’t had any rejections so far. Good question!
Eric in chat, replying to “When you decide to p…”: Fair. Cool. ThanksRelying on external repos is a big problem for SUSE. Some of the maintainers just discontinue their repo. One problem with SUSE is they don’t have an EPEL-like repo. EPEL is a great thing. The barrier to add a package to EPEL is not low but not high, either. If you if you’re an advanced packager you can add a package quick enough. Of course it requires review from others. But this a big problem for SUSE.
Lack of maintenance is a problem. We have a repo but they don’t update it; so I have to go find another repo from build.opensuse.org, change it, update the website, change our build instance, etc. That’s a big problem.
David Wheeler (he/him) in chat, replying to “The tooling ideally …”: I want to add build and test success/fail matrices to extension pages on PGXN
Florents Tselai in chat: How do you handle Pl/Python-based extensions + pip dependencies? Especially with virtualenv-based installations. i.e. Pl/Python usually relies on a /usr/bin/python3, but people shouldn’t install dependencies there.
And then there’s costs! What’s the cost of RPMifying an extension? Hosting a build server? We have a very beefy bare metal build server hosted by Enterprise DB, just because I’m working for them and they have a spare machine. Hosting a build server is a cost.
I have to use some external resources for architecture reasons, like some of our build instances, like PPC 64 ,is hosted somewhere else. There are some admin tasks to keep everything and running, like EDB’s IT team actually helped me to fix an issue today in both of our PPC instances.
Jason Petersen in chat, replying to “How do you handle Pl…”: I think early on he said he makes the extensions rely on RPMs that provide those Python dependencies
David Wheeler (he/him) in chat, replying to “How do you handle Pl…”: I have used this pattern for RPMifying Perl tools
Then, maintaining build instances requires keeping them up-to-date, and also that each update doesn’t break anything. It’s not like “dnf update and build a package”. No. It may be a problem with Fedora because Fedora may can update anything any time they want. But it’s a less problem for SUSE and RedHat, but we have to take care that the updates don’t break anything.
Redhat, the company, actually follows our release schedule. We release every three months. Unless something bad happens, we know the next release is in May, on a Thursday. So every Wednesday, one day before our minor release, RedHat releases their new maintenance releases. RedHat is going to release 9.4 on Wednesday before our minor release. What does that mean for us as an RPM packager for RedHat?
*RedHat releases a new version with a new LLVM, for example, and then it means we have to rebuild the packages against the new LLVM so that people can use it. That means I have to work until Thursday morning to build the packages. That’s fine but another problem is for Rocky and Alma Linux users, because they’re are not going to have the updated LLVM package, or any any updated package, like GCC. It’s not like the old RedHat days; they change everything uh in minor versions.
So I have to rebuild GCC and LLVM on our instances, add them to our special repo “sysupdates”, which is in the config file, and this takes many hours because building GCC and LLVM is a big thing.
In the last two years I have not been able to build the from GCC Source RPM. I had to edit everything and not edit the spec files blah blah to be able to build it. I have no idea how how they can break in Source RPM.
So that’s another cost: in May I’m going to spend lots of cycles to keep up with the latest RedHat release, and also make the make the Rocky Linux and Alma Linux users happier. Maintaining build systems is not as easy as running Yup or Zypper update. It requires employing the packager — because I have the bills pay I have the beers to drink.
[Addressing Florents’s PL/Python question]: I don’t know what the PL/Python based extensions are, but I tried to get rid of everything related to pip. I’m not a developer, a DBA isn’t a developer, a Sysadmin isn’t a developer. They’re not suposed to use pip; they are supposed to use the package manager to keep up with everything. My point is if someone needs pip then I should fix it. That’s what I did for Patroni. I added lots of packages to our Git repo just to be able to support Patroni.
Ian Stanton in chat: Need to drop, thank you Devrim!
Jeremy S in chat, replying to “How do you handle Pl…”: A lot of larger companies have inventory management and risk control processes that heavily leverage package management
Alvaro Hernandez in chat: Need to go, ttyl!
vrmiguel in chat, replying to “you said “gcc” and n…”: Do you
think there are no guarantees at all? For instance, Postgres loads up
the extension with dlopen
, which could fail with version mismatch
.
If that doesn’t occur and the extension loads ‘fine’, how likely do
you think an issue could be?
Also I’m curious how often you’ve seen problems arise from libc itself (rather than any of the many things that could cause UB in a C program) and how these problems have manifested
Ahmet Melih Başbuğ in chat: Thank you
ConclusionI thanked Devrim and all the discussion, and pitched the next mini-summit, where I think Jonathan Katz will talk about the TLE vision and specifics.
Thank you all for coming!
More about… Postgres PGXN Extensions PGConf Summit Yum ZYppKurze Zeit bevor das Europäische Parlament heute die Genehmigung zur freiwilligen Chatkontrolle durch US-Dienste wie Facebook, Instagram oder GMail um zwei Jahre verlängern will, gibt das Landgericht Kiel einen Rückschlag der Klage des Piraten-Europaabgeordneten Dr. Patrick Breyer gegen die Maßnahme bekannt: Es traf keine Entscheidung über Zulässigkeit der freiwilligen Chatkontrolle durch Meta, sondern lehnte die Klage in erster Instanz aus formalen Gründen ab. So könne Breyer der Chatkontrolle durch manuelle Aktivierung der Ende-zu-Ende-Verschlüsselung in jedem Chat entgehen. Zum anderen könne Breyer “die Durchsetzung einer politischen Kampagne … nicht im Rahmen eines Zivilprozesses” verfolgen. Breyer kündigt Berufung gegen die Entscheidung an:
“Ich schalte jetzt das Oberlandesgericht ein, damit Meta endlich sichere Verschlüsselung für alle Chats voreinstellt und die eigenmächtige wahllose Chatkontrolle auch durch andere Anbieter als Verstoß gegen das digitale Briefgeheimnis gestoppt wird. Zuckerbergs Meta-Konzern hat im Prozess alles daran gesetzt, zu verhindern, dass über die Zulässigkeit seiner eigenmächtigen, verdachtslosen Chatkontrolle entschieden wird. Das wird ihm auf Dauer nicht gelingen.” Breyer verweist auch auf die anhängige Parallelklage eines Missbrauchsbetroffenen vor dem Landgericht Passau, die von der Gesellschaft für Freiheitsrechte unterstützt wird.
“Es macht mich wütend, dass sich US-Konzerne mithilfe fehleranfälliger Verdächtigungsmaschinen zum Privatkontrolleur unserer persönlichen Nachrichten aufschwingen wollen. Dieser Big Brother-Angriff auf unsere Handys, Privatnachrichten und Fotos mithilfe fehleranfälliger Algorithmen ist, wie wenn die Post alle Briefe öffnen und scannen würde – ineffektiv und illegal. Missbrauchsbetroffene kritisieren schon länger, dass die Chatkontrolle ihnen durch Zerstörung privater Räume bei Hilferufen, Austausch, Therapie und Beratung schadet, statt zu helfen. Unsere ohnehin überlasteten Ermittler mit vielfach falschen Maschinendenunziationen zu überfluten und dadurch Kapazitäten für Ermittlungen gegen die Hintermänner zu stehlen, hat mit Effizienz nichts zu tun. Organisierte ‘Kinderporno-Ringe’ benutzen keine unverschlüsselten Messengerdienste. Folge ist vor allem die massenhafte Kriminalisierung Jugendlicher wegen unbedachter Chatnachrichten oder einvernehmlichem Sexting – so wird das Gegenteil von Kinderschutz erreicht.” Breyer verweist auf die gestern veröffentlichte polizeiliche Kriminalstatistik, derzufolge im vergangenen Jahr fast 40% der Tatverdächtigen wegen “Kinderpornografie” minderjährig waren.
Die EU-Innenminister beraten unterdessen über eine weltweit einzigartige Folgeverordnung (Chatkontrolle 2.0), welche die Chatkontrolle verpflichtend machen und sichere Ende-zu-Ende-Verschlüsselung zerstören soll.
xkcd nails it again.
SPD, Grüne und FDP haben laut Medienberichten den Weg frei gemacht für das von Justizminister Buschmann vorgeschlagene Quick-Freeze-Verfahren.
Der Europaabgeordnete Dr. Patrick Breyer (Piratenartei) kommentiert:
„Diese Koalitionsentscheidung ist ein Erfolg für die Bürgerrechtsbewegung, die seit Jahrzehnten auf der Straße und vor Gericht gegen die Idee einer flächendeckenden Totalerfassung unserer Kontakte, Bewegungen und Internetverbindungen kämpft. Wie im Koalitionsvertrag vereinbart setzt die Ampelkoalition auf eine effektive und anlassbezogene Datenspeicherung (Quick Freeze) und erteilt der extremen SPD- und Unionsforderung nach einer flächendeckenden Internet-Vorratsdatenspeicherung eine klare Absage. Diese wäre ein Dammbruch und ein Frontalangriff auf unser Recht auf anonyme Internetnutzung, auf das unzählige Menschen angewiesen sind.
In einem Punkt ist Buschmanns Quick Freeze-Gesetzentwurf aber massiv nachbesserungsbedürftig: Es fehlt die Vorgabe, dass die betroffenen Personen und Anschlüsse in der Freeze-Anordnung genau bezeichnet werden müssen. Mit dem bisherigen Wortlaut könnten Staatsanwälte und Amtsgerichte flächendeckend die Kommunikationsdaten sämtlicher Bürger auf Vorrat speichern lassen, weil sie für ein Ermittlungsverfahren künftig von Nutzen sein könnten – das muss dringend nachgebessert werden.
Die Koalitionseinigung ist auch nur ein Etappensieg: Die EU plant nach der Europawahl einen neuen Anlauf zur EU-weiten Wiedereinführung einer IP-Vorratsdatenspeicherung, die Vorrang vor deutschem Recht hätte. Das undemokratische Prinzip der anlasslosen Speicherung muss auch von der Tagesordnung der EU-Kommission und der Regierungen anderer Mitgliedsländer verschwinden – die EU-Arbeitsgruppe #EUGoingDark, die für eine EU-weite Wiedereinführung der Vorratsdatenspeicherung sorgen soll, gehört aufgelöst.
Und sollte der Ampel eine Große Koalition folgen, werden die schwarz-roten Grundrechtsterroristen unsere Privatsphäre in der Luft zerreißen. Wir Piraten werden als parlamentarischer Arm der Bürgerrechtsbewegung im Kampf gegen Massenüberwachung nicht locker lassen!“
Die Spitzenkandidatin der Piratenpartei zur EU-Wahl, Anja Hirschel kommentiert:
„Auf anlasslose Vorratsdatenspeicherung zu verzichten ist eine richtige und kluge Entscheidung für die wir lange gekämpft haben! Wir müssen aber auch jetzt genau hinschauen: Quick Freeze ist ein anlassbezogenes und machtvolles Ermittlungsinstrument, dass durch Zugriff auf E-Mail-Adressen, Telefonnummern, IP-Adressen, Standortdaten und weitere hoch-sensible Daten sehr tief in die Grundrechte von Bürgerinnen und Bürgern eingreifen kann. Wir müssen sicherstellen, dass Quick Freeze grundrechtskonform ausgestattet wird und ausschließlich maßvoll, demokratisch und transparent eingesetzt wird. Die Möglichkeiten, QuickFreeze einzusetzen müssen so ausgestaltet und gesichert sein, dass sie nicht von Feinden der Demokratie missbraucht werden können.
Any business that depends on third-party APIs that it does not control and is locked into using is not a good business.
I hate writing resumés. There’s always been something about the format that never really sat right with me; each entry presents work I’ve done, sometimes representing many multiple years of my life, without explaining the “why” or the through-line of how I got there. It’s always felt to me like they’re missing the fundamentals of the human being behind them — the values and mindsets of the living, breathing person you’d actually be hiring.
On top of that, mine was stuck in a document, when all of my work is on the web.
So I set out to rewrite mine, make it web-first, and turn it into something that I feel like actually represents me and my career. You can check it out here: I gave it its own subdomain at resume.werd.io.
The default version now displays my career in chronological order, each life event building on the next, and includes things that, while not technically being work, informed the work I’ve subsequently done. You’ll find stuff like the electronic hypertext magazine I built as a teenager and the popular meme site I built at university, which wouldn’t normally find their way into a standard resumé, but do help define my expertise and how I approach projects.
From that page, you can flip to a more traditional reverse-chronological resumé that omits the non-employment content and removes unnecessary description. Because it’s an edited-down version of my story, rather than a collection of bullet points, it’s probably better than any resumé I’ve had in the past. It’s web-first but there are a few print-only CSS rules in there to make sure it prints or turns into a PDF really nicely. It’s also nicely responsive, so it displays well on both a laptop / desktop and a phone.
Behind the scenes, here’s how it works:
Each element of my experience is a standalone HTML snippet file of the formstartyear-endyear.html
. Some are annotated to be “narrative-only”, which means that they won’t show up in the traditional view. The engine I wrote for this will also omit any entries marked “traditional-only” from the narrative view, but in practice I didn’t use that.
In narrative view, the engine reads the files in alphabetical order. In traditional view, the engine reads them in reverse-alphabetical order. The effect is to order the entries chronologically or reverse-chronologically as described.
Educational entries are marked as such. In narrative view they’re placed into the main flow; in traditional view they’re displayed at the end of the document.
In narrative view, any HTML element with a class traditional
is marked as display:none
in order to hide it; in traditional view, any HTML element with a class narrative
is hidden. This allows me to mix narrative content in with more traditional resumé content and only display them in one view.
Everything is marked up with the h-resume microformat.
The most important thing for me was being able to write my story as a story, and then edit down accordingly. I’m pretty happy with it! Let me know what you think.
Here’s the latest installment in the series on working with LLMS: https://thenewstack.io/code-in-context-how-ai-can-help-improve-our-documentation/.
Writing documentation from scratch is as uncommon as writing code from scratch. More typically, you’re updating or expanding or refactoring existing docs. My expectation was that an LLM-powered tool primed with both code and documentation could provide a powerful assist, and Unblocked did.
I don’t know how to measure the boost it gave me. But I do know that I’ll never again want to undertake this kind of project without a tool that can help me assemble the necessary context.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
12 Let’s talk: conversational software development
13 Using LLMs to Improve SQL Queries
14 Puzzling over the Postgres Query Planner with LLMs
15 7 Guiding Principles for Working with LLMs
16 Learn by Doing: How LLMs Should Reshape Education
Contrails form behind jet aircraft flying through the stratosphere. Since high-altitude aviation is happening all around the earth more or less constantly, planes are painting the sky everywhere. (Here is one time-lapse. And another. And one of my own.)
Many contrails don’t last, of course, but many do, and together they account for much of the cloud cover we see every day. The altocumulus, altostratus, and cirrus clouds that contrails produce are now officially recognized as homogenitus and homomutatus, which are anthropogenic: owing to human activity.
And today, Eclipse Day, Delta is offering to fly you along the path of totality. Others too? I don’t know. I’m taking a few moments to write this before we walk up to our hilltop cemetery to watch the eclipse for over four minutes, thanks to our lucky location near the very center of Totality.
I’m curious to see and hear contrail reports from others now awaiting their few minutes out of the sun.
1:14pm—The moon’s shadow made landfall in Mexico a short time ago. Here in Bloomington, the sky is well-painted by contrails. Mostly it looks like high-altitude haze, but believe me: if it weren’t for commercial aviation, the sky would be solid blue. Because the contrails today are quickly smeared sideways, losing their form but not their color.
5:00pm—Contrails were aplenty, and a spread-out contrail did slide in front of the sun and the moon…
but it was still a spectacular sight:
So, let’s get this out of the way first: I drive a Tesla Model 3. Yes, I know. And yes, even though I bought it a few years ago, there was plenty of evidence of the CEO’s bad behavior and of poor conditions in the factories. And I let my desire to drive an electric car override all that, and it was a poor decision, and now I’m stuck with it because it was also a very bad financial decision and it’s depreciated at such a rate that the diff of the value of the car and the remaining value of my car loan are not in a good place. It was silly. Can we just accept that? Okay, thank you. Moving on.
This month Tesla switched Full Self Driving (FSD) on .. as far as I can tell, every single car. It’s normally a five figure software-only upgrade, which you can pay for up-front or at the ongoing subscription price of $199 a month. But usage of the feature has been low, perhaps in part because if you’ve spent $40-90,000 on a car, spending another five figure sum is annoying, and perhaps in part because people generally value their own lives and the lives of the people they love. So now, for this month only, it’s free, and Tesla can juice their numbers.
And, yes, I tried it.
And let me be clear: nope. Nope nope nope nope nope nope nope.
I’ve rarely been so frightened behind the wheel of my own car. As an experience it ranks right up there with driving that one stretch of the 880 where you’re supposed to go at 45mph and everybody drives twice that, the time I was riding in a Lyft and was jackknifed by a tow truck, or that one time I was driving on the 101 North and a tech bro Lamborghini shot out of the Highway 12 offramp and came within two inches of hitting me, spun around in the road, and careened off into the distance.
To be added to those heart-stopping experiences soon, I have to surmise, is being hit by a Tesla in Full Self Driving Mode.
Unlike my Lambo encounter, I was driving at 25mph down my local road to the store. On this 10 minute drive (5 minutes there and back), my car clung surprisingly close to cars parked on the side of the road — but more importantly, I had to intervene once because, on a stretch of road that had been coned off and narrowed into one lane for both directions, it felt like the car was driving directly into an oncoming vehicle. It’s possible (and, in fact, quite likely) that the car wouldn’t have smashed into the oncoming Subaru. But it felt like it was about to, and I had no desire to make it a scientific test. FSD requires you to keep your hands on the wheel — a lean-back experience this is not — so safely intervening was very natural. The car then asks you to leave a recorded message explaining why you intervened, and the struggle is to use words instead of screaming over and over.
What’s particularly surprising to me is that FSD wasn’t just downloaded to my vehicle — it was switched on by default. At no point did I need to agree to the terms and conditions. All I needed to do was enter a destination into the navigation and pull the gear stalk down once (as if I was simply changing into another gear), and off it went. Autopilot, which is what Tesla calls its cruise control feature, is engaged in a very similar way, so I can see a world where a driver might even switch it on unintentionally.
So would I pay the money for it? Fear on this level seems like a pretty poor use of $12,000 or $199 a month. You can rent a horror movie for $2.99, and paying attention to America is free. But clearly I was curious. Genuinely, if the technology improved to the point where I didn’t feel like I was probably going to die, I would happily sit inside a self-driving vehicle. Less so, perhaps, for going to the store, but definitely for road trips. (Can you imagine an autonomous RV? It would cost an arm and a leg, but if I had the money, I would 100% use one to get across country.) Right now, though, I would rather pour vinegar onto my eyeballs. For those readers who don’t need to think hard about dropping $12,000 — I am not one of them — I would encourage you to spend the money on more caviar or whatever. This ain’t it.
This article shows how to implement a web application using backend for frontend security architecture for authentication and consumes data from a downstream API protected using a JWT access token which can only be accessed using an app-to-app access token. The access token is acquired using the OAuth2 client credentials flow and the API does not accept user access tokens from the UI application. OpenIddict is used as the OpenID Connect server. The STS provides both the OAuth2 client and the OpenID Connect client as well as the scope definitions.
Code: https://github.com/damienbod/bff-aspnetcore-angular-downstream-api
The BFF web application is implemented using ASP.NET Core and Angular as the UI tech stack. The Angular part of the web application can only use the ASP.NET Core APIs and secure same site cookies are used to protect the access. The whole application is authenticated using an OpenID Connect confidential code flow client (PKCE). If the web application requires data from the downstream API, a second OAuth client credentials flow is used to acquire the access token. The downstream API does not accept the user delegated access tokens from the UI application.
BFF OIDC code flow clientImplementing the OpenID Connect confidential client is really simple in ASP.NET Core. The AddAuthentication method is used with cookies and OpenID Connect. The cookies are used to store the session and the OpenID Connect is used for the challenge. All server rendered applications are setup like this with small changes required for the OIDC challenge. Due to these small differences, the different OIDC implementations provide specific implementations of the client. These are normally focused and optimized for the specific OIDC servers and do not work good with other OIDC servers. Once you use more than one OIDC server or require multiple clients from the same OIDC server, the client wrappers cause problems and you should revert back to the standards.
var stsServer = configuration["OpenIDConnectSettings:Authority"]; services.AddAuthentication(options => { options.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.DefaultChallengeScheme = OpenIdConnectDefaults.AuthenticationScheme; }) .AddCookie() .AddOpenIdConnect(options => { configuration.GetSection("OpenIDConnectSettings").Bind(options); options.SignInScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.ResponseType = OpenIdConnectResponseType.Code; options.SaveTokens = true; options.GetClaimsFromUserInfoEndpoint = true; options.TokenValidationParameters = new TokenValidationParameters { NameClaimType = "name" }; }); Yarp ProxyThe Angular UI can only request data from the ASP.NET Core backend using secure http only cookies. The Angular UI is deployed as part of the ASP.NET Core application in production builds. When creating applications, software developers need to use their preferred tools and YARP is used to support this in the development setup. As a further downstream API is used, YARP can also be used to support this. The proxy takes the API request, validates the cookie, uses another access token and forwards the request to the downstream API. YARP has an ITransformProvider interface which is used to implement this. This also means we have two different YARP configuration setups for development and deployments. (test, integration, production).
using System.Net.Http.Headers; using Yarp.ReverseProxy.Transforms; using Yarp.ReverseProxy.Transforms.Builder; namespace BffOpenIddict.Server.ApiClient; public class JwtTransformProvider : ITransformProvider { private readonly ApiTokenCacheClient _apiTokenClient; public JwtTransformProvider(ApiTokenCacheClient apiTokenClient) { _apiTokenClient = apiTokenClient; } public void Apply(TransformBuilderContext context) { if (context.Route.RouteId == "downstreamapiroute") { context.AddRequestTransform(async transformContext => { var access_token = await _apiTokenClient.GetApiToken( "CC", "dataEventRecords", "cc_secret"); transformContext.ProxyRequest.Headers.Authorization = new AuthenticationHeaderValue("Bearer", access_token); }); } } public void ValidateCluster(TransformClusterValidationContext context) { } public void ValidateRoute(TransformRouteValidationContext context) { } }The AddReverseProxy is used to add the YARP services.
builder.Services.AddReverseProxy() .LoadFromConfig(builder.Configuration.GetSection("ReverseProxy")) .AddTransforms<JwtTransformProvider>();And the middleware:
app.MapReverseProxy(); API client credentials clientThe YARP proxy uses the OAuth client credentials client to get an access token to access the downstream API. The token is stored in a cache and only rotated when it expires or is missing. The app-to-app security has nothing to do with the delegated client from the web application.
using IdentityModel.Client; using Microsoft.Extensions.Caching.Distributed; namespace BffOpenIddict.Server.ApiClient; public class ApiTokenCacheClient { private readonly ILogger<ApiTokenCacheClient> _logger; private readonly HttpClient _httpClient; private static readonly object _lock = new(); private readonly IDistributedCache _cache; private readonly IConfiguration _configuration; private const int cacheExpirationInDays = 1; private class AccessTokenItem { public string AccessToken { get; set; } = string.Empty; public DateTime ExpiresIn { get; set; } } public ApiTokenCacheClient( IHttpClientFactory httpClientFactory, ILoggerFactory loggerFactory, IConfiguration configuration, IDistributedCache cache) { _httpClient = httpClientFactory.CreateClient(); _logger = loggerFactory.CreateLogger<ApiTokenCacheClient>(); _cache = cache; _configuration = configuration; } public async Task<string> GetApiToken(string api_name, string api_scope, string secret) { var accessToken = GetFromCache(api_name); if (accessToken != null) { if (accessToken.ExpiresIn > DateTime.UtcNow) { return accessToken.AccessToken; } else { // remove => NOT Needed for this cache type } } _logger.LogDebug("GetApiToken new from STS for {api_name}", api_name); // add var newAccessToken = await GetApiTokenInternal(api_name, api_scope, secret); AddToCache(api_name, newAccessToken); return newAccessToken.AccessToken; } private async Task<AccessTokenItem> GetApiTokenInternal(string api_name, string api_scope, string secret) { try { var disco = await HttpClientDiscoveryExtensions.GetDiscoveryDocumentAsync( _httpClient, _configuration["OpenIDConnectSettings:Authority"]); if (disco.IsError) { _logger.LogError("disco error Status code: {discoIsError}, Error: {discoError}", disco.IsError, disco.IsError); throw new ApplicationException($"Status code: {disco.IsError}, Error: {disco.Error}"); } var tokenResponse = await HttpClientTokenRequestExtensions.RequestClientCredentialsTokenAsync(_httpClient, new ClientCredentialsTokenRequest { Scope = api_scope, ClientSecret = secret, Address = disco.TokenEndpoint, ClientId = api_name }); if (tokenResponse.IsError || tokenResponse.AccessToken == null) { _logger.LogError("tokenResponse.IsError Status code: {tokenResponseIsError}, Error: {tokenResponseError}", tokenResponse.IsError, tokenResponse.Error); throw new ApplicationException($"Status code: {tokenResponse.IsError}, Error: {tokenResponse.Error}"); } return new AccessTokenItem { ExpiresIn = DateTime.UtcNow.AddSeconds(tokenResponse.ExpiresIn), AccessToken = tokenResponse.AccessToken }; } catch (Exception e) { _logger.LogError("Exception {e}", e); throw new ApplicationException($"Exception {e}"); } } private void AddToCache(string key, AccessTokenItem accessTokenItem) { var options = new DistributedCacheEntryOptions() .SetSlidingExpiration(TimeSpan.FromDays(cacheExpirationInDays)); lock (_lock) { _cache.SetString(key, System.Text.Json.JsonSerializer.Serialize(accessTokenItem), options); } } private AccessTokenItem? GetFromCache(string key) { var item = _cache.GetString(key); if (item != null) { return System.Text.Json.JsonSerializer.Deserialize<AccessTokenItem>(item); } return null; } } Downstream APIThe downstream API is protected using JWT access tokens. This is setup using the AddJwtBearer method. The scope and other claims should also be validated.
services.AddAuthentication() .AddJwtBearer("Bearer", options => { options.Audience = "rs_dataEventRecordsApi"; options.Authority = "https://localhost:44318/"; options.TokenValidationParameters = new TokenValidationParameters { ValidateIssuer = true, ValidateAudience = true, ValidateIssuerSigningKey = true, ValidAudiences = ["rs_dataEventRecordsApi"], ValidIssuers = ["https://localhost:44318/"], }; }); NotesThis setup can be used for all server rendered applications. You should always use an external identity provider in enterprise setups and never roll out your own identity system as this is expensive to maintain and many enterprise environments no longer accept this due to the extra operation costs. Replacing Angular with react, Vue.js, Svelte or Blazor WASM does not require changes to the authentication. The different UI have differences on how the scripts are loaded or used and some require weaker session security setups.
You should also avoid downstream APIs if not required. Modular monoliths have performance advantages.
Linkshttps://github.com/damienbod/bff-aspnetcore-angular
https://learn.microsoft.com/en-us/aspnet/core/introduction-to-aspnet-core
https://nx.dev/getting-started/intro
https://github.com/isolutionsag/aspnet-react-bff-proxy-example
https://github.com/damienbod/bff-auth0-aspnetcore-angular
https://github.com/damienbod/bff-azureadb2c-aspnetcore-angular
https://github.com/damienbod/bff-aspnetcore-vuejs
https://github.com/damienbod/bff-MicrosoftEntraExternalID-aspnetcore-angular
https://microsoft.github.io/reverse-proxy/articles/transforms.html
https://github.com/microsoft/reverse-proxy/tree/main/samples/ReverseProxy.Transforms.Sample
Well, our house sold, and it looks like my next journey is about to begin. I decided to leave town and let the realtor do his thing. On April Fool’s Day we had multiple offers. Who knew that vintage charm held such sway in a world where the incessant drum beat of emerging tech tries to drown out everything else? The process was about as quick and painless as putting one’s life on display with money attached can be. Please know how much I appreciate all of you who have been sending good thoughts and prayers my way. It’s not an easy transition, but the long goodbye is coming to a close. I’ll be taking many boxes of books down to Arkansas by U-Haul next week – priorities, right? Even though the house sale went faster than I ever expected, I continued my planned trip through Syracuse, Oneida, Palmyra, Rochester, Burlington, and Montpelier. It was cold and rainy interspersed with sleet and snow, which made the stretch through the Adirondacks even more picturesque. I’m going to imagine it as a prolonged cleanse of my soul in preparation for the road ahead.
I did a brief overview video tonight, because I was afraid of not having time to do a more polished presentation. For the next month my focus has to be on clearing out the house and moving everything into storage. I did forget to mention my first stop at SUNY Binghamton on the ride up, the stomping grounds for Jeffrey Yass where he started his gambling habit. I’m going to drop some photos in this post, so you can see highlights from the trip so far. These aren’t my best hearts, because materials were limited, the weather was challenging, and the wind wanted to blow everything all over the place. Still, my good intentions were there even if my cold fingers and water drops on my glasses made the presentation less than polished. I do plan to stop at Ben and Jerry’s tomorrow – one of the first benefit corporations.
SUNY Binghamton, where Jeffrey Yass of Susquehanna International Group refined his gambling technique.
Oneida Community Mansion, Bible Communism, free love, divination, and eugenics in the context of corporate morality, with some amazing trees!
Cumorah and Joseph Smith’s Sacred Grove in Palmyra, NY –
Strong Memorial Hospital was the first “Flexner” model hospital, underwritten George Eastman of Eastman Kodak. The hospital was used by the University of Rochester for nonconsensual radiation exposure experiments during the Manhattan Project. It also had one of the first integrated psychiatric units. The Terrence Building, now abandoned, was one of the largest state mental hospitals in the United states from the 1950s through the 1990s, and had over 1,000 beds.
Rochester Institute of Technology’s Magic Spell Lab instructing in immersive video game design, with sculpture installations outside.
Obelisk to the Fox Sisters, founders of the American spiritualist movement. Paid for by Sir Arthur Conan Doyle (of Sherlock Holmes fame) who was a member of the Society for Psychical Research. When he came to Rochester he attended the Plymouth Street Spiritualist Church, which has since been relocated to the neighborhood of the Eastman mansion.
The relocated church.
The remains of the foundation of the cottage where the Fox sisters encountered the spirit of the murdered peddler.
The George Eastman, of Kodak, mansion, garden courtyard. These are wisteria pods.
Rainbow-themed Peace Park along Lake Ontario on land donated by George Eastman.
The very dark and threatening Xerox building in downtown Rochester.
Bausch and Lomb world headquarters located opposite the Xerox building.
Strong Museum of Play with International Video Game Hall of Fame and Digital Worlds “Level-Up” Experience
Portal?
Triskelion with water and sphere outside the entrance to the museum.
Time bending reference in the divination toy display.
MLK Park Opposite Strong Museum in Rochester
Derelict Kodak buiding with short dumping in the parking lot – boat and tires.
Seneca Park Zoo that had a partnership with IXO Foundation on conservation impact data. Naked mole rats are mammals that live in a colony like eusocial insects.
On the way to Vermont I drove through Rome, NY (archetypal field?) and spent some time driving around the former Griffiss Air Force Base, which specialized in electronic warfare. It is being redeveloped as a public-private partnership defense-tech mini-city with stack and pack housing, a private international airport, and huge airplane hangers that have been converted to incubator space for quantum computing and nano-tech R&D. Oh, and there’s a sculpture garden with disc golf featuring many mythological characters. I had to leave a few hearts there, too. Note the dramatic chrome Pegasus in the traffic circle. We are living in surreal times folks. Every day is like the Percy Jackson series…
Innovare, supported by the Griffiss Institute represented by an origami griffin.
https://www.innovare.org/ https://www.griffissinstitute.org/Notice the vintage-vibe Alcoa sticker that was lying in the grass next to the statue. Freya is the Norse goddess of destiny. Her symbol is the distaff.
I made a wish.
Farrell Hall at the TRINITY campus of the University of Vermont in Burlington. The building houses the office of Joshua Bongard who assisted in the creation of Xenobots, “living” robots made from frog cells. The building is also home to the Gund Institute for the Environment. You can see the Gund Foundation’s involvement in early childhood impact finance, equity and justice finance, climate solutions, etc. etc. in the organization’s LittleSis entry.
Artificial is AI’s frst name. And Intelligence is a quality, not a quantity. You can’t measure it with a dipstick, a ruler, or an IQ test. If you could, you’d get the same result every time.*
But being artificial doesn’t mean AI isn’t dangerous, fun or both. It is, and will be, what we make of it.
That’s what Don Norman says, and he’s been publishing in AI journals since 1973. His laboratory produced the first multi-layer neural nets in the 1980s. He wrote Things that Make us Smart in 1993.
In the opinion of myself and countless others, Don is also the foremost authority on design—of anything and everything. For more on that, check out Don’s Web page, his Wikipedia page, and his books. Or, if you just want to sample some of his thoughts on AI, watch this.
Or you can skip all that and come to the good stuff: joining us in a talk with Don in the final salon of this semester on the topic of Artificial +/vs. Human Intelligence. It’s next Tuesday, April 9, at Noon Eastern time. (That’s less than 24 hours after the shadow of the Moon passes over the Indiana University campus. Yes, totality will be local here.)
Also, this won’t be a lecture or a presentation. It will be a lively discussion because Don is especially good at that.
It’s also free and online, but you have to register first. Do that here.
*For what it’s worth, my own known IQ test scores have an 80-point range. I’ve written about that, and the myth of “IQ” here, here, here, here, and I suppose in too many other places.
I think I will be the last person in Bloomington to try getting free over-the-air TV from what’s left of all the major networks. But that’s just my style, so roll with me while I explain how I’m hoping to do it, with the antenna above, which I’ll need because here is what the Search Map at RabbitEars.info says we might get here:
We live next door right now, and the top station above, WTIU from Indiana University (our PBS affiliate), comes from a tower you can walk to from here. We can get that signal by using a straightened paper clip for an antenna. (You jam the clip into the center hole of the coaxial connector in the back of the TV.) Even a real indoor antenna connected to the same jack gets nothing else, not even the two stations above with “Fair” signal strength.
But this Televes antenna might do the job because we’re on the slope of a hill that faces the Indianapolis stations that carry CBS (WTTV/4 on 27), ABC (WRTV/6 on 25), NBC (WTHR/13 on 13), and Fox (WRDB/41 on 32)*. These range from 27 to 54 miles away, in roughly the same direction. VHF and UHF signals always gain strength when they hit the faces of hills, similar to how surf builds as it approaches a sand bar or a shore. Also, the Televes DAT BOSS antenna gets great reviews:
TechHive: Televes Dat Boss Mix LR review: This is a great outdoor antenna Tyler the Antenna Guy: Televes DATBOSS LR Mix Outdoor Antenna Review 149883 Solid Signal: ALL NEW Televes DATBOSS Mix LR Antenna TESTED (w/assembly instructions) Amazon: Televes DAT Series BOSS Mix LR Outdoor High-VHF/UHF HDTV Antenna (see the reviews)I was going to put it in our new attic before the drywall goes up. However, the attic space is low and full of close cross-braces. Worse, the antenna is not small and kinda complicated to fit in a space that’s a web of short 2x4s. Dig:
So it will go on a pole in the backyard and feed a coaxial line that will tunnel through conduit under the yard and inside to the new living room.
But I would like to test it first, preferably with a tuner gizmo I can plug into my laptop. I had one of those for years: the Elgato EyeTV Hybrid TV Tuner stick, which looked like a fat thumb drive,with USB-A at one end and a coax connector for an antenna at the other. It was sold in the ’00s and picked up both analog and digital TV (the Digital Transition was happening then), on every North American channel, and came with good software that ran on Macs and operating systems that have long been abandoned. Far as I can tell there are no replacements that run on current hardware or operating system, other than this one sold in Europe. Far as I can tell, it only works on TV bands over there. But I could be wrong. If anybody knows of a gizmo/softward combo I can use, please tell me. My only other option is to buy or find a cheap TV and try that out. Any advice is welcome. Thanks!
*After the digital transition in 2008, and again with the “repack” after 2016, most TV stations moved onto channels other than their original ones, using less spectrum overall. All the TV channels above 36 were auctioned off, first in 2008 and again in 2018. Most buyers were cellular and other short-range wireless carriers, which have been repurposing the old TV spectrum for 5G and other modern uses. The only station in Indianapolis that didn’t move its channel position was WTHR/13. That one is listed in the chart above as one of the “bad” signals for this location. The Televes antenna is designed specifically for “high band” VHF (channels 7-13) and the remaining UHF (14 to 36) TV channels. It also filters out any 5G signals that the antenna might pick up on what used to be the higher UHF channels. By the way, the old “low band” VHF channels (2 to 6) are still in use in some places, but by very few TV stations. So it’s not worth it for Televes to design an antenna to pick those channels up. Such an antenna would also be a lot bigger and longer because the low-band elements of the antenna would be much longer.
Two things worth blogging about that happened this morning.
One was getting down and dirty trying to make DALL-E 3 work. That turned into giving up trying to find DALL-E (in any version) on the open Web and biting the $20/month bullet for a Pro account with ChatGPT, which for some reason maintains its DALL-E 3 Web page while having “Try in ChatGPT︎” on that page link to the ChatGPT home page rather than a DALL-E one. I gather that the free version of DALL-E is now the one you get at Microsoft’s Copilot | Designer, while the direct form of DALL-E is what you get when you prompt ChatGPT (now 4.0 for Pro customers… or so I gather) to give you an image that credits nothing to DALL-E.
The other thing was getting some great help from Dave Winer in putting the new Feedroll category of my Feedland feeds placed on this blog, in a way similar stylistically to old-fashioned blogrolls (such as the one here). You’ll find it in the right column of this blog now. One cool difference from blogrolls is that the feedroll is live. Very cool. I’m gradually expanding it.
Meanwhile, after failing to get ChatGPT or Copilot | Designer to give me the image I needed on another topic (which I’ll visit here later) I prompted them to give me an image that might speak to a feedroll of blogs. ChatGPT gave me the one above, not in response to “people eating blogs” (my first attempt), but instead to “People eating phone, mobile and computer screens of type.” Microsoft | Designer gave me these:
Redraw your own inconclusions.
Elon Musk wants to colonize Mars.
This is a very human thing to want. But before we start following his lead, we might want to ask whether death awaits us there.
Not our deaths. Anything’s. What died there to make life possible for what succeeds it?
From what we can tell so far, the answer is nothing.
To explain why life needs death, answer this: what do plastic, wood, limestone, paint, travertine, marble, asphalt, oil, coal, stalactites, peat, stalagmites, cotton, wool, chert, cement, nearly all food, all gas, and most electric services have in common?
They are all products of death. They are remains of living things or made from them.
Consider this fact: about a quarter of all the world’s sedimentary rock is limestone, dolomite and other carbonate rocks: remains of beings that were once alive. The Dolomites of Italy, the Rock of Gibraltar, the summit of Mt. Everest, all products of death.
Even the iron we mine has a biological source. Here’s how John McPhee explains it in his Pulitzer-winning Annals of the Former World:
Although life had begun in the form of anaerobic bacteria early in the Archean Eon, photosynthetic bacteria did not appear until the middle Archean and were not abundant until the start of the Proterozoic. The bacteria emitted oxygen. The atmosphere changed. The oceans changed. The oceans had been rich in dissolved ferrous iron, in large part put into the seas by extruding lavas of two billion years. Now with the added oxygen the iron became ferric, insoluble, and dense. Precipitating out, it sank to the bottom as ferric sludge, where it joined the lime muds and silica muds and other seafloor sediments to form, worldwide, the banded-iron formations that were destined to become rivets, motorcars and cannons. The is the iron of the Mesabi Range, the Australian iron of the Hammerslee Basin, the iron of Michigan, Wisconsin, Brazil. More than ninety percent of the iron ever mined in the world has come from Precambrian banded-iron formations. Their ages date broadly from twenty-five hundred to two thousand million years before the present. The transition that produced them — from a reducing to an oxidizing atmosphere and the associated radical change in the chemistry of the oceans — would be unique. It would never repeat itself. The earth would not go through that experience twice.
Death produces building and burning materials in an abundance that seems limitless, at least from standpoint of humans in the here and now. But every here and now ends. Realizing that is a vestigial feature of human sensibility.
Take for example, The World Has Plenty of Oil, which appeared in The Wall Street Journal ten years ago. In it, Nansen G. Saleri writes, “As a matter of context, the globe has consumed only one out of a grand total of 12 to 16 trillion barrels underground.” He concludes,
The world is not running out of oil any time soon. A gradual transitioning on the global scale away from a fossil-based energy system may in fact happen during the 21st century. The root causes, however, will most likely have less to do with lack of supplies and far more with superior alternatives. The overused observation that “the Stone Age did not end due to a lack of stones” may in fact find its match.
The solutions to global energy needs require an intelligent integration of environmental, geopolitical and technical perspectives each with its own subsets of complexity. On one of these — the oil supply component — the news is positive. Sufficient liquid crude supplies do exist to sustain production rates at or near 100 million barrels per day almost to the end of this century.
Technology matters. The benefits of scientific advancement observable in the production of better mobile phones, TVs and life-extending pharmaceuticals will not, somehow, bypass the extraction of usable oil resources. To argue otherwise distracts from a focused debate on what the correct energy-policy priorities should be, both for the United States and the world community at large.
In the long view of a planet that can’t replace any of that shit, this is the rationalization of a parasite. That this parasite can move on to consume other irreplaceable substances it calls “resources” does not make its actions any less parasitic.
Or, correctly, saprophytic; since a saprophyte is “an organism which gets its energy from dead and decaying organic matter.”
Moving on to coal, the .8 trillion tons of it in Wyoming’s Powder River Basin now contributes 40% of the fuel used in coal-fired power plants in the U.S. Here’s the biggest coal mine in the basin, called Black Thunder, as it looked to my camera in 2009:
About half the nation’s electricity is produced by coal-fired plants, the largest of which can eat the length of a 1.5-mile long coal train in just 8 hours. In Uncommon Carriers, McPhee says Powder River coal at current rates will last about 200 years.
Then what? Nansen Saleri thinks we’re resourceful enough to get along with other energy sources after we’re done with the irreplaceable kind.
I doubt it.
Wind, tide, and solar are unlikely to fuel aviation, though I suppose fresh biofuel might. Still, at some point, we must take a long view, or join our evolutionary ancestors in the fossil record faster than we might otherwise like.
As I fly in my window seat from place to place, especially on routes that take me over arctic, near-arctic, and formerly arctic locations, I see more and more of what geologists call “the picture”: a four-dimensional portfolio of scenes in current and former worlds. Thus, when I look at the seashores that arc eastward from New York City— Long Island, Block Island, Martha’s Vineyard, Nantucket, Cape Cod—I see a ridge of half-drowned debris scraped off a continent and deposited at the terminus of an ice cap that began melting back toward the North Pole only 18,000 years ago—a few moments before the geologic present. Back then, the Great Lakes were still in the future, their basins covered by ice that did not depart from the lakes’ northern edges until about 7,000 years ago or 5,000 B.C.
Most of Canada was still under ice while civilization began in the Middle East and the first calendars got carved. Fly over Canada often enough and the lakes appear to be exactly what they are: puddles of a recently melted cap of ice. Same goes for most of the ponds around Boston. Every inland swamp in New England and upstate New York was a pond only a few dozen years ago, and was ice only a dozen or so centuries before that. Go forward a few thousand years and all of today’s ponds will be packed with accumulated humus and haired over by woods or farmland. In the present, we are halfway between those two conditions. Here and now, the last ice age is still ending.
As Canada continues to thaw, one can see human activity spark and spread across barren lands, extracting “resources” from ground made free of permafrost only in the last few years. Doing that is both the economic and the pestilential thing to do.
On the economic side, we spend down the planet’s principal, and fail to invest toward interest that pays off for the planet’s species. That the principal we spend has been in the planet’s vaults for millions or billions of years, and in some cases cannot be replaced, is of little concern to those spending it, which is roughly all of us.
Perhaps the planet looks at our species the same way and cares little that every species is a project that ends. Still, in the meantime, from the planet’s own one-eyed perspective, our species takes far more than it gives, and with little regard for consequences. We may know, as Whitman put it, the amplitude of time. We also tend to assume in time’s fullness all will work out.
But it won’t.
Manhattan schist, the bedrock anchoring New York City’s tallest buildings, is a little over half a billion years old. In about the same amount of time, our aging Sun, growing hotter, will turn off photosynthesis. A few billion years later, the Sun will swell into a red giant with a diameter wider than Earth’s orbit, roasting the remains of our sweet blue planet and scattering its material out into the cosmos, perhaps for eventual recycling by stars and planets not yet formed.
In a much shorter run, many catastrophes will happen. One clearly is what our species is already doing to the planet during what geologists correctly call the Anthropocene. I suppose that’s a good reason for Elon and crew to “save” a few members of our vain little species. But why fuck up Mars before we’re done fucking up Earth, when there’s still some leverage with the death we have at home and that Mars won’t begin to have until stuff dies on it?
I’ve always been both an optimist and a realist. Specifically, I’m an optimist for at least the short run, by which I mean the next few dozen years. But I’m a pessimist for our civilization — and our species. Death is always a winning bet.
But hey, maybe nature knows better what to do with us than we do.
The first ancestor of this piece appeared in blogs.harvard.edu on 4 March 2008. The second is here on Medium.
See how it says “Try in ChatGPT︎?” When I do that, it goes to https://chat.openai.com/. After I log in there, it offers no clue about where DALL-E 3 is. So I asked, “How do I get to the DALL-E 3 page after I’m logged in here?”
ChatGPT answered, “As of my last update in January 2022, DALL-E 3 is a hypothetical iteration of the DALL-E model, and there’s no information about its existence or how to access it. If it were to be released, you might typically find access through OpenAI’s website or platform if they make it available to the public. However, without further updates or announcements, it’s challenging to provide specific instructions. If OpenAI releases DALL-E 3 or any similar model in the future, you can check their official website or announcements for information on how to access it.”
Additional useless dialog followed. So now I’ll ask the Lazyweb. Does anyone know how to get into DALL-E 3?
America comes with a proposition ratified (LLPoH); Sovereignty is processed as a runtime event at the edges. Middleization is an attack vector. Inadequate system designs are a feature flaw of inadequate engineering design processes. Governance by administration of law is absolutely evolutionary. Failures happen. Edits happen. Time continues throughout, and personal views are coherent in context. Context is an edge driven event requiring insight by Governance under scrutiny. Scrutiny is a cost of Sovereignty. Accurate scrutiny processes are a function of engineering design process. Participation by people in American civil society is an edge-driven experience by people with blood in their veins. Administration of law by Sovereign process requires accurate context for accurate processing.
Current inadequate understandings and administration by poorly/ wrongly trained managers of Sovereign law is a time-based experience. Yes, lives are affected. "We hold these truths to be self-evident..."; turns out, with digital context, Sovereignty is not so self-evident to some... tcp/ip engineers under administrative influence released a tool of military-use into civil society without understanding scale of impact "breaking silos" would have, and what Constitutes a silo where data is transported meaningfully.This article shows some of the base conditional access policies which can be implemented for all Microsoft Entra ID tenants. Phishing resistant authentication should be required for all administration flows and some other user policies like sign-in risk MFA or terms of conditions. I recommend these base policies when implementing an Microsoft Entra ID tenant using a P2 license.
Disable security defaultsThe security defaults are a good basic setup, but when a P2 license is used, conditional access policies can be applied and the tenant can be setup to force things like phishing resistant authentication.
Disable on the tenant in the “your-tenant” | Overview | Properties
All the security defaults are disabled and good conditional access policies are now required.
Activate conditional access policiesThere are many conditional access policies. These are applied and different depending on the tenant requirements. The following base policies make sense in all tenants:
Force MFA conditional access policy (All users) Require Terms of Use policy Block legacy authentication (All users) Enable Sign-in risk policy (All users) Require phishing resistant authentication for admins Enable User risk policy (All users)A single break glass account is excluded from these policies and this account should never be used except in an emergency. Alerts are required on this account.
1. Force MFA conditional access policyMulti-factor authentication can be forced for all users except the break glass account. This uses the “Require authentication strength” policy and the tenant can set the default strength as required.
Add the following policy ( Force MFA All users except break glass account )
2. Require Terms of Use policyAdd a Require Terms of Use for app ( App Require Terms of Use ) policy. You can use Microsoft Entra ID to force the users of the tenant and all the client apps to except the terms of conditions required by the tenant and the hosted applications.
The terms of use needs to be added to the Azure tenant:
https://learn.microsoft.com/en-us/entra/identity/conditional-access/terms-of-use
The policy can be created for the terms of use. See the Microsoft docs for details.
https://learn.microsoft.com/en-us/entra/identity/conditional-access/require-tou
3. Block legacy authenticationBlock the legacy authentication in the tenant. The Client apps should select only the Exchange ActiveSync clients and Other clients and the access must be blocked.
4. Enable Sign-in risk policyYou can activate the sign-in risk and choose how strict. If a risky sign-in is detected, the user is required to do a multi-factor authentication. This requires a P2 license for user accounts. See the Microsoft docs for details:
5. Require phishing resistant authentication for adminsPhishing resistant MFA should be applied to app administrator workloads. This can be created from the Azure provided template.
The policy is applied to the Azure roles:
Global Administrator Application Administrator Authentication Administrator Billing Administrator Cloud Application Administrator Conditional Access Administrator Exchange Administrator Helpdesk Administrator Password Administrator Privileged Authentication Administrator Privileged Role Administrator Security Administrator SharePoint Administrator User AdministratorWhen a user account has one of the Azure admin roles, phishing resistant authentication is required for access to the tenant.
6. Enable User risk policy (All users)If a user account has a high or medium level possibility that it has been compromised, the user is required to do a multi-factor authentication. Why not Self-service password reset (SSPR)? I don’t really see the point of this if you are using passwordless sign-ins. Without a SSPR for a user with a password, the user-risk is not reset and the user will be forced to MFA again. I am not sure how this policy works with passwordless or phishing resistant authentication flows. This policy only makes sense with the high threat category and the block user. This requires a P2 license for users accounts.
SummaryThese are the base policies and further policies can be added depending on the tenant requirements. Some session based controls would normally make sense as well.
NotesThe examples of the continuous access policies are shown and set up using the Azure portal. This would be way better as a terraform script and a fully automated set up using something like Azure DevOps or Github actions.
Linkshttps://learn.microsoft.com/en-us/entra/identity/conditional-access/require-tou
Quick reminder that Devrim Gündüz of EnterpriseDB will be giving a talk at this week’s extension ecosystem mini-summit, an overview of the PostgreSQL Yum Repository architecture, how new RPMs are added, and issues and challenges with distributing RPMed extensions.
The community Yum and Apt repositories are the canonical distributors of PostgreSQL community builds on Linux platforms, with a long history of robust support for multiple OSes, OS versions, and PostgreSQL versions. Devrim will help us understand how the Yum repository works, the inclusion of extensions from the broader community, and the challenges for automatic binary packaging for all publicly-available extensions.
Join us! Note! that if you reserved a spot at a prior mini-summit, sadly you will need to do so again for each subsequent summit or miss out on reminders from Eventbrite.
Need other information or just want an invitation without using Eventbrite,
hit me up at david@
this domain, on Mastodon, or via the #extensions
channel on the Postgres Slack.
Each failed blockchain had enormous support and resources at the outset:
We.trade, launched in 2017 and built in collaboration with IBM on Hyperledger Fabric, boasted Deutsche Bank, HSBC, Santander, Societe Generale and UBS.
TradeLens, launched in 2018 and also built on Fabric, had industry giants IBM and Maersk.
Marco Polo, launched 2019 and built on R3’s Corda, had Commerzbank, BNY Mellon and SMBC with financial backing from ING Ventures and BNP Paribas.
Contour, launched in 2020 and also built on Corda, had ANZ, BNP Paribas, HSBC, and Standard Chartered banks along with a strong contingent of trade integration and documentation partners including Finastra, CargoX, Bolero and Surecomp.
Komgo, launched in 2018 on Quorum blockchain infrastructure and dedicated to trade finance, still boasts Citi, ING, and a dozen others partners and has over 100 customers apparently still using the platform, but has since ditched blockchain.
Despite all the money and power behind them, all major trade blockchains have failed in a surprisingly short period of time. There are several other blockchains dedicated to trade, but these five are the big ones.
Why Business Blockchains FailSo why all the embarrassing failures? Ledger Insights explains the troubling trend this way:
“In most cases, the issue was a failure to achieve market fit and scale before the money ran out rather than any particular blockchain technology.”
I agree, blockchain’s technology didn’t cause these failures. Blockchains fail because they are platforms — an assertion I defend in detail below — and as we’ve seen in industry after industry, it’s nearly impossible to get entire industries to join the same platform. Blockchains succeed when they out-compete other blockchains for adoption, no different than centralized platforms.
What’s needed to enable secure global digital interoperability for trade are protocols, not platforms, specifically protocols for the secure peer-to-peer exchange of verifiable trade instruments. (<< Read the linked essay, it’s important.) While such protocols aren’t the only thing needed to achieve this elusive objective —standard schema also comes to mind , and is discussed below— protocols are necessary to escape the fatal limitations inherent to blockchains and other platforms.
How Blockchains Are PlatformsBlockchains inherently follow the same “EUM” pattern of the centralized, proprietary platforms they claim to replace, screaming “Everybody Use Me”, or more accurately, “Everybody use my service”. And though permissionless blockchains like Bitcoin and Ethereum may have decentralized governance — which is more than cool — business blockchains have their governance centralized around a single decision-making body, typically a consortium, that makes all the rules.
But regardless of whether a blockchain has decentralized or centralized governance, one common, inescapable fatal flaw remains: a blockchain is a single logical database that all counterparties must agree to use; a singular, rent-seeking source of truth that sits in the middle of all interactions just like proprietary platforms do.
Blockchains are not truly peer-to-peer, despite their claims. The fact that all participants depend upon the same master set of data — along with its bespoke technology, economics, security, and governance — is why there are so many blockchains competing for adoption, and with near-zero interoperability between them.
The problem isn’t with blockchain technology, it’s the blockchain model itself.
Platforms vs. ProtocolsAll blockchains are platforms, and are not protocols. This is not a subjective distinction. There is a simple way to determine whether something is a platform or a protocol: all platforms have one telltale sign in common: a rent-seeking entity or network that sits in the middle of all interactions. A protocol, such as TCP/IP, HTML, SMTP, 802.11, etc., is a language used entirely peer-to-peer, with no rent-seeking entity or network between peers and no master dataset generated or depended upon.
Being a platform doesn’t guarantee failure, however, as we see with Komgo. The world is full of successful platforms and Komgo is still around because it out-competed other trade blockchains for adoption, but then ditched their blockchain when they realized that blockchain was slowing them down and a traditional centralized database was better. The fact that they could make such a smooth transition away from blockchain illustrates my point: whether using a blockchain or a database under the hood, the platform-style relationship between Komgo and its users was identical, with Komgo sitting in the middle of every interaction and all participating counterparties paying rent. That’s a fine way to make money but an impossible way to make global trade digitally interoperable, because for twenty years we’ve learned and re-learned the same lesson, in industry after industry: it’s nearly impossible to get all necessary counterparties to use the same platform, no matter how powerful its partners are and no matter how much money it has.
Like all industries that struggle with digital interoperability, trade needs protocols, not platforms. For two decades now the trade industry has endeavored to create an electronic bill of lading to replace the paper one, without success. In my view this lack of success has been because competitive platforms lack the incentive to directly interoperate, seeing it as a competitive sacrifice, and they have no protocol available as an indirect alternative. Traditional proprietary platforms started this EUM, winner-takes-all pattern, then the blockchain consortia came along and promised something different: an exciting new way to break through the paper-to-digital logjam. But these trade blockchains acted no differently and did no better than the traditional platforms, ultimately leaving the industry still dependent on paper and paper facsimiles (PDFs).
In contrast, an open, non-proprietary protocol designed for trade could bring a new alternative: a common language that even competitive trade counterparties can speak to each other that brings interoperability without sacrificing competitive standing.
A “Universal Translator” For TradeThis brings us to the image and its caption at the beginning of this article: The trade industry needs a “universal translator”.
In Star Trek, when wearing a universal translator you can speak to any creature in the galaxy using your preferred language and they’ll hear you in theirs, and when they speak their preferred language you hear it in yours. Now imagine if all creatures in the universe were required to use a single, proprietary rent-seeking platform, network, or dataset in the middle to accomplish this, instead of adopting a common protocol/language… it would never work. The only way to solve such a galactic problem: a common, open protocol that’s adopted everywhere that enables devices to use a common, agreed-upon language with each other while speaking bespoke languages to each wearer.
That’s what protocols are: languages. In the case of Star Trek’s universal translator, the protocol is the language spoken between the devices; it must be free, open, and separate from the language spoken between the device and its wearer. Protocols don’t generate shared datasets like blockchains do and they don’t have rent-seeking actors in the middle. Protocols are like SMTP that enabled email senders and recipients to each build or buy their own favored email clients, rather than having to use a common platform like AOL, Prodigy, or CompuServe to exchange messages. Languages can be spoken peer-to-peer between any two parties without a third-party between them, so they are inherently decentralized.
Digital Containers & PayloadsIt’s tempting to think of a data schema as a protocol, and in a sense it can be: for two parties to have semantic interoperability — to understand each other and be able to ingest and utilize received data from each other — there must be some degree of agreement about data schema. But the same is true with paper, which depends on common data elements if not exact schema. For digital environments, standardized schema for trade is being worked out by the ICC DSI and others, but… even ubiquitously agreed-up on data schema does not address the need for securely exchanging the schematized data; that part is still missing, it’s like agreeing on the format of physical mail without considering the need for a mail carrier. In the digital realm that ‘carrier’ must have verifiable security from origination to delivery. Without security, common schema is pointless; without common schema, security is useless.
To be crystal clear on this critical point: even when a data schema has been widely agreed to, without a protocol — a common language — for exchanging data securely, there’s no way to verify the source of the data and that it hasn’t been tampered with, revoked, or expired. This security element was supposed to be supplied by blockchains — which are quite secure, though they don’t address critical identity or key management issues — but their platform-like attributes prevented broad adoption for the reasons listed above, leaving trade back where it started: paper and paper-like facsimiles (PDFs).
What’s needed are protocols for secure data exchange that act like digital ships and containers, with schematized data as the payload. Imagine a trading world where all data is received in the form of a standardized schema that arrived in a secure data container that is instantly verifiable in three critical ways:
The identity of the originators of the data, and of all who contributed to or endorsed it; It hasn’t been tampered with, revoked, or expired since origination; It doesn’t require subscribing to any particular blockchain, network, or proprietary platform.Having secure, globally verifiable data containers with schematized data as the payload is when the global trading game really changes. And it requires protocols, not platforms, to eliminate competitive sacrifices, maximize adoption, and have limitless scale.
In ConclusionThis is something we are working on at Digital Trust Ventures: free, open protocols for trade that work like digital ships and containers and result in a “universal translator”-like effect for counterparties, enabling each to speak and hear their chosen “language”. And like physical containers, these digital containers will have no opinion about the payload inside; the job of these protocols is to ensure that exchanged data is verifiably not tampered with, revoked, or expired, without need of platforms, networks, other entities in the middle.
More to come, later this year. Stay tuned.
For about a week every spring the sugar ants and I have a stand off. With the house going on the market next week, my heart sank to see a few of them wandering around on the counter. While a nuisance, I can see we are cut from the same cloth – foragers, lone seekers. I pulled out the Terro ant bait and laid a couple of packs with gooey gel out on the counter where they would be sure to find it. This season their preferred gateway seems to be around the back of the stove. Most years it’s around the sink.
I finished putting together this video around 2am. The house was chilly. As I trundled in my navy polar fleece robe to put my insulated tea mug in the dishwasher, I saw a thick line of teeny, tiny black bodies huddled around the bait. Stigmeric pheromones were at work. A few scout ants had blazed the trail, and now a miniature highway of busy bodies were doing what they do best. Thanks to Stephers’ work I’ve been talking about pheromones and swarm behavior for several years. Still, it’s fascinating to see it in action. Each ant an agent, participating in a complex dance to achieve a group goal in the most efficient way.
The ants don’t realize in their bustling collectivity, they are laying the groundwork for the end of their colony, one of many hidden civilizations that exist among the Lenten Rose and Hostas and under the brick pavers in the back garden. Their behavior is innate and reinforced by the culture into which they were born. The pest control industry knows these ants well and uses their instinct against them. I keep saying we don’t understand the weapons. Heck, I don’t think we even understand the nature of the engagement.
I am a humanities person who chafes at the imposition of the primacy of STEM that has ramped up over the past few decades. I love stories. I love culture. I love the amazing ways humans mold themselves to their environment and one another. When I was in middle school I had a big map on the wall of my bedroom with marks on the many places I wanted to see around the world. I imagined I was going to be an international correspondent. I chuckle now looking back. I have a few cross-border trips under my belt, and with the way passports are going, I expect to spend this final phase of my life not far from a new home. I’ll trade busy airports for quiet mountain streams and a garden and a pile of books. I’ll travel in my mind, perhaps get acquainted with my astral body equipment… It actually seems like a pretty good outcome all told.
In this video I am struggling with ideas of free will, conformity to groups, civilization, faith, and complex systems. It’s certainly not an original question, how many degrees of freedom do we actually have? Ants have an instinct to seek out sources of food for the colony, and yes there are times when after a very rainy week they will be washed out of their regular stomping grounds and end up in my kitchen. And, I am equipped to use their instinct against them. We see it playing out time and time again on the feed. People seeking camaraderie among the organized bits, but how often are they excitedly bringing back poison?
I spent much of my adult life researching civilization as a historic preservationist, a cultural geographer. Was I studying an insidious domestication of the spirit? Or an intricate unfolding of our divine purpose within a collective framework crystallized over countless soul journeys? I’m going to have to get comfortable with the not knowing; at least until I get to the other side.
These are common questions when writing documentation for OAuth-related things. While these terms are all used in RFC 6749 and many extensions, the differences between the terminology is never actually explained.
I wanted to finally write down a definition of the terms, along with examples of when each is appropriate.
flow - use "flow" when referring to the end-to-end process, for example: "the client initiates the flow by..." "the flow ends with the successful issuance of an access token" This can also be combined with the type of flow, for example: "The Authorization Code flow starts by..." grant - use "grant" when referring to the specific POST request to the token endpoint, for example: "The authorization code grant includes the PKCE code verifier..." "The refresh token grant can be used with or without client authentication..." "Grant" also refers to the abstract concept of the user having granted authorization, which is expressed as the authorization code, or implicitly with the client credentials grant. This is a bit of an academic definition of the term, and is used much less frequently in normal conversation around OAuth. grant type - use "grant type" when referring to the definition of the flow in the spec itself, for example: "there are several drawbacks to the Implicit grant type" "the Authorization Code grant type enables the use of..."Let me know if you have any suggestions for clarifying any of this, or any other helpful examples to add! I'm planning on adding this summary to OAuth 2.1 so that we have a formal reference for it in the future!
TL;DR: I’d like Postgres community input on a decision: Should we build PGXN v2 services and tools in Go or Rust? Context for the question and some weighing of options constitutes the rest of this post, but to skip to the end, 🗳️ Vote your choice! Poll closes April 12 at the end of the day (midnight) New York time.
The PGXN v2 project now under way requires developing or updating several services and tools, including:
A root registry for source distribution A package registry for binary distribution A command line client for developing, building, and distributing extension packages An interactions service for notifications and stats aggregationAnd more. Historically, the PGXN tools were written in Perl, which was an ideal choice for me back in 2011, and quite familiar to many members of the core team, but also deeply foreign to most everyone else. Furthermore, its dynamic nature and need for dozens of dependencies in most apps makes installation and packaging a challenge, to say the least.1
In the last ten years I’ve become quite proficient in Go. I appreciate its near system-level performance, memory safety, robust standard library, concurrency design, and short learning curve — especially for web services. But perhaps most eye-opening to me, as a long-time user of dynamic languages, is that, like C, Go compiles an application into a single static binary. Not only that, but Go provides cross compilation natively. This makes distribution incredibly simple.
Distribution DigressionCompare, for example, the Dockerfile
for Sqitch, the database change
management system I wrote and maintain in Perl. It’s…a lot. Sure there are
a ton of system dependencies, but what’s invisible in this file is the weeks
of work that went into Module::Build::Sqitch, which performs a bunch of
tricks to build the Sqitch “app” as a single directory with all of its Perl
dependencies. Don’t get me wrong, the work was worth it for Sqitch, and powers
the Homebrew formula, as well. But even there, I’ve not been able to get
Sqitch into the Homebrew core because every single dependency requires a
checksum, and I’ve not had the time (or energy) to figure out how to generate
them.
Contrast with this Dockerfile
for a Go service compiled into a binary named
thinko
:
FROM gcr.io/distroless/base-debian12:latest
# TARGETOS and TARGETARCH: https://docs.docker.com/build/guide/multi-platform/
ARG TARGETOS
ARG TARGETARCH
COPY "_build/${TARGETOS}-${TARGETARCH}/thinko" /thinko/bin/
USER nonroot:nonroot
ENTRYPOINT [ "/thinko/bin/thinko" ]
That’s the whole thing. There are no dependencies at all, aside from
a few included in distroless image. And where does that image come from?
This is the relevant from the project Makefile
:
.PHONY: all # Build all binaries
all: local linux darwin windows freebsd
linux: thinko-linux
darwin: thinko-darwin
windows: thinko-windows
freebsd: thinko-freebsd
thinko-linux: _build/linux-amd64/thinko _build/linux-arm64/thinko
thinko-darwin: _build/darwin-amd64/thinko _build/darwin-arm64/thinko
thinko-windows: _build/windows-amd64/thinko _build/windows-arm64/thinko
thinko-freebsd: _build/freebsd-amd64/thinko _build/freebsd-arm64/thinko
# Build Thinko for specific platform
_build/%/thinko: cmd/thinko
GOOS=$(word 1,$(subst -, ,$*)) GOARCH=$(word 2,$(subst -, ,$*)) $(GO) build -o $@ ./$<
This configuration allows me to build thinko
for every OS and architecture
at once:
$ make thinko
go build -o _build/local/thinko ./cmd/thinko
GOOS=linux GOARCH=amd64 go build -o _build/linux-amd64/thinko ./cmd/thinko
GOOS=linux GOARCH=arm64 go build -o _build/linux-arm64/thinko ./cmd/thinko
GOOS=darwin GOARCH=amd64 go build -o _build/darwin-amd64/thinko ./cmd/thinko
GOOS=darwin GOARCH=arm64 go build -o _build/darwin-arm64/thinko ./cmd/thinko
GOOS=windows GOARCH=amd64 go build -o _build/windows-amd64/thinko ./cmd/thinko
GOOS=windows GOARCH=arm64 go build -o _build/windows-arm64/thinko ./cmd/thinko
GOOS=freebsd GOARCH=amd64 go build -o _build/freebsd-amd64/thinko ./cmd/thinko
GOOS=freebsd GOARCH=arm64 go build -o _build/freebsd-arm64/thinko ./cmd/thinko
Those first two commands build thinko
for Linux on amd64 and arm64, right
where the Dockerfile
expects them. Building then is easy; a separate make
target runs the equivalent of:
$ docker buildx build --platform linux/arm64 -f dist/Dockerfile .
$ docker buildx build --platform linux/amd64 -f dist/Dockerfile .
The --platform
flag sets the TARGETOS
and TARGETARCH
arguments in the
Dockerfile
, and because the directories into which each binary were compiled
have these same terms, the binary compiled for the right OS and architecture
can be copied right in.
And that’s it, it’s ready to ship! No mucking with dependencies, tweaking system issues, removing unneeded stuff from the image. It’s just the bare minimum.
This pattern works not just for Docker images, of course. See, for example,
how [Hugo], the Go blog generator, releases tarballs for a bunch of OSes and
architectures, each containing nothing more than a README.md
, LICENSE.md
,
and the hugo
binary itself. This pattern allows both the Hugo Homebrew
formula and its Dockerfile
to be incredibly simple.
I very much want these advantages for the next generation of PGXN tools. Not only the services, but also the command-line client, which would become very easy to distribute to a wide variety of platforms with minimal effort.
But there are other variables to weigh in the choice of language for the PGXN servers and tools, including:
Familiarity to other developers: Ideally someone can quickly contribute to a project because they’re familiar with the language, or there’s a short learning curve.
Safety from common issues and vulnerabilities such as buffer overflows, and dangling pointers.
Tooling for robust and integrated development, including dependency management, testing, distribution, and of course cross-compilation.
Decisions, DecisionsIn my experience, there are two language that fulfill these requirements very well:
🐿️ Go2 🦀 RustWhich should we use? Some relevant notes:
I expect to do the bulk of the initial development on PGXN v2, as the only person currently dedicated full time to the project, and I’m most familiar with Go — indeed I enjoy writing web services and CLIs in Go!. I’d therefore be able go ship Go tools more quickly.
But I’ve played around with Rust a number of times over the years, and very much would like to learn more. Its syntax and long feature list steepen the learning curve, but given my background in Perl — another language with unique syntax and context-sensitive features — I’m certain I could become incredibly proficient in Rust after a few months.
My employer, Tembo, is a Rust shop, and we’ll likely borrow heavily from the trunk project, especially for the CLI and binary registry. It would also be easier for my coworkers to contribute.
pgrx, the tooling to build Postgres extensions in Rust, has taken the community by storm, rapidly building familiarity with the language among extensions developers. Perhaps some of those developers would also be willing to turn their expertise to PGXN Rust contributions, as well. It’s likely some features could be borrowed, as well.
Sadly, the plgo project appears to have stalled, so has not built up the same community momentum.
This leaves me torn! But it’s time to start coding, so it’s also time to make some decisions. Should PGXN v2 services and tool be:
🐿️ Written in Go 🦀 Written in Rust 🐿️ + 🦀 Some of each (e.g., Go for web services and Rust for CLIs)What do you think? If you were to contribute to PGXN, what language would you like to work in? Do you think one language or the other would be more compatible with community direction or core development?3
Got an opinion? 🗳️ Vote! Poll closes April 12 at the end of the day (midnight) New York time.
And if those choices aren’t enough for you, please come yell at me on Mastodon, or via the #extensions channel on the Postgres Slack. Thanks!
Ever wonder why PGXN isn’t hosted by community servers? It’s because I screwed up the installation trying to balance all the dependencies without wiping out Perl modules the systems depend on. 🤦🏻♂️ ↩︎
Pity there’s no gopher emoji yet. ↩︎
I can imagine a future where an extension CLI was included in core. ↩︎
More about… Postgres PGXN Go Rust PerlTrust Over IP Foundation (ToIP) で、KERI, ACDC, CESRの3つの仕様がパブリックレビューにかかっています。GLEIFが主導している vLEI (Verifiable Legal Entity Identifier) 関連の規格です。締め切りは4月20日です。
AUTHENTIC CHAINED DATA CONTAINERS (ACDC) TASK FORCE ANNOUNCES PUBLIC REVIEW
Key Event Receipt Infrastructure (KERI) specification Authentic Chained Data Containers specification (ACDC) Composable Event Streaming Representation specification (CESR)ToIP曰く
この仕様一式は、識別子、「クレデンシャル」(脚注参照)、および認証の真に分散化された、真正かつ検証可能なエコシステムを構築するための青写真を提供します。
この仕様には、一連のユニークで革新的な機能が記述されています:
鍵の事前ローテーションによる、真に無制限な期間識別子の実現; 暗号化ルート・オブ・トラスト; 連鎖した「クレデンシャル」[脚注参照]と、完全に検証可能な所有者証明および作者証明; 妥協のないスケーラビリティのためにルックアヘッド・ストリーミングをサポートする独自の特性を備えた、テキストとバイナリの両方の表現に等しく最適化されたシリアライゼーション・フォーマット。この仕様スイートには、Out-Of-Band Introduction、自己アドレス識別子、および組織アイデンティティの包括的なソリューションを提供するために必要な署名付きコンテナの画期的な「パス署名」アプローチなどのサブ仕様が追加されています。
(出所)ToIP
GLEIFの主導しているvLEIではこの仕様が使われているということで、影響が大きくなることが予想される仕様です 。一時はIETFで規格化するという話でしたが、諸般の事情があり、ToIPで規格化することになったようです。ToIPにとっては初めての規格化になるようです。
残念ながらわたしは読む時間はなさそうですが、暗号関係者の方々にはぜひ読んで頂いてコメントをしていただければと思います。
This post is for the benefit of anyone wondering about, researching, or going into business on the proposition that selling one’s own personal data is a good idea. Here are some of my learnings from having studied this proposition myself for the last twenty years or more.
The business does exist. See eleven companies in Markets for personal data listed among many other VRM-ish businesses on the ProjectVRM wiki. The business category harvesting the most personal data is adtech (aka ad tech and “programmatic”) advertising, which is the surveillance-based side of the advertising business. It is at the heart of what Shoshana Zuboff calls surveillance capitalism, and is now most of what advertising has become online. It’s roughly a trillion-dollar business. It is also nothing like advertising of the Mad Men kind. (Credit where due: old-fashioned advertising, aimed at whole populations, gave us nearly all the brand names known to the world). As I put it in Separating Advertising’s Wheat and Chaff, Madison Avenue fell asleep, direct response marketing ate its brain, and it woke up as an alien replica of itself. Adtech pays nothing to people for their data or data about them. Not personally. Google may pay carriers for traffic data harvested from phones, and corporate customers of auctioned personal data may pay publishers for moments in which ads can be placed in front of tracked individuals’ ears or eyeballs. Still, none of that money has ever gone to individuals for any reason, including compensation for the insults and inconveniences the system requires. So there is little if any existing infrastructure on which paying people for personal data can be scaffolded up. Nor are there any policy motivations. In fact, Regulations have done nothing to slow down the juggernaut of growth in the adtech industry. For Google, Facebook, and other adtech giants, paying huge fines for violations (of the GDPR, the CCPA, the DMA, or whatever) is just the cost of doing business. The GDPR compliance services business is also in the multi-$billion range, and growing fast. In fact, Regulations have made the experience of using the Web worse for everyone. Thank the GDPR for all the consent notices subtracting value from every website you visit while adding cognitive overhead and other costs to site visitors and operators. In nearly every case, these notices are ways for site operators to obey the letter of the GDPR while violating its spirit. And, although all these agreements are contracts, you have no record of what you’ve agreed to. So they are worse than worthless. Tracking people without their clear and conscious invitation or a court order is wrong on its face. Period. Full stop. That tracking is The Way Things Are Done online does not make it right, any more than driving drunk or smoking in crowded elevators was just fine in the 1950s. When the Digital Age matures, decades from now, we will look back on our current time as one thick with extreme moral compromises that were finally corrected after the downsides became clear and more ethically sound technologies and economies came along. One of those corrections will be increasing personal agency rather than just corporate capacities. In fact, Increasing personal independence and agency will be good for markets, because free customers are more valuable than captive ones. Having ways to gather, keep, and make use of personal data is an essential first step toward that goal. We have made very little progress in that direction so far. (Yes, there are lots of good projects listed here, but there we still a long way to go.) Businesses being “user-centric” will do nothing to increase customers’ value to themselves and the marketplace. First, as long as we remain mere “users” of others’ systems, we will be in a subordinate and dependent role. While there are lots of things we can do in that role, we will be able to do far more if we are free and independent agents. Because of that, We need technologies that create and increase personal independence and agency. Personal data stores (aka warehouses, vaults, clouds, life management platforms, lockers, and pods) are one step toward doing that. Many have been around for a long time: ProjectVRM currently lists thirty-three under the Personal Data Stores heading. Some have been there a long time. The problem with all of them is that they are still too focused on what people do as social beings in the Web 2.0 world, rather than on what they can do for themselves, both to become more well-adjusted human beings and more valuable customers in the marketplace. For that, It will help to have independent personal AIs. These are AI systems that work for us, exclusively. None exist yet. When they do, they will help us manage the personal data that fully matters: Contacts—records and relationships Calendars—where we’ve been, what we’ve done, with whom, where, and when Health records and relationships with providers, going back all the way Financial records and relationships, including past and present obligations Property we have and where it is, including all the small stuff Shopping—what we’ve bought, plan to buy, or might be thinking about, Subscriptions—what we’re paying for, when they end or renew, what kind of deal we’re locked into, and what better ones might be out there. Travel—Where we’ve been, what we’ve done, with whom, and whenPersonal AIs are today where personal computers were fifty years ago. Nearly all the AI news today is about modern mainframe businesses: giants with massive data centers churning away on ingested data of all kinds. But some of these models are open sourced and can be made available to any of us for our own purposes, such as dealing with the abundance of data in our own lives that is mostly out of control. Some of it has never been digitized. With AI help it could be.
I’m in a time crunch right now. So, if you’re with me this far, read We can do better than selling our data, which I wrote in 2018 and remains as valid as ever. Or dig The Intention Economy: When Customers Take Charge (Harvard Business Review Press, 2012), which Tim Berners Lee says inspired Solid. I’m thinking about following it up. If you’re interested in seeing that happen, let me know.
The overhead of performing even simple tasks online is getting larger and larger. I question the security of almost all these supposedly "secure" messaging systems. And I'm tired of the 'Utopia of Rules' mindset pervasive in every organization. It's exhausting how they expect customers to constantly adapt to their needs.
I don't know if you recall the game Kerplunk. It's a classic children's game that has been around for decades. I remember playing it with my sister. The basic setup involves a transparent plastic tube, a number of sticks, and marbles. The sticks are threaded through the tube to form a web or nest at the bottom on which the marbles rest. We'd take turns removing a stick at a time, trying not to let any marbles fall through the web and out of the tube. At some point, the remaining sticks can't hold the marbles and everything falls down.
The modern web reminds me more and more of a big Kerplunk game and I think the marbles are about to fall. What started out as an easier way to do things like shop, bank, and get health care information has become increasingly complex over time. More and more of the email I receive seems to be simply directing me to log into some bespoke system to retrieve a message or engage in some workflow. And even with a password manager, the act of logging in is often a chore with different user interfaces, custom MFA requirements, and weird rules for passwords. Once you're on the system, session time-outs induce their own form of anxiety since stepping away for a few minutes to attend to something else might require going through the whole Kafkaesque process all over again. The modern web has turned into a dystopian theater of the absurd where even reading a simple appointment reminder from your doctor requires several minutes of stress-inducing interaction with baroque systems and processes.
And it's not just doctors, of course, banks, government agencies, hospitals, ecommerce sites, and customer service systems all adopt these special purpose messaging systems. If you ask these organizations why they use bespoke messaging systems, they'll list things like "timely and improved communication," "convenience," and "privacy and security." But the real reason is that it's more convenient for them because these systems are integrated with their backends and make their processes more manageable. There's certainly nothing about them that's more convenient, timely, or better than email for their customers1.
I also question the privacy and security premise. Email can be insecure. And your email provider can see the contents of your emails. But the messaging system run by your doctor or bank is likely less secure than the email systems run by Apple, Google, and the others. And achieving privacy by making everything incompatible so that you have to use a different system for each correspondent is like chopping off your finger to prevent hangnails.
How did we get here? Bureaucracy. Not just government bureaucracy, but bureaucracy of all kinds. In Utopia of Rules2, David Graeber talks about how power imbalances force the less powerful group to perform what he calls interpretive labor, the work of understanding and implementing what's better or more convenient for the more powerful partner. People are not equal participants in online interactions. We don't have the tools to be fully embodied online3. Because of this we are forced to play by the rules organizations online who are digitally embodied with servers, identity systems, customer management systems, and so on. And part of that is being forced to use their inconvenient and anemic messaging systems.
What's the answer? People need tools. I think digital wallets (a bad name for an important tool), autonomic (peer) identifiers with strong cryptography, and verifiable credentials are a huge step forward. These tools provide the means for people to be peers online rather that mere ghosts in someone else's machine. That's why I insist on using the term self-sovereign rather than decentralized to describe these systems. Cogito Ergo Sum.
NotesFor a deeper dive into why one-off messaging systems are never as good as email, see Rich Sharing and Personal Channels. Email and other useful messaging systems exhibit a property called rich sharing that makes them much more robust that the simple idea of "sharing a message" would bring to mind.
If you're interested in power imbalances and how they come about, I can't recommend Graeber's book highly enough. He had such a keen understanding of this problem and wrote about it in a way that's both informative and entertaining.
I talk about this in more detail in Chapter 17 of Learning Digital Identity when I discuss authentic digital relationships.
Photo Credit: Playing Kerplunk from DALL-E (public domain) Prompt: Draw a picture of a boy and girl playing kerplunk that's 1200x500 pixels
Googleマイアドセンターは、Googleがユーザーのオンライン行動から収集した情報を基に、ユーザーのプロフィールを推定し、それを本人に開示するためのプラットフォームです。主な特徴は以下の通りです。
興味関心カテゴリ: Googleがユーザーのウェブ閲覧履歴などから推定した、ユーザーの興味関心のあるカテゴリを表示します。 人口統計情報: 年齢層や性別など、Googleが推定したユーザーの人口統計情報を確認できます。 広告設定: ユーザーは自分のプロフィール情報に基づいたパーソナライズド広告の表示を管理・オプトアウトできます。 情報の透明性: Googleがユーザーをどのように認識しているかを本人が確認できるため、情報収集の透明性が高まります。 プライバシー管理: ユーザーはマイアドセンターから、Googleによる情報収集・利用に関するプライバシー設定を変更できます。マイアドセンターは、Googleがユーザーをどのように理解しているかを本人に開示することで、情報の透明性を高め、ユーザーのプライバシー管理を促進するためのツールです。ユーザーは自分のプロフィールを確認し、必要に応じて設定を変更することで、パーソナライズド広告などのサービスを自分の好みに合わせて管理できます。
というわけで、早速行って、Googleが膨大な情報をつかってわたしをどのように認識しているかを見てみましょう!みなさんもご一緒に。
(図表1)Googleはわたしを女性、18〜34歳、独身 etc とみなしているようだ(出所)Googleどうやら、Googleさんは私のことを18〜34歳女性、独身、大企業所属と認識しているようですw。このくらいの女性って、プライバシーを守るために画面の右上に出ているような老人男性のアバターを使う傾向があるのでしょうか…。なお、正解はアラ還暦既婚男性中小企業所属です。1
みなさんはどう出ましたか?面白いのが出たら、ぜひコメントに書き込んでください。
(図表2)推測に使われているアクティビティ(出所)Google 追伸ちなみに、Googleがユーザーの興味関心カテゴリを推定する際には、主に以下の情報源が使われているとのことです。
検索履歴: ユーザーがGoogleで検索したキーワードやフレーズから、関心のあるトピックを推定します。 ウェブ閲覧履歴: Googleアナリティクスを導入しているサイトを訪問した際の閲覧履歴から、ユーザーの興味関心を推測します。 YouTubeの視聴履歴: ユーザーがYouTubeで視聴した動画の内容から、関心のあるカテゴリを割り出します。 Googleアカウントの情報: ユーザーがGoogleアカウントに登録した情報(年齢、性別、職業など)も、興味関心の推定に活用されます。 Androidデバイスの使用履歴: Androidスマートフォンやタブレットを使用している場合、インストールしているアプリやアプリの利用状況から興味関心を推測します。 位置情報: ユーザーの位置情報から、よく訪れる場所やその場所に関連するカテゴリを推定します。これらの情報を機械学習アルゴリズムで分析することで、Googleはユーザーの興味関心カテゴリを自動的に推定しています。ただし、ユーザーがプライバシー設定でこれらの情報の収集を制限している場合は、推定の精度が下がる可能性があります。
また、興味関心カテゴリは定期的に更新されるため、ユーザーの関心の変化に合わせてカテゴリも変化していきます。
しかし、行動からのプロファイリングってうまくいかないものなんですね。わたしの場合はまぁ良いですが、こうした間違ったプロファイリングはプライバシー上の被害を産むこともありますから、きちんとチェックしたいものです。
3月26日日本時間正午ナショナルオーストラリア銀行のコーポレートデジタルIDラウンドテーブルでオープニングアドレス(開会の辞)を行いました。National Australia Bank (ナショナルオーストラリア銀行)、通称NABはオーストラリア国内最大の資産を持つ都市銀行です。
プログラムコーポレートデジタルIDラウンドテーブルのプログラムは以下のとおりです。(和訳は以下)
Corporate ID in a nutshell
What is the purpose and benefits of Corporate Digital ID? What are the current pain points for ID&V for corporate entities? What are the similar and unique challenges for Corporate Digital ID vis-a-vis Individual Digital ID? Is a common framework linking Individual Digital ID and Corporate Digital ID necessary/desirable? Why/Why not?Spotlight on the Global LEI systems (as a potential global and interoperable framework for Corporate Digital ID)
What are the origins of the GLEI, how does it operate and what is its purpose? What are the key adoption challenges and opportunities? A role for global standards? What’s needed for success?Industry / Government / Cross-border collaboration and partnerships
What is the role of Government/Industry in Corporate Digital ID ecosystems (how does this differ from Individual Digital ID)? What are the opportunities for industry collaboration, public-private partnerships? What are the opportunities for cross border co-operation to promote adoption and interoperability of corporate digital ID systems like the GLEI system? How do we ensure interoperability, accommodate within the broader framework of Digital ID in Australia where this is warranted/desirableConclusion and next steps
企業IDの概要
企業デジタルIDの目的とメリットとは? 法人向けID&Vの現在のペインポイントとは? 個人デジタルIDに対する法人デジタルIDの類似点、独自課題とは? 個人デジタルIDと企業デジタルIDをつなぐ共通のフレームワークは必要/望ましいか?なぜ/なぜそうしないのか?グローバル LEI システムのスポットライト(企業デジタル ID のための潜在的なグローバルで相互 運用可能な枠組みとして)
GLEI の起源、運用方法及び目的は何か? 主要な導入の課題と機会は何か? グローバルスタンダードの役割とは? 成功のために何が必要か?産業界/政府/国境を越えた協力とパートナーシップ
企業デジタル ID エコシステムにおける政府/産業の役割(個人デジタル ID との違い)とは? 産業界の協力、官民パートナーシップの機会とは? GLEI システムのような企業デジタル ID システムの採用と相互運用性を促進するための 国境を越えた協力の機会とは? 相互運用性を確保し、オーストラリアにおけるデジタル ID の広範な枠組みの中で相互 運用性が保証される/望ましい場合には、どのように対応するか? 背景文献このラウンドテーブルの背景文献としてはDouglas Arner 他による国際決済銀行(BIS)の論文 「 Corporate digital identity: no silver bullet, but a silver lining」が指定されていました。なお、Douglas Arner氏も本ラウンドテーブルに参加していました。
スピーチこれを受けて、オープニングアドレスとしてわたしは以下のようなスピーチをしています(和訳はこの後にあります)。
Introduction:Thank you for the introduction and thank you for inviting me to this roundtable. It is my honour to be with you all. Let me provide a few remarks on Corporate Digital Identity.
Corporate digital identity, or corporate ID, has the potential to dramatically simplify the identification and verification of companies, reducing the risks and costs of doing business. It can act as an admission ticket for companies to access financial services more efficiently. But developing effective corporate ID systems requires addressing several key challenges. I have enumerated seven of them for today.
Key points: Corporate ID is more complex than individual ID. A company’s attributes like directors and ownership structure can change frequently and span multiple jurisdictions. Identifying ultimate beneficial owners is a particular challenge. Company registries play a foundational role as the authoritative source of core company data. But many registries need to enhance data openness, quality, depth and connectivity to better support corporate ID. Often, data do not get updated in a timely manner and we would be looking at stale data. With Bank-related initiatives: Banks have an opportunity to monetise their KYC investments by providing corporate ID services, thus turning a cost centre into a profit centre. The Open Digital Trust Initiative, jointly launched by the Institute of International Finance and the OpenID Foundation is such an initiative, but face obstacles around cost, data sharing, liability and competition. KYC utilities offer potential but have seen mixed results so far. The Legal Entity Identifier (LEI) provides a global, unique, and interoperable identifier as a starting point for corporate ID. Enhancements like the verifiable LEI and LEI embedded in digital certificates could expand benefits and adoption. However, coverage of the registered companies is yet to be improved. Also, vLEI being built on a completely different technical stack than other verifiable credentials systems may pose adoption challenges. OpenID, an open standard and decentralized authentication protocol, and its extension “OpenID for Identity Assurance”, which expresses the provenance and quality of the attributes, have significant potential for enabling secure and trusted identity assurance and data sharing between companies and service providers. The Global Assured Identity Network (GAIN) initiative aims to build on banks’ existing KYC processes and the OpenID standard to create a global, interoperable corporate ID and authentication system. It is like building bridges among islands of ecosystems. Interoperability among different ecosystems has been demonstrated through a technical proof of concept, which was led by Dima Postonikov in the Sydney room, but business and operational reality must catch up before it becomes ready to take off. Decentralized identifiers and verifiable credential models, sometimes built on blockchain, aim to give companies more control over their data while enabling trusted data sharing. However, significant infrastructure investment and maturity are still needed. Some governments are proactively developing corporate ID infrastructure as a public good, such as the account aggregator framework in India which empowers SMEs to digitally share their data for better access to finance. While it has found tractions in these economies, whether the pattern will propagate to other parts of the world is yet to be determined. Conclusion:In summary, corporate ID is progressing but remains fragmented today. There is no silver bullet – a range of stakeholders including registries, banks, service providers and policymakers have important roles to play. Enhancements to the LEI system, OpenID-based initiatives like GAIN, decentralized identity standards, and public infrastructure could help accelerate the development of a trusted and inclusive corporate ID ecosystem supporting improved financial stability, integrity, and access. Political will and multi-stakeholder coordination is essential to realizing the full potential.
I am hopeful that I will find hints to some of the key challenges that I have cited in today’s roundtable.
以下、自動翻訳です。
はじめに:この円卓会議にお招きいただき、ありがとうございます。皆さんとご一緒できて光栄です。コーポレート・デジタル・アイデンティティについて少し述べさせていただきます。
コーポレート・デジタル・アイデンティティ、すなわち企業IDは、企業の識別と確認を劇的に簡素化し、ビジネスを行う上でのリスクとコストを削減する可能性を秘めています。企業が金融サービスをより効率的に利用するための入場券として機能します。しかし、効果的な企業IDシステムを開発するには、いくつかの重要な課題に取り組む必要があります。今日はそのうちの7つを列挙してみました。
キーポイント 法人IDは個人IDよりも複雑です。役員や所有形態など企業の属性は頻繁に変更され、複数の法域にまたがる可能性があります。最終的な受益者の特定は特に難しい課題です。 企業レジストリは、企業データの権威ある情報源として中核的な基礎的な役割を果たしています。しかし、多くのレジストリは、企業IDをよりよくサポートするために、データの公開性、質、深さ、および接続性を強化する必要があります。多くの場合、データはタイムリーに更新されず、私たちは古いデータを見ることになります。 銀行関連のイニシアティブ 銀行は企業IDサービスを提供することで、KYCへの投資を収益化し、コスト・センターをプロフィット・センターに変えるチャンスがあります。国際金融研究所(Institute of International Finance)とOpenIDファウンデーション(OpenID Foundation)が共同で立ち上げたオープン・デジタル・トラスト・イニシアチブ(Open Digital Trust Initiative)はそのようなイニシアチブですが、コスト、データ共有、責任、競争などの面で障害に直面しています。KYCユーティリティは可能性を秘めていますが、これまでのところ結果はまちまちです。 取引主体識別子(LEI)は、企業 ID の出発点として、グローバルで一意かつ相互運用可能な 識別子を提供。検証可能な LEI や電子証明書に組み込まれた LEI のような機能強化は、便益と採用を拡大する可能性がある。しかし、登録企業のカバレッジはまだ改善されていない。また、vLEI は他の検証可能なクレデンシャル・システムとは全く異なる技術スタック上に構築され ていることから、採用の課題が生じる可能性がある。 オープンスタンダードで分散型の認証プロトコルである OpenID と、その拡張機能である「OpenID for Identity Assurance」は、属性の実証性と品質を表現するものであり、企業とサービスプロバイダ間で安全で信頼できる ID 保証とデータ共有を可能にする大きな可能性を秘めています。グローバル・アシュアード・アイデンティティ・ネットワーク(GAIN)イニシアチブは、銀行の既存のKYCプロセスとOpenID標準を基盤として、グローバルで相互運用可能な企業IDおよび認証システムを構築することを目指しています。これは、エコシステムの島々の間に橋を架けるようなものです。異なるエコシステム間の相互運用性は、シドニーの部屋で参加しているディマ・ポストニコフが主導した技術的な概念実証を通じて実証されていますが、ビジネスと運用の現実は、それが離陸する準備が整う前に追いつかなければなりません。 非中央集権的な識別子と検証可能なクレデンシャル・モデルは、時にはブロックチェーン上に構築され、信頼できるデータ共有を可能にしながら、企業がデータをよりコントロールできるようにすることを目指しています。しかし、インフラへの多大な投資と成熟がまだ必要です。 インドのアカウント・アグリゲーター・フレームワークのように、公共財としての企業IDインフラを積極的に開発している政府もあります。このような経済圏では牽引役となっていますが、このパターンが世界の他の地域に伝播するかどうかはまだわかりません。 結論:要約すると、企業IDは進歩していますが、現在も断片的なままです。レジストリ、銀行、サービス・プロバイダー及び政策立案者を含む様々な利害関係者 が果たすべき重要な役割。LEI システムの強化、GAIN のようなオープン ID ベースのイニシ アティブ、分散化された ID 標準、及び公共インフラは、金融の安定性、完全性、及びアクセスの改 善を支援する信頼され包括的な企業 ID エコシステムの開発を加速するのに役立つ可能性があ ります。政治的な意志とマルチステークホルダーの協調が、その可能性を完全に実現するために不可欠です。
本日の円卓会議で私が挙げた重要な課題のいくつかにヒントが見つかることを期待しています。
We had such thoughtful and engaged discussion at this week’s Postgres Extension Ecosystem Mini-Summit! I did learn that one has to reserve a spot for each mini-summit individually, however. Eventbrite sends reminders for each one you sign up for, not all of them.
To reserve a spot and be reminded for forthcoming meetings, hit the Eventbrite page and select a date and hit “Reserve a Spot” for each date you’d like to attend.
Back to this week’s meetup. My colleague Ian Stanton of Tembo gave a great talk, “Building Trunk: A Postgres Extension Registry and CLI”, that provided background on the motivations and problems that inspired the creation of trunk, a binary packaging system for Postgres extensions.
The presentation was followed by 35+ minutes of questions, discussion, and brainstorming, which I’ve summarized below. But first, links!
Video PDF SlidesNow down to business.
Introduction I opened the meeting and introduced Ian Stanton. PresentationIan introduced himself and trunk, “an extension registry and CLI supporting Tembo Cloud. Wants to tell a story, starting with backstory.
Tembo founded November 2022, provide managed Postgres solution called Tembo Cloud. Idea is Postgres can be used for so many different things through the power of extensions, so built use-case optimized “stacks” flavors of Postgres powered by extensions and configurations. Super proud of them, including Message Queue, for which we build an open-source extension.
Envisioned ability to install any extension, including user-provided extensions. Knew we’d need an extension management solution. So we built it.
It’s called trunk, an extension registry and CLI, an open-source app for the community that hosts binary packages for extensions, and powers Tembo Cloud’s extension management.
Q1 2023 had build Tembo CLoud v1 with all extensions bundled in containers. But wanted way to install them on the fly, ideally with installable packages. Explored the ecosystem for tool we could use.
PGXN first we found. Love it, backed by the community, been around since 2011, but hosted source code, not binaries. Also little development since 2012.
Apt and Yum repositories are community-backed and are binaries, just what we wanted, but smaller subset of extensions relative to the 1000s available. Thought it would be too time-consuming to add them all through the community process.
*Steven Miller: in chat: “Also with apt packaging, it requires to install to a specific path, but we needed to customize the install path based on what pg_config shows for share lib and package lib dir. That way we could persist extension installations on tembo cloud”Weighed pros and cons of building one. Pros:
Full control over integration with Tembo Cloud Binary distribution We could build new features quickly We could publish new extensions quicklyCons:
How will the community react? Recreating the wheel?Expected to publish 2–3 extension a day, only do-able with a solution we built.
Want to build something meaningful for Tembo Cloud and the community.
Astronomer Registry for Airflow: Built by Astronomer to find modules for Airflow, very well received by the community.
PGXN, Apt, and Yum repos: Wanted to take the best of them and build on it.
crates.io: Wanted a similar great experience for Postgres extensions.
Vision boiled down to discoverability, categories, ratings system, certification, and indexing of cloud provider support.
Want to package any extension, whether SQL, C/SQL, or pgrx.
Simple experience, like cargo publish
and cargo install cargo-pgrx
.
Eric in chat: “❤️”
Hopes and Dreams: had idea people would magically show up, contribute to the code, and publish their extensions. Wanted to support multiple platforms, architectures, and Postgres versions, and for it to be a one-stop shop for Postgres extensions.
How it works.
CLI and Registry, written in Rust, uses Docker to build extensions. Packages
named <trunk-project-name>-<version>-<pg-version>.tar.gz
. Published with
trunk publish
and installed with trunk install
, putting all the files in
the right places.
Registry stores metadata and service web site and API, and uses S3 bucket for the tar-gzip files.
Example building semver extension:
Create Trunk bundle:
bitcode/src/semver/src/semver.bc
bitcode/src/semver.index.bc
semver.so
licenses/LICENSE
extension/semver--0.10.0--0.11.0.sql
extension/semver--0.11.0--0.12.0.sql
extension/semver--0.12.0--0.13.0.sql
extension/semver--0.13.0--0.15.0.sql
extension/semver--0.15.0--0.16.0.sql
extension/semver--0.16.0--0.17.0.sql
extension/semver--0.17.0--0.20.0.sql
extension/semver--0.2.1--0.2.4.sql
extension/semver--0.2.4--0.3.0.sql
extension/semver--0.20.0--0.21.0.sql
extension/semver--0.21.0--0.22.0.sql
extension/semver--0.22.0--0.30.0.sql
extension/semver--0.3.0--0.4.0.sql
extension/semver--0.30.0--0.31.0.sql
extension/semver--0.31.0--0.31.1.sql
extension/semver--0.31.1--0.31.2.sql
extension/semver--0.31.2--0.32.0.sql
extension/semver--0.32.1.sql
extension/semver--0.5.0--0.10.0.sql
extension/semver--unpackaged--0.2.1. sql
extension/semver.control
extension/semver.sql
manifest. json
Packaged to •/. trunk/pg_semver-0.32.1-pg15.tar.gz
Package up SQL files, control file, SO files, bitcode files into gzip file.
Once it’s published, API surfaces all this information:
[
{
"name": "pg_semver",
"description": "A semantic version data type for PostgreSQL.",
"documentation_link": "https://github.com/theory/pg-semver",
"repository_link": "https://github.com/theory/pg-semver",
"version": "0.32.0",
"postgres_versions": [
15
],
"extensions": [
{
"extension_name": "semver",
"version": "0.32.0",
"trunk_project_name": "pg_semver",
"dependencies_extension_names": null,
"loadable_libraries": null,
"configurations": null,
"control_file": {
"absent": false,
"content": ""
}
}
],
"downloads": [
{
"link": "https://cdb-plat-use1-prod-pgtrunkio.s3.amazonaws.com/extensions/pg_semver/pg_semver-pg15-0.32.0.tar.gz",
"pg_version": 15,
"platform": "linux/amd64",
"sha256": "016249a3aeec1dc431fe14b2cb3c252b76f07133ea5954e2372f1a9f2178091b"
}
]
},
{
"name": "pg_semver",
"description": "A semantic version data type for PostgreSQL.",
"documentation_link": "https://github.com/theory/pg-semver",
"repository_link": "https://github.com/theory/pg-semver",
"version": "0.32.1",
"postgres_versions": [
15,
14,
16
],
"extensions": [
{
"extension_name": "semver",
"version": "0.32.1",
"trunk_project_name": "pg_semver",
"dependencies_extension_names": null,
"loadable_libraries": null,
"configurations": null,
"control_file": {
"absent": false,
"content": "# semver extension\ncomment = 'Semantic version data type'\ndefault_version = '0.32.1'\nmodule_pathname = '$libdir/semver'\nrelocatable = true\n"
}
}
],
"downloads": [
{
"link": "https://cdb-plat-use1-prod-pgtrunkio.s3.amazonaws.com/extensions/pg_semver/pg_semver-pg14-0.32.1.tar.gz",
"pg_version": 14,
"platform": "linux/amd64",
"sha256": "f412cfb4722eac32a38dbcc7cd4201d95f07fd88b7abc623cd84c77aecc8d4bb"
},
{
"link": "https://cdb-plat-use1-prod-pgtrunkio.s3.amazonaws.com/extensions/pg_semver/pg_semver-pg15-0.32.1.tar.gz",
"pg_version": 15,
"platform": "linux/amd64",
"sha256": "9213771ffc44fb5a88726770f88fd13e62118b0f861e23271c3eeee427a23be9"
},
{
"link": "https://cdb-plat-use1-prod-pgtrunkio.s3.amazonaws.com/extensions/pg_semver/pg_semver-pg16-0.32.1.tar.gz",
"pg_version": 16,
"platform": "linux/amd64",
"sha256": "8ffe4fa491f13a1764580d274e9f9909af4461aacbeb15857ab2fa235b152117"
}
]
}
]
Includes different tar-gzip files for different versions of Postgres, the contents of the control file, dependencies; loadable libraries and configurations; and the one extension in this package — some can have many like PostGIS. Then Postgres version support and some other metadata.
What it looks like on the web site, includes README contents, data from the last slide, install command, etc.
This is what installation looks like:
$ trunk install pg_semver
Using pkglibdir: "/usr/lib/postgresql/16/lib"
Using sharedir: "/usr/share/postgresql/16"
Using Postgres version: 16
info: Downloading from: https://cdb-plat-usel-prod-pgtrunkio.s3.amazonaws.com/extensions/pg_semver/pg_semver-pg16-0.32.1.tar.gz
info: Dependent extensions to be installed: []
info: Installing pg_semver 0.32.1
[+] bitcode/src/semver/src/semver.bc => /usr/lib/postgresql/16/lib
[+] bitcode/src/semver. index.bc => /usr/lib/postgresql/16/lib
[+] semver.so => /usr/lib/postgresql/16/lib
info: Skipping license file licenses/LICENSE
[+] extension/semver--0.10.0--0.11.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.11.0--0.12.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.12.0--0.13.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.13.0--0.15.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.15.0--0.16.0.sql = /usr/share/postgresql/16
[+] extension/semver--0.16.0--0.17.0.sql => /us/share/postgresql/16
[+] extension/semver--0.17.0--0.20.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.2.1--0.2.4.sql => /usr/share/postgresql/16
[+] extension/semver--0.2.4--0.3.0.sql > /us/share/postgresql/16
[+] extension/semver--0.20.0--0.21.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.21.0--0.22.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.22.0--0.30.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.3.0--0.4.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.30.0--0.31.0.sql = /usr/share/postgresql/16
[+] extension/semver--0.31.0--0.31.1.sql => /usr/share/postgresql/16
[+] extension/semver--0.31.1--0.31.2.sql => /usr/share/postgresql/16
[+] extension/semver--0.31.2--0.32.0.sql => /usr/share/postgresql/16
[+] extension/semver--0.32.1.sql => /usr/share/postgresql/16
[+] extension/semver--0.5.0--0.10.0.sql => /usr/share/postgresql/16
[+] extension/semver--unpackaged--0.2.1.sql => /usr/share/postgresql/16
[+] extension/semver.control => /usr/share/postgresql/16
[+] extension/semver.sql => /usr/share/postgresql/16
***************************
* POST INSTALLATION STEPS *
***************************
Install the following system-level dependencies:
On systems using apt:
libc6
Enable the extension with:
CREATE EXTENSION IF NOT EXISTS semver CASCADE;
CLI pulls down the tar-gzip, unpacks it, and puts the files in the right places and tells the users what other commands are needed to enable the extension.
Pause to take a sip of water.
David Wheeler (he/him) in chat: “STAY HYDRATED PEOPLE!”State of the project. Trunk powers extension management for Tembo Cloud, 200 extensions on the platform, install and enable on the fly. Tembo Cloud likely trunk’s #1 user.
Get lots of site traffic, especially around categorization, addresses the discoverability problem set set out to solve.
Jeremy S in chat: “Interested in insights from site traffic - you mentioned that ‘categorization’ was popular - any other things that traffic patterns seem to suggest you might have done really well, or clearly is needed?”But pretty minimal community involvement, out fault for not involving the community early on.
Did we solve the problem?
For Tembo Cloud: yes! Trunk is core component of the Tembo Cloud platform that lest us offer high number of extensions. For the community: no! But helped bring more awareness to the opportunities to improve the ecosystem as a community. Saw other solutions arise around the same time, including dbdev and pgxman, and Yurri at Omnigres is working on something as well. Huge opportunity to solve this together. Steven Miller in chat: “I think it is very nice way to install other extensions via an extension how dbdev works” David Wheeler (he/him) in chat: “GRANT!” Grant Holly in chat: “Oh hi”Lessons Learned
It’s a really hard problem to solve! As you add more layers of complexity, like different architectures, versions of Postgres, it gets harder and harder.
Steven Miller in chat, Replying to “The approach to use …”: “The downside for this approach includes missing chip-specific instruction support, for example AVX512, which optimizes performance on some extensions. However if you are building with docker on the same architecture as the host, then it still includes these instructions.”
David Wheeler (he/him) in chat, Replying to “The approach to use …” “Also presumably no support for building for non-Linux platforms, yes?”
The extension ecosystem truly is the wild west, not really best practices around building, versioning, and releasing, and when you’re collecting and housing them, it makes things difficult. A huge opportunity for us to come up with those standards and share them with the community.
Community involvement is crucial, wish we’d done it better early on, that’s why we’re all here today! Solution to build together doesn’t happen if we don’t tackle it as a community.
Similarly, wish we’d reached out to folks like David and Devrim early on, to get more insight from them and bring the community into the project from the beginning
The future of trunk
Registry and CLI will continue to serve Tembo Cloud
Has paved the way for binary packaging and distribution in PGXN v2 that David is spearheading, will at least will inform and perhaps be the basis for that part of the project.
That’s all, thank you, back to you, David!
DiscussionDavid Wheeler (he/him): Thanks for history an context, Ian! Questions or discussion topics? Some comments in the thread from Steven and Tobias.
Tobias Bussmann: in chat: speaking of paths: it would be super helpful if postgresql would support loading extensions from additional paths beside the $SHAREDIR/extension and $PKGLIBDIR directories. At least following directory symlinks within…
Steven Miller in chat, Replying to “The approach to use …”: I tried to make it work for Mac, for example, but the docker support didn’t work basically. I think it might work for Windows, since they have better container support. However I didn’t try that yet.
David Wheeler (he/him): Reads Tobias’s comment. You can specify a
subdirectory in the sharedir
and maybe the moduledir
? But it’s a
little hinky right now. Steve, do you want to talk about the us of Docker to
build images?
Steven Miller: Yeah, I’d love to. To Tobias’s point, agree, on Tembo Cloud,
we have a persistent directory where wer’re sintalling extensions, but
because there is no way for an extra sharedir
or package dir, we’re
persisting all of the library files, including Postgres core. Not ideal,
especially for upgrades.
Approach for building ind Docker: been nice, do the build ina Dockerfile, start the container, then install and compare the difference between layers and zip up all the new files. Great for cross-compile but, not working for mac or other systems. Will need a fallback option to do a local build.
Jeremy S: in chat, Replying to “speaking of paths: i…”: Exactly same point was also mentioned just this morning on slack by MatthiasDavid Wheeler (he/him): Makes sense, thanks. What other bits do you feel like could be useful for packaging binaries at a community level?
Steven Miller: Sometimes we install binaries with trunk, but then difficult
to know what has been installed. Nothing like apt where there is a history
of what is installed or uninstall. Would be nice to do something like trunk list
and see everything that has been installed. Also, future should be not
just install but management, including turning extensions on, and there are
a lot of ways to turn them on.
Ian Stanton: uninstall
would be useful, too.
David Wheeler (he/him): Other questions about trunk or challenges to binary distribution it brings?
*Tobias Bussmann in chat, Replying to “speaking of paths: i…”: this would allow an immutable PostgreSQL base package and still allow to install extensions on top. This is esp. important if you need to have singned packages like on macOS
*nils in chat, Replying to “speaking of paths: i…”: Guess there is some prior art in how search_path in pg work, or the PATH in unix’s.
Should be doable to allow to specify some kind of ordered search path, where Postgres will look for extensions. That way, Postgres can protect it’s own libs to no be overwritten by external libs, but allow for loading them from extra paths.
Yurri: There is CREATE EXTENSION
and other extensions like logical
decoding plugins. Does trunk handle them?
Steven Miller: We think of it as four types extensions into 2x2 matrix: 1.
Does it require CREATE EXTENSION
true or false; and 2. Does it have a
loadable library true or false. The false/false category is output plugins;
The true/true category, e.g. pg_partman
, pg_cron
; CREATE EXTENSION
false
and loadable library true, e.g., autoexplain
, just a library, no upgrade
concerns; and then CREATE EXTENSION
true and loadable library false is the
default case.
Ian Stanton: Steven wrote a blog on this.
Eric in chat: Does pgrx make the process of building easier or harder and is there anything we can do today to make rust extension building better?
Jason Petersen in chat: Yeah, it sounds like we need some sort of system database like apt has; would enable management, uninstall, version list, whether upgrades are available, etc
Yurri: That would be great. What other modules are there without extensions,
like autoexplain
?
Ian Stanton: auth delay is another, base backup to shell, considered parts of postgres, but we have trouble categorizing them. There are 10-15 I’ve come across.
Yurri: ARe these categories on Tembo, can you click a button?
Ian Stanton: Not a category, but would be a good one to add.
Steven Miller in chat: This one! https://tembo.io/blog/four-types-of-extensions
It’s in the API metadata
Sorry if I mispronounced your name Tobias
David Wheeler (he/him) in chat: SAME
Yurri: Did you say output plugins are handled with Tembo:
Steven Miller: YOu can install them with trunk, yes.
Yurri: And you have the build pipeline that will work without plugins too, yeah, cool.
David Wheeler (he/him): Tobias, did you want to say more about the path issues?
Tobias Bussmann: Sure! We are building the Postgres.app, distribution for macOS, working different from Linux systems. We distribute some extensions directly, but also allow building and installing extensions on it. Works nicely, even with pgxn client, but it’s built within the application, which breaks the code signature.
We always have to fight against a breaking system to allow that. Possible, but would be much cleaner to specify an extra directory where extensions could be loaded, and we could distribute packages with binary extensions that the user could download and install separately from the Postgres.app.
David Wheeler (he/him): You’re not suggesting a different directory for every extension with a module, but just another path in the search path that’s not subject to the signature verification.
Tobias Bussmann: Yes, that would be an option, but with a flexible system could use one per extension or just specify a second directory. Contrib extensions sometimes seen as part of Postgres, and they’re all stuffed in the same directory with third party extensions, which gets confusing and hard to manage.
Steven Miller in chat: In the previous extensions mini summit, Yuri mentioned that he was working on a patch to postgres for extra libdir, extra share dir, but I have not been tracking this onenils: That’s what I was saying in chat, there is prior art in Postgres and Unix systems where you can specify a search path in postgres for a list of schemas, and in Unix the path is to find binaries. Give me a delimited list of directories on my system. Could be super user only, where they can specify where they’re installed, and we can go through the list ot find an extension.
David Wheeler (he/him): I might be imagining this, but I seem to recall there was a proposal to have extensions in their own directories, which would be nice for packaging, but then every time you add one you have to add another directory to the list and there is some fear the lookup time could be too long.
Jeremy S in chat, replying to “speaking of paths: i…”: (Or like LD_LIBRARY_PATH )
David Wheeler (he/him) in chat, replying to “speaking of paths: i…”: LD_LIBRARY_PATH is all but dead on macOS
Jason Petersen: If it happens at startup I can’t imagine that being a concern. If the list changes you reboot. It’s not gonna be a performance problem, I hope.
*David Wheeler (he/him): Or HUP it if you don’t want downtime.
Jason Petersen: Sure, but it doesn’t need to be on every command.
*David Wheeler (he/him): Eric, do you want to pose your question about pgrx?
Eric: Sure. Wanted to know, were there stumbling blocks to get pgrx support built into trunk, and does it make things easy or difficult? Different from C path, are there things we could do to make things easier today?
Ian Stanton: Yeah, I think the issue is mostly on our end. We have a separate image for each version of pgrx, and keeping up with the releases is challenging. We need to rethink our image building strategy. Shouldn’t be one image for each version of pgrx. That’s the biggest thing I’ve noticed, mostly on our side.
*David Wheeler (he/him): Because you need the install the version of pgrx that the extension requires before you do the build, and that’s just too slow?
Ian Stanton: Could be too slow. We’ve known about this problem for some time, just hasn’t been addressed yet.
Eric: Okay, maybe we can talk about it offline one day, be happy to chat. I think we’re close to being able to have the CLI, cargo-pgrx, be a different version than whatever version the extension uses.
Ian Stanton: That would be super useful!
Eric: Yeah, I think we’re close to being at that point, if not there already. We can talk about that offline.
Ian Stanton: Nice! We’ll reach out in Discord.
*David Wheeler (he/him): Other comments or questions, or people who have worked on other kinds of binary registry things, would love to hear more from other perspectives. Devrim is going to talk about the Yum repository next week [ed. correction: in two weeks].
Steven Miller in chat: Daniele last time mentioned Pip is good example of mixing source and binary distributions
Eric: I have a random question related to this. In the past and recent history, has hackers talked about some way of storing extension in the database rather than relying on the file system?
*David Wheeler (he/him): Yes! In this long thread from 2011 [ed. Correction: 2013] Dimitri was proposing a “unit”, a placeholder name, where the object would be stored in the database. Very long thread, I didn’t read the whole thing, lot of security challenges with it. If it needs a shared object library loading having to be written to the file system it’s just not going to happen. I don’t know whether that’d be required or not.
Dimitri also worked on a project called pginstall where you could install extensions from the database like dbdev, but not just TLEs, but anything. The idea is a build farm would build binaries and the function in the database would go to the registry and pull down the binaries and put them in the right places on the file system.
There were a lot of interesting ideas floating around, but because of the legacy of the PGXS stuff, it has always been a bit of a struggle to decide not to use it, to support something not just on the machine, but do something over libpq or in SQL. Lot of talk, not a lot of action.
*Tobias Bussmann in chat in response to “In the previous ex…”: still searching on hacker for it. Meanwhile I found: https://commitfest.postgresql.org/5/170/
Steven Miller in chat: That approach is very awesome (install via extension)
Eric: I can see why it would take some time to sort it all out. One thing to require super user privileges to create an extension, but also having root on the box itself? Yeah.
Yurri: TLE plugs into that a little bit for a non-shared object. Not exactly storing it in the database, but does provide a SQL based/function method of installing from inside the database, but only for trusted languages, not shared objects.
*David Wheeler (he/him): dbdev install
does download it from database.dev
and stores it in the database, and has hooks into the CREATE EXTENSION
command and pulls it out of its own catalog. Was a similar model with
pginstall
, but with binary support, too.
Yurri: Back to trunk. When you start building, and have to deal with binaries, pgxn you can put the source up there, but I want to get to the whole matrix of all the different versions. Every extension author does it a little different. Some extensions have versions for Postgres 15, another for 14, some have the same version across all the majors, sometimes an extension works for some majors and others. Has trunk expanded to other Postgres versions to support the whole exploding matrix of stuff that does and doesn’t work, 5-6 majors, gets to be a large matrix, a lot to keep track of. How’s that working out for the builds and managing that matrix.
Steven Miller in chat: Dimensions I think are: pg version architecture chip-specific instructions (edge case for native builds?)Steven Miller in chat: We just announced support for 14 and 16
David Wheeler (he/him) in chat, replying to “Dimensions I think a…”: OS, OS version
Steven Miller in chat,: Replying to “Dimensions I think a…”: Ah right
Ian Stanton: Steven do you want to take that one?
Steven Miller: Oh yeah. We’ve started toe-dipping on this one. Started with
Tembo Cloud’s platform, but have no released Postgres 14 and 16, and also
trunk has built-in support for other architectures, such as arm, or whatever
the Docker --platform
flag supports. We looked at mac builds, not working
yet, might work for Windows, which ahs better container support, but I don’t
know, and also there is an edge case for pg_vector especially, which
compiles to include ship-specific instructions for AVX512, which helps with
vector. So that’s another dimension to consider.
Yurri: Part of the idea behind this forum is to see if we can chart a path forward, maybe not solve everything. What can we solve, how can we make something a little better for Postgres at large?
Eric in chat: Even as a Mac user I don’t know the answer to this… what’s the common Postgres package there? Postgres dot app, homebrew, something else?
David Wheeler (he/him) in chat: pgenv! (self-promotion)
Eric in chat: I assume folks don’t use macOS in prod but developers are important too
nils in chat, Replying to “Even as a Mac user I…”:
$ git clone ..
$ ./configure
$ make
$ make install
At least that is what I do 😄
Steven Miller: In my opinion, the way to approach it is to know all the dimensions you need, and in the metadata API say which binaries are available. Then get through it with testing and badging If we let things get built, to what extent is it tested and used? That can help. Daniele was in the previous call, said we could look to Pip and Wheel files for inspiration, and Adam on our team has said the same. This is something that has some binary and some source, and falls back on doing the build when it needs to.
*David Wheeler (he/him): I’ve been thinking about this quite a bit lately. Can see needing to take advantage of multiple platforms available through GitHub workflow nodes or the community’s build farm, which has a vast array of different architectures and platforms to build stuff. There are precedents!
I imagine a system where, when something is published on PGXN, another system is notified and queues it up to all its build farm members to build binaries, ideally without full paths like trunk, and making them available for those platforms. Building out that infrastructure will take a fair bit of effort, I think. With cross-compiling is available it might be…doable? But most modules and for SQL and maybe Rust or Go extensions, but a challenge for C extensions.
This is a problem I’d like us to solve in the next year or two.
Steven Miller in chat, replying to “I assume folks don’t…”: Yeah exactly, like trunk install after brew install postgres
Tobias Bussmann in chat, replying to “Even as a Mac user…”: this seems to be quite spread. There are also people that prefer docker based installs
Eric in chat: pgrx supports cross compilation
With a caveat or two!
Eric in chat, replying to “Even as a Mac user I…” @nils same. For v9.3 though 16!
*David Wheeler (he/him): What else? Reading the comments.
Yurri: I think maybe that PGXN JSON file, I know you’ve been spending time on it, David, including the proposal on namespacing a few days ago. That feels like it could be helpful to be part of this. IF it could be something we could center around… The first time I wanted to put an extension on PGXN, it took me a long time to figure out that JSON file. I didn’t find the blog post that goes through it in nice detail till like two weeks after. If I’d found it sooner I could have skipped so many things I tried to figure out on my own.
If we can center around that file, it’ll draw more attention to it, more links back to it, more examples people blog about here and there, it helps going forward. The trick is getting it right not being this massive thing no one can figure out, or has too many options, but hits all the points we need.
nils in chat, replying to “Even as a Mac user I…”: Well, mostly for extension, for Postgres I rely on David’s pgenv
*Eric * in chat, replying to “Even as a Mac user I…”: @Tobias Bussmann hmm. Makes it difficult to get an extension installed.
*David Wheeler (he/him): I’ve been thinking about this a lot, drafted a doc some of my colleagues at Tembo have read over and I hope to publish soon [ed. Note: now published], thinking through what a v2 of the PGXN Meta Spec might include. I think we should extend with list of external libraries required, or the architectures it supports, or it’s a loadable library or an app that doesn’t even go into the database.
I would like soon to draft an actual revision of the spec, and document it well but also turn it into a JSON Schema document so we can automate publishing it and verification in the same place. I also imagine building an eventual replacement or evolution of the PGXN client or trunk client or some client that you can use to manage that thing. I think pgrx does that, adding metadata via the client rather than parse and understand the whole file.
I’m with you it could get really complicated, but I’m not sure I see an alternative other than building good tooling to minimize the pain.
Ian Stanton: I think automatically pulling that information when it’s readily available would be super helpful. We use it as an app to just take care of things for people.
*David Wheeler (he/him): Right, and then if we’re successful in getting it
done it’s getting people to take up the tools and start using them. There’s
only so much we can infer. I can tell how to do a build if there’s a
Makefile
or a configure
file or a cargo.toml
, but that doesn’t reveal
what libraries are required. This is why there’s a lot of hand-tuning of
RPM and Apt spec files.
Steven Miller in chat: We are calling this “system dependencies”
Ssl and glibc the main ones 🙂
Jason Petersen in chat: And sometimes the package names aren’t even 1—1 mappings
Eric in chat: Ha! Try relying on elasticsearch as a runtime dependency! 😞
Yurri: That’s another thing to touch on. A lot of extensions are just a thin
layer of glue between Postgres and some OSS library that someone else
maintains. But the trick, when you want to build a Yum package, the
dependency has a different name than the rest of the RedHat ecosystem vs.
the Debian ecosystem. So part of what Devrim has to do to maintain the RPM
packages is manually sort all that out, because you can’t automatically…
libc! It’s called glibc
in RedHat and just libc
in Debian, and every
package has slightly different names. Do how do you manage that in trunk? Do
you pull the source for any dependencies? Does your Docker image…I don’t
know how this is working.
David Wheeler (he/him) in chat: I want to build a json schema validation extension in Rust using https://github.com/Stranger6667/jsonschema-rs or something
Tobias Bussmann in chat, replying to “Ha! Try relying o…”: or V8 🤯
Ian Stanton: Two sides to that one is build time dependencies, and there
there are runtime dependencies. I just dropped an example for some random
extension. Tthe way we’ve been building this is to write out a Dockerfile
that can include build time dependencies. [hunts for link…]
Ian Stanton: We specify them all there. But for runtime, we don’t know what’s required until we test the thing. We have stuff in our CI pipelines to install and enable the extension to see if it works. If it doesn’t, it will report a missing dependency. Then we know we need to add it to our Postgres images. Not the best flow for finding these dependencies. Steven, want to add anything more to the build time dependency piece?
David Wheeler (he/him) in chat, replying to “Ha! Try relying on …”: Next version of plv8 released on PGXN will have v8 bundledSteven Miller: A lot share the same ones, SSL and glibc, so we just build with the same versions we run on Tembo Cloud. In the metadata we list all system dependencies, that’s what we build towards, and include them in the Docker image. If you pick a different stack, like the Machine Learning stack, it has all the Python stuff in the base image. We don’t really love this, but this is something where Python wheel might inspire us, becaus it has packaging and system dependencies.
Eric in chat, replying to “I want to build a js…”: I feel like I’ve seen one already?
David Wheeler (he/him) in chat, replying to “I want to build a js…”: GIMME
Yurri: If you really want to od this right, just like in the RPM
repositories, you have to know what the dependencies are. David, I’m
curious, what your thoughts are, if this is to be done right, there has to
be a way to indicate dependencies in the META.json
file, but then I’m
talking about Debian and RedHat, but what about Mac? Windows doesn’t really
have a packaging system. There are BSDs, other places Postgres can run,
probably have to narrow the scope a bit to solve something.
Tobias Bussmann in chat, responding to “Ha! Try relying o…” Sounds promising, but for which architectures? I have good hope for pljs as replacement for plv8
Ian Stanton in chat: https://github.com/tembo-io/trunk/blob/d199346/contrib/fuzzystrmatch/Trunk.toml#L13
David Wheeler (he/him): Fortunately there are only around 1100 extensions in the world, a relatively low barrier at this point. Some of these other things have thousands or millions of extensions.
Yurri: I guess when you put it that way! But I wasn’t going to go through all 1000 of them one-at-a-time.
David Wheeler (he/him): No. I posted about this on Ivory a few weeks ago [ed. correction: he means on Mastodon]: how does one do this in a platform-neutral way. There are some emerging standards where people are trying to figure this stuff out. One is called purl, where you specify dependencies by packing URLs, or “purls”, and then it’s up to the installing client to resolve them vai whatever the packaging system it depends on.
I would assume on Windows we’d have to say “it works great as long as you use Chocolatey” or something like that. But it’s certainly a difficult problem. I’m looking forward to your talk about your unique approach to solving it, Yurrii [ed. note: that’s the May 1 mini-summit], that’s going to be super interesting.
David G. Johnston: Ultimately you just crowd sourcing. If we just say “this is what we call this thing in PostgreSQL world”, then if people need to compile it on Chocolatey on Windows, they figure it out and contribute it. Or on Debian or RedHat. Just facilitate crowd-sourcing, metadata in a database.
David Wheeler (he/him): My initial idea was a global registry that people contribute to just by editing files in a GitHub repository.
David G. Johnston: HashiCorp has to have something like that already, there’s stuff out there, no need to reinvent the wheel. This is a global problem if we open-source it we can solve it.
David Wheeler (he/him): Right. Really appreciate everyone coming. Great discussion, I appreciate it. In two weeks, Devrim Gündüz is going to talk about the Yum Community Repository and the challenges of RPMifying extensions. I had this idea of automating adding extensions to the Yum and Apt repositories, an Devrim is a little skeptical. So super look forward to his perspective on this stuff. Two weeks from today at noon [ed.: America/New_York]. Thanks for coming!
Eric in chat: Thanks a ton! This is exciting stuff.
Tobias Bussmann in chat: Thanks all!
Grant Holly in chat: Thanks everyone. Great discussion
Jeremy S: in chat: Thanks david
Steven Miller in chat: Thanks all! Cya next time
Jeremy S in chat: Isn’t bagel supposed to come for the end
Ian Stanton in chat: Thanks all :)
More about… Postgres PGXN Extensions PGConf Summit trunkThere is a Social Contract with sites and their name policies. Some how companies still don’t really understand this (See what WOW did in 2010) . There is a massive story breaking this week about how GlassDoor a site where people who can create profiles and anonymously rate their employers over their entire careers has […]
The post Real Names & Glassdoor & Fishbowl appeared first on Identity Woman.
Update 2024-03-22: To compliment high-level discussions on the #extensions channel on the Postgres Slack, I also created a pull request to make it easer directly comment to this post point by point. Keep the feedback coming, and thank you!
Update 2024-03-25: Clarified the definition of “Release” and made “Maintainers” plural. Thanks to Matthias van de Meent for the suggestions!
This post proposes a new metadata standard for extension packaging, distribution, and delivery, building on the PGXN Meta Spec to address its shortcomings and emerging use cases 12 years on. The goals include:
Don’t break the existing standard, but add to it Add explicit support for different types of Postgres extensions, such as background workers and loadable modules Add additional metadata for curation and automated binary compilation Add hooks for registry and third-party-contributed metadata, including binary distribution options and stats & reports.There are two high level categories of metadata, split into two separate files:
Package Metadata provided by extension package authors Registry Metadata aggregated by the root registry from various sources, including data derived from the extension source code or package metadata, but also trusted third parties such as packagers, smoke testers, security scanners, and more.Following community discussion of this proposal, the Package Metadata will lead to a draft for PGXN Meta Spec version 2.0.0, while the Registry Metadata will guide the design and implementation of the Root Registry APIs required to provide it.
TermsDefinition of terms as used in this document, because every language and distribution system uses terms differently. These are ours.
Extension: a software component that extends the capabilities of a
PostgreSQL database or cluster. Extensions may be CREATE EXTENSION
extensions, background workers, command-line apps, loadable modules,
shared libraries, and more.
Package: A collection of extensions that are released, versioned, and distributed together. Packages may be downloaded directly from version control repositories or in archive files generated by a release tag.
Package Path: Identifies a package, declared in the package metadata file. A package path should describe both what the package does and where to find it. Typically, a package path consists of a repository root path — the directory that contains the metadata file — and a directory within the repository.
Repository: The version control repository where the package is developed, referenced as package path or URL.
Repository root path: The portion of the package path that corresponds
to the root directory of the version control repository where the package
is developed, and which contains the metadata file. Most package are
defined in their repository’s root directory, so this is usually the
entire path. For example, github.com/example/pgtap
is the repository
root path for the package of the same name.
Source Distribution: The contents of a single package bundled together
with package metadata into distributable archive
file, usually named with the last part of the package path or the main
extension, a dash, and the version, e.g., pgtap-1.14.3.zip
.
Binary Distribution: A binary software distribution of a package, compiled and packaged for a particular OS version, platform, and hardware architecture. Examples include deb, RPM, and wheel.
Release: A single version of the package made available to the public on
PGXN, expressed as the package path, an at sign, and the semver. Example:
github.com/theory/pgtap@v1.14.3
.
The extension author provides this data as part of a release submitted to the root registry. The registry itself will not change this data in any way, but provide it as-is.
EssentialsEssential information about the package itself, including its path, name, version, list of authors, license, etc. Ecosystem applications use this data for indexing, naming conventions, and display information.
Fields (all required):
Package: A package path uniquely identifying the package (usually the source repo host name and path; see this RFC) Name: The name of the extension package, usually the same as the main extension, not unique but conflicts are resolved by escalation topackage
Version: The version of the package, expressed as a SemVer
Abstract: A short description of the purpose of the package
Description: A longer description of the package, answering the
question “what is this thing and what value is it?”
Maintainers: List of maintainers, each an object with name
and either
email
or url
(or both)
License: An SPDX 2.3 license expression comprised of one or more
licenses from the SPDX License List
Spec: Information about the metadata spec itself, a version and URL
Example:
{
"package": "github.com/example/pg-pair",
"name": "pair",
"version": "1.1.0",
"abstract": "A key/value pair data type",
"description": "Adds a key/value pair data type to Postgres, useful for specifying arbitrary key/value function parameters.",
"maintainers": [
{
"name": "Naomi Nagata",
"email": "naomi@example.com",
"url": "https://example.com/roci/naomi"
}
],
"license": "PostgreSQL OR MIT",
"spec": {
"version": "2.0.0",
"url": "https://pgxn.org/meta/spec.txt"
}
}
Other possibilities:
License File: An alternative tolicense
for nonstandard licenses
(from cargo)
Contributors: List of people who have contributed to the package
Emeriti: List of former maintainers and contributors
PGXN Compatibility:
Overloads the string representation of the spec v1 field, but it should be able to parse either one, especially with the spec version to differentiate. Uniqueness ofpackage
and relationship to name
PGXN has maintainer
; is it really worth pluralizing it? Maybe we don’t
need it at all, given repository and issue links.
Artifacts
A list of links and checksums for downloading the extension package in one or more formats, including source code, binaries, system packages, and more. Apps use this information (along with registry metadata) to determine the best option for installing an extension on a particular system.
This information is optional, and provided in addition to the source code
available from the root registry itself. The idea is that, on release, the
release manager creates artifacts in one or more locations (such as GitHub
releases), and it’s useful to link to them from the registry. Binary builds
may be unlikely today, but leave the option open for now by allowing arbitrary
text in the type
field.
Each item must have:
Type: The type of artifact: Source, binary, RPM, etc. URL: Link the the artifact SHA: A SHA checksumEach URL must properly resolve and the checksum must match.
[
{
"type": "source",
"url": "https://github.com/theory/pg-pair/releases/download/v1.1.0/pair-1.1.0.zip",
"sha256": "2b9d2416096d2930be51e5332b70bcd97846947777a93e4a3d65fe1b5fd7b004"
},
{
"type": "binary",
"url": "https://github.com/theory/pg-pair/releases/download/v1.1.0/pair-1.1.0-linux-amd64.tar.gz",
"sha1": "12d9bc5cfb6bc3c453627eac69511f48be63cfc0"
},
{
"type": "binary",
"url": "https://github.com/theory/pg-pair/releases/download/v1.1.0/pair-1.1.0-linux-arm64.tar.gz",
"sha1": "787dc39137f7d1510a33ab0a1b8905cd5f3f72d1"
}
]
PGXN Compatibility:
New object, no conflicts.
ResourcesExternal information about the package, mostly links, including source code repository, bug reporting, documentation, badges, funding, etc. Apps use this data for links, of course, but also full text indexing, documentation rendering, and displaying useful information about the extension.
Fields:
Homepage: URL for the package’s home page Issues: URL to issues Documentation: URL to documentation Support: URL to support Repository: Source code repository URL Badges: Array of badge link objects Funding: Array of funding objects{
"homepage": "https://pair.example.com",
"issues": "https://github.com/example/pair/issues",
"documentation": "https://pair.example.com/docs",
"support": "https://github.com/example/pair/discussions",
"repository": "https://github.com/example/pair",
"badges": [
{
"alt": "Test Status",
"src": "https://test.packages.postgresql.org/github.com/example/pair.svg"
}
],
"funding": [
{
"type" : "individual",
"url" : "http://example.com/donate"
},
{
"type" : "patreon",
"url" : "https://www.patreon.com/example"
}
]
}
PGXN Compatibility:
issues
replaces bugtracker
and is just a URL. Can support either
repository
is just a URL instead of an object. Can support either
but with the package
key referencing the repository path perhaps
we don’t need to change it.
Contents
A description of what’s included in the package. Often a package consists of
multiple extensions, such as PostGIS, which includes postgis
,
postgis_tiger_geocoder
, address_standardizer
, and more.
Furthermore, some extensions are not CREATE EXTENSION
-type extensions at
all, such as background workers, command-line apps, loadable modules,
shared libraries, and more. Each should be listed along with documentation
links where they differ from the package overall (or are simply more
specific).
Fields:
Extensions: Object describingCREATE EXTENSION
extensions. Keys
are extension names, fields are objects with the fields sql
, doc
,
abstract
, control
(same as control file, but can use the file itself),
tle
(meaning it can be used as a trusted language extension), and
preload
(to indicate whether its libraries need to be loaded in advance
via shared_preload_libraries
, session_preload_libraries
, or
local_preload_libraries
).
Workers: Object describing background workers. Keys are worker names,
fields are objects with the fields bin
, doc
, and abstract
.
Apps: Object describing applications, command-line or otherwise. Keys
are app names, fields are objects with the fields bin
, doc
, and
abstract
.
Modules: Object describing loadable modules that can be loaded into
Postgres (not necessary for extensions that include libraries). Keys are
module names, fields are objects with the fields lib
(without file
extension), doc
, abstract
, and preload
.
Libraries: Other libraries that may ship in the package and need to be
installed but are not loadable modules, such as a dynamic library used
by an app. Keys are library names, fields are objects with the fields
lib
(without file extension) or dir
(for a directory of files), doc
,
and abstract
.
{
"extensions": {
"pair": {
"sql": "sql/pair.sql",
"doc": "doc/pair.md",
"abstract": "A key/value pair data type",
"preload": "session",
"tle": true,
"control": {
"directory": "pair",
"module_pathname": "$libdir/pair",
"relocatable": true
}
}
},
"workers": {
"pair_pruner": {
"bin": "bin/pair_pruner",
"doc": "doc/pair_pruner.md",
"abstract": "A worker to periodically prune pairs"
}
},
"apps": {
"pair_rand": {
"bin": "bin/pair_rand",
"doc": "doc/pair_rand.md",
"abstract": "Command to generate random pairs of strings"
}
},
"modules": {
"lib_pair": {
"lib": "lib/lib_pair",
"doc": "doc/lib_pair.md",
"abstract": "A library hooking function calls to convert pairs to named parameters",
"load": "shared_preload_libraries"
}
},
"libraries": {
"ruby_pair": {
"dir": "lib/gems",
"abstract": "Ruby libraries required to run the extension"
}
}
}
Other options:
Allow or require an array of docs? Specify support for specific doc formats (markdown, manpage, plain text, MkDocs, etc.)? Support glob patterns? Add libraries as sub-key for workers and apps, e.g. for a Ruby app that requires a directory of gems?PGXN Compatibility:
Significantly different from the existing provides
object, so key this one
under contents
or manifest
and continue to support both.
A list of external dependencies required to configure, build, test, install, and run the extensions in the package. These include not only other extension packages, but also external libraries and system dependencies. And let’s not forget the versions of Postgres required, as well as any OS and version dependencies (e.g, does it work on Windows? FreeBSD? What versions?) and architectures (arm64, amd64, etc.)
Fields:
platforms: Platforms supported by the package, defined as OS and architecture. Assume all platforms if none are listed.
postgres: Versions of PostgreSQL required by the package, specified as
a version range, as well as compiled features (--with-*
configure
options)
pipeline: The build pipeline required to build the extension. Options:
pgxs
, meson
(?) pgrx
, gnu
(./configure
), gem
, cpan
, pip
,
go
, etc.
prereqs: Object defining external dependencies required for different phases:
configure: Dependencies to configure the package (e.g., items required for./configure
or make
to work)
build: Dependencies to build the package (in addition to run
dependencies)
test: Dependencies to test the package (in addition to build and
run dependencies)
run: Dependencies to run the package
develop: Dependencies to develop the package (in addition to all
other phase dependencies)
Each points to an object with at least one of these keys:
requires: Required to use the package recommends: Not required, but recommended as a best practice suggests: Not required, but nice to have conflicts: Package will not work with these itemsThese, in turn, contain at least one of these keys:
package: Other packages, expressed by their package paths, pointing to a version expression external: External dependencies not included with the package contrib: List of Postgres contrib or development packagesvariations: A list of dependency variations, each with two fields:
where: Specifies a configuration that, when matched, includes the dependencies. For example, to specify alternate dependencies for Linux arm64, it would be"platforms": { "linux": "arm64" }
. For a specific
version of Postgres, it would be something like
"postgres": { "version": ">= 16, < 17" }
. [This likely needs some
deep thinking through.]
dependencies: An object containing of the other key structures in
this object, defining the additional dependencies for environments
that match the where
field.
The packages
and external
keys point to objects in which the keys are
dependency names and the values are version range expressions (empty string
means any version). The postgres.version
field is also a version range
expression.
The contrib
key points to an array of Postgres-supplied apps and extensions.
Versions are not necessary because they effectively have the same version as a
supported Postgres version.
Example:
{
"postgres": {
"version": ">= 12, < 17",
"with": [ "xml", "uuid", "perl" ]
},
"pipeline": "pgrx",
"platforms": {
"linux": [ "amd64", "arm64" ],
"darwin": [ "amd64", "arm64" ],
"windows": [ "amd64" ],
"freebsd": [ "amd64" ]
},
"dependencies": {
"configure": {
"requires": {
"external": { "cargo-pgrx": "" }
}
},
"build": {
"requires": {
"external": {
"curl": "",
"awk": "",
"sed": "",
"perl": "5.20"
}
},
"recommends": {
"external": {
"jq": "",
"perl": "5.38"
}
}
},
"test": {
"requires": {
"contrib": [ "pg_regress", "plpgsql", "plperl" ],
"packages": {
"github.com/theory/pgtap": "1.1.0"
}
}
},
"run": {
"requires": {
"contrib": [ "plperl" ],
"packages": {
"github.com/theory/hostname": ""
}
}
}
}
}
Notes:
The external
field is the tricky one. How does one provide a canonical
dependency for a third-party library or app that may have loads of different
names on different platforms, different packaging systems (apt vs rpm), and
even different versions of them, or split apart for development and runtime
use? Some possibilities:
Other notes:
Decide on a version range standards to adopt. Start with the PGXN version range, pep-0508, npm ranges, and Gem::Version. Ispipeline
really necessary, given configure requirements? I think so,
because it tells the client the preferred build system to use, in case it
can’t detect it for some reason.
PGXN Compatibility:
Significantly differs from the existing prereqs
object, so this one is keyed
under dependencies
and we can support either.
Classification metadata lets the extension developer associate additional information to improve discovery, such as key words. It might also allow selections from a curated list of extension classifications, such as the category slugs supported for the cargo categories field.
Ecosystem apps use this data to organize extensions under key words or categories, making it easier for users to find extensions that are frequently used together or for specific workloads or tasks.
Example:
{
"tags": [
"testing",
"pair",
"parameter"
],
"categories": [
"Machine Learning"
]
}
PGXN Compatibility:
Conflicts with the existing root-level tags
array, but we can support either
one.
A hand-coded example that attempts to capture all the complexity of the pgml extension:
{
"package": "github.com/postgresml/postgresml/pgml-extension",
"name": "pgml",
"version": "2.8.2",
"abstract": "pgml: Created by the PostgresML team",
"maintainers": [
{
"name": "the PostgresML team",
"url": "https://github.com/postgresml/postgresml/"
}
],
"license": "MIT",
"spec": {
"version": "2.0.0",
"url": "https://pgxn.org/meta/spec.txt"
},
"artifacts": [
{
"type": "source zip",
"url": "https://github.com/postgresml/postgresml/archive/refs/tags/v2.8.2.zip",
"sha256": "2b9d2416096d2930be51e5332b70bcd97846947777a93e4a3d65fe1b5fd7b004"
},
{
"type": "source tgz",
"url": "https://github.com/postgresml/postgresml/archive/refs/tags/v2.8.2.tar.gz",
"sha256": "845f28339c6159ac32daccea1cd17b386ea083c3e60bb8d58fb737725afe7eb5"
}
],
"resources": {
"homepage": "https://postgresml.org/",
"issues": "https://github.com/postgresml/postgresml/issues",
"documentation": "https://postgresml.org/docs/",
"support": "https://discord.com/invite/DmyJP3qJ7U",
"repository": "https://github.com/postgresml/postgresml",
"badges": [
{
"alt": "Tests Passing",
"src": "https://github.com/postgresml/postgresml/actions/workflows/ci.yml/badge.svg"
},
{
"alt": "Chat Activity",
"src": "https://camo.githubusercontent.com/1988e7aaaa6a0c8ddcf880faacf1ec3263e23fac4aeff4710922082fde84442a/68747470733a2f2f696d672e736869656c64732e696f2f646973636f72642f31303133383638323433303336393330303939",
"link": "https://discord.gg/DmyJP3qJ7U"
}
]
},
"manifest": {
"extensions": {
"pgml": {
"sql": "sql/pgml--2.8.2.sql",
"preload": "shared",
"tle": false,
"control": {
"comment": "pgml: Created by the PostgresML team",
"default_version": "2.8.2",
"module_pathname": "$libdir/pgml",
"relocatable": false,
"superuser": true,
"schema": "pgml",
"trusted": true
}
}
}
},
"dependencies": {
"postgres": {
"version": ">= 15, < 16"
},
"pipeline": "pgxs",
"platforms": {
"linux": [
"amd64",
"arm64"
],
"darwin": [
"amd64",
"arm64"
]
},
"dependencies": {
"configure": {
"requires": {
"external": {
"cargo-pgrx": "",
"bison": "",
"cmake": "",
"flex": "",
"libclang-dev": "",
"libopenblas-dev": "",
"libpython3-dev": "",
"libreadline-dev": "",
"libssl-dev": "",
"pkg-config": ""
}
}
},
"run": {
"requires": {
"external": {
"libopenblas": "",
"libpython3": "",
"libreadline": "",
"libssl": "",
"python3": ""
}
},
"recommends": {
"external": {
"python(pyarrow)": "=11.0.0",
"python(catboost)": "",
"python(lightgbm)": "",
"python(torch)": "",
"python(torchaudio)": "",
"python(torchvision)": "",
"python(xgboost)": "",
"python(accelerate)": "",
"python(bitsandbytes)": "",
"python(ctransformers)": "",
"python(huggingface-hub)": "",
"python(deepspeed)": "",
"python(einops)": "",
"python(optimum)": "",
"python(peft)": "",
"python(tokenizers)": "",
"python(transformers)": "",
"python(transformers-stream-generator)": "",
"python(InstructorEmbedding)": "",
"python(sentence-transformers)": "",
"python(rouge)": "",
"python(sacrebleu)": "",
"python(sacremoses)": "",
"python(datasets)": "",
"python(orjson)": "",
"python(langchain)": ""
}
}
}
},
"variations": [
{
"where": {
"platforms": {
"linux": []
}
},
"dependencies": {
"prereqs": {
"run": {
"recommends": {
"external": {
"python(auto-gptq)": "",
"python(xformers)": ""
}
}
}
}
}
}
]
},
"classification": {
"tags": [
"machine learning",
"ml",
"transformers"
],
"categories": [
"Machine Learning"
]
}
}
Note that PostgresML also provides a dashboard app, but it does not ship with the extension, so it’s not listed here. It could have its own package metadata and be separately distributed.
For the Python dependencies, in the absence of a neutral standard for
specifying package, here they’re specified using a syntax borrowed from RPM to
make clear that they’re Python dependencies. A couple of those dependencies
are Linux-only, so listed in variations
where the OS is Linux.
The second metadata format is registry metadata. It starts by with a copy the package metadata, but builds from there, adding information (such as the artifact link, SHA, and cryptographic signature from the registry itself) and sections (defined below).
Providing a separate metadata format enables broader community collaboration to augment the metadata for extension packages.
Ideally an ecosystem of trusted services will be developed by various parties, who will report back to the root registry as the source of record for all metadata about extension packages. For example, a binary distribution service like apt.postgresql.org or trunk will submit links and checksums for binary packages and perhaps periodic download stats.
Broadly speaking, there are three typologies for registry metadata: Distributions, Reports, and Classifications.
DistributionsA list of links and checksums for downloading binary distributions for the extension package. The root registry page for the package can therefore list all the ways to install an extension and expand the list as new binary registries add it.
Along with the Artifacts package metadata, the distribution metadata allows installer tools to select the best option to install based on the OS, Postgres version, and cluster configuration (e.g., install the community Apt package if the cluster was installed from the community Apt registry).
Each item must have:
Registry: The name of the distribution registry, unique Type: The type of distribution: Apt, RPM, TLE, etc. URL: Link to the the registry’s page for the distribution Command: In lieu of a URL and SHA it can provide instructions to install the distribution, e.g.,apt-get install postgresql16-pair
.
Each distribution registry must be trusted by the root registry to submit this information to add to the metadata, and have its own page with additional contextual information: its home page, docs, basic instructions, whatever. A README of sorts.
Example:
[
{
"registry": "trunk.pgxn.org",
"type": "trunk",
"command": "pgxn install github.com/example/pair"
},
{
"registry": "apt.postgresql.org",
"type": "apt",
"command": "apt-get install postgresql16-pair"
},
{
"registry": "yum.postgresql.org",
"type": "rpm",
"command": "yum install postgresql16-pair"
},
{
"registry": "pgt.dev",
"type": "apt",
"url": "https://pgt.dev/extensions/pair",
"command": "trunk install pair"
},
{
"registry": "pgxman.com",
"type": "apt",
"url": "https://pgxman.com/x/pair",
"command": "pgxman install pair"
},
{
"registry": "database.dev",
"type": "sql",
"url": "https://database.dev/example/pair",
"command": "SELECT dbdev.install('example-pair');"
},
]
Other Options:
Information about installing from a registry that doesn’t offer URLs, like Homebrew, where one just needs to know what the formula is called. Maybe the same should apply to Apt? Metrics and ReportsThis object includes data such as number of downloads, build and test status on various Postgres/OS/version combinations, binary packaging distributions, test coverage, security certification, vulnerability detection, quality metrics and user ratings, and more. Some of these bits of data might include badges, e.g., for ratings or security assessments.
In the broader ecosystem, it would be the responsibility of the root registry to ensure that data comes only from trusted sources. However this data will be publicly readable, allowing any downstream applications to extend and publish metrics and reports with their own information.
Example:
{
"aggregates": {
"downloads": 32
},
"sources": {
"smoke-tester.example.com": {
"stats": {
"passes": 32,
"fails": 8,
"unknown": 0
},
"link": "https://smoke-tester.example.com/extension/pair",
"badge": "https://smoke-tester.example.com/extension/pair.svg"
},
"pgt.dev": {
"downloads": 12,
"tags": ["two", "kv"],
"categories": ["Analytics"]
},
"pgxman.com": {
"downloads": 20
},
"ratings.example.com": {
"stats": {
"ratings": 324,
"average": 3.2,
"comments": 13
},
"link": "https://ratings.example.com/extension/pair",
"badge": "https://ratings.example.com/extension/pair.svg"
},
"stats.example.com": {
"contributors": 2,
"stars": 14,
"last_active": "2024-01-12",
},
"security-scan.example.com": {
"advisories": {
"high": 2,
"moderate": 11,
"low": 1,
"cves": 0
},
"link": "https://security-scan.example.com/extension/pair",
"badge": "https://security-scan.example.com/extension/pair.svg"
}
}
}
Notes:
The aggregates
section aggregates results from multiple sources, for
example summing all downloads or averaging ratings. The list of items to
aggregate could evolve regularly.
Each key in sources
identifies a trusted downstream source of
information. Each would have its own schema describing its objects and
their meaning, along with URI templates to link to. For example,
stats.example.com
might have these templates:
{
"contributors": {
"alt": "List of people who have contributed to the {name} project",
"badge": "https://stats.example.com/{ package }/contributors.svg",
"uri": "https://stats.example.com/{ package }/contributors"
},
"stars": {
"alt": "Star ratings for the {name} project",
"badge": "https://stats.example.com/{ package }/stars.svg",
"uri": "https://stats.example.com/{ package }/stars"
}
}
Might be worth adopting more of an API of multiple files, rather than one big file. Example: Homebrew analytics. Note also its support for date ranges for analytics (30/90/365 days, stats when directly installed vs. installed as a dependency).
More about… Postgres PGXN Extensions Packaging MetadataMy co-authors and I published updated versions of eight specifications in preparation for IETF 119 in Brisbane. The specifications span three working groups: JOSE, COSE, and OAuth. The updated specifications and outcomes when discussed at IETF 119 are as follows.
1, 2, & 3: JSON Web Proof, JSON Proof Algorithms, and JSON Proof Token. Updates were:
Normatively defined header parameters used Populated IANA Considerations sections Allowed proof representations to contain multiple base64url-encoded parts Specified representation of zero-length disclosed payloads Added Terminology sections Updated to use draft-irtf-cfrg-bbs-signatures-05 Updated to use draft-ietf-cose-bls-key-representations-04 More and better examples Improvements resulting from a full proofreadingContinued reviews and feedback from implementations are requested.
4: Fully-Specified Algorithms for JOSE and COSE. Updates were:
Published initial working group document following adoption Added text on fully-specified computations using multiple algorithms Added text on KEMs and encapsulated keys Updated instructions to the designated expertsIt was agreed during the JOSE meeting to describe what fully-specified algorithms for ECDH would look like, for consideration by the working group.
5: OAuth 2.0 Protected Resource Metadata. Updates were:
Switched from concatenating.well-known
to the end of the resource identifier to inserting it between the host and path components of it
Have WWW-Authenticate
return resource_metadata
URL rather than resource
identifier
It was decided to start working group last call during the OAuth meeting.
6: COSE “typ” (type) Header Parameter. Updates were:
Added language about media type parameters Addressed working group last call comments Changed requested assignment from 14 to 16 due to conflict with a new assignment Addressed GENART, OPSDIR, and SECDIR review commentsThis document is scheduled for the April 4, 2024 IESG telechat.
7: Barreto-Lynn-Scott Elliptic Curve Key Representations for JOSE and COSE. Updates were:
Changed to use key typeEC
for JOSE and equivalent EC2
for COSE for uncompressed key representations
Changed identifier spellings from “Bls” to “BLS”, since these letters are people’s initials
We received feedback to not add compressed key representations to the draft.
8: Use of Hybrid Public-Key Encryption (HPKE) with JavaScript Object Signing and Encryption (JOSE). Updates were:
Use existing"alg": "dir"
value for HPKE Direct Encryption mode
Aligned choices more closely with those of Use of Hybrid Public-Key Encryption (HPKE) with CBOR Object Signing and Encryption (COSE)
Defined both Integrated Encryption mode and Key Encryption mode
Added IANA Considerations section
Removed Post-Quantum Considerations
It was decided to start a working group call for adoption during the JOSE meeting.
Thanks to all who contributed to the progress made on these specifications, both before and during IETF 119!
After we came to Bloomington in the summer of 2021, we rented an apartment by Prospect Hill, a quiet dome of old houses just west of downtown. There we were surprised to hear, nearly every night, as many police and ambulance sirens as we’d heard in our Manhattan apartment. Helicopters too. Soon we realized why: the city’s hospital was right across 2nd Street, a couple blocks away. In 2022, the beautiful new IU Health Bloomington Hospital opened up on the far side of town, and the sounds of sirens were replaced by the sounds of heavy machinery slowly tearing the old place down.
Being a photographer and a news junkie, I thought it would be a good idea to shoot the place often, to compile a chronicle of demolition and replacement, as I had done for the transition of Hollywood Park to SoFi Stadium in Inglewood, California. But I was too busy doing other things, and all I got was that photo above, which I think Dave Askins would categorize as a small contribution to topical history.
Dave is a highly productive local journalist, and—by grace of providence for a lifelong student of journalism such as me—the deepest and most original thinker I know on the topic of what local news needs in our time—and going forward. I’ve shared some of Dave’s other ideas (and work) in the News Commons series, but this time I’m turning a whole post over to him. Dig:::::
In the same way that every little place in America used to have a printed newspaper, every little place in America could have an online local chronicle.
Broadly speaking, an online local chronicle is a collection of facts organized mostly in chronological order. The “pages” of the chronicle can be thought of as subsets of a community’s universal timeline of events. These online local chronicles could become the backbone of local news operations.
First a word about what a local chronicle is not. It is not an online encyclopedia about the little place. It’s not a comprehensive history of the place in any conventional sense. Why should it not try to be those things? Because those things are too hard to think about building from scratch. Where would you even start?
It is at least conceivable that an online local chronicle could be built from scratch because you start by adding new facts that are newsworthy today. A new fact added to the chronicle is a touchstone, about which anyone can reasonably ask: What came just before that?
A working journalist in a little place with an online local chronicle will be in a good position to do two things: (1) add new facts to the local chronicle (2) help define sets of old facts that would be useful to include in the online local chronicle.
A journalist who is reporting the news for a little place would think not just about writing a report of new facts for readers today. They would keep this question in mind: What collection of old facts, if they were included in the local chronicle, would have made this news report easier to write?
Here’s a concrete example. A recent news report written for The B Square Bulletin included a mention of a planned new jail for Monroe County. It included a final sentence meant to give readers, who might be new to that particular topic, a sense of the basic reason why anyone was thinking about building a new jail: “A consultant’s report from two and a half years ago concluded that the current jail is failing to provide constitutional levels of care.”
About that sentence, a reader left the following comment on the website: “I know it’s the last sentence in an otherwise informative article, but at the risk of nit-picking that sentence seems inadequate to the task of explaining the context of the notion of a new jail and the role of the federal court and the ACLU.”
The comment continues: “It would have been better, Dave, to link to your own excellent previous work: https://bsquarebulletin.com/2023/02/20/monroe-county-sheriff-commissioners-square-off-at-committee-meeting-aclu-lawyer-says-look-you-need-a-new-jail-everyone-knows-that/”
What this reader did was to identify a set of old facts that should be a collection (a page) in Bloomington’s local chronicle.
It’s one thing to identify a need to add a specific collection of facts to the local chronicle. It’s quite another to figure out who might do that. Working journalists might have time to add a new fact or two. But to expect working journalists to add all the old sets of facts would, I think, be too tall an order.
The idea would be to recruit volunteers to do the work of adding old facts to the online local chronicle. They could be drawn from various segments of the community—including groups that have an interest in seeing the old facts about a particular topic not just preserved, but used by working journalists to help report new facts.
I think many community efforts to build a comprehensive community encyclopedia have foundered, because the motivation to make a contribution to the effort is mostly philosophical: History is generally good to preserve.
The motivation for helping to build the online local chronicle is not some general sense of good purpose. Rather it is to help working journalists provide useful facts for anyone who in the community who is trying to make a decision.
That includes elected leaders. They might want to know what the reasons were at the time for building the current jail at the spot where it is now.
Decision makers include voters, who might be trying to decide which candidate to support.
Decision makers also include rank-and-file residents—who might be trying to decide where to go out for dinner and want to know what the history of health inspections for a particular restaurant are.
For the online local chronicle I have set up for Bloomington, there are very few pages so far. They are meant to illustrate the general concept:
https://thebloomingtonchronicle.org/index.php/Main_PageThe pages are long on facts that are organized in chronological order, and short on narrative. Here are the categories that include at least one page.
Category: People
Category: Data
Category: Boards, Commissions, Councils
Category: List
Category: Topical History
==========
–Dave
By the way, if you’d like to help local journalism out, read more about what Dave is up to—and what he needs—here: https://bsquarebulletin.com/about/
A few weeks ago I brainstormed about decentralized Postgres extension publishing, inspired in part by an examination of Go decentralized publishing. It was…a lot. I’ve been deeply pondering the future of PGXN and the broader extension ecosystem, and want to start to nail down some decisions. To that end, I’d like to propose an update to extension namespacing.
Status QuoThere are currently three ways in which an extension is considered unique:
Only one extension can have a given name within a single Postgres cluster. Names are defined by the name of the control file. It is therefore not possible to have two extensions with the same name in the same Postgres cluster. PGXN follows this pattern: Only one extension can have a given name in the PGXN registry. The first person to release an extension then “owns” its name, and no one else can release an extension with the same name.1 I think dbdev follows the same pattern. Other registries like trunk and pgxman define an extension by the distribution name, at least for the purposes of selecting a binary to install. Thus when youtrunk install postgis
, you get all of the
extensions included, as you’d expect, while
trunk install address_standardizer
wouldn’t work at all. In the few places
that trunk
supports installation by extension name, it prompts the user to
use the appropriate package name if there’s a conflict.
A Modest Proposal
I’d like to propose the following changes to the PGXN Meta Spec to start to move away from extension uniqueness in the broader extension ecosystem and more toward package name.
Add a new field, call itmodule_path
, project_path
, project_uri
, that,
if present, uniquely identifies an extension project and all of its parts.
It should be to a Go-style module path (or URI) that identifies the
project repository path where a META.json
file lives.
Retain the provides
object where keys identify extensions, but those
keys will no longer be globally unique to the registry. In other words, the
combination of module_path
and extension name uniquely identifies an
extension, including an empty module_path
.
How it Works
Some examples. Let’s say there is an existing extension named pair
, included
in the distribution named pg_pair
:
{
"name": "pg_pair",
"version": "1.2.3",
"provides": {
"pair": {
"file": "pair.sql",
"version": "1.2.0"
}
}
}
The extension name pair
is unique, and pgxn install pair
will download
the pg_pair v1.2.3 bundle and compile and install pair v1.2.0.
Now someone else comes along and wants to make their own pair with this metadata:
{
"name": "my_pair",
"version": "0.2.3",
"provides": {
"pair": {
"file": "pair.sql",
"version": "0.2.3"
}
}
}
Just like today, this upload would be rejected, because there is already a
registered pair
extension. Under my proposal, they can disambiguate by
providing a module_path
:
{
"name": "my_pair",
"module_path": "github/example/pair",
"version": "0.2.3",
"provides": {
"pair": {
"file": "pair.sql",
"version": "0.2.3"
}
}
}
This upload would be allowed. With these two releases, someone attempting to
install pair
would see something like this:
$ pgxn install pair
ERROR: Duplicate extension name “pair”. Install one of these instead:
* pgxn.org/dist/pair
* github/example/pair
Note the the module path pgxn.org/dist/pair
in the the first option. This is
the default module path for distributions without a module path.2 But
now the user can select the proper one to install:
$ pgxn install pgxn.org/dist/pair
INFO: latest version: pgxn.org/dist/pair@1.2.3
INFO: building extension
INFO: installing extension
INFO: done!
Furthermore, the PGXN client will prevent the user from later installing a conflicting extension. The failure would look something like:
$ pgxn install github/example/pair
INFO: latest version: pgxn.org/dist/pair@0.2.3
ERROR: Cannot install extension “pair” from pgxn.org/dist/pair:
ERROR: A conflicting extension named “pair” is already installed
ERROR: from pgxn.org/dist/pair
Features with Benefits
I see a number of benefits to this change:
Compatibility with the v1 metadata spec, so that no data migration or distribution indexing is required. It loosens up extension namespacing (or name registration, if you prefer) while adding additional metadata to help users evaluate the quality of an extension. For example, does it come from a well-known developer? You can see it right in the module path. It creates a pattern to eventually allow auto-indexing of extensions. For example, if you runpgxn install github.com/example/pew
, and PGXN doesn’t
have it, it can look for a META.json
file in that repository and, if it
exists, and there’s a semver release tag, it could try to index it and let
the user install it. There are ownership issues to be worked out, but it
has possibilities.
It preserves the Postgres core concept of extension identity while putting
in place a well-established (by Go modules and widespread use of URIs in
general) that the Postgres core could eventually adopt to allow more
flexible extension namespacing.
Request for Comments
What do you think? Good idea? Terrible idea? Please hit me with your thoughts on Mastodon, or via the #extensions channel on the Postgres Slack. I’d like to get this decision (and a few others, stay tuned!) nailed down soon and start development, so don’t hesitate? I need your help to prevent me from making a huge mistake.
Unless the owner would like to share ownership with someone else, in which case they can email me to request that another user be granted “co-ownership”. They can also request to transfer ownership to another user, after which the original owner will no longer be able to release the extension. ↩︎
Or, if the META.json
file has a repository resource with a
URL, PGXN could index it as the implied module path. Or, failing that, maybe
it should fall back on the distribution name instead of a pgxn.org
path,
and prompt with pg_pair/pair
. ↩︎
ファイルサーバをほっていたら、XNS Tecnical Specs version 1.0(2002年7月9日版) が出てきたのでここに貼っておきます。
XDI.orgのサイトが生きていればそこからダウンロードできればよかったのですが、サイトが放棄されてしまっているので、わたしが代わりに置き場所を提供します。
XNSは後にXRI/XDIになり、OpenID 2.0のコア仕様となった技術です。現在のDIDもその延長線上にあります。わりと参考になることが色々あると思います。昨今分散IDとかいう人はこれは読んでおいても良い仕様かとおもいます。
XNS_Technical_SpecsダウンロードNHKの放送した番組というのは国民の財産なわけですが、過去に放映した番組がわからないと、その財産のありかもわからないことになります。そのためには、番組についてのメタデータが検索できるようになっているのが望ましいですが、その第一歩として、過去の番組表が検索できるだけでもだいぶ違います。
現在のNHKのメインサイト「nhk.jp」ではサイトトップから番組表検索へのリンクがありますが、これでは直近分しか検索することができません。これに対してNHKクロニクルでは過去分を検索することができます。
【図表】NHKクロニクルでは過去の番組表を検索できる(出所)https://www.nhk.or.jp/archives/chronicle/ためしに、サイトウ・キネンと入れて検索してみましょう。すると、どさっと大量の検索結果が逆時系列で出てきます。わたしが知りたかったのは2007年に放映されたことを覚えている番組なので、そのあたりまで時系列を遡っていきます。
【図表】14ページ目で2007年に到達今回は右上のページ表示をクリックして徐々に遡っていきましたが、URLに「&page=14」のように記入することによってページを飛ぶこともできるようです。こうやっていって、わたしが知りたかった番組は2007年12月29日に放映されていたことがわかりました。
【図表】知りたかった番組は2007年12月29日のっぽいといっても、上記の画像からわかるように、このページだけではどんな番組だったかわかりません。なので、「番組詳細」を順番にクリックしていってこの番組だとわかったのでした。
【図表】詳細をクリックしていって番組を発見 (出所)https://www.nhk.or.jp/archives/chronicle/detail/?crnid=A200712291800001301000これ、実はサイトウ・キネン・フェスティバルでデュティユー自身も来日して「Le Temps l’Horloge」が初演された録画でとても貴重なものです。作曲者自身が拍手しているところが写っています。また、「瞬間の神秘」も録画されているのはなかなか珍しいのではないかと思います。
この詳細ページのURLは「https://www.nhk.or.jp/archives/chronicle/detail/?crnid=A200712291800001301000」となっています。このcrnid というのがこのコンテンツの識別子のようです。(←ちゃんとIDネタを振った!)
放送時期がよくわからなかったらどうするか:=>&検索もできる!上記では、放送時期をわたしが覚えていたので遡っていって検索するという技がつかえました。これだと放送時期がわからないと検索がすごく大変になります。ここで「デュティユー サイトウ・キネン」のように&検索ができればぐっと対象を絞れます。なので、やってみましたらできました。半角スペースでキーワードを区切って検索すればよいようです。
【図表】&検索も可能 (出所)https://www.nhk.or.jp/archives/chronicle/search/?keyword=デュティユー%E3%80%80サイトウ・キネンこの年2回放映されていることがわかります。わたしが実際に見たのは9月8日の生中継の方ですね。実際に演奏された直後なので。番組詳細を見てみます。
【図表】こちらのほうが番組詳細が詳しい (出所)https://www.nhk.or.jp/archives/chronicle/detail/?crnid=A200709081900001301000なんと、こちらのほうが番組詳細が詳しい!「瞬間の神秘」がそれだけでなく、正式タイトル「瞬間の神秘(24の弦楽器、ツィンバロムと打楽器のための)1」としてのっています。12月のは再放送なので内容は同じはずですが、番組情報には差があるのですね。一つ一つ見に行ったほうが良さそうです。
Google検索ではひっかからないこのように便利に検索できるNHKクロニクルですが、残念なことがひとつあります。それはGoogle検索でひっかからないということです。試しに「site:www.nhk.or.jp 瞬間の神秘(24の弦楽器、ツィンバロムと打楽器のための)」と検索してみて下さい。2024年3月18日現在、何も返ってきません。これは、robots.txt でクローリングが禁止されているからですね。
User-agent: *
【図表】chronicleがrobots.txtで禁止されている (出所)https://www.nhk.or.jp/robots.txt
Disallow: /*.cgi$
Disallow: /*.cgi?
Disallow: /*/api/
(中略)
Disallow: /chronicle/
国民の利便性という観点ではちょっと残念な気がします。ここはallowしてほしいところです。2
というわけで、NHKクロニクルが便利。でもGoogleからは検索できないので直接NHKクロニクルに行って検索しないとだめ、というお話でした。あと、こうして検索したものにどうやったらアクセスできるようになるかですね。デジタル化の進展だけでなく権利処理の話も関わってきますが、このあたり、アクセスしやすくなるとよいですね。
Update: 2024-03-22: Slides and video linked below.
This week, my fellow Tembonaut Ian Stanton will present the extension ecosystem mini-summit talk, “Building Trunk: A Postgres Extension Registry and CLI”. We felt it important to get some insight from a couple of the recently-developed Postgres extension registries: what problems they set out to solve, how the were built and operate, successes addressing their issues, and what issues remain, both for the projects and the ecosystem overall. Ian plans to give us the low-down on trunk.
Join us! Need other information or just want an invitation
without using Eventbrite, hit me up at david@
this domain, on Mastodon, or
via the #extensions channel on the Postgres Slack.
Update: 2024-03-22: Such a great discussion, was great! Missed it? I got you. Links:
Video PDF Slides Notes More about… Postgres Trunk Extensions PGConf Summitわたしの顧問先のPwC Japanグループ主催のDigital Identity and Privacy Tech Forum 2024【配信期間:2024年3月11日(月)~2024年4月30日(火)】に出演しています。同フォーラムは、「デジタル化に伴うプライバシー保護領域の重要テーマであるデジタルアイデンティティとプライバシーテックの最新動向について詳しく解説」するオンライン・オンデマンドのフォーラムで、いくつかのセッションが提供されています。
デジタル社会の信頼を構築する「デジタルID」トラスト、日本のデジタルIDのこれから デジタルアイデンティティを支える技術―ソリューション導入の失敗事例と成功事例― プライバシーガバナンス最前線―データ利活用を促進していくために、いま企業に求められていること― プライバシーテックの活用と今後の展望わたしが出演しているのは、このセッションを企画したPwCコンサルティング合同会社 ディレクター 柴田健久率いる、【1. デジタル社会の信頼を構築する「デジタルID」トラスト、日本のデジタルIDのこれから】です。
セッションの参加者ご一緒させていただいたのは、
デジタル庁セキュリティアーキテクト 満塩尚史 OIX チーフ・アイデンティティ・ストラジテスト ニック・マザショウ PwCコンサルティング合同会社 ディレクター 柴田健久の3氏に、パーソナリティとしてフォーブスジャパンの藤吉氏が出演しておられます。
内容は
「デジタルアイデンティティの概念」 「グローバルレベルでの、デジタルIDトラストの標準化の動き」 「日本の政府関係者のIDエコシステムへの期待」 「日本企業の実態と今後の活用に向けた議論」 「未来への展望」といったことについて各人の見解と意見交換を行っています
収録はLEDで壁や床が作ってあるスタジオ1でで、なかなか良い経験2をさせていただきました。最近の視力低下でカンペが全く見えない〜それどころか次のトピックも見えない状況でアドリブ力全開でやっていますが、いかがでしょうか。ぜひご覧になってご感想をお聞かせ願えればと思います。
なお、他の3セッションもタイトルからして非常に興味を惹かれるものです。まだわたしも見ていませんが、ぜひ時間を作って視聴したいと思っています。
視聴申込はこちらのページよりお願いいたします。
https://www.pwc.com/jp/ja/seminars/digital-identity-forum2024.html
Great turnout and discussion for the first in a series of community talks and discussions on the postgres extension ecosystem leading up to the Extension Ecosystem Summit at pgconf.dev on May 28. Thank you!
The talk, “State of the Extension Ecosystem”, was followed by 15 minutes or so of super interesting discussion. Here are the relevant links:
Video Keynote PDF SlidesFor posterity, I listened through my droning and tried to capture the general outline, posted here along with interspersed chat history and some relevant links. Apologies in advance for any inaccuracies or missed nuance; i’m happy to update these notes with your corrections.
And now, to the notes!
IntroductionIntroduced myself, first Mini Summit, six leading up to the in-person summit on May 28 at PGConf.dev in Vancouver, Canada.
Thought I would get it things started, provide a bit of history of extensions and context for what’s next.
PresentationPostgres has a long history of extensibility, originally using pure SQL or shared preload libraries. Used by a few early adopters, perhaps a couple dozen, including …
Explicit extension support added in Postgres 9.1 by Dimitri Fontaine, with
PGXS, CREATE EXTENSION
, and pg_dump
& pg_restore
support.
Example pair--1.0.0.sql
:
-- complain if script is sourced in psql and not CREATE EXTENSION
\echo Use "CREATE EXTENSION pair" to load this file. \quit
CREATE TYPE pair AS ( k text, v text );
CREATE FUNCTION pair(text, text)
RETURNS pair LANGUAGE SQL AS 'SELECT ROW($1, $2)::pair;';
CREATE OPERATOR ~> (LEFTARG = text, RIGHTARG = text, FUNCTION = pair);
Bagel makes an appearance.
Example pair.control
:
# pair extension
comment = 'A key/value pair data type'
default_version = '1.0'
module_pathname = '$libdir/pair'
relocatable = true
Example Makefile
:
EXTENSION = pair
MODULEDIR = $(EXTENSION)
DOCS = README.md
DATA = sql/pair--1.0.sql
TESTS = test/sql/base.sql
REGRESS = base
REGRESS_OPTS = --inputdir=test
MODULES = src/pair
PG_CONFIG ?= pg_config
PGXS := $(shell $(PG_CONFIG) --pgxs)
include $(PGXS)
Build and Install:
$ make
make: Nothing to be done for `all'.
$ make install
mkdir -p '/pgsql/share/extension'
mkdir -p '/pgsql/share/pair'
mkdir -p '/pgsql/share/doc/pair'
install -c -m 644 pair.control '/pgsql/share/extension/'
install -c -m 644 sql/pair--1.0.sql '/pgsql/share/pair/'
install -c -m 644 README.md '/pgsql/share/doc/pair/'
$ make installcheck
# +++ regress install-check in +++
# using postmaster on Unix socket, default port
ok 1 - base 15 ms
1..1
# All 1 tests passed.
CREATE EXTENSION
:
$ psql -d try -c 'CREATE EXTENSION pair'
CREATE EXTENSION
$ pg_dump -d try
--
-- Name: pair; Type: EXTENSION; Schema: -; Owner: -
--
CREATE EXTENSION IF NOT EXISTS pair WITH SCHEMA public;
--
-- Name: EXTENSION pair; Type: COMMENT; Schema: -; Owner:
--
COMMENT ON EXTENSION pair IS 'A key/value pair data type';
Many of us saw opportunity in this new feature.
PostgreSQL today is not merely a database, it’s an application development platform.
— Me, 2010
Proposed to build PGXN. Raised funds to build it in late 2010. Launched site April 2011; Daniele Varrazzo released CLI, and Dickson Guedes released the dev CLI.
Problems PGXN set out to solve:
Source code distribution with user registration and namespacing Discovery: Search, read docs, brows tags Installation: CLI to compile and install using PGXS or ConfigurePGXN Components:
Meta Spec Manager Root Mirror API Server Site Client Utils CLI CI/CD ImageProblems out of scope for PGXN:
Binary packaging and distribution Defer to apt/yum Developer tooling (though dev utils helped) Build tooling Defer to core (PGXS)PGXN Shortcomings:
Little development since 2012 Search limitations Docs preferred Most distributions have few docs Some issues addressed in last few weeks Source of Record Minority of available extensions on PGXN Releases uneven or neglectedIn classic SDLC fashion, PGXN POC shipped as an MVP and was neglected.
— Me, Just Now
Been peripheral to Postgres extensions for the last 10-12 years, but some things have happened.
Non-Core extension counts:
PGXN: 382 joelonsql/PostgreSQL-EXTENSIONs.md: 1,186Daniele asks about that last source, which is just a list in a gist.
Joe Nelson links to the gist in Zoom chat. It is not his list, contrary to my off-the-cuff guess
Why haven’t extensions taken off?
Lost Opportunities
No one canonical source to discover and install extensions Difficult to find and discover extensions without canonical registry Most extensions are under-documented and difficult to understand They are also hard to configure and install; most people don’t want or need a compiler The maturity of extensions can be difficult to gauge, not systematized, must each be independently researched David Christensen in Chat “attention economy/awareness, NIH, etc” Jeremy S in chat: “Maybe some people don’t know they are using extensions (I think that’s possible to some degree)” There is no comprehensive binary packaging Centralized source distribution is insufficient (even if it were complete) jubilee in chat: Trust aspect? David Johnson in chat: To seem legit you need to touch the repo at least annually to ensure it works on the newest major release. Even if you just do your compile and update the readme. I mention using pgxn-utils and GitHub workflows to ensure my extensions continue working There is insufficient developer tooling; pgxn-utils not well-maintained, don’t build on recent Rubies, but pgrx has a lot of Rust-oriented tooling Eric in chat: ❤️ jubilee in chat: 🦀 mentioned!Filling the Gaps
dbdev: “The Database Package Manager for Trusted Language Extensions”: Includes only TLEs, no binary extensions
trunk: “A Postgres Extension Registry”: Binary distribution of curated extensions, desires to be comprehensive and cross-platform
pgxman: “npm for PostgreSQL”: Binary Apt package distribution of curated extensions packaged with, desires to be comprehensive and cross-platform
Emphases: Ease of Use. Screenshot from pgxman:
$ curl -sfL https://install.pgx.sh | sh -
👏🎉 pgxman successfully installed
$ pgxman install pgvector
The following Debian packages will be installed:
postgresql-14-pgxman-pgvector=0.5.1
Do you want to continue? [Y/n] y
pgvector has been successfully installed.
Daniele in chat: “Missing a “curl | sudo sh” there…. 👀”
Greg Mullane (CrunchyData) [he/him] in chat: “Really not a fan of that
“pipe curl stuff from internet into sh” system.”
Jeremy S in chat: “Someone recently reprimanded me for putting curl |
psql in an extension README. From a security perspective it probably
sets a better example to do curl >file.sql … psql file.sql (encourage
users not to run from Internet but read/review first)” * jubilee in
chat: “apt/yum install is just a better UI over curl | sh :^)”
Jeremy S in chat: “Yes and once you’re to that point there’s already
more supply chain verification happening”
Jeremy S in chat: “It’s usually just the initial bootstrap into any
system, if the setup wasn’t already in your distro”
Emphases: Platform neutrality. Screenshot from trunk:
Architecture x86-64 Operating system Debian/Ubuntu
Emphases: Stats. Screenshot from dbdev:
Downloads20 all time downloads 0 downloads in last 30 days 1 download in last 90 days 0 downloads in last 180 days
Emphases: Curation. Screenshot from trunk:
Featured 7 Analytics 13 Auditing / Logging 7 Data Change Capture 6 Connectors 27 Data / Transformations 49Damien Clochard in chat: gtg, see you later guys !
MVPs
trunk: Manual integration, Currently Debian-only
pgxman: Form-based submission, Currently Apt-only
dbdev: TLEs only, CLI publishing
David Christensen in chat: “go has a pretty good extensions infra, imho, wrt discovery/docs, etc. also has the benefit of the package names being the URL to access it, which is a nice convention.”
New Opportunities Today
What are the community opportunities for the extension ecosystem?
Some ideas:
Improved dev tools: More than pgxn-utils and pgrx Canonical registry: All publicly-available extensions in one pac3 Easy publishing: auto-discovery or CI/CD pipeline publishing Continuous Delivery: CI/CD pipeline publishing File-free installation: TLEs Documentation: Something like Go docs or Rust docs File management: Put all the files for an extension in one directory Improved metadata Library Dependencies: utilities used by extensions Platform Dependencies: system packages Build pipelines: PGXS, pgrx, make, cpan, pypi, etc. Artifacts: Binaries build on release Classification: Curated in addition to tags Extension Types: Extensions, apps, background workers, loadable libraries Derived Services Binary Packaging: Distributed binaries for many platforms Ratings & Reviews: Stars, thumbs, comments Aggregated Stats: Repository stats, etc. Smoke Testing: Matrix of multi-platform test results Security Scanning: Reporting vulnerabilities Badging & Curation: Third-party classification, badging various statusesCollaborate to examine the ongoing work on PostgreSQL extension distribution, examine its challenges, identify questions, propose solutions, and agree on directions for execution.
🏔️ Your Summit Organizers
David Wheeler, Tembo, PGXN Jeremy Schneider, AWS, dsef David Christensen, Crunchy Data, pg_kaboom Keith Fiske, Crunchy Data, pg_partman Devrim Gündüz, EnterpriseDB, yum.postgresql.orgDevrim Gunduz in chat: Thanks David!
Schedule:
March 6: David Wheeler, PGXN: “State of the Extension Ecosystem” March 20: Ian Stanton, Tembo: “Building Trunk: A Postgres Extension Registry and CLI” April 3: Devrim Gündüz: “yum.postgresql.org and the challenges RPMifying extensions” April 17: Jonathan Katz: “TLE Vision and Specifics” May 1: Yurii Rashkovskii, Omnigres: “Universally buildable extensions: dev to prod” May 15: David Wheeler, PGXN: “Community Organizing Summit Topics”Ultimately want to talk about what’s important to you, the members of the community to make extensions successful.
DiscussionEric: I’m Eric Ridge, one of the developers behind pgrx, as you’re going through this process of building a modern extension ecosystem, let us know what we can do on the Rust side to help make your lives easier, we’re happy to help any way we can.
Steven Miller in chat:
These are some areas of interest we noticed building Tembo
Binary packaging / distribution:
Variable installation location System dependencies / uncommon system dependencies or versions Chip specific instructions (e.g. vector compiled with avx512) Extension-specific file types / extra data files (e.g. anonymizer .csv data)Turning on extensions automatically
Different ways to enable extensions does it need load (shared_preload_libraries, session_… etc)? Does it use create extension framework? Does it require a specific schema? What about turning on in multiple DBs at the same time in the same cluster, with background worker? Disabling, what data will be lost? Validating safety / user feedback on upgrade?In cloud / SaaS:
Installing + enabling extensions quickly, without restart Persisting extension files Extension-specific files (e.g. libraries) versus postgres’ libraries Updating Troubleshooting crashes / core dumpsAnyone else have similar problems / tips?
Steven Miller: These were just things I noted during the presentation. Curious if these are interesting to others on the call.
Daniele in chat: “Regards binary distributions, python wheels might be a useful reference.”
Steven Miller: That’s good point! What do people think of idea to just install extensions onto servers, not packages, persisted on the disk, next to PGDATA so they go into a single persistent volume, and the rest is managed by an immutable container.
Daniele: Had experience on Crunchy where we had to replace an image to get an extension. Looked for feature to have a sidecar or a volume with the extension.
Steven Miller: Didn’t have a separate directory just for extensions, it’s
just pg_config --libdir
fore everything. Had to persist entire directory,
including those files form the base build, their internal files. Would have
been nice to have a separate directory, extra-libdr or extra-sharedir,
something like that.
Yurii Rashkovskii: I was working on a patch to do exactly that, but haven’t completed it. Was going to introduce additional directories to search for this stuff.
Steven Miller: That would be really awesome.
Jeremy S in chat: “Advantage of that is that a single image can be shared among systems with different needs”
Eric in chat: “Thoughts around “enterprise repositories” that could be self-hosted and disconnected from the internet?”
Ian Stanton in chat: “I’ll touch on this in the next talk, it’s crossed our minds when building the Trunk registry”Steven Miller: I think that’s a great idea.
Bagel reappears.
David Wheeler: PGXN originally designed so anyone could run Manager and their own root mirror, and maybe rsync from the community one. Don’t know that anyone ever did, it’s a little complicated and most people don’t want to work with Perl. [Chuckles]. Definitely think there’s space for that. If you work with Java or Go or maybe Rust, lots of orgs like Artifactory that provide internal registries. Could be cool use case for Postgres extensions.
David Christensen in chat: “something that could support extension batches; like groups of related extensions that could be installed in bulk or loaded in bulk (so could accommodate the shared systems with different individual extension needs, but could be shared_preload_library configured)”
“Steven Miller” in chat: “Sounds familiar”
Greg Mullane (CrunchyData) [he/him] in chat: “All these items remind me of CPAN. We should see what things it (and other similar systems) get right and wrong. I’ve learned from CPAN that ratings, reviews, and badging are going to be very difficult.”
David Christensen in chat: “I’d assumed at the time that it was largely lifted (at least in philosophy/design) from CPAN. 🙂” David Wheeler (he/him) in chat: “yes”Jeremy S: I think this is mostly focused on developers, but I had recent experience where multiple people in the past few months, new to Postgres, are trying to understand extensions. They install a version and then see there are like 15 versions installed, so confused. Goes back to the install file. Bit of UX angle where there are sharp edges where people trying to make sense of extensions, the flexibility makes it hard to understand. Some might be some nice guides, some architectural things explaining PGXS, or improvements to make to the design. Related, not main topic, but good to keep end user UX and devs building on Postgres but not Postgres developers, who run their businesses.
David Wheeler: Yeah all the files can be confusing, which is why I think trunk and pgxman trying to simplify: Just run this command and then you have it.
Steven Miller in chat: “I really agree with what Jeremy is saying. Right now PGXN and Trunk are taking and approach like “whatever an extension could do / how it may work, it should work on this registry”. But I think more standards / “what is a normal extension” would make the UX much easier.”
Jeremy S: Even with that the available extensions view is still there. Some of that is just schema management, and that’s how core is doing schema management.
Steven Miller in chat: I exactly agree about the concern about multiple extensions within a package. Also version and name mismatches
David Wheeler: And not everything is an extension, you just want to work, or your extension is just utility like pg_top you just want to use. Extensions I think were a tremendous contribution to Postgres itself, but a lot of it was wrangling the existing system for building Postgres itself to make it work for that. Could be very interesting, though quite long term — and I know Dimitri has tried this multiple times — to build a proper package management system within Postgres itself, to eas a lot of that pain and burden.
Tobias Bussmann in chat: “Thank you for the great overview and for taking this topic further! Unfortunately, I’ll not be able to join at pgConf.dev but will follow whatever results this will lead to. As a package maintainer, I am constantly looking in a easy way to allow users to use extensions without having to package everything ;)”
Steven Miller in chat: “Like auto explain for example right. i.e. a LOAD only “extension””
*Yurii Rashkovskii: An interesting topic, what extensions are capable of doing and how they can be more self-contained. Like Steven was saying in chat: how easy is it to load and unload extensions. Example: want an extension to hook into a part of Postgres: executor, planner, etc. How do you go about enabling them? How you unload them, introspect, list of hooks.
Omni extension provides a list of all hooks, and when you remove an extension it removes the hooks that provide the extension, but still not part of the core. Hooks one of the greatest ways to expand the functionality of Postgres, allows us to experiment with Postgres before committing to the full cycle of getting a patch into Postgres. Lets us get it to users today to try. if it makes a lot of sense and people want it, time to commit to the process of getting a patch committed. But if we don’t hve this venue, how to get extensions in, our ability to try things is limited.
jubilee in chat: Hmm. It seems my audio is not working.
David Wheeler: The next session is two weeks from today: Ian Stanton is going to talk about “Building Trunk: A Postgres Extension Registry and CLI”. Will be interesting because a number of people have decided to build a binary packaging system for extensions, just to air out what the challenges were, what problems they wanted to solve, what problems remain, and where they want to take it in the future.
Jeremy S* in chat: “Bagel clearly has something to say”
*David Wheeler: jubileee I see your audio issues, do you just want to type your question into chat? We can also discuss things in the #extensions channel on the Postgres Slack
David Wheeler: Thank you all for coming!
jubilee in chat: “I had a question which is about: Does Postgres actually support docs for extensions? Like, a lot of people don’t really WANT to read a README. Can you get docstrings for a function in psql?”
Ian Stanton in chat: “Thank you David!”
jubilee in chat: And if not, why not?
Post Presentation Discussion David Wheeler: I see now that “jubilee” left their question in the Zoom chat. David Wheeler: The closest attempt at this I’ve seen is pg_readme, which will generate Markdown for an extension from comments in the catalog and write it out to a file. David G. Johnson: The comment on command adds in database comments that psql describe commands should display.Also on Slack, Greg Sabino Mullane started a longish thread on the things we want to do and build.
More about… Postgres PGXN Extensions PGConf Summit Bagelロンドン時間3月11日、英財務省は、マネーロンダリング規制 (マネロン規制, MLRs1) の有効性を向上させるためのパブコメ2を開始しました。このパブコメには、顧客を確認する際にデジタルアイデンティティの使用を最適にサポートする方法についての検討が含まれます。
マネロン規制は、金融サービス、不動産取引、ギャンブル、美術品の販売など、デジタルアイデンティティサービスを採用する可能性のあるさまざまな規制対象セクターをカバーしています。デジタルアイデンティティサービスは、マネーロンダリング防止チェックなどのマネロン規制要件を満たすために使用できますが、(英国において)認定されたデジタルアイデンティティサービスを規制要件を満たすためにどのように使用できるかについての具体的なガイダンスはありません。
昨年、英国科学・イノベーション・技術省3が開催したデジタルアイデンティティと属性の普及スプリント4では、英国のデジタルアイデンティティと属性信頼フレームワークとマネロン規制との関係が曖昧であることが、導入の障壁になっているという声が聞かれました。この関係を明確にすることで、重要なたくさんのユースケースにおいてデジタルアイデンティティの効率性と安全性の利点を活用し、経済犯罪との闘いを支援することができるでしょう。
信頼されたデジタルアイデンティティサービスの普及を可能にするための活動に携わっておられる各人・各団体におかれては、このパブコメに関心をもたれる向きも多いでしょう。このパブコメに回答をご希望の場合は、こちらから行うことができます。
パブコメは12週間で、2024年6月9日に締め切られます。OpenID Foundationではパブコメ提出のための準備に取り掛かっています。
In 2012 the Europeans released the first proposal for their General Data Protection Regulations (GDPR), which ran a four-year political gauntlet and evolved into its final, official version in 2016. Because of the ghastly surveillance situation we now find ourselves in America and elsewhere, perpetrated by both private industry and government, I think America also desperately needs comprehensive privacy rules, rules informed by now eight years of hindsight and infused with an American value not found in Europe’s GDPR: privacy from government.
If I could wave a magic wand, an American GDPR would improve on Europe’s GDPR in two fundamental ways:
1. Eliminate the idea that data about people can be “anonymized” or “de-identified”.Data about people can no longer be effectively de-identified by removing personally identifying information (PII) from the dataset. Maybe this was generally still possible in 2016, but not any more.
GDPR set off a wave of innovation among data scientists who, like the old game “Name That Tune”, took the new regulation as a challenge and developed numerous ways to re-identify people using only a few data points that aren’t considered PII, techniques they’ve since made widely available. And that was before the recent explosion of AI tools which make re-identification easy without the direct use of such tools. Unfortunately, fully GDPR-compliant “de-identified” data sets are now trivially easy to re-identify for anyone with access to a computer.
(For more details on this topic, see this Harvard paper about “simple” attacks that defeat GDPR’s PSO Secure / “singling out” privacy protection provisions.)
If almost any data about a person is usable to re-identify them, then the whole concept PII is no longer relevant; any data about a person is PII. That is precisely how an AGDPR definition of “personal data” can improve upon the European one: any data about an person. It’s both simpler and more accurate.
2. Include protections for privacy *from government*, in addition to privacy from the private sector.Europe’s GDPR specifically exempts government, AGDPR should do the opposite: it should bind government’s activities in relation to obtaining, using and retaining personal data.
There are obviously necessary and important reasons for government to obtain, use, and retain personal data, and those reasons should be explicitly enumerated and protected. But the U.S. federal government has gone absolutely bananas when it comes to how much of our personal data they are obtaining about us and our activities, through legal but largely unchallenged means. The degree to which it’s happening is way beyond what’s reasonably needed in a free country that espouses limited government, and that has a Fourth Amendment that secures:
“the right of the people to be secure in their persons, houses, papers, and effects, against unreasonable searches and seizures”.
How is data about us not our “effects”? How is government gathering our effects en masse without our explicit consent not “unreasonable”?
Journalist Byron Tau’s new book about U.S. government surveillance of law-abiding citizens, Means of Control, is outrageous. (Summary article here.) You cannot read it and not become deeply alarmed at the enormous, secret, ubiquitous surveillance state that already exists in the U.S. No doubt European governments are doing the same with their citizens’ data, but — and I’m generalizing here — they don’t have the same foundational distrust of government that the U.S. was born with and so may not be bothered enough to do anything about it, as evidenced by government’s total exemption from their GDPR. I hope, and believe, that once more Americans become aware of the extent of what’s happening, an American GDPR that clearly defines government’s role in privacy can become a real, politically viable possibility.
There is a model to follow. Christopher Bramwell, the Chief Privacy Officer of Utah, was the driving force behind a groundbreaking new piece of privacy legislation just enacted in Utah that constrains how personal data must be treated by government, without saying anything about private industry. As far as I know it’s the first of its kind, anywhere, and hopefully the first of many to follow in other states and countries. This bill gained a fortunate political boon as it advanced: it became a ‘vote against privacy’ to not support it; it passed unanimously.
Of course, privacy from big tech, surveillance capitalism, and private industry in general is a big deal, but private industry doesn’t have a monopoly on violence and taxes like government does; they mostly just want to sell me stuff. Still, the European GDPR shows an example of how to constrain private industry, and an American GDPR should learn from and incorporate those lessons.
The ideal outcome of an AGDPR would provide privacy both from private industry and from government, and finally put a halt to all federal activities of indiscriminate obtaining, using, and retaining of our personal digital “effects”, whether or not they’re considered PII.
The Accidental Superpower discusses a post-Bretton Woods world where easy, safe shipping cannot be assumed. I think in this "scared new world," renewables change the dynamics of energy flows by moving production closer to energy use.
My wife, Lynne, recently gave me a copy of Peter Zeihan's book, The Accidental Superpower: Ten Years On. The book was originally published in 2014, but Zeihan has updated it by inserting chapters talking about what he got right in 2014, what he got wrong, and why. The focus of the book is geopolitics—how geography and demographics shapes the world order—and how Bretton Woods changed that in significant ways. The book makes the case that so much of what made Bretton Woods useful to the US and why the US engaged with the rest of the world for the 70 years following World War II is changing. As it changes the free trade system enabled by Bretton Woods is also changing. This will have significant impact on every country in the world.
Much of what changes has to do with energy. One of the things1 Zeihan got right was his assertion that unlike much of the rest of the developed world, the US doesn't need to import energy—specifically oil—we are a net energy importer. This changes the dynamic wherein the US is willing to be the protector of shipping lanes for the entire world. As a result, the future could see a US that has the luxury of ignoring events in the Middle East, Ukraine, and elsewhere, whereas Europe (to take just one example) cannot. The book is full of other interesting predictions and conclusions just like this one. I encourage you to read it if you find this as fascinating as I do.
Zeihan makes a big deal of shale oil production, which accounted for 66% of US production in 2022. But as I read this, I was thinking about renewables. As I wrote in 2020, I've gone in big on solar power at my house, love my EV, and have replaced most things in the house (like the furnaces) with versions that run on electricity. I did this because it made my life easier and saves me money. The fact that it's good for the environment is a bonus.
But, solar and wind are not just renewable, they also allow energy production to be decentralized in ways oil and natural gas can't. Oil and natural gas deposits are where they are. Some countries are blessed with them and the others have to buy from those countries. And they're often far away, requiring shipping through potentially hostile waters. But that's not true of renewables. They can usually be built and located where ever the need is2. This changes geopolitical equation in significant ways, but areas of the world that are not energy independent, like Europe, are moving toward renewables too slowly to prevent future energy shocks. The problem with renewables is that they're long-lead items—they take years to plan and bring online.
Petroleum and Bretton Woods enabled the modern world, providing portable, storable sources of energy that could easily and safely move to where ever it was needed.3 If we are indeed at the end of the Bretton Woods era, the world is in for significant changes as it adjusts to a life where free trade, and easy access to petroleum-based energy, cannot be assumed. Moving energy production closer to the places it's used is one strategy for dealing with this world-altering disruption. Buckle up.
NotesThere are other things that are important to the books overall conclusion besides energy. I'm just cherry picking that because I was thinking about it. For example, the US is largely self-sufficient from an overall import/export standpoint. We don't import nearly as much as many other countries and could replace what we do import relatively easily.
It's not just renewables. Nuclear power can also be located closer to demand than an oil deposit. I started my career as a nuclear metallurgist, so I'm a fan. I think many countries are going to be sorry they've closed nuclear plants and made them too hard to construct profitably.
The feats of engineering that have enabled these energy flows is truly astounding.
Photo Credit: Oil Tanker at Sunset from Terski (Pixabay)
Sometimes it’s not okay to look down from the world.
Update: 2024-03-06: Slides and video linked below.
A quick reminder that I’ll be giving a brief talk on the “State of the Extension Ecosystem” on Wednesday at noon US Eastern / 17:00 UTC. This talk is the first in a series of community talks and discussions on the postgres extension ecosystem leading up to the Extension Ecosystem Summit at pgconf.dev on May 28.
I plan to give a brief history of Postgres extension tools and distribution, the challenges encountered, recent developments, and opportunities for the future. It should take about 30 minutes, followed by discussion. Following this pattern for all the talks in the series, I hope to set up some engaging discussions and to surface significant topics ahead of the summit.
Join us! Need other information or just want an invitation
without using Eventbrite, hit me up at david@
this domain, on Mastodon, or
via the #extensions channel on the Postgres Slack.
Update: 2024-03-06: Great turnout and discussion, thank you! Links:
Video Keynote PDF Slides Notes More about… Postgres PGXN Extensions PGConf SummitThis article shows how to onboard different clients or organizations in an ASP.NET Core application to use separated Azure blob containers with controlled access using security groups and RBAC applied roles. Each user in a client group can only access a single blob storage and has no access to blob containers belonging to different clients. Microsoft Entra ID is used to implement the blob storage access.
Code: https://github.com/damienbod/AspNetCoreEntraIdBlobStorage
Blogs in this series Using Blob storage from ASP.NET Core with Entra ID authentication Delegated read and application write access to blob storage using ASP.NET Core with Entra ID authentication Multi client blob storage access using ASP.NET Core with Entra ID authentication and RBAC Security context diagramThe web application uses different Enterprise applications to access the different Azure APIs. One Enterprise application is used to implement the blob application contributor access which can only be used from the application. This is used when a user in the application needs to write a file to the blob through the application. A second Enterprise application is used to create the RBAC access for the blob container reader access to the files. This is used when creating a new client or new organization. A third Enterprise application is used to implement the web application OpenID Connect web client. This is created using an Azure app registration and only allows delegated permissions. The user App roles are defined in this application. The Microsoft Graph APIs can be implemented using delegated permissions or application permissions. If using Graph application permissions to create or remove the groups, a separate Enterprise application is used to create the groups. You can also used the app service managed identity and use the service principal instead of the 3 enterprise applications to assign the required permissions.
Multi client blob storage setupThe Blob account uses Microsoft Entra ID to access the blob containers. The application can write to all containers and a security group is given RBAC blob container reader access, one per security group. Users are added to the security groups per client or per organization. The setup for the client blob container is implemented in three steps:
Create a Microsoft Entra ID security group Create an Azure storage blob container Create an RBAC to give the security group Blob storage reader permissions Create a Microsoft Entra ID security groupThe CreateSecurityGroupAsync method creates a new security group in Microsoft Entra ID. This is created using Microsoft Graph and returns the group with the group ID. The service uses application permissions and is implemented in a separate Enterprise application.
using System.Text; using Microsoft.Graph.Models; namespace MultiClientBlobStorage.Providers.GroupUserServices; public class ApplicationMsGraphService { private readonly GraphApplicationClientService _graphApplicationClientService; public ApplicationMsGraphService( GraphApplicationClientService graphApplicationClientService) { _graphApplicationClientService = graphApplicationClientService; } public async Task<Group?> CreateSecurityGroupAsync(string group) { var graphServiceClient = _graphApplicationClientService .GetGraphClientWithClientSecretCredential(); var formatted = RemoveSpecialCharacters(group); var groupName = $"blob-{formatted.Trim()}-{Guid.NewGuid()}".ToLower(); var requestBody = new Group { DisplayName = groupName, Description = $"Security group for all users from {groupName}", MailEnabled = false, MailNickname = formatted, SecurityEnabled = true }; var result = await graphServiceClient.Groups.PostAsync(requestBody); return result; } private string RemoveSpecialCharacters(string str) { var sb = new StringBuilder(); foreach (var c in str) { if (c is >= '0' and <= '9' || c is >= 'A' and <= 'Z' || c is >= 'a' and <= 'z' || c == '.' || c == '_') { sb.Append(c); } } return sb.ToString(); } } Create an Azure storage blob containerThe CreateContainer method creates a new Azure blob container using the BlobServiceClient from the Azure.Storage.Blobs nuget package.
private async Task<BlobContainerClient> CreateContainer(string name) { try { var formatted = RemoveSpecialCharacters(name); string containerName = $"blob-{formatted.Trim()}-{Guid.NewGuid()}" .ToLower(); var storage = _configuration.GetValue<string>("AzureStorage:Storage"); var credential = _clientSecretCredentialProvider .GetClientSecretCredential(); if (storage != null && credential != null) { var blobServiceClient = new BlobServiceClient(new Uri(storage), credential); var metadata = new Dictionary<string, string?> { { "name", name }, }; // Create the root container var blobContainerClient = await blobServiceClient .CreateBlobContainerAsync( containerName, PublicAccessType.None, metadata); if (blobContainerClient.Value.Exists()) { Console.WriteLine( $"Created container: {name} {blobContainerClient.Value.Name}"); } return blobContainerClient.Value; } throw new Exception($"Could not create container: {name}"); } catch (RequestFailedException e) { Console.WriteLine("HTTP error code {0}: {1}", e.Status, e.ErrorCode); Console.WriteLine(e.Message); throw; } } Create an RBAC to give the security group Blob storage reader permissionsThe ApplyReaderGroupToBlobContainer method creates an RBAC for the security group on the blob container itself. The group and the container were created in the previous steps and this takes an unknown length of time. Polly is used to repeat until the group and the container are ready and it creates the assignment.
public async Task ApplyReaderGroupToBlobContainer( BlobContainerClient blobContainer, string groupId) { var maxRetryAttempts = 20; var pauseBetweenFailures = TimeSpan.FromSeconds(3); var retryPolicy = Policy .Handle<Exception>() .WaitAndRetryAsync(maxRetryAttempts, i => pauseBetweenFailures); await retryPolicy.ExecuteAsync(async () => { // RBAC security group Blob data read await _azureMgmtClientService .StorageBlobDataReaderRoleAssignment(groupId, blobContainer.AccountName, blobContainer.Name); // NOTE service principal blob write is configured on root }); }Azure management REST API is used to create the RBAC. This rest API is implemented using a HttpClient and uses an Enterprise application to define the required permissions. This requires an administration Azure role and with this, you have full control of the Azure tenant.
using System.Net.Http.Headers; using System.Text.Json.Serialization; namespace MultiClientBlobStorage.Providers.Rbac; public class AzureMgmtClientService { private readonly AzureMgmtClientCredentialService _azureMgmtClientCredentialService; private readonly IHttpClientFactory _clientFactory; private readonly IConfiguration _configuration; private readonly ILogger<AzureMgmtClientService> _logger; public AzureMgmtClientService(AzureMgmtClientCredentialService azureMgmtClientCredentialService, IHttpClientFactory clientFactory, IConfiguration configuration, ILogger<AzureMgmtClientService> logger) { _azureMgmtClientCredentialService = azureMgmtClientCredentialService; _clientFactory = clientFactory; _configuration = configuration; _logger = logger; } /// <summary> /// Storage Blob Data Reader: ID: 2a2b9908-6ea1-4ae2-8e65-a410df84e7d1 /// Role assignment required for application in Azure on resource group /// https://learn.microsoft.com/en-us/rest/api/authorization/role-assignments/create-by-id?view=rest-authorization-2022-04-01&tabs=HTTP /// https://learn.microsoft.com/en-us/azure/role-based-access-control/role-assignments-rest /// </summary> public async Task StorageBlobDataReaderRoleAssignment(string groupId, string storageAccountName, string blobContainerName) { // The role ID: Storage Blob Data Reader var roleId = "2a2b9908-6ea1-4ae2-8e65-a410df84e7d1"; var roleNameUnique = $"{Guid.NewGuid()}"; // Must be a guid var subscriptionId = _configuration["AzureMgmt:SubscriptionId"]; // the service principal ID var servicePrincipalId = groupId; // the resource group name var resourceGroupName = _configuration["AzureMgmt:ResourceGroupName"]; var objectId = $"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Storage/storageAccounts/{storageAccountName}/blobServices/default/containers/{blobContainerName}"; var url = $"https://management.azure.com{objectId}/providers/Microsoft.Authorization/roleAssignments/{roleNameUnique}?api-version=2022-04-01"; var client = _clientFactory.CreateClient(); var accessToken = await _azureMgmtClientCredentialService.GetAccessToken(); client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken); client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json")); var roleDefinitionId = $"{objectId}/providers/Microsoft.Authorization/roleDefinitions/{roleId}"; var PayloadRoleAssignment = new PayloadRoleAssignment { Properties = new Properties { RoleDefinitionId = roleDefinitionId, PrincipalId = servicePrincipalId, PrincipalType = "Group" } }; // view containers //var getRe = $"https://management.azure.com/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Storage/storageAccounts/{storageAccountName}/blobServices/default/containers?api-version=2023-01-01"; //var response = await client.GetAsync(getRe); //var test = await response.Content.ReadAsStringAsync(); var response = await client.PutAsJsonAsync(url, PayloadRoleAssignment); if (response.IsSuccessStatusCode) { var responseContent = await response.Content.ReadAsStringAsync(); _logger.LogInformation("Created RBAC for read group {blobContainerName} {responseContent}", blobContainerName, responseContent); return; } var responseError = await response.Content.ReadAsStringAsync(); _logger.LogCritical("Created RBAC for read group {blobContainerName} {responseError}", blobContainerName, responseError); throw new ApplicationException($"Status code: {response.StatusCode}, Error: {response.ReasonPhrase}, {responseError}"); } private class PayloadRoleAssignment { [JsonPropertyName("properties")] public Properties Properties { get; set; } = new(); } /// <summary> /// "properties": { /// "roleDefinitionId": /// "subscriptions/SUBSCRIPTION_ID/resourcegroups/RESOURCE_GROUP_NAME/providers/Microsoft.Storage/storageAccounts/STORAGE_ACCOUNT_NAME/providers/Microsoft.Authorization/roleDefinitions/ROLE_ID", /// "principalId": "SP_ID" /// } /// </summary> private class Properties { [JsonPropertyName("roleDefinitionId")] public string RoleDefinitionId { get; set; } = string.Empty; [JsonPropertyName("principalId")] public string PrincipalId { get; set; } = string.Empty; [JsonPropertyName("principalType")] public string PrincipalType { get; set; } = "Group"; } } Putting it togetherA Razor page can be used to create the new clients. This method takes an unknown length of time to run and the RBAC also take an unknown length of time to get applied.
[Authorize(Policy = "blob-admin-policy")] public class CreateClientModel : PageModel { private readonly ClientBlobContainerProvider _clientBlobContainerProvider; private readonly ApplicationMsGraphService _applicationMsGraphService; [BindProperty] public string ClientName { get; set; } = string.Empty; public CreateClientModel( ClientBlobContainerProvider clientBlobContainerProvider, ApplicationMsGraphService applicationMsGraphService) { _clientBlobContainerProvider = clientBlobContainerProvider; _applicationMsGraphService = applicationMsGraphService; } public void OnGet() { } public async Task<IActionResult> OnPostAsync() { if (ModelState.IsValid) { var group = await _applicationMsGraphService .CreateSecurityGroupAsync(ClientName); var blobContainer = await _clientBlobContainerProvider .CreateBlobContainerClient(ClientName); if(blobContainer != null && group != null && group.Id != null) { await _clientBlobContainerProvider .ApplyReaderGroupToBlobContainer(blobContainer, group.Id); } } return Page(); } } NotesThis works well but requires that the application has high privileged access permissions. Most IT departments will not allow this and the creation of blob containers would have to use the IT preferred tools. This type of automation requires 2 different Azure APIs and is not well documented.
LinksUsing Blob storage from ASP.NET Core with Entra ID authentication
Implementing secure Microsoft Graph application clients in ASP.NET Core
https://learn.microsoft.com/en-us/azure/storage/blobs/authorize-access-azure-active-directory
https://docs.microsoft.com/en-us/azure/storage/blobs/storage-blobs-introduction
https://github.com/AzureAD/microsoft-identity-web
Blender, the absolute powerhouse of FOSS 3d (and increasingly 2d) graphics! We give an overview of the software's history, some personal history of our relationships to the software, what it can do, and where we're excited to see it go!
Links:
Some historical Blender videos from the NeoGeo and Not a Number days: Did It, Done It, Not a Number commercial, Come and See
Elephants Dream, aka Project Orange
Previous episodes on blender:
Blender for open movie productions and education Sophie Jantak on pet portraits and Blender's Grease PencilBlender Conference videos mentioned:
Inklines Across The Spiderverse My Journey Across the Spider-Verse: from Hobbyist to Hollywood Forensic Architecture - spatial analysis for human rights casesThe MediaGoblin campaign video (well, the second one)
14th anniversary animation gift to Morgan
Seams to Sewing Pattern (a Blender plugin for making clothes and stuffed animals!) (could we make Free Soft Wear patterns with it?)
Wing It! Production Logs and Blenderheads
Episodes about lisp, because obviously Blender needs more lisp (who's going to do it):
Yesterday, a request was made to like a post from an account with unicode in the username. As Terence Eden notes in Internationalise The Fediverse
Mastodon (the largest ActivityPub service) doesn’t allow Unicode usernames and has resisted efforts to change.
So, I tried with Irwin on otisburg.social and the code threw an exception when I tried to interact with the account
(URI::InvalidURIError) "URI must be ascii only \"https://i18n.viii.fi/@\\u4F60\\u597D\""
This is not an acceptable uri to pass to URI.parse(). In this case, I found some help from a stackoverflow post and fixed the code so I could interact with the account and like the post.
From the comments in Terence’s blog post, I saw Allowed characters in preferredUsername has also been identified as an issue with webfinger.
Arrive at the appointed place and time.
Swim the waters.
Intuit the rules even as you recognize their fluidity.
We are agents, of which Conway would approve.
Choose and choose again.
Those around you choose.
From choices trees unfurl.
Expansive arbors lick at the horizon.
Pixels, neighbors, nodes blink off and on.
Life as pattern in motion.
Ulam and von Neumann’s cellular automata.
Signals synchronize.
Stigmergic swarms generate exquisite gliders and spaceships to speed across crystalline lattice.
A note in ballpoint on the bottom of a pencil drawer in the Hans Bethe house on Bathtub Row: “A mathematician does something on a piece of paper, and then lo and behold a big explosion can occur. S Ulam – Phila. 1967.”
And that “explosion”
Hydrogen bomb?
Or superposition?
The grail…
Atoms broken?
Or linear time?
Consider consciousness harnessed.
Put to bed Georgia Guidestone fairytales.
“Useless eaters” are not so “useless” when networked towards the Omega point.
Biodigital convergent infrastructure maximizes return on humans tethered to sacred fields.
Liquid crystal fascial computing, soulful frequencies dance through entrained torus fields.
Electroceuticals, biofeedback, “white hat” nano join to decrypt secrets beyond human knowing.
Hypercubes architected for group-mind memory storage.
Manifestation protocols where pictographic tokens masquerade as community currency.
Sophisticated storylines weave consent for Santa Fe’s B game.
Human potential harvested from the cacophony of the wise guy commons.
Is the terrain dangerous or didactic?
The conducting programmer…
Benevolent? Malevolent? Indifferent? Curious? Bored?
Can you count the degrees of freedom?
Find your way to the edge?
Flip the board?
Become the governor?
An empire of one?
One man is an island…
Chaotic cycles churn through gap junctions.
Emotional distance offers fleeting grace, a respite of unexpected order.
Thoughtlessly step into the void.
Life’s obvious turning points are outnumbered by countless split paths traversed on autopilot.
Doors are opened, opportunities are taken off the table, outcomes obscured.
Five senses cannot perceive beyond the bend,
But probiotics on standby may orchestrate the holobiont stochastic symphony.
Even as a gut-led leap year duck visitation arose en-route to Montauk.
There, textured yarns were spun of nuclear estuary rituals entangled with digital totem poles, tree spirits, astral projection, and advice on finding your way home.
Where lighthouse and radar tower flank hoodoo bluffs.
Cobbled, glacial beaches kiss Atlantic waves, a naval domain of photonics and frequency.
Microwave ether claimed sodden oak, velvet moss, lichen and quartz.
Inner knowing dispells history’s iron curtain.
Are you the hero?
Is a hero what’s needed?
Interactive Map: https://embed.kumu.io/595f0be99ffa93a388addc2e1b262158#untitled-map?s=bm9kZS1Id1NxMmg4Zw%3D%3D
I’m pleased to announce that some pals and I have organized and will host the (first annual?) Extension Ecosystem Summit at PGConf.dev in Vancouver (and more, see below) on May 28:
Enabling comprehensive indexing, discovery, and binary distribution.
Participants will collaborate to examine the ongoing work on PostgreSQL extension distribution, examine its challenges, identify questions, propose solutions, and agree on directions for execution.
Going to PGConf? Select it as an “Additional Option” when you register, or update your registration if you’ve already registered. Hope to see you there!
Extension Ecosystem Mini-SummitBut if you can’t make it, that’s okay, because in the lead up to the Summit, to we’re hosting a series of six virtual gatherings, the Postgres Extension Ecosystem Mini-Summit.
Join us for an hour or so every other Wednesday starting March 6 to hear contributors to a variety of community and commercial extension initiatives outline the problems they want to solve, their attempts to so, challenges discovered along the way, and dreams for an ideal extension ecosystem in the future. Tentative speaker lineup:
March 6: David Wheeler, PGXN: “State of the Extension Ecosystem” March 20: Ian Stanton, Tembo: “Building Trunk: A Postgres Extension Registry and CLI” April 3: Devrim Gündüz: “yum.postgresql.org and the challenges RPMifying extensions” April 17: Jonathan Katz: “TLE Vision and Specifics” May 1: Yurii Rashkovskii, Omnigres: “Universally buildable extensions: dev to prod” May 15: David Wheeler, PGXN: “Community Organizing Summit Topics”Hit the event page for details. Many thanks to my co-organizers Jeremy Schneider, David Christensen, Keith Fiske, and Devrim Gündüz, as well as the PGConf.dev organizers for making this all happen!
Update: 2024-03-06: Updated the talk schedule.
More about… Postgres PGXN Extensions PGConf Vancouver SummitLately I’ve been thinking a lot about metadata for Postgres extensions.
Traditional use cases include control file metadata, which lives in
.control
files used by CREATE EXTENSION
and friends, and PGXN metadata,
which lives in META.json
files used by PGXN to index and publish extensions.
But these two narrow use cases for SQL behavior and source code distribution
don’t provide the information necessary to enable other use cases, including
building, installing, configuration, and more.
So I have also been exploring other metadata formats, including:
Gogo.mod
Cargo Manifest File
npm package.json
trunk.toml
pgxman buildkit
These standards from neighboring communities reveal a great deal of overlap, as one might expect (everything has a name, a version, an author, license, and so on), but also types of metadata that had not occurred to me. As I took notes and gathered suggestions from colleagues and coworkers, I began to recognize natural groupings of metadata. This lead to the realization that it might be easier — and more productive — to think about these groupings rather than individual fields.
I therefore propose a typology for Postgres extension metadata.
Extension Metadata Typology EssentialsEssential information about the extension itself, including its name (or unique package name), version, list of authors, license, etc. Pretty much every metadata format encompasses this data. Ecosystem applications use it for indexing, installation locations, naming conventions, and display information.
ArtifactsA list of links and checksums for downloading the extension in one or more formats, including source code, binaries, system packages, and more. Apps use this information to determine the best option for installing an extension on a particular system.
ResourcesExternal information about the extension, mostly links, including source code repository, bug reporting, documentation, badges, funding, etc. Apps use this data for links, of course, but also full text indexing, documentation rendering, and displaying useful information about the extension.
ContentsA description of what’s included in the extension package. Often an “extension”
consists of multiple extensions, such as PostGIS, which includes postgis
,
postgis_tiger_geocoder
, address_standardizer
, and more. Furthermore, some
extensions are not CREATE EXTENSION
-type extension at all, such as background
workers, command-line apps, libraries, etc. Each should be listed along with
documentation links where they differ from the package overall (or are simply
more specific).
A list of external dependencies required to configure, build, test, install, and run the extension. These include not only other extensions, but also external libraries and OS-specific lists of binary package dependencies. And let’s not forget the versions of Postgres required, as well as any OS and version dependencies (e.g, does it work on Windows? FreeBSD? What versions?) and architectures (arm64, amd64, etc.)
How to Build ItMetadata that apps use to determine how to build the extension. Does it use the
PostgreSQL PGXS build pipeline? Or perhaps it needs the cargo-based pgrx
toolchain. Maybe a traditional ./configure && make
pattern? Perl, Ruby,
Python, Go, Rust, or NPM tooling? Whatever the pattern, this metadata needs to
be sufficient for an ecosystem app to programmatically determine how to build
an extension.
Usually an extension of the build metadata, the install metadata describes how to install the extension. That could be PGXS or pgrx again, but could also use other patterns — or multiple patterns! For example, perhaps an extension can be built and installed with PGXS, but it might also be TLE-safe, and therefore provide details for handing the SQL files off to a TLE installer.
This typology might include additional data, such as documentation files to install (man pages anyone?), or directories of dependent files or libraries, and the like — whatever needs to be installed for the extension.
How to Run ItNot all Postgres extensions are CREATE EXTENSION
extensions. Some provide
background workers to perform various tasks; others simply provide Utility
applications like pg_top and pg_repack. In fact pg_repack provides both
a command-line application and a CREATE EXTENSION
extension in one package!
This metadata also provides configuration information, both control file
parameters like trusted
, superuser
, and schema
, but also load
configuration information, like whether an extension needs its libraries
included in shared_preload_libraries
to enable LOAD
or requires a
cluster restart. (Arguably this information should be in the “install” typology
rather than “run”.)
Classification metadata lets the extension developer associate additional information to improve discovery, such as key words. It might also allow selections from a curated list of extension classifications, such as the category slugs supported for the cargo categories field. Ecosystem apps use this data to organize extensions under key words or categories, making it easier for users to find extensions often used together or for various workloads or tasks.
Metrics and ReportsThis final typology differs from the others in that its metadata derives from third party sources rather than the extension developer. It includes data such as number of downloads, build and test status on various Postgres/OS/version combinations, binary packaging distributions, test coverage, security scan results, vulnerability detection, quality metrics and user ratings, and more.
In the broader ecosystem, it would be the responsibility of the root registry to ensure such data in the canonical data for each extension comes only from trusted sources, although applications downstream of the root registry might extend metrics and reports metadata with their own information.
What More?Reading through various metadata standards, I suspect this typology is fairly comprehensive, but I’m usually mistaken about such things. What other types of metadata do you find essential for the use cases you’re familiar with? Do they fit one of the types here, or do they require some other typology I’ve failed to imagine? Hit the #extensions channel on the Postgres Slack to contribute to the discussion, or give me a holler on Mastodon.
Meanwhile, I’ll be refining this typology and assigning all the metadata fields to them in the coming weeks, with an eye to proposing a community-wide metadata standard. I hope it will benefit us all; your input will ensure it does.
More about… Postgres Extensions Metadata Classification Categories RFCOrie Steele and I have updated the COSE “typ” (type) Header Parameter Specification to address feedback received during IETF Last Call. No normative changes were made.
Thanks to those that reviewed the specification!
The specification is available at:
https://www.ietf.org/archive/id/draft-ietf-cose-typ-header-parameter-03.htmlBesides the spec being useful on its own, it’s worth noting that the CBOR Web Token (CWT) Claims in COSE Headers specification references this spec, and so won’t exit the RFC Editor queue as an RFC until this one also does.
This article shows how an ASP.NET Core application can control the write access to an Azure blob storage container using an application app registration. Microsoft Entra ID is used to control the user access and to implement the authentication of the web application.
Code: https://github.com/damienbod/AspNetCoreEntraIdBlobStorage
Blogs in this series Using Blob storage from ASP.NET Core with Entra ID authentication Delegated read and application write access to blob storage using ASP.NET Core with Entra ID authentication Multi client blob storage access using ASP.NET Core with Entra ID authentication and RBACThe solution provides a secure upload and secure download using Microsoft Entra ID. Users are authenticated using Microsoft Entra ID. The blob storage containers use Microsoft Entra ID security groups to control the read access. The upload access uses the an Enterprise application for the contributor role. This is used from the ASP.NET Core application for file upload.
In Azure, only the application can upload files and the users of the groups can only read the files.
The RBAC are setup as shown:
Blob storage application write accessThe application can upload files to Azure blob storage. Client secrets are used to acquire the access token using the client credentials OAuth flow. This can be further improved by using a certificate and using the client assertions or even a managed identity from the host service. For development, I normally use a secret for simplicity.
using Azure.Identity; namespace DelegatedReadAppWriteBlobStorage.FilesProvider.AzureStorageAccess; public class ClientSecretCredentialProvider { private readonly IConfiguration _configuration; public ClientSecretCredentialProvider(IConfiguration configuration) { _configuration = configuration; } public ClientSecretCredential GetClientSecretCredential() { // Values from app registration var tenantId = _configuration.GetValue<string>("AzureAd:TenantId"); var clientId = _configuration.GetValue<string>("ApplicationClient:ClientId"); var clientSecret = _configuration.GetValue<string>("ApplicationClient:ClientSecret"); var options = new ClientSecretCredentialOptions { AuthorityHost = AzureAuthorityHosts.AzurePublicCloud }; // https://docs.microsoft.com/dotnet/api/azure.identity.clientsecretcredential var clientSecretCredential = new ClientSecretCredential( tenantId, clientId, clientSecret, options); return clientSecretCredential; } }The BlobApplicationUploadProvider uses the token and makes it possible to upload files to the Azure Blob storage. The provider uses the IFormFile as a parameter for the file data. Meta data is persisted in the local SQL database.
using Azure.Storage.Blobs; using Azure.Storage.Blobs.Models; namespace DelegatedReadAppWriteBlobStorage.FilesProvider.AzureStorageAccess; public class BlobApplicationUploadProvider { private readonly IConfiguration _configuration; private readonly ClientSecretCredentialProvider _clientSecretCredentialProvider; public BlobApplicationUploadProvider(ClientSecretCredentialProvider clientSecretCredentialProvider, IConfiguration configuration) { _configuration = configuration; _clientSecretCredentialProvider = clientSecretCredentialProvider; } public async Task<string> AddNewFile(BlobFileUploadModel blobFileUpload, IFormFile file) { try { return await PersistFileToAzureStorage(blobFileUpload, file); } catch (Exception e) { throw new ApplicationException($"Exception {e}"); } } private async Task<string> PersistFileToAzureStorage( BlobFileUploadModel blobFileUpload, IFormFile formFile, CancellationToken cancellationToken = default) { var storage = _configuration.GetValue<string>("AzureStorage:StorageAndContainerName"); var fileFullName = $"{storage}/{blobFileUpload.Name}"; var blobUri = new Uri(fileFullName); var blobUploadOptions = new BlobUploadOptions { Metadata = new Dictionary<string, string?> { { "uploadedBy", blobFileUpload.UploadedBy }, { "description", blobFileUpload.Description } } }; var blobClient = new BlobClient(blobUri, _clientSecretCredentialProvider.GetClientSecretCredential()); var inputStream = formFile.OpenReadStream(); await blobClient.UploadAsync(inputStream, blobUploadOptions, cancellationToken); return $"{blobFileUpload.Name} successfully saved to Azure Blob Storage Container"; } } Blob storage delegated read accessThe blob storage reader access is given to all users in the security group. This security group uses the app-role from the Azure app registration and the group can access the blob container, not the blob account. No SAS are used .
using Azure.Core; using Microsoft.Identity.Client; using Microsoft.Identity.Web; namespace DelegatedReadAppWriteBlobStorage.FilesProvider.AzureStorageAccess; public class DelegatedTokenAcquisitionTokenCredential : TokenCredential { private readonly ITokenAcquisition _tokenAcquisition; private readonly IConfiguration _configuration; public DelegatedTokenAcquisitionTokenCredential(ITokenAcquisition tokenAcquisition, IConfiguration configuration) { _tokenAcquisition = tokenAcquisition; _configuration = configuration; } public override AccessToken GetToken(TokenRequestContext requestContext, CancellationToken cancellationToken) { throw new NotImplementedException(); } public override async ValueTask<AccessToken> GetTokenAsync(TokenRequestContext requestContext, CancellationToken cancellationToken) { string[]? scopes = _configuration["AzureStorage:ScopeForAccessToken"]?.Split(' '); if (scopes == null) { throw new Exception("AzureStorage:ScopeForAccessToken configuration missing"); } AuthenticationResult result = await _tokenAcquisition .GetAuthenticationResultForUserAsync(scopes); return new AccessToken(result.AccessToken, result.ExpiresOn); } } NotesUsing an application for write access to the blob storage has both advantages and disadvantages in context of security. Removing the write access to all users is positive, but when using applications or if the application has weak security, this approach can be bad. Using a managed identity for the deployed service would improve the security and would remove the need for secrets or certificates to authenticate the application. This prepares the access for the next solution which would be to implement a multi-tenant or multi-client application using the Azure blob.
LinksUsing Blob storage from ASP.NET Core with Entra ID authentication
Implementing secure Microsoft Graph application clients in ASP.NET Core
https://learn.microsoft.com/en-us/azure/storage/blobs/authorize-access-azure-active-directory
https://docs.microsoft.com/en-us/azure/storage/blobs/storage-blobs-introduction
https://github.com/AzureAD/microsoft-identity-web
Today I was fortunate to have my picture taken with Morris Lukowich during Hockey Day in Houston at Saint Arnold Brewing. The best hockey games I have ever watched in-person were the 1977-78 Houston Aeros. The WHA All-Star game was in Quebec City on January 17, 1978 and for the picture I believe it is Lukowich’s All-Star jersey from that game that I have borrowed.
OpenID Federation draft 33 has been published at https://openid.net/specs/openid-federation-1_0-33.html and https://openid.net/specs/openid-federation-1_0.html. The working group continues refining the specification to make it more consistent and easier to read and implement.
We published draft 33 now to get these improvements out to implementers. Per the history entries at https://openid.net/specs/openid-federation-1_0-33.html#name-document-history, a summary of changes made in -32 and -33 is:
-33:
Addressed #2111: The metadata_policy_crit claim MAY only appear in Subordinate Statements and its values apply to all metadata_policies found in the Trust Chain. Fixed #2096: Authorization Signed Request Object may contain trust_chain in its payload and should not in its JWS header parameters. Strengthen language requiring client verification with automatic registration. Fixed #2076: Promoted Trust Marks to be a top-level section. Added General-Purpose JWT Claims section. Moved Federation Endpoints section before Obtaining Federation Entity Configuration Information section. Fixed #2110: Explanation text when multiple entity_type parameters are provided in the Subordinate Listing endpoint. Fixed #2112, #2113, and #2114: Defined that client authentication is not used by default and that the default client authentication method, when used, is private_key_jwt. Specified that requests using client authentication use HTTP POST. Fixed #2104: Allow trust marks in Subordinate Statements for implementation profiles that might want this. Fixed #2103: Addressed ambiguities in the definition of constraints.-32:
Tightened OpenID Connect Client Registration section. Tightened appendix examples. Fixed #2075: Trust Mark endpoint for the provisioning of the Trust Marks. Fixed #2085: Trust Marked Entities Listing, added sub URL query parameter. Made fetch issuer unambiguous by making the iss parameter REQUIRED. Introduced the term “Subordinate Statement” and applied it throughout the specification. Also consistently use the term “registration Entity Statement” for Explicit Client Registration results. Clarified where Entity Statement claims can and cannot occur. Renamed policy_language_crit to metadata_policy_crit. Fixed #2093: Numbered the list defining the order policy operators are applied in.Special thanks to Stefan Santesson for his thorough review of the specification in the context of the Swedish Federation deployment!
I recently spoke with Riley Hughes of Trinsic on his Future of Identity podcast about the birth of Sovrin Foundation, it's inevitable growing pains, self-sovereign identity, identity metasystems, and adoption. Give it a listen.
I'm grateful to Riley for having me on as a guest.
Some of the ideas in this podcast are explored more deeply in my latest book, Learning Digital Identity from O’Reilly Media. You can support my writing by buying a copy!
If you enjoyed this, please consider sharing it with a friend or twenty.
The Rich Authorization Request extension to OAuth 2, or RAR, is a way to talk about access in the OAuth space beyond what scopes allow, and it is defined in RFC9396. One of the key motivations behind RAR was admitting, as a community, that a lot of times you need more than a set of scope values to properly describe access to an API.
A Larger ScopeScopes work pretty well in OAuth, but they’re ultimately just a set of strings. It’s worlds better than the all-or-nothing access that OAuth 1 or API keys give you, and if you have an API, they allow you to easily separate read and write access. But what if you want to be more specific? What if you want to, say, let someone access a specific account for certain features for a certain amount of time? Or read in one place but write to another? And what if you want to compose that set of features differently, such as any account for an unlimited time but only if the user’s online? The ways of describing API access are as varied as the APIs being accessed.
When faced with this problem, the first thing that many people realize is that because scopes are just strings, their own API can impose semantics and syntax on those strings. So people add parameters, or define composition rules, or even define a whole query language embedded into the scope string. But those approaches require a lot of complex processing and lack interoperable structure that would let you easily combine protection of multiple kinds of APIs.
What RAR gives you, instead, is an array of JSON objects to describe your API access. So instead of trying to cram everything into a single string, you can now put together an object that defines exactly what you want.
[This object is specific to the API in question and carries with it all the detail that is needed for successful processing. Each kind of API can define its own type value, which in turn defines what’s allowed to go into the rest of the object. And if you need more than one view of things, like read access to A but write access to B, then you can pass in multiple objects in the same structure.
One important question arises out of this: who needs to know this level of detail?
Who Cares About RARIn this regard, RAR really is built on top of the concept of a scope. In an OAuth delegation, there are four parties. The client, resource owner, authorization server (AS), and resource server (RS).
The four parties in OAuth delegation, illustration from OAuth 2 In ActionThese parties have particular relationships, and each of them might care about a RAR object or a scope in a slightly different way. However, the more important question is about which relationship is in play.
Client -> AS: Requesting AccessWhen requesting an access token, the client needs to be able to describe to the AS what it wants. RAR allows the client to get VERY specific, if the client knows what details it wants ahead of time. Maybe the client has prompted the resource owner for an account identifier, or has learned through some other protocol where the target system is located, or it’s just been configured to know that it needs to ask for specific objects in order to do specific things. In all of these cases, the client can send RAR objects to the AS just like it would a scope, in the hopes of getting an access token that can do what it asks for.
AS -> Client: Granting AccessWhen the access token is granted, the AS can tell the client which RAR objects have been applied to the token. While this information is no substitute for an API discovery protocol, this approach can let the client differentiate what an access token is good for in different dimensions. For example, a client can ask for a token for an available signing service, and then be granted a token for use at a specific signing service, indicated through the locations field in the RAR object.
AS -> Resource owner: Gathering AuthorizationDuring the delegation process, the AS often needs to prompt the resource owner to see if they’re OK with what’s being delegated. While this starts as the Client->AS request, RAR gives the AS an opportunity to fine-tune the access by asking the resource owner to be specific, or even filling in values that get put into the resulting RAR object. Maybe the client is asking for account access but the resource owner stipulates that it’s only good for the next five minutes. This does come at a usability cost, since it’s much easier to display a list of scope strings with checkboxes. But experience has shown that this list is not a great security measure anyway, since most users won’t change the checkboxes, and often don’t understand the differentiated access being granted.
AS -> RS: Describing AccessThe access token itself represents a certain set of rights that have been granted. These can be described in the metadata of the token, available through either a structured token field or an introspection response. In this way, the RS can learn what an access token is good for, and apply its policies appropriately. Does the token grant access for the HTTP GET command on the resource at /photo/123-fda1d? Is this token even good at this specific RS, or is it meant for somewhere else? The RAR object can be used to describe all of this.
Not Everything Has To MatchFinally, it’s important to note that the all of these different branches need not match each other in a single transaction. In one of the applications where I’ve personally deployed RAR, the client never sees the RAR objects. The client knows to ask for a specific scope, and the AS knows that when it sees that scope, the resulting token needs to apply to a whole set of things represented by the current user’s access within the system. The downstream APIs know nothing about users or accounts, but they do know the resources they protect.
As a consequence, the AS translates the client’s incoming scope request to a set of RAR objects that the APIs understand. The APIs never see or care about the scope, and the client never sees or cares about the RAR. In this way, internal API details stay internal and do not leak unnecessarily into the wider system.
However, a different client in this same ecosystem does have insight into the details of the API structure, and therefore its requests do specify RAR objects that target the APIs. These objects are processed in exactly the same way by the API servers, which gives us a powerful parallelism and profound code reuse in production.
GNAP NativeIn GNAP, one of our main goals was to see what an OAuth-style system would look like without the constraints and history of OAuth, and one such constraint includes scopes. Consequently, GNAP’s native access rights is an array of objects that look suspiciously like RAR objects. This design is, of course, intentional, and in many ways RAR is the backport of GNAP’s access rights system to work on top of OAuth 2. While GNAP doesn’t have scopes in the same way, GNAP’s reference-based approach to its API design does allow for the use of a simple string to stand in for the objects in question, allowing a request to have both shortcut and fully specified items in the same request.
"access": [RAR support is starting to show up across different vendors, though it’s not universal yet. One of the companies I work for, Authlete, supports RAR natively. Other products can often have RAR grafted on top, since it takes the form of an extra parameter to be processed by an extension or module.
The real value is that we are starting to see API access defined in terms of RAR objects, replacing the awkward and error-prone string composition practices of the past. RAR may seem complex, but when you look at how APIs are defined and scopes are used, the power of that complexity really starts to show its value.
Every software distribution system deals with versioning. Early in the design of PGXN, I decided to require semantic versions (SemVer), a clearly-defined and widely-adopted version standard, even in its pre-1.0 specification. I implemented the semver data type that would properly sort semantic versions, later ported to C by Sam Vilain and eventually updated to semver 2.0.0.
As I’ve been thinking through the jobs and tools for the Postgres extension ecosystem, I wanted to revisit this decision, the context in which it was made, and survey the field for other options. Maybe a “PGXN v2” should do something different?
But first that context, starting with Postgres itself.
PostgreSQL Extension Version StandardFrom the introduction extensions in PostgreSQL 9.1, the project side-stepped the need for version standardization and enforcement by requiring extension authors to adopt a file naming convention, instead. For example, an extension named “pair” must have a file with its name, two dashes, then the version as listed in its control file, like so:
pair--1.1.sql
As long as the file name is correct and the version part byte-compatible with
the control file entry, CREATE EXTENSION
will find it. To upgrade an extension
the author must provide a second file with the extension name, the old version,
and the new version, all delimited by double dashes. For example, to upgrade our
“pair” extension to version 1.2
, the author supply all the SQL commands
necessary to upgrade it in this file:
pair--1.1--1.2.sql
This pattern avoids the whole question of version standards, ordering for upgrades or downgrades, and all the rest: extension authors have full responsibility to name their files correctly.
PGXN VersionsSemVer simplified a number of issues for PGXN in ways that the PostgreSQL
extension versioning did not (without having to re-implement the core’s file
naming code). PGXN wants all metadata for an extension in its META.json
file, and not to derive it from other sources that could change over time.
Following the CPAN model, PGXN also required that extension releases never
decrease the version.1 The well-defined sortability of semantic versions
made this validation trivial. PGXN later relaxed enforcement to allow updates
to previously-released versions. SemVer’s clearly specified sorting made this
change possible, as the major.minor.patch
precedence intuitively compare from
left to right.
In other words, if one had previously released version 1.2.2, then released
1.3.0, a follow-up 1.2.3 is allowed, increasing the 1.2.x
branch version, but
not, say, 1.2.1, which decreases the 1.2.x
branch version.
Overall, semantic versions have been great for clarity of versioning of PGXN
extensions. The one bit of conflict comes from extensions that use some other
other version standard in the control file, usually a two-part x.y
version not
allowed by SemVer, which requires x.y.z
(or, more specifically,
major.minor.patch
).
But such versions are usually compatible with SemVer, and because PGXN cares
only about the contents of the META.json
, they’re free to use their own
versions in the control file, just as long as the META.json
file uses SemVers.
For example, the recent nominatim_fdw v1.0.0 release, which of course lists
"version": "1.0.0"
in its META.json
file, sticks to its preferred
default_version = '1.0'
in its control file. The extension author simply
appends .0
to create a valid SemVer from their preferred version, and as long
as they never use any other patch number, it remains compatible.
Surveying the versioning landscape in 2024 yields a number of approaches. Might we prefer an alternative for future extensions distribution? Let’s look at the possibilities.
Ad Hoc VersionsAs described above, the Postgres file naming convention allows ad hoc versions. As far as I can tell, so does the R Project’s CRAN. This approach seems fine for systems that don’t need to follow version changes themselves, but much trickier for systems that do. If I want to install the latest version of an extension, how does the installer know what that latest version is?
The answer is that the extension author must always release them in the proper order. But if someone releases 1.3.1 of an extension, and then 1.2.1, well then 1.2.1 is the latest, isn’t it? It could get confusing pretty quickly.
Seems better to require some system, so that download and install clients can get the latest version — or the latest maintenance version of an earlier release if they need it.
User ChoiceQuite a few registries allow users to choose their own versioning standards, but generally with some very specific recommendations to prevent confusion for users.
Python Packaging is fairly liberal in the versions it allows, but strongly recommends semantic versioning or calendar versioning (more on that below). CPAN (Perl) is also fairly liberal, due to its long history of module distribution, but currently requires “Decimal versions”, which are evaluated as floating-point numbers, or dotted integer versions, which require three dot-separated positive integers and must begin with the letterv
.
RubyGems does not enforce a versioning policy, but warns that “using an
‘irrational’ policy will only be a disservice to those in the community who
use your gems.” The project therefore urges developers to follow SemVer.
These three venerable projects date from an earlier period of registration and distribution, and have made concessions to times when no policies existed. Their solutions either try to cover as many legacy examples as possible while recommending better patterns going forward (Python, Perl), or simply make recommendations and punt responsibility to developers.
SemVerMore recently-designed registries avoid this problem by requiring some level of versioning standard from their inception. Nearly all use SemVer, including:
Go Modules, where “Each version starts with the letter v, followed by a semantic version.” Cargo (Rust), which “uses SemVer for specifying version numbers. This establishes a common convention for what is compatible between different versions of a package.” npm, where the “version must be parseable by node-semver, which is bundled with npm as a dependency.” CalVerCalVer eschews context-free incrementing integers in favor of semantically-meaningful versions, at least for some subset of a version string. In other words: make the version date-based. CalVer-versioned projects usually include the year and sometimes the month. Some examples:
Ubuntu usesYY.0M.MICRO
, e.g., 23.04
, released in April 2023, and
23.10.1
, released in October 2023
Twisted uses YY.MM.MICRO
, e.g., 22.4.0
, released in April 2022
Ultimately, adoption of a CalVer format is a more choice about embedding calendar-based meaning into a version more than standardizing a specific format. One can of course use CalVer semantics in a semantic version, as in the Twisted example, which is fully-SemVer compliant.
In other words, adoption of CalVer need not necessitate rejection of SemVer.
Package ManagersWhat about package managers, like RPM and Apt? Some canonical examples:
RPM packages use the format:
<name>-<version>-<release>.<architecture>
Here <version>
is the upstream version, but RPM practices a reasonable (if
baroque) version comparison of all its parts. But it does not impose a
standard on upstream packages, since they of course vary tremendously
between communities and projects.
Apt packages use a similar format:
[epoch:]upstream_version[-debian_revision]
Again, upstream_version
is the version of the upstream package, and not
enforced by Apt.
APK (Alpine Linux) packages use the format
{digit}{.digit}...{letter}{_suf{#}}...{-r#}
I believe that {digit}{.digit}...{letter}
is the upstream package version.
This pattern makes perfect sense for registries that repackage software from dozens of upstream sources that may or may not have their own policies. But a system that defines the standard for a specific ecosystem, like Rust or PostgreSQL, need not maintain that flexibility.
RecommendationGiven this survey, I’m inclined to recommend that the PostgreSQL community
follow the PGXN (and Go, and Rust, and npm) precedent and continue to rely on
and require semantic versions for extension distribution. It’s not
perfect, given the contrast with the core’s lax version requirements. CalVer
partisans can still use it, though with fewer formatting options (SemVer forbids
leading zeros, as in the Ubuntu 23.04
example).
But with its continuing adoption, and especially its requirement by more recent, widely-used registries, and capacity to support date-based semantics for those who desire it, I think it continues to make the most sense.
Wrong!I’m probably wrong. I’m often mistaken in one way or another, on the details or the conclusion. Please tell me how I’ve messed up! Find me on the #extensions channel on the Postgres Slack or ping me on Mastodon.
Why? Because every module on CPAN has one and only one entry in the index file. Ricardo Signes explains. ↩︎
More about… Postgres Extensions PGXN Versioning SemVer CalVerRemember endless hours ripping your CD collection to digital…why are we spending endless hours scanning analog ID documents over and over again? Alternatives exist. Let’s think differently about how Mobile KYC fails customers
A recent thread on a work-oriented social platform caught my attention. Some people I know to be the people they are could not verify their profiles using a mobile KYC solution. This article isn’t to bash those solutions, or the myriad improvements made, but to urge our industry to understand the complexity we’ve foisted on our users and incite action to do different.
Analog to Digital Conversion, anyone? “Ripping IDs” to confirm identity should go the way of Ripping CDs… How do I know the problems that exist?In December 2014, before a presentation to the Identity Ecosystem Steering Group about how to “Bring the trust of the Driver’s license online”, MorphoTrust USA filed a provisional application that became US Patent 10,678,939. The front-back-selfie wave was born, admittedly without the system of record connection described in that patent text. I spent several years leading a smart team improving an identity verification product line.
I’ve watched thousands of people try to run these selfie-to-DL matching steps in various forms and helped debug their issues with smiles, vision acuity, phone models, and lighting. I’ve spent weekends helping hundreds of great Utahns use mobile registration live in a credit union lobby.
Visually impaired people cannot get over these hurdles. It takes too many hands for these solutions to be inclusive. Back-facing phone camera quality varies greatly with how much you can spend. These solutions are not inclusive by any stretch of the imagination, and the fallback is to go to a physical location.
The Places Within the Selfie-ID Verification Process that Fail Manually taking a quality, flat, well-lit, high-res picture of a card is hard Taking an ICAO-quality, well-lit, machine-matchable portrait is difficult. Everybody wants to smile (or make duck lips …idk…) in a selfie Webcams are very low resolution compared to phones and vary widely PDF417 Barcodes from the back of DL/ID cards are easy to generate and nearly every Fake ID has the same information on the back and front Connections to databases that would verify the authenticity and validity of a DL/ID are expensive or restricted to law enforcement (makes sense) Most hard-to-forge security features of an ID card require multi-spectral light, are tactile, or require magnification. Phones capture visible light Cropping the card portrait results in a small, low-res photo for machine-matching that has security lines, holograms, and indicia through it Cropping a portrait from a poorly captured, low-res ID card will not give sufficient resolution to measure the facial features for matching You need cloud/phone processing power to determine that a human is live and real for the probe image — called Presentation Attack Detection I have to ID myself repeatedly with each new service that wants (notice I didn’t say “needs”) ID and each KYC system differs, creating doubtCan I stop here? We haven’t even gotten to the accuracy of biometric matching algorithms (new entries, if used, have solved any racial/gender bias, so lighting is the major hurdle to accurate matching). People think biometrics are 100% when a human visual verificdation is only ~97% (per a 2017 Google study that I cannot find).
Oh, did I mention that people end up with images of their IDs in photo rolls and synchronized to every app they grant photo library permission?
Humans are humans and will do what humans do using technology.
You simply cannot account for the variations people and machines will cause to happen (credit Jurassic Park)If there are this many variables to a process that seems conceptually simple, one or more of those variables will go wrong.
We are turning away good potential customersThere are tremendous capture, scan, photo, and biometric matching systems out there from excellent, reputable vendors. The improvements in the decade since selfie matching started are incredible and laudable. This process is significantly easier and more accurate than it ever was.
Still, people sitting at their desks get turned away from having “verified profiles”. Now try taking a picture of your ID with one hand, holding your phone in the other, on a sidewalk balancing your potential rental scooter against your hip in bright, vacation sunlight. Ya, no!
Let’s go DigitalMobile Driver’s Licenses (mDL) are now available to greater than 17% of the US cardholding population. Adoption isn’t awesome yet because people don’t know they have existed in production for nearly 2 years and in concept for four-plus years. ISO/IEC 18013–5 is solid and functional. The data is digitally signed by your Issuer but you control it. It provides a level of control over data and transparency into data-storage that users haven’t experienced for decades. There are verifier apps and equipment from multiple vendors. Places do accept mDL.
In the coming months of 2024, ISO/IEC 18013–7 will add consented, selective-disclosure, digitally-signed mDL sharing to apps on the same device AND to websites over the Internet. (This will be the topic of a forthcoming post of mine.) So imagine sharing just your age to be granted entry to that website you don’t want your name associated with… or accurately filing for benefits without typing into a form. During the final approval stages, the ISO/IEC 18013–7 Technical Specification will be available for implementation.
Let’s Go Digital to Digital“The first bank to replace their Mobile KYC with a connection to a Mobile Driver’s License is going to garner attention from the press and obtain new customers.”
It’s time.
The mDL provides digitally signed, matchable portraits upon consent as well as only the digitally signed data relevant for the approval. ISO/IEC 18013–7 will allow app-to-app consent and retrieval of mDL data similar to the way your email launches your airline application. It will also present a subset of mDL data to a website after consent for uses like age verification.
The challenge is made: Let’s short-circuit problems 1, 3, 4, 5, 6, 7, 8, and 10 above. Let’s make better self-registration and selfie-KYC solutions. Let’s work together on mDL acceptance. Who will sign up:
Relying Party (bank): _____________________________________
Biometric Match and Liveness Vendor: ______________________
mDL Solution Provider: ____________________________________
Test Subject mDL Holders: _________________________________
David can be reached for comments and feedback at david at decipher dot id
Over on the Tembo blog I’ve published a thinking-through of what all the jobs to be done of the ideal Postgres extension ecosystem might be:
More about… PGXN Extensions Metadata Jobs to be DoneThese challenges and the interest and energy put into exploring new solutions make clear that the time has come to revisit the whole idea of the PostgreSQL extension ecosystem: to work though the jobs to be done, specify the tools to do those jobs, and outline a plan for the broader Postgres community to design and build them.
Future posts will dream up the tools and make the plan; today we begin with the jobs.
🎬 Let’s get started.
This article shows how to use a CSP nonce in a Blazor Web application using the InteractiveServer server render mode. Using a CSP nonce is a great way to protect web applications against XSS attacks and other such Javascript vulnerabilities.
Code: https://github.com/damienbod/BlazorServerOidc
NotesThe code in this example was built using the example provided by Javier Calvarro Nelson.
https://github.com/javiercn/BlazorWebNonceService
Services and middlewareThe Blazor Web application is implemented using the AddInteractiveServerComponents for the InteractiveServer server render mode. The nonce can be used by implementing a nonce service using the CircuitHandler. The nonce service is a scoped service.
builder.Services.AddRazorComponents() .AddInteractiveServerComponents(); builder.Services.TryAddEnumerable(ServiceDescriptor .Scoped<CircuitHandler, BlazorNonceService>(sp => sp.GetRequiredService<BlazorNonceService>())); builder.Services.AddScoped<BlazorNonceService>();The headers are implemented using the NetEscapades.AspNetCore.SecurityHeaders package. The headers are added to the Blazor nonce service using the NonceMiddleware middleware.
app.UseSecurityHeaders(SecurityHeadersDefinitions.GetHeaderPolicyCollection( app.Environment.IsDevelopment(), app.Configuration["OpenIDConnectSettings:Authority"])); app.UseMiddleware<NonceMiddleware>(); Setup Security headersThe security headers CSP script tag is setup as best possible for a Blazor Web application. A CSP nonce is used as well as the fallback definitions for older browsers.
.AddContentSecurityPolicy(builder => { builder.AddObjectSrc().None(); builder.AddBlockAllMixedContent(); builder.AddImgSrc().Self().From("data:"); builder.AddFormAction().Self().From(idpHost); builder.AddFontSrc().Self(); builder.AddBaseUri().Self(); builder.AddFrameAncestors().None(); builder.AddStyleSrc() .UnsafeInline() .Self(); // due to Blazor builder.AddScriptSrc() .WithNonce() .UnsafeEval() // due to Blazor WASM .StrictDynamic() .OverHttps() .UnsafeInline(); // fallback for older browsers when the nonce is used }) Setup Middleware to add the nonce to the stateThe NonceMiddleware uses the nonce header created by the security headers package and sets the Blazor nonce service with the value. This is updated on every request.
namespace BlazorWebFromBlazorServerOidc; public class NonceMiddleware { private readonly RequestDelegate _next; public NonceMiddleware(RequestDelegate next) { _next = next; } public async Task Invoke(HttpContext context, BlazorNonceService blazorNonceService) { var success = context.Items.TryGetValue( "NETESCAPADES_NONCE", out var nonce); if (success && nonce != null) { blazorNonceService.SetNonce(nonce.ToString()!); } await _next.Invoke(context); } } Using the nonce in the UIThe BlazorNonceService can be used from the Blazor components in the InteractiveServer render mode. The nonce is applied to all script tags. If the script does not have the correct nonce, it will not be loaded. The GetNonce method reads the nonce value from the BlazorNonceService service.
@inject IHostEnvironment Env @inject BlazorNonceService BlazorNonceService @using System.Security.Cryptography; <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <base href="/" /> <link rel="stylesheet" href="css/bootstrap/bootstrap.min.css" /> <link href="css/site.css" rel="stylesheet" /> <link href="BlazorWebFromBlazorServerOidc.styles.css" rel="stylesheet" /> <HeadOutlet @rendermode="InteractiveServer" /> </head> <body> <Routes @rendermode="InteractiveServer" /> http://_framework/blazor.web.js </body> </html> @code { /// <summary> /// Original src: https://github.com/javiercn/BlazorWebNonceService /// </summary> [CascadingParameter] HttpContext Context { get; set; } = default!; protected override void OnInitialized() { var nonce = GetNonce(); if (nonce != null) { BlazorNonceService.SetNonce(nonce); } } public string? GetNonce() { if (Context.Items.TryGetValue("nonce", out var item) && item is string nonce and not null) { return nonce; } return null; } } NotesNonces can be applied to Blazor Web using the server rendered mode and the BlazorNonceService which implements the CircuitHandler. Thanks the Javier Calvarro Nelson for providing a solution to this. Next would be to find a solution for the AddInteractiveWebAssemblyComponents setup. You should always use a CSP nonce on a server rendered application and only load scripts with the CSP nonce applied to it.
Linkshttps://github.com/javiercn/BlazorWebNonceService
https://github.com/andrewlock/NetEscapades.AspNetCore.SecurityHeaders
“Life begins like a dream, becomes a little real, and ends like a dream.” ― Michael Bassey Johnson, The Oneironaut’s Diary
As many of you already know, SAP has made public its plans on how SAP IDM will be retired as a supported offering. I’ve been stewing on this for a bit as I try to figure out exactly how I feel about this and what needs to happen next.
To be fair, I haven’t worked with the product much for just over four years, and even then, I was working more with Version 7 than with Version 8. My opinions are completely my own and do not represent my current employer, any previous employer, or SAP.
While IDM is certainly showing its age, there are some very good things about it that I would love to see as an open-source offering. First is the Batch Processing capabilities of IDM, based on the old MaXware Data Synchronization Engine/MetaCenter solutions. It features some powerful functionality to synchronize and cleanse data. It sets up fairly easily and is quite easy to configure. I’m sure the open-source community could do well with maintaining the UI (It definitely should be JAVA-based rather than the old Windows MMC) that will fit better in today’s Enterprise setting. Also, easy integration with SaaS services is a needed upgrade.
The other thing that should be released into the wild is the Virtual Directory. It also provides powerful functionality for several use cases, from pass-through authentication to assisting in M&A use cases. It’s the perfect example of a “Black Box” offering that just works. It also makes it much easier to synchronize and cleanse data by representing many different back ends via the easy-to-consume LDAP standard.
It saddens me that SAP is choosing to move away from IDM, as one of the key selling points of SAP IDM is its ability to integrate seamlessly with the SAP ecosystem. I hope SAP will help all LCM/IGA vendors connect more easily with systems. SaaS integration should be easy or standards-based, but we still need to be concerned for organizations still using on-premises SAP tools.
SAP has indicated that Microsoft’s Entra ID will be the main partner in the future, but I hope they make this information open to all vendors and that there will be continuing support of standard protocols. This article gives me some hope, but actions speak louder than words. I do have some concerns that SAP, known as a vast software ecosystem that supports itself and tends to ignore the enterprise, is handing off to another large software provider whose management tools tend to support their software ecosystem first and consider the enterprise second. Let’s face it: most of Microsoft’s Identity and Access Management efforts have been about supporting the Office 365 family of products. Don’t get me wrong; it’s better than SAP in this regard, but it’s not that high of a level to meet. For what it’s worth, I am guardedly optimistic, but I always try to remain hopeful.
Finally, I think it’s important to thank the IDM team in Sofia for all their hard work over the years, which, of course, would not have been possible without the vision and effort of the original MaXware team based in Trondheim, Norway, and associated teams in the UK, Australia, and the US. The production from these small teams helped define what Identity Management is to this day.
Will this be my last blog entry on the topic of SAP IDM? I don’t know. Part of it will depend on if there are any moves towards the Open Source world. There have been at least three times in my life when I thought I was done with this tool, and deep down, I’m pretty sure there is a little more in my future.
In the meantime, I hope to resume blogging more regarding the Identity and Access Management field in the near future. Time will tell.
Registration of the composable event streaming representation (CESR) format in the IANA Media Type Registry shows a recent development of the key event receipt infrastructure (KERI) and authentic chained data containers (ACDC) space and how the space is growing.
See the following link for the official entry: IANA Media Type Registry entry for CESR (application/cesr)
Here’s the latest installment in the series on working with LLMS: Creating a GPT Assistant That Writes Pipeline Tests.
Once you get the hang of writing these tests, it’s mostly boilerplate, so I figured my team of assistants could help. I recruited Cody, GitHub Copilot, and Unblocked — with varying degrees of success. Then I realized I hadn’t yet tried creating a GPT. As OpenAI describes them, “GPTs are custom versions of ChatGPT that users can tailor for specific tasks or topics by combining instructions, knowledge, and capabilities.”
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
12 Let’s talk: conversational software development
13 Using LLMs to Improve SQL Queries
14 Puzzling over the Postgres Query Planner with LLMs
15 7 Guiding Principles for Working with LLMs
Working on it.. OYO AI by kidOYO® Learning Software, Education Services
In 2024, the utility of words yielding philosophical clarity that becomes embedded into the design of systems being deployed globally, and Nationally, yields methods that must be structured accurately in order to abide by the Sovereign systems they serve.
In America, people own root authority, or the Sovereign infrastructure does not confer accuracy for, of, by human use. Data is the life blood of AI systems. Data structure yields Sovereign results, and across our fast advancing world, inaccuracy deconstructs faster than accuracy builds accurately. The time has come for open transparent accuracy in the data structure of Soveriegnty itself to be laid bare, enabling the development of "Sovereign AI" upon foundations that serve people.
Many moons ago, this structural conversation began in the world of identity management. Professionally-deployed systems were challenged to confront inaccuracies in their modeling of human identity. Ubiquitously, people were no longer being conveyed structural constraints ensuring the structural accuracy of their root administrative authority over data systems of ultimate importance to their Sovereign participation and administration under well-founded laws that were crafted pre-tcp/ip, pre-digital data.
Identity systems have been challenged now for over 20 years to align their practices to the people they service. The work is not done. Self-Sovereign ID principles that emerged here on this blog, led to decentralized identity methods and practices advancing for developer use, and into general awareness by a population that is intensely interested in digital frontiers where their lives meet opportunity, security, and civil system integrity. The fire walls of Sovereign integrity, having been breached many times in consequential ways, started exposing their own structural deficiencies.
Enter AI: human identity that primarily exists in a database-driven system, and is founded on an old-era of physical presence, is now the domain of AI. Human beings can not compete structurally here, as AI derives utility that people provide, and far too often, provide ignorantly, without much personal insight or accountability for the structural choices conveyed upon them. Laws, as dependencies function, evolve at a much slower pace, and seem to lack insight into the structural underpinnings of identity silos that tcp/ip was advanced to break down and add utility too. Unfortunately, protections were not advanced with the same insight, and civil society is finding itself in a reactive mode, reacting to change like a surfer riding a wave, rather than a break wall securing civil participation in an AI-enabled society.
This is the moment. AI Sovereignty has a basic and tremendously important dependency in American civil society: people own root.
If the data structure of human participation in America does not convey this basic structural reality, then people do not exist in a civil society, as defined by founding documents, intent, and Constitutional reach. Work is underway on this vector, and as always, the resulting choices and structures advanced will yield the results being pursued. The question on the table being asked is simple: do innovators understand what it means in structural Terms to ensure that people own root authority?
This article shows how to implement a secure upload and a secure download in ASP.NET Core using Azure blob storage. The application uses Microsoft Entra ID for authentication and also for access to the Azure Blob storage container.
Code: https://github.com/damienbod/AspNetCoreEntraIdBlobStorage
Blogs in this series Using Blob storage from ASP.NET Core with Entra ID authentication Delegated read and application write access to blob storage using ASP.NET Core with Entra ID authentication Multi client blob storage access using ASP.NET Core with Entra ID authentication and RBAC Security architectureThe application is setup to store the file uploads to an Azure Blob storage container. The authentication uses delegated only flows. A user can authenticate into the application using Microsoft Entra ID. The Azure App registration defines App roles to use for access authorization. The roles are used in the enterprise application. Security groups link the users to the roles. The security groups are used in the Azure Blob container where the RBAC is applied using the groups. A SQL database is used to persist the meta data and integrate into the other parts of the application.
Setting up Azure Blob storageTwo roles were created in the Azure App registration. The roles are assigned to groups in the Enterprise application. The users allowed to used to Azure Blob storage are assigned to the groups.
The groups are then used to apply the RBAC roles in the Azure Blob container. The Storage Blob Data Contributor and the Storage Blob Data Reader roles are used.
AuthenticationMicrosoft Entra ID is used for authentication and implemented using the Microsoft.Identity.Web Nuget packages. The is a standard implementation. Two policies were created to validate the two different roles used in this solution.
string[]? initialScopes = configuration.GetValue<string> ("AzureStorage:ScopeForAccessToken")?.Split(' '); services.AddMicrosoftIdentityWebAppAuthentication(configuration) .EnableTokenAcquisitionToCallDownstreamApi(initialScopes) .AddInMemoryTokenCaches(); services.AddAuthorization(options => { options.AddPolicy("blob-one-read-policy", policyBlobOneRead => { policyBlobOneRead.RequireClaim("roles", ["blobonereadrole", "blobonewriterole"]); }); options.AddPolicy("blob-one-write-policy", policyBlobOneRead => { policyBlobOneRead.RequireClaim("roles", ["blobonewriterole"]); }); }); services.AddRazorPages().AddMvcOptions(options => { var policy = new AuthorizationPolicyBuilder() .RequireAuthenticatedUser() .Build(); options.Filters.Add(new AuthorizeFilter(policy)); }).AddMicrosoftIdentityUI(); UploadThe application uses the IFormFile interface with the file payload and uploads the file to Azure Blob storage. The BlobClient is setup to use Microsoft Entra ID and the meta data is added to the blob.
public BlobDelegatedUploadProvider(DelegatedTokenAcquisitionTokenCredential tokenAcquisitionTokenCredential, IConfiguration configuration) { _tokenAcquisitionTokenCredential = tokenAcquisitionTokenCredential; _configuration = configuration; } [AuthorizeForScopes(Scopes = ["https://storage.azure.com/user_impersonation"])] public async Task<string> AddNewFile(BlobFileUploadModel blobFileUpload, IFormFile file) { try { return await PersistFileToAzureStorage(blobFileUpload, file); } catch (Exception e) { throw new ApplicationException($"Exception {e}"); } } private async Task<string> PersistFileToAzureStorage( BlobFileUploadModel blobFileUpload, IFormFile formFile, CancellationToken cancellationToken = default) { var storage = _configuration.GetValue<string>("AzureStorage:StorageAndContainerName"); var fileFullName = $"{storage}/{blobFileUpload.Name}"; var blobUri = new Uri(fileFullName); var blobUploadOptions = new BlobUploadOptions { Metadata = new Dictionary<string, string?> { { "uploadedBy", blobFileUpload.UploadedBy }, { "description", blobFileUpload.Description } } }; var blobClient = new BlobClient(blobUri, _tokenAcquisitionTokenCredential); var inputStream = formFile.OpenReadStream(); await blobClient.UploadAsync(inputStream, blobUploadOptions, cancellationToken); return $"{blobFileUpload.Name} successfully saved to Azure Blob Storage Container"; }The DelegatedTokenAcquisitionTokenCredential class is used to get access tokens for the blob upload or download. This uses the existing user delegated session and creates a new access token for the blob storage access.
using Azure.Core; using Microsoft.Identity.Client; using Microsoft.Identity.Web; namespace DelegatedEntraIDBlobStorage.FilesProvider.AzureStorageAccess; public class DelegatedTokenAcquisitionTokenCredential : TokenCredential { private readonly ITokenAcquisition _tokenAcquisition; private readonly IConfiguration _configuration; public DelegatedTokenAcquisitionTokenCredential(ITokenAcquisition tokenAcquisition, IConfiguration configuration) { _tokenAcquisition = tokenAcquisition; _configuration = configuration; } public override AccessToken GetToken(TokenRequestContext requestContext, CancellationToken cancellationToken) { throw new NotImplementedException(); } public override async ValueTask<AccessToken> GetTokenAsync(TokenRequestContext requestContext, CancellationToken cancellationToken) { string[]? scopes = _configuration["AzureStorage:ScopeForAccessToken"]?.Split(' '); if (scopes == null) { throw new Exception("AzureStorage:ScopeForAccessToken configuration missing"); } AuthenticationResult result = await _tokenAcquisition .GetAuthenticationResultForUserAsync(scopes); return new AccessToken(result.AccessToken, result.ExpiresOn); } } DownloadThe download creates a BlobClient using the user delegated existing session. The file is downloaded directly.
using Azure.Storage.Blobs; using Azure.Storage.Blobs.Models; using Microsoft.Identity.Web; namespace DelegatedEntraIDBlobStorage.FilesProvider.AzureStorageAccess; public class BlobDelegatedDownloadProvider { private readonly DelegatedTokenAcquisitionTokenCredential _tokenAcquisitionTokenCredential; private readonly IConfiguration _configuration; public BlobDelegatedDownloadProvider(DelegatedTokenAcquisitionTokenCredential tokenAcquisitionTokenCredential, IConfiguration configuration) { _tokenAcquisitionTokenCredential = tokenAcquisitionTokenCredential; _configuration = configuration; } [AuthorizeForScopes(Scopes = ["https://storage.azure.com/user_impersonation"])] public async Task<Azure.Response<BlobDownloadInfo>> DownloadFile(string fileName) { var storage = _configuration.GetValue<string>("AzureStorage:StorageAndContainerName"); var fileFullName = $"{storage}/{fileName}"; var blobUri = new Uri(fileFullName); var blobClient = new BlobClient(blobUri, _tokenAcquisitionTokenCredential); return await blobClient.DownloadAsync(); } } NotesThe architecture is simple and has the base features required for a secure solution. Data protection and virus scanning needs to be applied to the files and this can be configured in the Azure Blob storage. The access is controlled to the users in the group. If this needs to be controlled more, the write access can be removed from the users and switched to a service principal. This can have both security advantages and disadvantages. Multiple clients might also need access to files in this solution and the security needs to be enforced. This requires further architecture changes.
Linkshttps://learn.microsoft.com/en-us/azure/storage/blobs/authorize-access-azure-active-directory
https://docs.microsoft.com/en-us/azure/storage/blobs/storage-blobs-introduction
https://github.com/AzureAD/microsoft-identity-web
When a person is already logged into a mastodon instance, if they visit some pages on their instance associated with a user from another server, they are not redirected to the remote server because it is easier to interact with the remote user with their existing local session. However, if a person without an account is just visiting or they have an account but are logged out, mastodon redirects them to the remote server presumably because mastodon doesn’t know whether they have a local account and visiting the remote server will have the complete and authoritative data for that remote user.
A welcome update to mastodon.social (included in 4.3.0-nightly) is a warning presented to visitors or logged out users before mastodon redirects them to a remote server for the original page. The code for Add confirmation when redirecting logged-out requests to permalink is particularly relevant to mastodon.social compared to other fediverse instances as mastodon.social has become a relatively big target for phishing. It’s a good bet that if someone is navigating the fediverse that their account is on mastodon.social. So, if an arbitrary victim is logged out of their mastodon.social account and visits a mastodon.social page belonging to the attacker, prior to this mitigation, mastodon.social would automatically redirect the victim to the attacker’s page which might be a fake login form to trick the victim into submitting their login credentials to the attacker’s site. Unfortunately, a significant percentage of people will submit the form.
One could imagine mastodon.social maintaining a list of trusted servers for automatic redirects but that would be an undesirable hornet’s nest and it’s not a bad thing when web surfers are conscious of the trust boundaries on the web.
The physical world is full of zero trust examples, but they gather attributes for the access control decisions in a very different way than we're used to online.
Presenting your ID to buy beer is used so often as an example of how verifiable credentials work that it's cliche. Cliche or not, there's another aspect of using an ID to buy beer that I want to focus on: it's an excellent example of zero trust
Zero Trust operates on a simple, yet powerful principle: “assume breach.” In a world where network boundaries are increasingly porous and cyber threats are more evasive than ever, the Zero Trust model centers around the notion that no one, whether internal or external, should be inherently trusted. This approach mandates continuous verification, strict access controls, and micro-segmentation, ensuring that every user and device proves their legitimacy before gaining access to sensitive resources. If we assume breach, then the only strategy that can protect the corporate network, infrastructure, applications, and people is to authorize every access.
From Zero Trust
Referenced 2024-02-09T08:25:55-0500
The real world is full of zero trust examples. When we're controlling access to something in the physical world—beer, a movie, a boarding gate, points in a loyalty program, prescriptions, and so on—we almost invariably use a zero trust model. We authorize every access. This isn't surprising, the physical world is remarkably decentralized and there aren't many natural boundaries to exploit and artificial boundaries are expensive and inconvenient.
The other thing that's interesting about zero trust in the physical world is that authorization is also usually done using Zero Data. Zero data is a name StJohn Deakin gave to the concept of using data gathered just in time to make authorization and other decisions rather than relying on great stores of data. There are obvious security benefits from storing less data, but zero data also offers significantly greater convenience for people and organizations alike. To top all that off, it can save money by reducing the number of partner integrations (i.e., far fewer federations) and enable applications that have far greater scale.
Let's examine these benefits in the scenario I opened with. Imagine that instead of using a credential (e.g., driver's license) to prove your age when buying beer, we ran convenience stores like a web site. Before you could shop, you'd have to register an account. And if you wanted to buy beer, the company would have to proof the identity of the person to ensure they're over 21. Now when you buy beer at the store, you'd log in so the system could use your stored attributes to ensure you were allowed to buy beer.
This scenario is still zero trust, but not zero data. And it's ludicrous to imagine anyone would put up with it, but we do it everyday online. I don't know about you, but I'm comforted to know that every convenience store I visit doesn't have a store of all kinds of information about me in an account somewhere. Zero data stores less data that can be exploited by hackers (or the companies we trust with it).
The benefit of scale is obvious as well. In a zero data, zero trust scenario we don't have to have long-term transactional relationships with every store, movie, restaurant, and barber shop we visit. They don't have to maintain federation relationships with numerous identity providers. There are places where the ability to scale zero trust really matters. For example, it's impossible for every hospital to have a relationship with every other hospital for purposes of authorizing access for medical personal who move or need temporary access. Similarly, airline personal move between numerous airports and need access to various facilities at airports.
How do we build zero data, zero trust systems? By using verifiable credentials to transfer attributes about their subject in a way that is decentralized and yet trustworthy. Zero data aligns our online existence more closely with our real-world interactions, fostering new methods of communication while decreasing the challenges and risks associated with amassing, storing, and utilizing vast amounts of data.
Just-in-time, zero data, attribute transfer can make many zero trust scenarios more realizable because it's more flexible. Zero trust with zero data, facilitated by verifiable credentials, represents a pivotal transition in how digital identity is used in authorization decisions. By minimizing centralized data storage and emphasizing cryptographic verifiability, this approach aims to address the prevalent challenges in data management, security, and user trust. By allowing online interactions to more faithfully follow established patterns of transferring trust from the physical world, zero trust with zero data promotes better security with increased convenience and lower cost. What's not to like?
You can get more detail on many of the concepts in this post like verifiable credentials in my new book Learning Digital Identity from O'Reilly Media.
Photo Credit: We ID Everyone from DALL-E (Public Domain) DALL-E apparently thinks a six-pack has 8 bottles but this was the best of several attempts.
This article shows how to implement an ASP.NET Core Blazor Web application using Microsoft Entra ID for authentication. Microsoft.Identity.Web is used to implement the Microsoft Entra ID OpenID Connect client.
Code: https://github.com/damienbod/Hostedblazor8MeID
Note: I based this implementation on the example provided by Tomás López Rodríguez and adapted it.
SetupThe Blazor Web application is an OpenID Connect confidential client (code flow, PKCE) which uses Microsoft Entra ID for authentication. An Azure App registration (Web configuration) is used to create the client and only delegated scopes are used. A secret is used to authenticate the application in development. Client assertions can be used in production deployments. NetEscapades.AspNetCore.SecurityHeaders is used to implement the security headers as best possible for Blazor Web. No identity management or user passwords are handled in the application.
The client part of the Blazor Web application can use the PersistentAuthenticationStateProvider class to read the user profile data.
This uses data from the server part implemented in the PersistingRevalidatingAuthenticationStateProvider class. See the code in the github repo.
OpenID Connect confidential clientThe AddMicrosoftIdentityWebAppAuthentication method is used to implement the client authentication using the Microsoft.Identity.Web packages. I use a downstream API to force that the client uses code flow with PKCE instead of the implicit flow. Microsoft Graph is only requesting delegated user profile data.
// Add authentication services var scopes = builder.Configuration.GetValue<string>("DownstreamApi:Scopes"); string[] initialScopes = scopes!.Split(' '); builder.Services.AddMicrosoftIdentityWebAppAuthentication(builder.Configuration) .EnableTokenAcquisitionToCallDownstreamApi(initialScopes) .AddMicrosoftGraph("https://graph.microsoft.com/v1.0", scopes) .AddInMemoryTokenCaches();The client automatically reads from the AzureAd configuration. This can be changed if you would like to update the product name. The client uses the standard Microsoft Entra ID setup. You need to add the permissions in the Azure App registration created for this application.
"AzureAd": { "Instance": "https://login.microsoftonline.com/", "Domain": "[Enter the domain of your tenant, e.g. contoso.onmicrosoft.com]", "TenantId": "[Enter 'common', or 'organizations' or the Tenant Id (Obtained from the Azure portal. Select 'Endpoints' from the 'App registrations' blade and use the GUID in any of the URLs), e.g. da41245a5-11b3-996c-00a8-4d99re19f292]", "ClientId": "[Enter the Client Id (Application ID obtained from the Azure portal), e.g. ba74781c2-53c2-442a-97c2-3d60re42f403]", "ClientSecret": "[Copy the client secret added to the app from the Azure portal]", "ClientCertificates": [ ], // the following is required to handle Continuous Access Evaluation challenges "ClientCapabilities": [ "cp1" ], "CallbackPath": "/signin-oidc" }, "DownstreamApi": { "Scopes": "User.ReadBasic.All user.read" }, Login and LogoutAn AuthenticationExtensions class was used to implement the login and the logout for the application. The Login method is an HTTP GET request which redirects to the OpenID Connect server. The Logout method is an authentication HTTP POST request which requires CSRF protection and accepts no parameters. The return URL to the unauthenticated signed out page is fixed and so no open redirect attacks are possible. The login cleans up the local cookies as well as a redirect to the identity provider to logout on Microsoft Entra ID.
using Microsoft.AspNetCore.Authentication.Cookies; using Microsoft.AspNetCore.Authentication.OpenIdConnect; using Microsoft.AspNetCore.Authentication; namespace BlazorWebMeID; public static class AuthenticationExtensions { public static WebApplication SetupEndpoints(this WebApplication app) { app.MapGet("/Account/Login", async (HttpContext httpContext, string returnUrl = "/") => { await httpContext.ChallengeAsync(OpenIdConnectDefaults.AuthenticationScheme, new AuthenticationProperties { RedirectUri = !string.IsNullOrEmpty(returnUrl) ? returnUrl : "/" }); }); app.MapPost("/Account/Logout", async (HttpContext httpContext) => { var authenticationProperties = new AuthenticationProperties { RedirectUri = "/SignedOut" }; await httpContext.SignOutAsync(OpenIdConnectDefaults.AuthenticationScheme, authenticationProperties); await httpContext.SignOutAsync(CookieAuthenticationDefaults.AuthenticationScheme); }).RequireAuthorization(); return app; } } Security headersThe security headers are used to protect the session. When using AddInteractiveWebAssemblyComponents mode, the script CSP header is really weak and adds little protection leaving the application open to numerous XSS, Javascript attacks. It is not possible to use CSP nonces with Blazor Web using the InteractiveWebAssemblyComponents mode, or I have not found a way to do this, as the Blazor Web components cannot read the HTTP headers in the response. A Blazor WASM hosted in an ASP.NET Core application can use CSP nonces and is a more secure application.
namespace HostedBlazorMeID.Server; public static class SecurityHeadersDefinitions { public static HeaderPolicyCollection GetHeaderPolicyCollection(bool isDev, string? idpHost) { ArgumentNullException.ThrowIfNull(idpHost); var policy = new HeaderPolicyCollection() .AddFrameOptionsDeny() .AddContentTypeOptionsNoSniff() .AddReferrerPolicyStrictOriginWhenCrossOrigin() .AddCrossOriginOpenerPolicy(builder => builder.SameOrigin()) .AddCrossOriginResourcePolicy(builder => builder.SameOrigin()) .AddCrossOriginEmbedderPolicy(builder => builder.RequireCorp()) .AddContentSecurityPolicy(builder => { builder.AddObjectSrc().None(); builder.AddBlockAllMixedContent(); builder.AddImgSrc().Self().From("data:"); builder.AddFormAction().Self().From(idpHost); builder.AddFontSrc().Self(); builder.AddStyleSrc().Self(); builder.AddBaseUri().Self(); builder.AddFrameAncestors().None(); // due to Blazor Web, nonces cannot be used with AddInteractiveWebAssemblyComponents mode. // weak script CSP.... builder.AddScriptSrc() .Self() // self required .UnsafeEval() // due to Blazor WASM .UnsafeInline(); // only a fallback for older browsers when the nonce is used }) .RemoveServerHeader() .AddPermissionsPolicy(builder => { builder.AddAccelerometer().None(); builder.AddAutoplay().None(); builder.AddCamera().None(); builder.AddEncryptedMedia().None(); builder.AddFullscreen().All(); builder.AddGeolocation().None(); builder.AddGyroscope().None(); builder.AddMagnetometer().None(); builder.AddMicrophone().None(); builder.AddMidi().None(); builder.AddPayment().None(); builder.AddPictureInPicture().None(); builder.AddSyncXHR().None(); builder.AddUsb().None(); }); if (!isDev) { // maxage = one year in seconds policy.AddStrictTransportSecurityMaxAgeIncludeSubDomains(); } policy.ApplyDocumentHeadersToAllResponses(); return policy; } } NotesI am starting to understand how Blazor Web works and have difficultly with the session state and sharing this between different components. Some basic browser security cannot be used, i.e. CSP nonces. The mixed mode has strange UI effects which I could not clean up.
There are now four types of Blazor applications.
Blazor WASM hosted in an ASP.NET Core application Blazor Server Blazor Web Blazor WASM standaloneBlazor WASM hosted in an ASP.NET Core application and Blazor Server can be secured in a good way using the recommended security best practices (OpenID Connect confidential client). Blazor Web can implement a confidential client but is missing the recommend script session protection. Blazor WASM standalone cannot implement the recommended authentication as it is a public application and should no longer be used in secure environments.
Linkshttps://github.com/CrahunGit/Auth0BlazorWebAppSample/tree/master/BlazorApp4
https://github.com/dotnet/blazor-samples/tree/main/8.0/BlazorWebAppOidc
https://github.com/AzureAD/microsoft-identity-web
https://github.com/andrewlock/NetEscapades.AspNetCore.SecurityHeaders
As I started digging into the jobs and tools for the Postgres extension ecosystem as part of my new gig, I realized that most people have little knowledge of the PGXN architecture. I learned a lot designing PGXN and its services, and am quite pleased with where it ended up, warts and all. So I thought it worthwhile to put together a brief presentation on the fundamental design principals (static REST file API), inter-related services (root mirror, manager, API, site) and tools (CLI, CI/CD).
Yesterday, the Tembo blog published the presentation, including the video and slides, along with a high-level architecture diagram. I hope it’s a useful point of reference for the Postgres community as we look to better distribute extensions in the future.
More about… PGXN Software Architecture REST JSON TemboAs I think through the future of the Postgres extension ecosystem as a key part of the new job, I wanted to understand how Go decentralized publishing works. In this post I work it out, and think through how we might do something similar for Postgres extension publishing. It covers the Go architecture, namespacing challenges, and PGXS abuse; then experiments with URL-based namespacing and ponders reorganizing installed extension files; and closes with a high-level design for making it work now and in the future.
It is, admittedly, a lot, mainly written for my own edification and for the information of my fellow extension-releasing travelers.
I find it fascinating and learned a ton. Maybe you will too! But feel free to skip this post if you’re less interested in the details of the journey and want to wait for more decisive posts once I’ve reached the destination.
IntroductionMost language registries require developers to take some step to make releases. Many automate the process in CI/CD pipelines, but it requires some amount of effort on the developer’s part:
Register for an account Learn how to format things to publish a release Remember to publish again for every new version Create a pipeline to automate publishing (e.g., a GitHub workflow) Decentralized PublishingGo decentralized publishing has revised this pattern: it does not require user registration or authentication to to publish a module to pkg.go.dev. Rather, Go developers simply tag the source repository, and the first time someone refers to the tag in Go tools, the Go module index will include it.
For example, publishing v1.2.1
of a module in the github.com/golang/example
repository takes just three commands:
git tag v1.2.1 -sm 'Tag v1.2.1'
git push --tags
go list -m github.com/golang/example@v1.2.1
After a few minutes, the module will show up in the index and then on
pkg.go.dev. Anyone can run go get -u github.com/golang/example
to get the
latest version. Go developers rest easy in the knowledge that they’re getting
the exact module they need thanks to the global checksum database, which Go
uses “in many situations to detect misbehavior by proxies or origin servers”.
This design requires go get
to understand multiple source code management
systems: it supports Git, Subversion, Mercurial, Bazaar, and Fossil.1
It also needs the go.mod
metadata file to live in the project defining the
package.
But that’s really it. From the developer’s perspective it could not be easier to publish a module, because it’s a natural extension of the module development tooling and workflow of committing, tagging, and fetching code.
Decentralized Extension PublishingCould we publish Postgres extensions in such a decentralized pattern? It might look something like this:
The developer places a metadata file in the proper location (control file,META.json
, Cargo.toml
, whatever — standard TBD)
To publish a release, the developer tags the repository and calls some sort
of indexing service hook (perhaps from a tag-triggered release workflow)
The indexing service validates the extension and adds it to the index
Note that there is no registration required. It simply trusts the source code
repository. It also avoids name collision: github.com/bob/hash
is distinct from github.com/carol/hash
.
This design does raise challenges for clients, whether they’re compiling extensions on a production system or building binary packages for distribution: they have to support various version control systems to pull the code (though starting with Git is a decent 90% solution).
NamespacingThen there’s name conflicts. Perhaps github.com/bob/hash
and
github.com/carol/hash
both create an extension named hash
. By the current
control file format, the script directory and module path can use any name,
but in all likelihood the use these defaults:
directory = 'extension'
module_pathname = '$libdir/hash'
Meaning .sql
files will be installed in the Postgres share/extension
subdirectory — along with all the other installed extensions — and library
files will be installed in the library directory along with all other libraries.
Something like this:
pgsql
├── lib
│ └── hash.so
└── share
└── extension
│ └── hash.control
│ ├── hash--1.0.0.sql
└── doc
└── hash.md
If both projects include, say, hash.control
, hash--1.0.0.sql
, and hash.so
,
the files from one will stomp all over the files of the other.
Go avoids this issue by using the domain and path from each package’s repository
in its directory structure. For example, here’s a list of modules from
google.golang.org
repositories:
$ ls -1 ~/go/pkg/mod/google.golang.org
api@v0.134.0
api@v0.152.0
appengine@v1.6.7
genproto
genproto@v0.0.0-20230731193218-e0aa005b6bdf
grpc@v1.57.0
grpc@v1.59.0
protobuf@v1.30.0
protobuf@v1.31.0
protobuf@v1.32.0
The ~/go/pkg/mod
directory has subdirectories for each VCS host name, and each
then subdirectories for package paths. For the github.com/bob/hash
example,
the files would all live in ~/go/pkg/mod/github.com/bob/hash
.
Could a Postgres extension build tool follow a similar distributed pattern by
renaming the control file and installation files and directories to something
specific for each, say github.com+bob+hash
and github.com+carol+hash
? That
is, using the repository host name and path, but replacing the slashes in the
path with some other character that wouldn’t create subdirectories — because
PostgreSQL won’t find control files in subdirectories. The control file entries
for github.com/carol/hash
would look like this:
directory = 'github.com+carol+hash'
module_pathname = '$libdir/github.com+carol+hash'
Since PostgreSQL expects the control file to have the same name as the extension, and for SQL scripts to start with that name, the files would have to be named like so:
hash
├── Makefile
├── github.com+carol+hash.control
└── sql
└── github.com+carol+hash--1.0.0.sql
And the Makefile
contents:
EXTENSION = github.com+carol+hash
MODULEDIR = $(EXTENSION)
DATA = sql/$(EXTENSION)--1.0.0.sql
PG_CONFIG ?= pg_config
PGXS := $(shell $(PG_CONFIG) --pgxs)
include $(PGXS)
In other words, the extension name is the full repository host name and path and
the Makefile MODULEDIR
variable tells pg_config
to put all the SQL and
documentation files into a directories named github.com+carol+hash
—
preventing them from conflicting with any other extension.
Finally, the github.com+carol+hash.control
file — so named becaus it must
have the same name as the extension — contains:
default_version = '1.0.0'
relocatable = true
directory = 'github.com+carol+hash'
module_pathname = '$libdir/github.com+carol+hash'
Note the directory
parameter, which must match MODULEDIR
from the
Makefile
, so that CREATE EXTENSION
can find the SQL files. Meanwhile,
module_pathname
ensures that the library file has a unique name — the same
as the long extension name — again to avoid conflicts with other projects.
That unsightly naming extends to SQL: using the URL format could get to be a mouthful:
CREATE EXTENSION "github.com+carol+hash";
Which is do-able, but some new SQL syntax might be useful, perhaps something like:
CREATE EXTENSION hash FROM "github.com+carol+hash";
Or, if we’re gonna really go for it, use slashes after all!
CREATE EXTENSION hash FROM "github.com/carol/hash";
Want to use both extensions but they have conflicting objects (e.g., both create
a “hash” data type)? Put them into separatre schemas (assuming
relocatable = true
in the control file):
CREATE EXTENSION hash FROM "github.com/carol/hash" WITH SCHEMA carol;
CREATE EXTENSION hash FROM "github.com/bob/hash" WITH SCHEMA bob;
CREATE TABLE try (
h1 carol.hash,
h2 bob.hash
);
Of course it would be nice if PostgreSQL added support for something like Oracle packages, but using schemas in the meantime may be sufficient.
Clearly we’re getting into changes to the PostgreSQL core, so put that aside and we can just use long names for creating, modifying, and dropping extensions, but not necessarily otherwise:
CREATE EXTENSION "github.com+carol+hash" WITH SCHEMA carol;
CREATE EXTENSION "github.com+bob+hash" WITH SCHEMA bob;
CREATE EXTENSION "gitlab.com+barack+kicker_type";
CREATE TABLE try (
h1 carol.hash,
h2 bob.hash
kt kicker
);
Namespacing Experiment
To confirm that this approach might work, I committed 24134fd and pushed it in
the namespace-experiment branch of the semver extension. This commit changes
the extension name from semver
to github.com+theory+pg-semver
, and follows
the above steps to ensure that its files are installed with that name.
Abusing the Postgres extension installation infrastructure like this does work, but suffers from a number of drawbacks, including:
The extension name is super long, as before, but now so too are the files in the repository (as opposed to the installer renaming them on install). The shared library file has to have the long name, so therefore does the.c
source file. The SQL files must all start with
github.com+theory+pg-semver
, although I skipped that bit in this commit;
instead the Makefile
generates just one from sql/semver.sql
.
Any previous installation of the semver
type would remain unchanged, with
no upgrade path. Changing an extension’s name isn’t a great idea.
I could probably script renaming and modifying file contents like this and make
it part of the build process, but it starts to get complicated. We could also
modify installers to make the changes, but there are a bunch of moving parts
they would have to compensate for, and given how dynamic this can be (e.g., the
semver Makefile
reads the extension name from META.json
), we would rapidly
enter the territory of edge case whac-a-mole. I suspect it’s simply too
error-prone.
Perhaps the Go directory pattern could inspire a similar model in Postgres, eliminating the namespace issue by teaching the Postgres extension infrastructure to include all but one of the files for an extension in a single directory. In other words, rather than files distributed like so for semver:
pgsql
├── lib
│ └── semver.so
└── share
└── extension
│ └── semver.control
│ ├── semver--0.32.1.sql
│ ├── semver--0.32.0--0.32.1.sql
└── doc
└── semver.md
Make it more like this:
pgsql
└── share
└── extension
└── github.com
└── theory
└── pg-semver
└── extension.control
└── lib
│ └── semver.so
└── sql
│ └── semver--0.32.1.sql
│ └── semver--0.32.0--0.32.1.sql
└── doc
└── semver.md
Or perhaps:
pgsql
└── share
└── extension
└── github.com
└── theory
└── pg-semver
└── extension.control
└── semver.so
└── semver--0.32.1.sql
└── semver--0.32.0--0.32.1.sql
└── semver.md
The idea is to copy the files exactly as they’re stored in or compiled in the
repository. Meanwhile, the new semver.name
file — the only relevant file
stored outside the extension module directory — simply points to that path:
github.com/theory/pg-semver
Then for CREATE EXTENSION semver
, Postgres reads semver.name
and knows where
to find all the files to load the extension.
This configuration would require updates to the control file, now named
extension.control
, to record the full package name and appropriate locations.
Add:
name = 'semver'
package = 'github.com/theory/pg-semver'
This pattern could also allow aliasing. Say we try to install a different
semver
extension from github.com/example/semver
. This is in its
extension.control
file:
name = 'semver'
package = 'github.com/example/pg-semver'
The installer detects that semver.name
already exists for a different package
and raises an error. The user could then give it a different name by running
something like:
make install ALIAS_EXTENSION_NAME=semver2
This would add semver2.name
right next to semver.name
, and its contents
would contain github.com/example/semver
, where all of its files are installed.
This would allow CREATE EXTENSION semver2
to load the it without issue
(assuming no object conflicts, hopefully resolved by relocate-ability).
I realize a lot of extensions with libraries could wreak some havoc on the library resolver having to search so many library directories, but perhaps there’s some way around that as well? Curious what techniques experienced C developers might have adopted.
Back to Decentralized PublishingAn updated installed extension file structure would be nice, and is surely worth a discussion, but even if it shipped in Postgres 20, we need an updated extension ecosystem today, to work well with all supported versions of Postgres. So let’s return to the idea of decentralized publishing without such changes.
I can think of two pieces that’d be required to get Go-style decentralized extension publishing to work with the current infrastructure.
Module UniquenessThe first is to specify a new metadata field to be unique for the entire index,
and which would contain the repository path. Call it module
, after Go (a
single Git repository can have multiple modules). In PGXN Meta Spec-style JSON
it’d look something like this:
{
"module": "github.com/theory/pg-semver",
"version": "0.32.1",
"provides": {
"semver": {
"abstract": "A semantic version data type",
}
}
}
Switch from the PGXN-style uniqueness on the distribution name (usually the name
of the extension) and let the module be globally unique. This would allow
another party to release an extension with the same name. Even a fork where only
the module
is changed:
{
"module": "github.com/example/pg-semver",
"version": "0.32.1",
"provides": {
"semver": {
"abstract": "A semantic version data type",
}
}
}
Both would be indexed and appear under the module name, and both would be
find-able by the provided extension name, semver
.
Where that name must still be unique is in a given install. In other words,
while github.com/theory/pg-semver
and github.com/example/pg-semver
both
exist in the index, the semver
extension can be installed from only one of
them in a given Postgres system, where the extension name semver
defines its
uniqueness.
This pattern would allow for much more duplication of ideas while preserving the existing per-cluster namespacing. It also allows for a future Postgres release that supports something like the flexible per-cluster packaging as described above.2
Extension Toolchain AppThe second piece is an extension management application that understands all this stuff and makes it possible. It would empower both extension development workflows — including testing, metadata management, and releasing — and extension user workflows — finding, downloading, building, and installing.
Stealing from Go, imagine a developer making a release with something like this:
git tag v1.2.1 -sm 'Tag v1.2.1'
git push --tags
pgmod list -m github.com/theory/pg-semver@v1.2.1
The creatively named pgmod
tells the registry to index the new version
directly from its Git repository. Thereafter anyone can find it and install it
with:
pgmod get github.com/theory/pg-semver@v1.2.1
— installs the specified version
pgmod get github.com/theory/pg-semver
— installs the latest version
pgmod get semver
— installs the latest version or shows a list of
matching modules to select from
Any of these would fail if the cluster already has an extension named semver
with a different module name. But with something like the updated extension
installation locations in a future version of Postgres, that limitation could be
loosened.
Every new idea comes with challenges, and this little thought experiment is no exception. Some that immediately occur to me:
Not every extension can be installed directly from its repository. Perhaps the metadata could include a download link for a tarball with the results of any pre-release execution? Adoption of a new CLI could be tricky. It would be useful to include the functionality in existing tools people already use, like pgrx. Updating the uniqueness constraint in existing systems like PGXN might be a challenge. Most record the repository info in the resources META.json object, so it would be do-able to adapt into a new META format, either on PGXN itself or in a new registry, should we choose to build one. Getting everyone to standardize on standardized versioning tags might take some effort. Go had the benefit of controlling its entire toolchain, while Postgres extension versioning and release management has been all over the place. However PGXN long ago standardized on semantic versioning and those who have released extensions on PGXN have had few issues (one can still use other version formats in the control file, for better or worse). Some PGXN distributions have shipped different versions of extensions in a single release, or the same version as in other releases. The release version of the overall package (repository, really) would have to become canonical.I’m sure there are more, I just thought of these offhand. What have you thought of? Post ’em if you got ’em in the #extensions channel on the Postgres Slack, or give me a holler on Mastodon or via email.
Or does it? Yes, it does. Although the Go CLI downloads most
public modules from a module proxy server like proxy.golang.org
, it
still must know how to download modules from a version control system when
a proxy is not available. ↩︎
Assuming, of course, that if and when the Postgres core adopts more bundled packaging that they’d use the same naming convention as we have in the broader ecosystem. Not a perfectly safe assumption, but given the Go precedent and wide adoption of host/path-based projects, it seems sound. ↩︎
More about… Postgres PGXN Extensions Go Packaging Distributed PublishingOver on the PGXN Blog I’ve posted a brief update on recent bug fixes and improvements to the pgxn-tools Docker image, which is used fairly widely these days to test, bundle, and release Postgres extensions to PGXN. This fix is especially important for Git repositories:
v1.4.1 fixes an issue where
git archive
was never actually used to build a release zip archive. This changed at some point without noticing due to the introduction of thesafe.directory
configuration in recent versions of Git. Inside the container the directory was never trusted, and thepgxn-bundle
command caught the error, decided it wasn’t working with a Git repository, and used thezip
command, instead.
I also posted a gist listing PGXN distributions with a .git
directory.
The OpenID Federation editors were invited to give a presentation on OpenID Federation at the 18th FIM4R Workshop, which was held at the 2024 TIIME Unconference. Giuseppe De Marco, Roland Hedberg, John Bradley, and I tag-teamed the presentation, with Vladimir Dzhuvinov also participating in the Q&A. Topics covered included motivations, architecture, design decisions, capabilities, use cases, history, status, implementations, and people.
Here’s the material we used:
OpenID Federation 1.0: Shaping The Advanced Infrastructure of TrustIt was the perfect audience – chock full of people with practical federation deployment experience!
The “Fully-Specified Algorithms for JOSE and COSE” specification has been adopted by the JOSE working group. See my original post about the spec for why fully-specified algorithms matter. Thanks to all who supported adoption and also thanks to those who provided useful detailed feedback that we can address in future working group drafts.
The specification is available at:
https://www.ietf.org/archive/id/draft-ietf-jose-fully-specified-algorithms-00.htmlI woke up with piles of boxes filling one end of my living room. They’d been there since last weekend when I hauled them up from the basement and down from the third floor. Given our recent investigations into fascia, the psyche, and computation, perhaps the physicality of my cardboard wrangling was a subconscious tactic to help me process the loss. Contained within an assemblage of Playmobil, YA fantasy novels, vintage ephemera, and yellowed diplomas were the remaining belongings of two-thirds of my tiny nuclear family. At 8:30am movers were scheduled to arrive to whisk everything to storage, so I made sure to be up and out of the house early. Everything was organized and labelled with notes for my husband on what was to go, and which items were to be relocated in the house. It’s what moms do, right?
The night before I tucked away “Piggy,” my child’s beloved stuff animal companion, threadbare again after one flesh-colored terry cloth “chest” transplant. I gave him one last big hug and placed him gently next to “Brown Wolf,” both of them atop a quilt of wild 70s prints made by my grandmother in the summer camp trunk covered with Dolly’s Dairy Bar stickers, a Brevard, NC staple. It is the end of an era. I wept softly and alone in the chill of the sewing room.
After I had a few years of parenting under my belt, I would proffer parents of infants this insight – no matter how terrible or how wonderful life is at any given moment, the one constant is change. Cherish the sweet moments and know that the miserable ones will pass eventually. It may seem like forever, but it isn’t. I still think it’s solid advice, though bittersweet all the same. I’ve come to accept the situation and scrabble for scraps of grace to get through another day until the house sells and I can start to start over. We are still human, right? Feeling all of the big feelings is part of the job description, and yes, I’m here for it.
While packing up some lovely textiles woven by my husband’s late mother, I came across a few concert t-shirts from back in the day when he wore army jackets and his hair longish and was a DJ for late night hardcore shows that aired on college radio stations. Me? I was pretty square, naive, knew very little of the world. We met during a study abroad semester in Venice. On foggy fall nights we would walk holding hands to the tip of Dorsoduro, past the Salute plague church, to “the point,” Punta Della Dogana – young love. There we would sit watching the lights edging St. Mark’s Square where the Canale Grande met La Giudecca, the deep channel.
Back then I had no conception of water memory, Neptune, psi, or waves of consciousness. Unseen from street level, Atlas and Fortuna kept watch over the point, the latter holding a rudder aloft showing the direction of the wind, and our fate. I can look back and see my course was set when I was just a callow child off on a big adventure that would eventually sweep me to the City of Brotherly Love nestled between the Delaware and the Schuylkill, and then on, apparently, to the very different burbling 4,000-year-old waters of the Ozarks.
A few years back, my friend Dru shared with me the Chinese parable of the man who lost his horse. The gist was that when something happens in our lives that is apparently good or apparently bad, it is best to keep a neutral outlook, because the good might lead to the bad, or the bad to the good. Life cycles up and down. That our lives together started out under the globe of the heavens held by two atlas figures topped by a seventeenth century statue of Fortune charting human weather, commerce, navigation, bureaucratic systems…it’s quite a perfect summation of the tapestry I’ve been weaving without quite being aware of it until now.
Good? Bad? I guess I shall stay the course and see what comes next and try to hold onto my sense of wonder and playfulness as the trickster energy bubbles up around me.
I texted my soon-to-be-ex-husband a picture of “Misfits: Legacy of Brutality,” saying it seems the tables have turned. Now he’s an institutional administrator while I’ve gradually slid over into the outlier spot. Earlier in the process of letting go I spent a few days sifting through drawers of old cards and letters and report cards and crayon drawings, the fleeting realms of moms. Back then I was still a beloved wife and mother and daughter. The cards said so, at least. Now, I am an out-of-tune, dissonant dissident in a world rushing obliviously forward. Only a handful of people seem to be able to see and comprehend the layers of logic protocols and invisible sensors bathing us in invisible harmonic frequencies and smart cybernetic governance to tap into our soulful connection to the divine.
This is the world my child will navigate. Capably, I have no doubt.
And me? I will watch the phase shift coalesce from the sidelines. If I’m lucky I will view the etheric confluence barefoot peeking over a bed of glorious rainbow zinnias with a tomato sandwich in hand, juice running down my forearm, and when the sandwich is done, I will put a kayak on my aging Subaru and point it towards the crystal clear waters of Lake Ouachita.
I have a memory of being in a circle of families. Our children were part of a semester school in the Pisgah Forest south of Asheville. We were about to entrust our precious ones to four months of expeditionary learning, which in retrospect wasn’t my best parenting choice but you do the best with the information you have at the time. One of the teachers read Khalil Gibran’s poem “On Children.”
And a woman who held a babe against her bosom said, Speak to us of Children.
And he said:
Your children are not your children.
They are the sons and daughters of Life’s longing for itself.
They come through you but not from you,
And though they are with you yet they belong not to you.
You may give them your love but not your thoughts,
For they have their own thoughts.
You may house their bodies but not their souls,
For their souls dwell in the house of tomorrow, which you cannot visit, not even in your dreams.
You may strive to be like them but seek not to make them like you.
For life goes not backward nor tarries with yesterday.
You are the bows from which your children as living arrows are sent forth.
The archer sees the mark upon the path of the infinite, and He bends you with
His might that His arrows may go swift and far.
Let your bending in the archer’s hand be for gladness.
For even as He loves the arrow that flies, so He loves also the bow that is stable.
I remember being nonplussed and unsettled. I wasn’t ready, but we never are. I understand more about information fields and the divine creation and energy than I did then. My child did come through me, and settled so far, so far away, launched from breast milk, home cooked dinners from our CSA farm box, hand sewn Halloween costumes, art classes, bedtime stories, family trips, walks to the library, and kisses on the brow, even after adopting the habit of pushing me away in annoyance.
With everything in order, I headed out to get a leisurely breakfast at a restaurant that was about a twenty-minute walk from home just off the Ben Franklin Parkway, Mr. Electric himself. There was a steady rain washing away last week’s snow leaving craggy miniature mountains of ice chips languishing on the soggy drifts of leaves that didn’t manage to get bagged up during the fall – crystalline phase shift. It was a mild, drizzly rain, the kind that has a hint of the spring that is to come. There wasn’t much wind, and the misty droplets coated all the edges, plants and buildings with sparkly beads of water – billions of miniscule lenses abstracting and expanding the limits of the ordinary material world. It was magical.
Ironically the umbrella I’d grabbed wasn’t my regular rainbow one, or even the boring black one, but another that was emblazoned with the UPenn Arts and Sciences logo on it. The past week or so I’ve spent some time learning about Mark Johnson, a philosopher from the University of Oregon who spent his career talking about the ways we use our body to make meaning in the world. You see, our intelligence isn’t just in our brains and the nervous system that keeps it jumping, it’s also in our body and the liquid crystal fascia is an amazing part of that system. Art, culture, creativity, sports, dance are all gifts we toss back and forth with the quantum field of our collective consciousness.
These goofballs really want to meld all of that with natural philosophy, STEM we call it now, to create a unified computational system. This goal of melding social and natural sciences extends back at least to Leibniz who sought to create a universal computer where the grace of the human soul might be leveraged to shine a light on the secrets of the cosmos. I don’t think it’s likely to work, at least not how they think, but I chuckled all the same that the umbrella I’d unthinkingly grabbed on the way out the door had a special message for me. I was walking away from the life represented by that UPenn logo and soon heading south into the unknown, and the water was there as my witness.
In his “Stalking the Wild Pendulum” Itzhak Bentov wrote of our lives as embodied information organizers, pattern seekers, and meaning makers. Bentov felt that as we make sense of the material world through our experiences, our epiphanies, heartbreaks, and joy, there are invisible tapestries woven on the warp and weft of psyche and biology. These complex works of art, infinitely varied, are gradually gifted to the universal mind upon the passing of our bodies. That vision really resonates with me, particularly in combination with Johnson’s theories about non-linguistic communication and the importance of art and culture and the movement of the body in carrying out that important work on both and individual and social level.
Stephers shared with me a fascinating paper about social systems and how we use artefacts to leave traces of ourselves on our environments and those traces then influence the behaviors of others. Our use of cultural artefacts to imprint our consciousness on the energetic fields of the universe that vibrates around us is similar to the concept of pheromones that coordinate the collective efforts of eusocial creatures like ants or termites. My sense is that Web3 hopes to make cultural artefacts calculable at scale, converting them into universal coordinating signals to coax digital harmonies from the remaining wild corners that are slowly being overtaken by silicon. I mapped out some notes on this in my Dallas Mythos map below.
Source: https://embed.kumu.io/dc9b79f81e2bb35bc4fce22d59dde62b#untitled-map?s=bm9kZS1iU0kxa29DZA%3D%3DWhat follows are images from my walk to breakfast and back, with a slight extension of the route to the former GlaxoSmithKline US headquarters that has since been turned into a String Theory charter school. This embodied meaning making exhorts us to go out into the world, the real world and see what’s out there to be seen! For me, since everything turned upside down, I’ve felt inclined to stay close to home and disconnect. Maybe it’s not a healthy way to be, but for right now cocooning feels right. Still, when I go out with intention and my eyes open, the universe tells me stories and leaves me clues. No, not like I’m “hearing” things or “getting downloads,” more like I’m just aligned with the symbolic language of the universe and open to exploring in a wondering, wandering way.
First, I encountered a large puddle at an intersection. I paused for a few minutes watch the ripples of the raindrops and all of the rings that danced across the surface of the water. Bentov’s book spoke of information being stored in interference patterns. He described a three-pebble experiment where the ripples where flash frozen into a holographic information storage system in the water. In my case there weren’t pebbles, but dancing drops that captivated me.
And then just a half block down I had to stop again and take a picture of this surprising gift – a fish ornamented with spirals swimming through a colorful mosaic sea, as if to remind me that we don’t get to choose our river. We can, however, pay attention and find the best way to work with the flow.
And after I finished my mushroom toast, all the better to get in touch with the mycelial elements, I came out of the restaurant and saw that in the chainlink fence surrounding the empty lot across the street someone had created an impromptu party cup installation, mashing the plastic into the gaps to spell out the word “HOPE” in crude, but very legible letters. I smiled.
At this point I decided to go a bit further afield rather than head straight back to the house. I passed the Temple of the Church of Jesus Christ of Latter Day Saints, which stands about a block and a half from the Catholic Cathedral of Saints Peter and Paul.
And between the two on a sidewalk above the I-676 crosstown highway was a pretty lame art installation of Ben Franklin with his lightening bolts and keys, all electrical potential and trusted cryptography and Metaverse portals. It’s all right there if you have the eyes to see it. In the background is the former GlaxoSmithKline building.
I knew I wanted to take a photo of the Zenos Frudakis 2000 bronze sculpture “Freedom.” Zenos is the name of an Old Testament prophet featured in the Book of Mormon, which is interesting since you can see the temple from the piece. The composition was inspired by Rodin’s “Gates of Hell,” in turn inspired by Dante, which can be found a few blocks further along the Parkway.
I’d seen the piece a few times before in passing, but I’d never stopped to look at it very closely. I was kind of shocked.
The image conveys a person escaping the confines of the background block. For me, however, knowing the multiverse phase shift that is underway this “freedom,” especially one sponsored by a company working with Google Alphabet’s Verily on electroceutical development, feels a bit off.
Could it be the animal skull at the base?
Or the smirking cat staring out?
Or an incongruous jester, which is totally out of keeping wit the rest of the piece?
The texture of the figures spoke to me of fascia – movement, communication through embodiment, fractal tissues, today glistening with water.
Bodies at different scales embedded in their environment, a miniature twin tucked in one corner.
I felt drawn to reach out and put my hand on the outstretched hand. It reminded me of my dad, whose hands were so big and the way his fingers would curl down over mine and give them a firm squeeze. I miss that.
As I headed west to go home there was a colorfully odd sticker on a metal plate. A ghost with rainbow balloons – the souls are with us in all of their photonic playfulness.
Tucked up next to the retaining was of the highway was a clutch of pine trees with an abundance of small cones scattered on the pavers below, which I took as a sign that I should fill my pockets and proceed to Sister Cities Park.
It’s an uptight pocket park, managed within an inch of its life in that Disneyesque, public-private partnership, “don’t walk on the grass”, “we have the rights to all pictures taken of you here,” and “yes, you need a permit authorization to do anything fun,” neutered nature tamed and contained vibe.
Oh, and this is the first time I noticed a wayside elucidating the fact that the site was formerly a pauper’s burial ground and execution site. Not sure how our Sister Cities, Tel Aviv among them, would feel if they knew. St. Peter in his alcove with his keys overlooks the crystal fountain (based in Toronto). At the corner where the park met the sidewalk was Robert Indiana’s Latin take on his iconic Love statue. “Amor” commissioned in 2015 for the papal visit and World Meeting of Families.
Of course I wanted to leave a heart on the LED light fountain. Today’s kids don’t get swimming pools, just interactive splash pads. Who knows how that’s going to fit into stigmergic social computational artefacts once we’re all hydrosapiens. As I was laying down the pine cones the security guard hustled over. Now, it’s January and raining and no one but me is in the park, so she must have been inside watching me on some closed circuit cameras. She asked what I was doing and I replied what does it look like I’m doing? She said are those acorns? I said they were pine cones and just kept doing what I was doing. She turned and left saying I had to “clean it up.” I encircled the heart with needles from a few pine branches than had dropped in the storm and set an intention. Then I crossed over to the Cathedral. As soon as I stepped away she swooped in with a broom and dustpan to rid the park of my dissonant heart. But she didn’t pick up the actual litter under the bench even after I pointed it out. Then she and the other guard went and hid inside the closed cafe.
So I decided to make another heart. This one at the base of the Shakepeare statue outside the library – all the world’s a stage. I used rock salt left over on the sidewalks for the outline and filled it in with small magnolia pods and a few springs of yarrow that were unbelievably still a very cheery yarrow. I guess I should cut the security guard some slack. It is a spectacle after all, and she was simply playing her assigned archetypal role.
I went inside the library thinking that I would take a photo of the computer terminals in the children’s department that annoyed me so much when we made visits when my child was so little. I was pleasantly surprised to see that the beeping, jarring computer game terminals for toddlers were gone. Yay. I did see a display in the lobby that the public transit division wanted your daydreams, which is pretty creepy. I also had a long and rewarding conversation with the social worker who was manning the services desk in the lobby. I explained to her about social impact bonds and pay for success and blockchaining unhoused people (MyPass in Austin) and offered my theory that it was about biohybrid computing. She looked up Michael Levin on her phone and seemed genuinely interested, which gave some meaning to a morning that would otherwise have been rather bleak.
Three is such a nice number, so I decided to make a final heart on the fountain outside the Barnes Foundation – talk about the power of curated artifacts! I remember back to the lockdown days when a few of us did an esoteric tour of the Parkway and left some lovely crystals in the water. The water was turned off for the season, but there was a bit in one corner from the rain. This one was simple – not too much material around – twigs, sycamore ball fluff, an auburn oak leaf, and a smidge of pine.
The rest of the way home was pretty uneventful. I stopped to dip my hand in the water of the PeCO electrical manhole cover – liquid portal.
I saw an acupuncture storefront with the sign for Still Waters.
And a window with artwork that looked like a black and white concept game – the Niantic Pokemon Go version of digital “Playful Cities” in abstracted, quantifiable reality.
And as I got to my house I saw my tree, that I will miss very much. In the city you only have room for at most one tree in the front. Ours was a thornless honey locust and we put it in when we bought our house in 1998. The past year or so the bark started peeling back and I noticed some larvae under it and I was worried for it. We had an injection put into the roots to stop the sucking bugs. A big hunk of bark fell off last year and I was very upset. But now six months later it’s healing up with new bark. You can still see the scarred place, but I want to believe it will bounce back, even in spite of all the incessant geoengineering.
I am a Sagitarrius and we are rather mercurial, but as spring arrives I will try to remember the parable of the man who lost his horse. Good-bad, bad-good – we just need to practice swimming in the flow in which we’ve found ourselves. I hope you’ve enjoyed coming with me on this damp stroll through the city that I loved and that I’m leaving. I’m sure I will find new stories in new places to share.
Here’s the latest installment in the series on working with LLMS: How to Learn Unfamiliar Software Tools with ChatGPT.
Ideally, tools like GeoGebra and Metabase provide interfaces so intuitive that you rarely need to read the docs, and you can learn the software just by poking around in it. In reality, of course, we need those docs — and they still need to be excellent. But now, we’ll extract a new benefit from them. When we can partner with machines that have read the docs, and can look over our shoulders as we try to do the things described in the docs, we’ll turbocharge our ability to dive into unfamiliar software tools and quickly learn how to use them.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
12 Let’s talk: conversational software development
13 Using LLMs to Improve SQL Queries
14 Puzzling over the Postgres Query Planner with LLMs
Thoughts on Self-Sovereign Identity: A Systematic Review, Mapping and Taxonomy
I ran across a paper named Self-Sovereign Identity: A Systematic Review, Mapping and Taxonomy. It was published mid-2022 so it is not the most up to date for the topic but it is very interesting and still of high value. It’s a meta-study of four research questions about self-sovereign identity.
RQ-1: What Practical Problems Have Been Introduced and Solved? RQ-2: What Properties, Formal Definitions and Cryptographic Tools Have Been Used? RQ-3: What Conceptual Ideas Have Been Introduced or Refuted? RQ-4: When, Where, and by Whom Were SSI Studies Published?It spends a lot of text before the research questions on how it built the study objectively, criteria for the data, and criteria for the inclusion of the other research papers. Though interesting, it was not what compelled me in the paper. As you might imagine, it was the red meat of the paper, the research questions, that I really found most interesting.
You’ll find in the research question sections that it does a nice inventory of various papers and a description of what they cover. I found RQ-1 to be the most interesting as it covers a lot of verifiable credentials and verifiable presentation topics.
Of RQ-1 I found section 6.2 to be of special interest. It covers:
The operational facet is divided into two facets: VC and VP.And includes:
Revocation Decentralized Identifiers Issuer Authorization Delegation Backup and RecoveryRQ-3 is a short section and starts with an interesting statement that is probably less true today than when written but still holds a lot of truth.
...that there is currently no agreement on a definition of SSI...Though I appreciate RQ-4 and it makes sense in the context of the paper, I found the least value in its presentation. It did remind me of a relationship graph I created a number of years back except that my graph was on the relationship of the specifications at the time. The header image of this post is a small rendering of that graph. You can find the useful version at Verifiable Credentials Specification Map. Reader beware that the specification map I list was last updated late May of 2021 so it is not an accurate source of the state of specifications for today though many of the relationships it does show are likely valid. This is really a topic for a different day.
All in all, despite the relative age of the paper, the other papers it refers to are often still valid today in their intent and basic questions, agreements, and refutations. I think it is well worth your time to look at the research questions portions if interested in self-sovereign identity (a phrase that seems to be moving more so out of popular use) and verifiable credentials.
I’ve happily used MS Paint as my basic bitmap editor since Windows 3, almost 25 years ago. Mostly I’ve used it to create images from screenshots, but that has suddenly become way harder. Formerly, when I’d cut a region, the now-empty region would display using the default white background. Now it displays a checkered background like so.
Here is the procedure to refill the white background:
Switch the foreground color to white Use the Fill tool to fill the checkered region Then switch the foreground back to black.ARE YOU KIDDING ME?
Nope. It’s evidently an unintended consequence of a pair of new feature: layers and transparency.
To get started, click on the new Layers button in the toolbar, which will open a panel on the side of the canvas.”
Microsoft also revealed today that an upcoming Paint feature is support for image transparency, which will add the ability to open and save transparent PNG files.
During editing, users will notice a prominent checkerboard pattern displayed on the canvas, serving as a visual indicator and highlighting the transparent regions within the image.
This ensures that when content is erased from the canvas, it is completely removed, eliminating the need to cover unwanted regions of an image with white fill.
I never asked for these “long-awaited” new features, Paint is (or was) useful to me precisely because it only does the kind of basic bitmap editing I need when compositing screenshots. But I can opt out, right?
Nope.
ARE YOU KIDDING ME?
Nope.
This feature (layers and image transparency) seems to be introduced in September 2023 and doesn’t actually allow to be turned off.
Doing what vengy proposes for each and every image being edited is a natural madness and will drive even the most sane person crazy.
What worked for me was to uninstall Paint and replace it with a classic version:
Uninstalling can be done by simply right-clicking Paint icon in Start Menu and selecting Uninstall from context menu. Classic Paint can be get from here or here. Download and install it. Go to Settings → Apps → Apps & Features → More settings → App execution aliases. Toggle the switch to Off for mspaint.exe and pbrush.exe items.
Evidently people are willing to hack their systems in order to revert to a now-unsupported version that they prefer. As insane as it would be, I’m considering whether to become one of those people. Sigh. I guess 25 years was a pretty good run.
Last week, I informally shared Extension Ecosystem: Jobs and Tools with colleagues in the #extensions channel on the Postgres Slack. The document surveys the jobs to be done by the ideal Postgres extension ecosystem and the suggests the tools and services required to do those jobs — without reference to existing extension registries and packaging systems.
The last section enumerates some questions we need to ponder and answer. The first one on the list is:
What will PGXN’s role be in this ideal extension ecosystem?
The PostgreSQL Extension Network, or PGXN, is the original extension distribution system, created 2010–11. It has been a moderate success, but as we in the Postgres community imagine the ideal extension distribution future, it’s worthwhile to also critically examine existing tools like PGXN, both to inform the project and to realistically determine their roles in that future.
With that in mind, I here jot down some thoughts on the challenges with PGXN.
PGXN ChallengesPGXN sets a lot of precedents, particularly in its decoupling of the registry from the APIs and services that depend on it. It’s not an all-in-one thing, and designed for maximum distributed dissemination via rsync and static JSON files.
But there are a number of challenges with PGXN as it currently stands; a sampling:
PGXN has not comprehensively indexed all public PostgreSQL extensions. While it indexes more extensions than any other registry, it falls far short of all known extensions. To be a truly canonical registry, we need to make it as simple as possible for developers to register their extensions. (More thoughts on that topic in a forthcoming post.)
In that vein, releasing extensions is largely a manual process. The pgxn-tools Docker image has improved the situation, allowing developers to create relatively simple GitHub workflows to automatically test and release extensions. Still, it requires intention and work by extension developers. The more seamless we can make publishing extensions the better. (More thoughts on that topic in a forthcoming post.)
It’s written in Perl, and therefore doesn’t feel modern or easily accessible to other developers. It’s also a challenge to build and distribute the Perl services, though Docker images could mitigate this issue. Adopting a modern compiled language like Go or Rust might increase community credibility and attract more contributions.
Similarly, pgxnclient is written in Python and the pgxn-utils developer tools in Ruby, increasing the universe of knowledge and skill required for developers to maintain all the tools. They’re also more difficult to distribute than compiled tools would be. Modern cross-compilable languages like Go and Rust once again simplify distribution and are well-suited to building both web services and CLIs (but not, perhaps native UX applications — but then neither are dynamic languages like Ruby and Python).
The PGXN Search API uses the Apache Lucy search engine library, a project that retired in 2018. Moreover, the feature never worked very well, thanks to the decision to expose separate search indexes for different objects — and requiring the user to select which to search. People often can’t find what they need because the selected index doesn’t contain it. Worse, the default index on the site is “Documentation”, on the surface a good choice. But most extensions include no documentation other than the README, which appears in the “Distribution” index, not “Documentation”. Fundamentally the search API and UX needs to be completely re-architected and -implemented.
PGXN uses its own very simple identity management and basic authentication. It would be better to have tighter community identity, perhaps through the PostgreSQL community account.
Given these issues, should we continue building on PGXN, rewrite some or all of its components, or abandon it for new services. The answer may come as a natural result of designing the overall extension ecosystem architecture or from the motivations of community consensus. But perhaps not. In the end, we’ll need a clear answer to the question.
What are your thoughts? Hit us up in the #extensions channel on the Postgres Slack, or give me a holler on Mastodon or via email. We expect to start building in earnest in February, so now’s the time!
More about… Postgres PGXN ExtensionsLast week, I flew to LA To attend the Children’s Digital Privacy Summit hosted by Denise Tayloe and her team at Privo. I’ve known Denise since the early days of IIW, and it was great to meet her team for the first time. They put on a great show what began with a talk […]
The post Event Reflection: Children’s Digital Privacy Summit 2024 appeared first on Identity Woman.
We can't have broad adoption of verifiable credentials until we find a way to scale their presentation by providing tooling that credential verifiers can use to reduce their risk and gain confidence in the facts presented to them.
When I hand a merchant in London a piece of plastic that I got from a bank in Utah to make a purchase, a tiny miracle happens. Despite the fact that the merchant has never met me before and has no knowledge of my bank, she blithely allows me to walk out of the store with hundreds of dollars of merchandise, confident that she will receive payment. I emphasized the word confident in the last sentence because it's core to understanding what's happened. In the past, these kinds of transactions required that the merchant trust me or my bank. But in the modern world, trust has been replaced by confidence.
We often mix these concepts up and I'm as guilty as anyone. But trust always involves an element of risk, whereas confidence does not. These are not binary, but rather represent a spectrum. In the scenario I paint above, the merchant is still taking some risk, but it's very small. Technology, processes, and legal agreements have come together to squeeze out risk. The result is a financial system where the risk is so small that banks, merchants, and consumers alike have confidence that they will not be cheated. There's a name in the financial services industry for the network that reduces risk so that trust can be replaced with confidence: an acceptance network.
Acceptance NetworksAn acceptance network is the network of merchants or service providers that accept a particular form of payment, usually credit or debit cards, from a particular issuer or payment network. The term refers to a broad ecosystem that facilitates these transactions, including point-of-sale terminals, online payment gateways, and other infrastructure. Each component of the acceptance network plays a crucial role in ensuring that transactions are processed efficiently, securely, and accurately. This drives out risk and increases confidence. Acceptance networks are foundational components of modern payment ecosystems and are essential to the seamless functioning of digital financial transactions. Visa, Mastercard, American Express, and Discover are all examples of acceptance networks.
Before the advent of acceptance networks, credit was a spotty thing with each large merchant issuing it's own proprietary credit card—good only at that merchant. My mom and dad had wallets full of cards for JC Penney, Sears, Chevron, Texaco, and so on. Sears trusted its card. Chevron trusted its card. But it was impossible to use a Chevron card at Sears. They had limited means to verify if it was real and no way to clear the funds so that Chevron could pay Sears for the transaction.
That scenario is similar to the state of digital identity today. We have identity providers (IdPs) like Google and Apple who control a closed ecosystem of relying parties (with a lot of overlap). These relying parties trust these large IdPs to authenticate the people who use their services. They limit their risk by only using IdPs they're familiar with and only accepting the (usually) self-asserted attributes from the IdP that don't involve much risk. Beyond that they must verify everything themselves.
Fixing this requires the equivalent of an acceptance network for digital identity. When we launched Sovrin Foundation and the Sovrin network1 in 2016, we were building an acceptance network for digital identity, even though we didn't use that term to describe it. Our goal was to create a system of protocols, processes, technology and governance that would reduce the risk of self-sovereign identity and increase confidence in an identity system that let the subjects present verifiable credentials that carried reliable attributes from many sources.
I've written previously about identity metasystems that provide a framework for how identity transactions happen. Individual identity systems are built according to the architecture and protocols of the metasystem. Acceptance networks are an instantiation of the metasystem for a particular set of users and types of transactions. A metasystem for self-sovereign identity might have several acceptance networks operating in it to facilitate the operation of specific identity systems.
Problems an Acceptance Network Can SolveTo understand why an acceptance network is necessary to reduce risk and increase confidence in identity transactions, let's explore the gaps that exist without it. The following diagram shows the now familiar triangle of verifiable credential exchange. In this figure, issuers issue credentials to holders who may or may not be the subject of the credentials. The holder presents cryptographic proofs that assert the value of relevant attributes using one of more of the credentials that they hold. The verifier verifies the proof and uses the attributes.
Verifiable Credential ExchangeLet's explore what it means for the verifier to verify the proof. The verifier wants to know a number of things about the credential presentation:
Were the credentials issued to the entity making the presentation?
Have any of the credentials been tampered with?
Have any of the credentials been revoked?
What are the schema for the credentials (to understand the data in them)?
Who issued the credentials in the proof?
The first four of these can be done cryptographically to provide confidence in the attestation. The technology behind the credential presentation is all that's necessary. They can be automated as part of the exchange. For example, the proof can contain pointers (e.g., DIDs) to the credential definitions. These could contain public keys for the credential and references to schema.
The last one—who issued the credential—is not a technical matter. To see why, imagine that Alice (as holder and subject) has been issued a credential from her university (the issuer) giving information about her educational experiences there. She's applying for a job and wants to present the credential to a prospective employer (the verifier). How does the employer know that Alice didn't just make the credential herself or buy it from a diploma mill?
Knowing who issued the credential is not something that can be done solely with technology (although it can help). The employer in this scenario wants more than an identifier for the issuer. And they want to know that the public key really does belong to the university. In short, the employer wants to resolve the identifier to other information that tells them something about the university and the credential. There are lots of ways to do that—people have been doing this sort of thing for centuries: states keep registries of businesses (universities are businesses), accreditation organizations keep registries of schools they've accredited, the Department of Education has registries of various institutions of higher education in the US, and so on.
The employer could make use of these by building its own database of university identifiers it trusts. And every time a new one shows up, they could investigate and add it to their registry (or not)2. But going back to the magic of the credit card scenario that I opened this article with, if every merchant had to keep their own registry of banks, the experience wouldn't be magical for me or the merchant. The financial acceptance network makes it easy for the merchant to have confidence that they'll be paid because they have not only technology, but processes, protocols, governance, and legal agreements that make the verification process automatable.
Acceptance Networks for Digital IdentityFor some use cases, keeping your own registry of the issuers you trust works. But for many, it's just too much work and makes it difficult to make use of a variety of credentials. This kind of "localized trust" is unwieldy in an identity system that might involve millions of issuers and identifiers and credentials for billions or even trillions of subjects. I've written extensively about identity metasystems and what they provide to help bridge the gap. This one, on how metasystems help provide life-like identity for digital systems is perhaps the most comprehensive. Acceptance networks implement metasystems.
An acceptance network for digital identity must have a number of important properties, including the following:
Credentials are decentralized and contextual—There is no central authority for all credentials. Every party can be an issuer, a holder (identity owner), or a verifier. Verifiable credentials can be adapted to any country, any industry, any community, or any set of trust relationships.
Credential issuers decide on what data is contained in their credentials—Anyone can create a credential schema for their use case. Anyone can create a credential definition based on any of these schemas.
Verifiers make their own trust decisions about which credentials to accept—There's no central authority who determines what credentials are important or which are used for what purpose. The acceptance network supplies the technical underpinnings for credential exchange and support protocols for automating the verification of credential issuers.
Credential verifiers don't need to have any specific technical, contractual, or commercial relationship with credential issuers—Verifiers do not need to contact issuers to perform verification.
Credential holders are free to choose which credentials to carry and what information to disclose—People and organizations are in control of the credentials they hold (just as they are with physical credentials) and determine what to share with whom.
You may be thinking "but these are mostly about decentralized decision making." While it would be easier to imagine the acceptance network as a big directory, that solution can't possible support all the different ways people and organizations might want to use credentials. That doesn't mean an acceptance network couldn't be run by a single organization, like some financial services networks. Just that it has to support a variety of credential ecosystems running common protocols. I also think that there will be more than one and most issuers and verifiers will be part of several (again, like in financial services).
Structure of an Acceptance NetworkOne of the things we can take away from the architecture of financial services acceptance networks is that they are built in layers. No one has thought more about how this can work than Drummond Reed and the Trust Over IP Foundation (ToIP).3 This figure, from ToIP, shows how such a stack works.
Trust Over IP StackThe layers build on each other to provide something the lower level didn't. Layer 1 is the foundational functionality, like DID methods. Layer 2 builds on that to support creating digital relationships with anyone. Layer 3 uses those relationships to effect credential exchange. Layer 4 is the ecosystems that say things about the issuers for different use cases. The dual stack emphasizes the need for governance at every layer.
The acceptance network specifies the accepted protocols and technologies. The acceptance network also supports ecosystems, providing governance models and technology. The acceptance network is involved at each layer. Here are some examples of things an acceptance network might do at each layer:
Layer 1—limit the allowed DID methods and certify them.
Layer 2—require that wallets and agents using the network support specific versions of the DIDComm protocol. Provide a certification framework for wallet and agent vendors for security and interoperability.
Layer 3—require specific versions of the exchange protocols. Participate in protocol development. Provide a certification framework for specific implementations to aid with security and interoperability.
Layer 4—support the formation, certification, and discovery of credential ecosystem providers. Govern what is required to be a certified ecosystem provider and provide models for acceptable ecosystem governance.
As part of it's overall governance of the ecosystem, the acceptance network also provides model legal agreements for and between the various participants, trust mark rights (think of the Visa logo), and drives a uniform user experience.
The following diagram shows the credential exchange from the preceding figure with an acceptance network providing support to the verifier so that it can have confidence in the data the issuer has supplied through the holder.
Acceptance Network in OperationCredential issuers who know their credential might be widely used would join one or more acceptance networks. They agree to follow the rules and regulations in the governance framework of the acceptance network. The acceptance network issues a credential to them that they can use to prove they are a member.4 The acceptance network maintains a registry—likely a registry of registries—that verifiers can use to discover information about the issuer of a credential that has been presented to them.
Using an Acceptance NetworkReturning to our previous scenario, Alice holds a credential issued by her university. She presents it to a prospective employer who wants to know that the credential is from an accredited university. Alice's university has been accredited by an accreditation organization5. They have followed their process for accrediting Alice's university and issued it a credential. They have also added the university to their registry. The university and the accrediting organization are members of an acceptance network. The employer's systems know to automatically query the acceptance network when it received a credential proof from a issuer it does not know. Doing so provides the assurance that the issuer is legitimate. It could also provide information about the accreditation status of the university. This information reduces the risk that the employer would otherwise bear.
In this scenario, the employer is trusting the processes and structure of the acceptance network. The employer must decide which acceptance networks to use. This is much more scalable than having to make these determinations for every credential issuer. The acceptance network has allowed the verification process to scale and made the overall use of verifiable credentials easier and less risky.
A Note on ImplementationThis discussion of acceptance networks has undoubtedly brought images to your mind about how it is structured or how to build one. The comparison to financial services acceptance networks points to a network run by an organization. And the term registrybrings to mind a database of some kind. Why these are certainly possibilities, I think it's also possible to imagine more decentralized solutions. For example, the registry could be a distributed ledger or blockchain. The governance is likely most easily done by an organization, but there are other options like a decentralized autonomous organization (DAO). The scenario I described above illustrates a federated system where certifying authorities for specific ecosystems determine their own methods, processes, and requirements, but link their registry to that of the acceptance network.
ConclusionAs I mentioned above, we've been solving the problem of how to know which institutions to trust for centuries. We have ways of knowing whether a university is accredited, whether a bank is real, whether a company is actually registered and what its reputation is. What is missing is an easy way to make use of this information digitally so that processes for reducing risk can be automated. Acceptance networks rationalize the process and provide the needed tooling to automate these checks. They reduce the many-to-many problem that exists when each verifier has to determine whether to trust each issuer with a more scalable many-to-several system. Acceptance networks allow credential presentation to scale by providing the needed infrastructure for giving verifiers confidence in the facts that holders present to them.
NotesYou can see in the linked post how we used trust to describe what we were building, even as we were reducing risk and inspiring confidence.
Note that this investigation could make use of technology. Knowing the universities name, they could look up a well known location on the universities web site to find the identifier. They could use PKI (digital certificates) to be sure they're talking to the right place. They could look up the university in an online registry of accredited universities.
Trust over IP isn't the only one working on this. Marie Wallace of Accenture and Stephen Wilson of Lockstep Partners have been writing about this idea.
Note that there could be different levels or types of members who perform different roles in the ecosystem and make different agreements.
An example is the Northwest Commission on Colleges and Universities.
Photo Credit: Data flowing over networks from DALL-e
I am sharing the following exchange by text upon the request of the other party involved. This is someone I became acquainted with on education issues and over the past year around how their state fits into the topics I research. I did a livestream following this exchange, relating aspects of my experience to recent findings from Michael Levin’s lab around group information fields and communication blocking.
If you’re not sure what human weather is and how linguistic concepts manipulate it, check out this short link:
If you’d like to check out the clips from the Levin lab on embryology and information fields I have a playlist of clips here. Each one is super important.
Now in case you haven’t been following me for awhile, and you imagine I am excited about the prospect of using electrically engineered trachea cells to build swishy buildings in outer space, I’m not. In fact, I don’t support any of the work coming out of Levin’s lab or other biotechnology labs. My position at this time is that I seek to understand the concepts that are shaping the world around us, and the future, and demand that we have open, candid public conversations about the ethics of all of these things and how we should or should not proceed. Fair enough?
I don’t think I have to spend paragraphs or many minutes dramatizing my anger / fear / frustration over where we have landed as a culture. As far as I’m concerned, for me at least, that is a waste of valuable time. That said, if you feel directing your energy to that end is helpful, but all means don’t let me stop you. You do you, and I will do me, and if you are not into me or the insights I offer by all means move along to some other content creator. There’s something for everyone out there.
Below is a stream I did this week reflecting on our fraught exchange. At the time, I chose not to share the person’s name or the contents of our private exchange, because that wouldn’t have been proper. However, this person seems to believe that I misrepresented the conversation and requested I share the text exchange. So, in the spirit of openness I am honoring that request. Cheers to human weather, but I could use a little less turbulence.
These were two comments left on my livestream. I want to point out that I do not expect this person will share my perspective.
That said, I was also within my rights to remove myself from that emotional, energetic whirlwind. The commentary was very personally directed at me, and it was unkind.
My inclination, having researched the mechanics of the system, is that the words above are an example of how our inner worlds can be shaped by collective thought forms, Levin’s information fields. This is not to discount in any way the trauma we have all been through, the profound losses in all their myriad permutations. It is my opinion, that for me, staying in a negative energy state only serves the system’s goals. Therefore I am choosing for myself to continue with my research in a calm, level-headed way. If there are people out there who want to imagine that by stepping back from the outrage machine I am somehow agreeing to the plan, there’s nothing I can do about that. I am not about to transform myself to fit someone else’s idea of who they think I should be or what I should be doing. I will work hard not to try and force others to be who I might imagine they should be. Fair is fair.
We each have our own ways of being in the world. May we work towards fulfillment in ways that do not drag other people down. I have done that in the past myself. I recognize it, and I am trying to do better. We are all human. We are all learning. We all stumble. We all have grace.
Human weather can get ugly. Let us enjoy the clear, calm days when we have them.
In the exchange below, my comments are on the blue background. The other person’s comments are on the light gray background.
The Substack shared above can be read here.
I will pause here and note I have NEVER said “some new medical paradigm will be better than the old.” NEVER. This is attributing opinions to me that are not mine, but something imagined in the head of the person texting. This is human weather.
It was a check-in when I was discussing piezoelectricity and radium and the Curies’ ties to the Society for Psychical Research.
Ok, so the above statement to me implied that the work I am doing is a luxury – “quite a few people don’t have the luxury to worry about metaphysical research.” In my mind I do this work knowing that people in precarity are likely unable to do it and that since I am able, I have a responsibility to do it and put the conversation out into the public sphere for discussion.
Aaron Parecki and I have published a draft of the “OAuth 2.0 Protected Resource Metadata” specification that addresses all the issues that we’re aware of. In particular, the updates address the comments received during the discussions at IETF 118. As described in the History entry for -02, the changes were:
Switched from concatenating.well-known
to the end of the resource identifier to inserting it between the host and path components of it.
Have WWW-Authenticate
return resource_metadata
rather than resource
.
The specification is available at:
https://www.ietf.org/archive/id/draft-ietf-oauth-resource-metadata-02.htmlHere’s the latest installment in the series on working with LLMS: Learn by Doing: How LLMs Should Reshape Education.
If you’re teaching SQL, this article points to a pedagogical challenge/opportunity: How would I create a lesson that guides a student to an understanding of CROSS JOIN without ever mentioning or explicitly teaching anything about it?
If you’re teaching anything else, the same question could (I’ll argue should) apply. How to scaffold learning by doing?
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
12 Let’s talk: conversational software development
13 Using LLMs to Improve SQL Queries
New year, new job.
I’m pleased to announce that I started a new job on January 2 at Tembo, a fully-managed PostgreSQL developer platform. Tembo blogged the news, too.
I first heard from Tembo CTO Samay Sharma last summer, when he inquired about the status of PGXN, the PostgreSQL Extension Network, which I built in 2010–11. Tembo bundles extensions into Postgres stacks, which let developers quickly spin up Postgres clusters with tools and features optimized for specific use cases and workloads. The company therefore needs to provide a wide variety of easy-to-install and well-documented extensions to power those use cases. Could PGXN play a role?
I’ve tended to PGXN’s maintenance for the last fourteen years, and thanks in no small part to hosting provided by depesz. As of today’s stats it distributes 376 extensions on behalf of 419 developers. PGXN has been a moderate success, but Samay asked how we could collaborate to build on its precedent to improve the extensions ecosystem overall.
It quickly became apparent that we share a vision for what that ecosystem could become, including:
Establishing the canonical Postgres community index of extensions, something PGXN has yet to achieve Improving metadata standards to enable new patterns, such as automated binary packaging Working with the Postgres community to establish documentation standards that encourage developers to provide comprehensive extension docs Designing and building developer tools that empower more developers to build, test, distribute, and maintain extensionsOver the the past decade I’ve have many ideas and discussion on these topics, but seldom had the bandwidth to work on them. In the last couple years I’ve enabled TLS and improved the site display, increased password security, and added a notification queue with hooks that post to both Twitter (RIP @pgxn) and Mastodon (@pgxn@botsin.space). Otherwise, aside from keeping the site going, periodically improving new accounts, and eyeing the latest releases, I’ve had little bandwidth for PGXN or the broader extension ecosystem.
Now, thanks to the vision and strategy of Samay and Tembo CEO Ry Walker, I will focus on these projects full time. The Tembo team have already helped me enumerate the extension ecosystem jobs to be done and the tools required to do them. This week I’ll submit it to collaborators from across the Postgres community1 to fill in the missing parts, make adjustments and improvements, and work up a project plan.
The work also entails determining the degree to which PGXN and other extension registries (e.g., dbdev, trunk, pgxman, pgpm (WIP), etc.) will play a role or provide inspiration, what bits should be adopted, rewritten, or discarded.2 Our goal is to build the foundations for a community-owned extensions ecosystem that people care about and will happily adopt and contribute to.
I’m thrilled to return to this problem space, re-up my participation in the PostgreSQL community, and work with great people to build out the extensions ecosystem for future.
Want to help out or just follow along? Join the #extensions channel on the Postgres Slack. See you there.
Tembo was not the only company whose representatives have reached out in the past year to talk about PGXN and improving extensions. I’ve also had conversations with Supabase, Omnigres, Hydra, and others. ↩︎
Never be afraid to kill your darlings. ↩︎
More about… Personal Work Tembo Postgres ExtensionsWe held the first of three planned tenth anniversary celebrations for the completion of OpenID Connect at the OpenID Summit Tokyo 2024. The four panelists were Nov Matake, Ryo Ito, Nat Sakimura, and myself. We shared our perspectives on what led to OpenID Connect, why it succeeded, and what lessons we learned along the way.
The most common refrain throughout our descriptions was the design philosophy to “Keep simple things simple”. I believe that three of the four of us cited it.
I recounted that we even had a thought experiment used to make the “Keep simple things simple” principle actionable in real time: the “Nov Matake Test”. As we considered new features, we’d ask ourselves “Would Nov want to add it to his implementation?” And “Is it simple enough that he could build it in a few hours?”
The other common thread was the criticality of interop testing and certification. We held five rounds of interop testing before finishing the specifications, with the specs being refined after each round based on the feedback received. The early developer feedback was priceless – much of it from Japan!
Our OpenID Connect 10th anniversary presentations were:
Remarks by Mike Jones Remarks by Nov Matake Remarks by Ryo Ito Remarks by Nat SakimuraThanks to the OpenID Foundation Japan for the thought-provoking and enjoyable OpenID Summit Tokyo 2024!
Thanks to those of you who elected me to a two-year term on the OpenID Foundation board of directors. This is an incredibly exciting time for the OpenID Foundation and for digital identity, and I’m thrilled to be able to contribute via the OpenID board. Thanks for placing your trust in me!
I’d like to also take this opportunity to congratulate my fellow board members who were also elected: George Fletcher, Atul Tulshibagwale, and Mark Verstege. See the OpenID Foundation’s announcement of the 2024 election results.
My candidate statement was:
I am on a mission to build the Internet’s missing identity layer. OpenID specifications and initiatives are key to realizing that vision.
Widespread deployment of OpenID specifications has the potential to make people’s online interactions more seamless, secure, and valuable. I have been actively working since 2007 to make that an everyday reality.
2024 has huge potential for advances in digital identity. People are starting to have identity wallets holding digital credentials that they control. National and international federations are being established. Open Banking and Open Finance deployments are ongoing. Adoption of OpenID Connect (which we created a decade ago!) continues going strong. We’re on track to have OpenID Connect be published as ISO standards. OpenID specifications and programs are essential to all these outcomes.
While many of you know me and my work, here’s a few highlights of my contributions to the digital identity space and the OpenID community:
– I was primary editor of OpenID Connect, primary editor of the OAuth 2.0 bearer token specification [RFC 6750], and primary editor of the JSON Web Token (JWT) specification [RFC 7519] and the JSON Object Signing and Encryption (JOSE) specifications [RFCs 7515-7518], which are used by OpenID Connect. I was an editor of the Security Event Token specification [RFC 8417], which is used by Shared Signals and OpenID Connect. I’m an editor of the SIOPv2 specification and a contributor to the other OpenID for Verifiable Credentials specifications. I’m an editor of the OpenID Federation specification. The OAuth DPoP specification [RFC 9449] was my latest RFC. I’m an author of 32 RFCs and 17 final OpenID specifications, with more of each in the pipeline.
– I spearheaded creation of the successful OpenID Connect certification program and continue actively contributing to its success. Over 2,800 certifications have been performed and the pace keeps increasing! Certification furthers the Foundation’s goals of promoting interoperation and increasing the quality of implementations. It’s also become an important revenue stream for the Foundation.
– My contributions to the Foundation have included serving on the board since 2008, serving as board secretary during most of my tenure. I’ve helped organize numerous OpenID summits and working group meetings and regularly present there. I chaired the election committee that developed the Foundation’s election procedures and software. I co-chaired the local chapters committee that developed the policies governing the relationships with local OpenID chapters around the world. I serve on the liaison committee, facilitating our cooperation with other organizations. And way back in 2007, I worked with the community to create the legal framework for the OpenID Foundation, enabling both individuals and corporations to be full participants in developing OpenID specifications and ensuring that they can be freely used by all.
I’d like to continue serving on the OpenID board, because while the OpenID community is having notable successes, our work is far from done. Taking it to the next level will involve both additional specifications work and strategic initiatives by the Foundation. We need to continue building a broad base of supporters and deployers of OpenID specifications around the world. We need to continue fostering close working relationships with partner organizations. And we need to continue safeguarding OpenID’s intellectual property and trademarks, so they remain freely available for all to use.
I have a demonstrated track record of energetically serving the OpenID community and producing results that people actually use. I plan to continue taking an active role in making open identity solutions even more successful and ubiquitous. That’s why I’m running for a community board seat in 2024.
Mike Jones
michael_b_jones@hotmail.com
Blog: https://self-issued.info/
Professional Website: https://self-issued.consulting/
Lenin supposedly said:
There are decades where nothing happens, and there are weeks where decades happen.
It’s the same in technology.
I came to Silicon Valley in the mid-90’s, just in time to see the dot-com boom unfold. Lots happened very quickly in that time. There were a few more such periods of rapid change since, like when centralized social media got going, and when phones turned into real computers. But for many years now, not much has happened: we got used to the idea that there’s a very small number of ever-larger tech giants, which largely release incremental products and that’s just that. Nothing much happens.
But over the last year or so, suddenly things are happening again. I think not only are the spaces for innovation and entrepreneurship now more open than they have been for at least a decade or more; it’s possible they have never been as open as they are now.
Consider:
Everybody’s favorite subject: machine learning and AI. I don’t believe in much of what most people seem to believe about AI these days. I’m not part of the hype train. However, I do believe that machine learning is a fundamental innovation that allows us to program computers in a radically different way than we have in the past 50 and more years: instead of telling the computer what to do, we let it observe how it’s done and have it copy what it saw. Most of what today’s AI companies use machine learning for, in my view, is likely not going to stand the test of time. However, I do believe that this fundamentally different way of programming a computer is going to find absolutely astounding and beneficial applications at some point. It could be today: the space for invention, innovation and entrepreneurship is wide open.
The end of ever-larger economies of scale and network effects in tech. The dominant tech companies are very close to having pretty much all humans on the planet as customers. The number of their users is not going to double again. So the cost structure of their businesses is not going to get reduced any more simply by selling the same product to more customers, nor is the benefit of their product going to grow through growing network effects as much as in the past. It’s like they are running into a physical limit to the size of many things they can do. This opens space for innovation and successful competition.
Most interesting, it allows the creation of bespoke products again; products that are optimized for particular markets, customer groups and use cases. Ever noticed that Facebook is the same product for everybody, whether you are rich or poor, whether you have lots of time, or none, whether you are CEO or a kid, whether you like in one place or another, whether you are interested in sports or not and so forth? It’s the same for products of the other big platform vendors. That is a side effect of the focus on economies of scale. All of a sudden, increased utility for the user will need to come from serving their specific needs, not insisting that all cars need to be black. For targeted products, large platforms have no competitive advantages over small organizations; in fact, they may be at a real disadvantage. Entrepreneurs, what are you waiting for?
The regulators suddenly have found their spine and aren’t kidding around, starting with the EU.
The Apple App Store got in the way of your business? They are about to force the App Store open and allow side loading and alternate app stores (although Apple is trying hard to impede this as much as possible; a fight is brewing; my money is on the regulators).
The big platforms hold all your data hostage? Well, in many jurisdictions around the world you now have the right to get all copy of all your data. Even better, the “continuous, real-time access” provision of the EU’s Digital Markets Act is about to come into force.
The platforms don’t let you interoperate or connect? Well, in the EU, a legal requirement for interoperability of messaging apps is already on the books, and more are probably coming. Meta’s embrace of ActivityPub as part of Threads is a sign of it.
Imagine what you can do, as an entrepreneur, if you can distribute outside of app stores, use the same data on the customer that the platforms have, and you can interoperate with them? The mind boggles … many product categories that previous were impossible to compete with suddenly are in play again.
Social networking is becoming an open network through the embrace of ActivityPub by Meta’s Threads. While nobody outside of Meta completely understands why they are doing this, they undoubtedly are progressing towards interoperability with the Fediverse. Whatever the reasons, chances are that they also apply to other social media products, by Meta and others. All of a sudden competing with compelling social media application is possible again because you have a fully built-out network with its network effects from day one.
Consumers know tech has a problem. They are more willing to listen to alternatives to what they know than they have in a long time.
And finally, 3D / Spatial Computing a la Apple. (I’m not listing Meta here because clearly, they don’t have a compelling vision for it. Tens of billions spent and I still don’t know what they are trying to do.)
Apple is creating an an entirely new interaction model for how humans can interact with technology. It used to be punch cards and line printers. Then we got interactive green-screen terminals. And then graphics displays, and mice. That was in the 1980’s. Over the next 40 years, basically nothing happened (except adding voice for some very narrow applications). By using the space around us as a canvas, Apple is making it possible to interact with computing in a radically different way. Admittedly, nobody knows so far how to really take advantage of the new medium, but once somebody does, I am certain amazing things will happen.
Again, an opportunity ripe for the taking. If it works, it will have the same effects on established vendors as the arrival of the web had on established vendors: some managed to migrate, or the arrival graphical user interfaces on the vendors of software for character terminals; most failed to make the switch. So this is another ideal entrepreneurial territory.
But here’s the kicker: what if you combined all of the above? What can you build if your primary interaction model is 3D overlayed over the real world, with bespoke experiences for your specific needs, assisted by (some) intelligence that goes beyond what computers typically do today, accomplished by some form of machine learning, all fed by personal data collected by the platforms, and distributed outside of the straightjacket and business strategies of app stores?
We have not seen as much opportunity as this in a long time; maybe ever.
Something to keep in mind as big tech connects to the fediverse is Winer’s Law of the Internet which ends with
The large companies always try to make the technology complicated to reduce competition to other organizations with large research and development budgets.
This is 20 years old but it has stood the test of time.
I was recently on the Identity at the Center podcast speaking with hosts Jim McDonald and Jeff Steadman. We discussed my journey into the field of identity, Internet Identity Workshop, and my latest book "Learning Digital Identity." We also discussed the book writing process, key takeaways from the book, and the future of identity innovation. It was a fun conversation. I hope you enjoy it too.
This article shows how to migrate a Blazor server application to a Blazor Web application. The migration used the ASP.NET Core migration documentation, but this was not complete and a few extra steps were required. The starting point was a Blazor Server application secured using OpenID Connect for authentication. The target system is a Blazor Web application using the “InteractiveServer” rendermode.
History2024-02-12 Updated to support CSP nonces
Code: https://github.com/damienbod/BlazorServerOidc
MigrationThe following Blazor Server application was used as a starting point:
https://github.com/damienbod/BlazorServerOidc/tree/main/BlazorServerOidc
This is a simple application using .NET 8 and OpenID Connect to implement the authentication flow. Security headers are applied and the user can login or logout using OpenIddict as the identity provider.
As in the migration guide, steps 1-3, the Routes.razor was created and the imports were extended. Migrating the contents of the Pages/_Host.cshtml to the App.razor was more complicated. I have a Layout in the original application and this needed migration into the App file as well.
This completed Blazor Web App.razor file looked like this:
@inject IHostEnvironment Env <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <base href="/" /> <link rel="stylesheet" href="css/bootstrap/bootstrap.min.css" /> <link href="css/site.css" rel="stylesheet" /> <link href="BlazorWebFromBlazorServerOidc.styles.css" rel="stylesheet" /> <HeadOutlet @rendermode="InteractiveServer" /> </head> <body> <Routes @rendermode="InteractiveServer" /> <script nonce="@BlazorNonceService.Nonce" src="_framework/blazor.web.js"></script> </body> </html>The App.razor uses the routes component. Inside the routes component, the CascadingAuthenticationState is used and a new component for the layout called the MainLayout.
@inject NavigationManager NavigationManager <CascadingAuthenticationState> <Router AppAssembly="@typeof(Program).Assembly"> <Found Context="routeData"> <AuthorizeRouteView RouteData="@routeData" DefaultLayout="@typeof(Layout.MainLayout)"> <NotAuthorized> @{ var returnUrl = NavigationManager.ToBaseRelativePath(NavigationManager.Uri); NavigationManager.NavigateTo($"api/account/login?redirectUri={returnUrl}", forceLoad: true); } </NotAuthorized> <Authorizing> Wait... </Authorizing> </AuthorizeRouteView> </Found> <NotFound> <LayoutView Layout="@typeof(Layout.MainLayout)"> <p>Sorry, there's nothing at this address.</p> </LayoutView> </NotFound> </Router> </CascadingAuthenticationState>The MainLayout component uses two more new razor components, one for the nav menu and one for the login, logout component.
@inherits LayoutComponentBase <div class="page"> <div class="sidebar"> <NavMenu /> </div> <main> <div class="top-row px-4"> <LogInOrOut /> </div> <article class="content px-4"> @Body </article> </main> </div> <div id="blazor-error-ui"> An unhandled error has occurred. <a href="" class="reload">Reload</a> <a class="dismiss">🗙</a> </div>The login, logout component uses the original account controller and improved the logout.
@inject NavigationManager NavigationManager <AuthorizeView> <Authorized> <div class="nav-item"> <span>@context.User.Identity?.Name</span> </div> <div class="nav-item"> <form action="api/account/logout" method="post"> <AntiforgeryToken /> <button type="submit" class="nav-link btn btn-link text-dark"> Logout </button> </form> </div> </Authorized> <NotAuthorized> <div class="nav-item"> <a href="api/account/login?redirectUri=/">Log in</a> </div> </NotAuthorized> </AuthorizeView>The program file was updated like in the migration docs. Blazor Web does not support reading the HTTP headers from inside a Blazor component and so the security headers were weakened which is a very bad idea. CSP nonces are not supported and so a super web security feature is lost if updating to Blazor Web. I believe moving forward, the application should be improved.
using BlazorWebFromBlazorServerOidc.Data; using Microsoft.AspNetCore.Authentication.Cookies; using Microsoft.AspNetCore.Authentication.OpenIdConnect; using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.Mvc.Authorization; using Microsoft.IdentityModel.JsonWebTokens; using Microsoft.IdentityModel.Protocols.OpenIdConnect; using Microsoft.IdentityModel.Tokens; namespace BlazorWebFromBlazorServerOidc; public class Program { public static void Main(string[] args) { var builder = WebApplication.CreateBuilder(args); builder.Services.TryAddEnumerable(ServiceDescriptor.Scoped<CircuitHandler, BlazorNonceService> (sp => sp.GetRequiredService<BlazorNonceService>())); builder.Services.AddScoped<BlazorNonceService>(); builder.Services.AddAuthentication(options => { options.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.DefaultChallengeScheme = OpenIdConnectDefaults.AuthenticationScheme; }) .AddCookie() .AddOpenIdConnect(options => { builder.Configuration.GetSection("OpenIDConnectSettings").Bind(options); options.SignInScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.ResponseType = OpenIdConnectResponseType.Code; options.SaveTokens = true; options.GetClaimsFromUserInfoEndpoint = true; options.TokenValidationParameters = new TokenValidationParameters { NameClaimType = "name" }; }); builder.Services.AddRazorPages().AddMvcOptions(options => { var policy = new AuthorizationPolicyBuilder() .RequireAuthenticatedUser() .Build(); options.Filters.Add(new AuthorizeFilter(policy)); }); builder.Services.AddRazorComponents() .AddInteractiveServerComponents(); builder.Services.AddSingleton<WeatherForecastService>(); builder.Services.AddControllersWithViews(options => options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute())); var app = builder.Build(); JsonWebTokenHandler.DefaultInboundClaimTypeMap.Clear(); if (!app.Environment.IsDevelopment()) { app.UseExceptionHandler("/Error"); app.UseHsts(); } // Using an unsecure CSP as CSP nonce is not supported in Blazor Web ... app.UseSecurityHeaders( SecurityHeadersDefinitions.GetHeaderPolicyCollection(app.Environment.IsDevelopment(), app.Configuration["OpenIDConnectSettings:Authority"])); app.UseMiddleware<NonceMiddleware>(); app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseAuthentication(); app.UseAuthorization(); app.UseAntiforgery(); app.MapRazorPages(); app.MapControllers(); app.MapRazorComponents<App>() .AddInteractiveServerRenderMode().RequireAuthorization(); app.Run(); } }With the weakened security headers the application works and the authentication flow works.
ConclusionBlazor Web in the InteractiveServer mode can use CSP nonces and it is possible to implement a secure web application.
Linkshttps://learn.microsoft.com/en-us/aspnet/core/migration/70-80
Securing a Blazor Server application using OpenID Connect and security headers
https://github.com/dotnet/aspnetcore/issues/53192
https://github.com/dotnet/aspnetcore/issues/51374
https://github.com/javiercn/BlazorWebNonceService
Here’s the latest installment in the series on working with LLMS: 7 Guiding Principles for Working with LLMs.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
12 Let’s talk: conversational software development
Getting immersed in a new subject is a great experience. ISO SC 37 wrapped up a week-long series of meetings yesterday in Okayama Japan. Here are some things I learned along the way:
SC 37 is smaller than the other ISO committees I’m in – about 60 in-person plus about 20 online this week across 6 work groups. It makes for a tight-knit group and provides lots of time to build relationships. It also means that the workload is sane – on other committees I have to ignore all but the 2 or 3 projects I’m directly involved in.
The main work of the committee is extensible data exchange formats, APIs, evaluation of biometric technologies, performance testing and societal aspects.
SC 37 was started in the early 2000s to meet the needs of ICAO to add biometric information to passports – face, finger and iris formats were the first. Since then, work has been done to cover other biometric modalities such as voice, skeletal structure, vein pattern and others.
Participants are primarily from Government border control, immigration, public safety, law enforcement departments. I’m one of a very small number focused on consumer-provided sensors and devices for use in commercial scenarios.
Biometrics is all about statistical analysis – I never really thought much about it – using the lazy term “probabilistic” – but it really is about estimation and weighting of data samples. So I might have to break open a textbook or two to relearn by sum-overs, means, medians, and standard deviations – not looking forward that that part!
“Convoluted Neural Networks” appear to be broadly used to look for patterns and help to draw conclusions. Another area I must learn about quickly.
There is a significant desire to apply biometric binding of people to data records – for example during ID Proofing and Verification. But it was clear that SC 37 is not the best place to do that work. SC 17 for mDoc credentials or SC 27/WG 5 for identity management seem better suited for this work.
I realized by the end of the week that being an “identity management” guy in the “biometrics” world is a very good thing – the latter can be applied to operations of the former and I get to work on both sides.
So I’m looking forward to working with the committee members and digging deep into the material. Hopefully I can bring useful opinions from the consumer world to drive discussions and improve the body of work.
I moved my account from @herestomwiththeweather@mastodon.social to @tom@herestomwiththeweather.com on January 2nd. In the spirit of learning from post-mortems, I am documenting a few mistakes I made.
One of the main motivations for the move was that over a year ago, I had configured webfinger on this site to point to the account I had on mastodon.social. But once someone has found me on mastodon, I would from then on be known by my mastodon identifier rather than the identifier with my personal domain. If I lost access to that particular mastodon account for whatever reason, I would be unreachable by that mastodon identifier. However, as I described in Webfinger Expectations, if my webfinger configuration points me to a server that will allow me to participate on the fediverse with my own personal identifier using my own domain, then in theory, if I lose access to the account on that server, I can swap it out with another similar server and be reachable again with my personal identifier. So, last week I moved to Otisburg.social which is running what I consider a minimum viable activitypub server called Irwin. As it is experimental, I am the only user on the server.
So what did I screw up? I didn’t plan for two things. Both are related to the diversity of software and configurations on the Fediverse.
First, although I was vaguely aware of the optional Authorized Fetch mastodon feature, I didn’t anticipate that it would prevent me from re-following some of my followers. Prior to the migration, I assumed this feature would not be enabled on any of the servers the people I followed were using. I quickly realized that I could not re-follow people on 3 servers which had this feature enabled. So, I lost contact with the people on those servers for a few days until I fixed it by also signing GET requests in addition to POST requests.
Second, I didn’t adequately prepare for the possibility that some of my followers would not automatically move to the new server. Of 96 followers, I had about 15 that did not successfully re-follow. It seems that some of these failed because they were not on a Mastodon server and their server did not adequately handle the Move activity sent by mastodon.social. Unfortunately, although mastodon allowed me to download a csv file of the people I followed, it did not provide a link to download a file of followers so I don’t know everyone I lost during the move.
Otherwise, the move went well and it is a great feature and I’m glad to see an effort underway to standardize it.
One unresolved issue is that when someone visits my profile on a mastodon site, selecting “open original page” will fetch https://otisburg.social/actor/tom@herestomwiththeweather.com and the user would expect to see my status updates or toots or whatever you call them. However, currently that url redirects to this website and activitypub status updates are not available here.
Hierarchy appears to be the only option to pull a large group of individuals together toward a common goal. Many insects did it well before humans, but over the last 10,0000 years, humans have moved from decentralised clans into centralised nation-states consisting of hundreds of millions of people. From the pharaohs to Max Weber’s 20th bureaucratic management structure, we can now exist only in such a system because as a society or organisation grows beyond a few dozen people, the hierarchical pyramid is seen as the only option for the organisation. The justification is that it is nature and natural.
The “ideal organisation” was defined by Max Weber as a clear and strong hierarchy underpinned by the division of tasks based on specialisation. A fundamental assumption was that each unit takes care of one piece of the chain and the division of tasks within the unit is clearly defined, work can be executed much more efficiently. Weber envisioned this as a “superior structure” because it focused on rationality and equal treatment of all - assuming everyone was happy with their 1984 dystopian jobs and oversight. By formalising processes within organisations, but especially in government, favouritism and abuse of power could be prevented - as he assumed that those in charge and specialised workers remained. A really poor underlying assumption was that people act rationally, efficiently, and professionally. He also ignored the concept of shareholder primacy that would emerge. However, so strong is the argument that it remains the leading business philosophy today, and we still teach that hierarchy, administration and bureaucracy driven by efficiency and effective thinking underpinned by a better division of tasks and responsibilities to drive organisational performance measurable objective is still best practice. The justification is that it represents nature and is natural.
Different models emerged from the internet, web, and digital thinking allowing us to ponder if something better is possible. Small iterations, such as matrix management, never really changed the fundamentals and whilst embracing all stakeholders and ESG has added complexity to the decisions and models - hierarchy remains. However, it is possible to sense in the noise signals that “Data” is finally about to disrupt and dislodge a love/ hate affair with hierarchy, power, and control.
Counter to this and according to both scientists, individuals, including leaders, do not make their decisions after a thorough analysis, but first decide, unconsciously and intuitively, and then rationalise their decision. We have become experts in seeking out framed and biased data to justify and provide a rational argument for a decision we have already made.
-----
Endless inches have been written about structure, who and how to lead and what a digital organisation looks like. However, right now, we are likely to be in the death phase for a pure CDO (chief data/ digital officer) role as it becomes clear that the CTO, CIO and CDO roles have very similar mandates and are now actually competing for power, resources and a clear value to the organisation. The ridged ideals of separation and specialism models driving structures, management, incentives, KPI’s and hierarchy cannot work with the emergence of highly complex interdependency in a digital age - are we witnessing that the division of labour falls apart at scale because of complexity and speed?
For a complicated task, the separation of that task into modules, and components to create the scale of efficiency and effectiveness works, and it works really well. For any task where the output directly and immediately, because of close feedback loops, changes the inputs for the next unit of creation and consumption - the hierarchy fails. The ridged division of labour into separate tasks, modules, parts or components does not work - because it fundamentally needs repeatability.
When operating at speed and scale - but there is a high level of repeatability, hierarchy delivers, and we know its problems. However, data is enabling us to operate at speed, scale and uniqueness (non-repeatability) - this is different.
However, data is enabling us to operate at speed, scale and uniqueness (non-repeatability) - this is different.
---
We have recognised for a while that leadership/ chief roles are actually a representation of the ontology of an organisation (structure), the question has never been if you need a CEO, CFO, CMO, CTO as these were clearly delineated roles and specialisms. We understand the roles, mandates, powers and delegated authority the experts and leaders bring and have.
In a digital business, we have created four roles CTO, CIO, CDO and CISO. But the reality is that the CMO and CDO need the CFO’s data. The CFO wants the COO and CMO’s data. The CIO has lots of data but no direct path to showing value. The CISO needs other data from outside the organisation and the CTO needs data the CMO has not yet imagined. Organisations opted to create new roles such as Chief Data or Digital Officer to address the emerging complexity and to avoid too much power being vested in the CTO.
Breaking the axis of old - the CEO/ CFO
The CEO/CFO axis has this framing; we’ll do more things that are cheaper in the short term and less of those that are more expensive in the long term as our incentive and remuneration guide us. In a company with unified incentives, there is a uniciation of framing which means it is difficult for new ideas to emerge. This incentive friction or incentive dependency means we always face the same decisions in the same way.
Many leaders are realising that data is forging changes, and this change is not about tech, data or analysis, but it is about whether we, the leadership, exec or board, are making the right decisions. Decisions of the past were framed by the ideal hierarchy underpinned by efficiency and effectiveness at scale driven by the demands for specialisation. However, to know we are making the right decision going forward, we need to understand we have the right choices today, which means we need all “data” and our data can no longer be about isolation, separation or specialisation.
I expect that we are about to see a new axis of power arrive with the emergence of a new chief role as we realise, we need a new power dynamic structure. A two-way axis (CEO/CFO) becomes a three-way stool. The emergence of the CEO/ CFO/ CxO. The CEO/ CFO will maintain the remits of controlling and understanding the speed and scale at which the hierarchy operates, with the new role focussing on uniqueness - how data breaks the old hierarchy. It does not matter what you call the X, the point is what is their mandate. They have to own:
the data philosophy
all data emerging from the company and its ecosystem
data governance and security
direct the current and future platform's abilities, functionality and performance
The point here is that this role is not about the analysis of the data but about ensuring the quality, attestation, providence, provenance, function, form, taxonomy, ontology and pedagogy of the data needed for decision and that we will continue to access to and enjoy the data we need to make better decisions.
The critical point is that once the CEO/CFO could know if they were making the right decisions as the framing they needed was about efficiency and effectiveness within a specialism model, however going forward, this axis is not only insufficient, it is damaging. This new third member is needed to provide modern-day stabilisation and governance to ensure that as an expanded team, “we know we are making the right decisions”, as we must regularly ask efficacy questions that were not needed under the old repeatability model, as the mission was sufficient as the guide.
The first victim will be the CDO, as it exists in a specialisation-driven hierarchy that has no choice but to change - not its structure and how it thinks.
This article shows how to secure a Blazor Server application. The application implements an OpenID Connect confidential client with PKCE using .NET 8 and configures the security headers as best possible for the Blazor Server application. OpenIddict is used to implement the identity provider and the OpenID Connect server.
Code: https://github.com/damienbod/BlazorServerOidc
OpenID Connect flowIn the first step, the authentication can be solved using OpenID Connect. With this, the process of user authentication is removed from the client application and delegated to an identity provider. In this demo, OpenIddict is used. The OpenID Connect code flow with PKCE is used and the application uses a client secret to authenticate. This can be further improved by using a certificate and client assertions when using the code from the OpenID Connect flow to request the tokens. The flow can also be improved to use OAuth 2.0 Pushed Authorization Requests PAR, if the identity provider supports this.
In ASP.NET Core or Blazor, this can be implemented using the Microsoft ASP.NET Core OpenIdConnect package.
Microsoft.AspNetCore.Authentication.OpenIdConnectThe authentication flow requires some service setup as well as middleware changes in the pipelines. The solution uses the OpenID Connect scheme to take care of the user authentication and saves this in a cookie. The name claim is set to use the “name” claim. This is different with every identity provider and also depending how the ASP.NET Core application maps this. All razor page requests must be authenticated unless otherwise specified.
builder.Services.AddAuthentication(options => { options.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.DefaultChallengeScheme = OpenIdConnectDefaults.AuthenticationScheme; }) .AddCookie() .AddOpenIdConnect(options => { builder.Configuration.GetSection("OpenIDConnectSettings").Bind(options); options.SignInScheme = CookieAuthenticationDefaults.AuthenticationScheme; options.ResponseType = OpenIdConnectResponseType.Code; options.SaveTokens = true; options.GetClaimsFromUserInfoEndpoint = true; options.TokenValidationParameters = new TokenValidationParameters { NameClaimType = "name" }; }); builder.Services.AddRazorPages().AddMvcOptions(options => { var policy = new AuthorizationPolicyBuilder() .RequireAuthenticatedUser() .Build(); options.Filters.Add(new AuthorizeFilter(policy)); }); builder.Services.AddControllersWithViews(options => options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute()));The application pipeline is setup after the services are built. ASP.NET Core adds some automatic claim mapping and renames some claims per default. This can be reset to use the exact claims sent back from the identity provider.
var app = builder.Build(); JsonWebTokenHandler.DefaultInboundClaimTypeMap.Clear(); if (!app.Environment.IsDevelopment()) { app.UseExceptionHandler("/Error"); app.UseHsts(); } app.UseSecurityHeaders( SecurityHeadersDefinitions.GetHeaderPolicyCollection( app.Environment.IsDevelopment(), app.Configuration["OpenIDConnectSettings:Authority"])); app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseAuthentication(); app.UseAuthorization(); app.MapRazorPages(); app.MapControllers(); app.MapBlazorHub().RequireAuthorization(); app.MapFallbackToPage("/_Host"); app.Run(); CascadingAuthenticationStateThe CascadingAuthenticationState is used to force and share the authentication requirements in the UI and the Blazor Server components. If the application and the user are not authenticated and authorized, the user is redirected to the identity provider.
@inject NavigationManager NavigationManager <CascadingAuthenticationState> <Router AppAssembly="@typeof(App).Assembly"> <Found Context="routeData"> <AuthorizeRouteView RouteData="@routeData" DefaultLayout="@typeof(MainLayout)"> <NotAuthorized> @{ var returnUrl = NavigationManager.ToBaseRelativePath(NavigationManager.Uri); NavigationManager.NavigateTo($"api/account/login?redirectUri={returnUrl}", forceLoad: true); } </NotAuthorized> <Authorizing> Wait... </Authorizing> </AuthorizeRouteView> </Found> <NotFound> <LayoutView Layout="@typeof(MainLayout)"> <p>Sorry, there's nothing at this address.</p> </LayoutView> </NotFound> </Router> </CascadingAuthenticationState> Authentication flow UI PagesThe menu needs to display the login or the logout buttons depends on the state of the application. Blazor provides an AuthorizeView component for this. This can be used in the UI to hide or show elements depending on the authenticate state.
<AuthorizeView> <Authorized> <span>@context.User.Identity?.Name</span> <form action="api/account/logout" method="post"> <button type="submit" class="nav-link btn btn-link text-dark"> Logout </button> </form> </Authorized> <NotAuthorized> <a href="api/account/login?redirectUri=/">Log in</a> </NotAuthorized> </AuthorizeView>I added a log in, log out in an account controller and signed out Razor page to handle the authentication requests from the UI. The application automatically authenticates so the login is only used in the signed out page. The login removes the authenticated user from both schemes; the cookie and the OpenID Connect scheme. To remove the authenticated user from the identity provider, the application needs to redirect. This cannot be implemented in an ajax request.
[IgnoreAntiforgeryToken] // need to apply this to the form post request [Authorize] [HttpPost("Logout")] public IActionResult Logout() { return SignOut( new AuthenticationProperties { RedirectUri = "/SignedOut" }, CookieAuthenticationDefaults.AuthenticationScheme, OpenIdConnectDefaults.AuthenticationScheme); }The SignedOut is the only unauthorized page, component in the application. The AllowAnonymous is used for this.
using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Mvc.RazorPages; namespace BlazorServerOidc.Pages; [AllowAnonymous] public class SignedOutModel : PageModel { public void OnGet() { } } Security headersThe user is authenticated. The application need to protect the session as well. I use NetEscapades.AspNetCore.SecurityHeaders for this.
NetEscapades.AspNetCore.SecurityHeaders NetEscapades.AspNetCore.SecurityHeaders.TagHelpersBlazor Server is a severed rendered application with UI components. The application can implement nonce based CSP. All the typical security headers are added as best possible for this technology.
namespace BlazorServerOidc; public static class SecurityHeadersDefinitions { public static HeaderPolicyCollection GetHeaderPolicyCollection(bool isDev, string? idpHost) { ArgumentNullException.ThrowIfNull(idpHost); var policy = new HeaderPolicyCollection() .AddFrameOptionsDeny() .AddContentTypeOptionsNoSniff() .AddReferrerPolicyStrictOriginWhenCrossOrigin() .AddCrossOriginOpenerPolicy(builder => builder.SameOrigin()) .AddCrossOriginResourcePolicy(builder => builder.SameOrigin()) .AddCrossOriginEmbedderPolicy(builder => builder.RequireCorp()) // remove for dev if using hot reload .AddContentSecurityPolicy(builder => { builder.AddObjectSrc().None(); builder.AddBlockAllMixedContent(); builder.AddImgSrc().Self().From("data:"); builder.AddFormAction().Self().From(idpHost); builder.AddFontSrc().Self(); builder.AddBaseUri().Self(); builder.AddFrameAncestors().None(); builder.AddStyleSrc() .UnsafeInline() .Self(); builder.AddScriptSrc() .WithNonce() .UnsafeInline(); // only a fallback for older browsers when the nonce is used // disable script and style CSP protection if using Blazor hot reload // if using hot reload, DO NOT deploy with an insecure CSP }) .RemoveServerHeader() .AddPermissionsPolicy(builder => { builder.AddAccelerometer().None(); builder.AddAutoplay().None(); builder.AddCamera().None(); builder.AddEncryptedMedia().None(); builder.AddFullscreen().All(); builder.AddGeolocation().None(); builder.AddGyroscope().None(); builder.AddMagnetometer().None(); builder.AddMicrophone().None(); builder.AddMidi().None(); builder.AddPayment().None(); builder.AddPictureInPicture().None(); builder.AddSyncXHR().None(); builder.AddUsb().None(); }); if (!isDev) { // maxage = one year in seconds policy.AddStrictTransportSecurityMaxAgeIncludeSubDomains(); } policy.ApplyDocumentHeadersToAllResponses(); return policy; } }The CSP nonces are added to all scripts in the UI.
@using Microsoft.AspNetCore.Components.Web @namespace BlazorServerOidc.Pages @addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers @addTagHelper *, NetEscapades.AspNetCore.SecurityHeaders.TagHelpers <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <base href="~/" /> <link rel="stylesheet" href="css/bootstrap/bootstrap.min.css" /> <link href="css/site.css" rel="stylesheet" /> <link href="BlazorServerOidc.styles.css" rel="stylesheet" /> <component type="typeof(HeadOutlet)" render-mode="ServerPrerendered" /> </head> <body> @RenderBody() <div id="blazor-error-ui"> <environment include="Staging,Production"> An error has occurred. This application may no longer respond until reloaded. </environment> <environment include="Development"> An unhandled exception has occurred. See browser dev tools for details. </environment> <a href="" class="reload">Reload</a> <a class="dismiss">🗙</a> </div> <script asp-add-nonce src="_framework/blazor.server.js"></script> </body> </html> Next stepsIn a follow up blog, I would like to implement the same type of security for the new .NET 8 Blazor web applications.
Linkshttps://learn.microsoft.com/en-us/aspnet/core/blazor/security/server/
https://stackoverflow.com/questions/64853618/oidc-authentication-in-server-side-blazor
How OpenID Connect Works
https://github.com/openiddict/openiddict-core
https://datatracker.ietf.org/doc/html/rfc9126
https://learn.microsoft.com/en-us/aspnet/core/security/authentication/claims
Another year, another ISO committee!
I have joined the Standards Council of Canada mirror committee for SC 37 (Biometrics) and will be attending the international meetings. The hard work has begun learning the committee culture, personalities and flow of projects.
Getting started in an ISO committee involves applying for accreditation as a national standards body expert in the domain; connecting up to the wikis and email lists; attaching to the document management system; and locating the ‘active coal face’1 – the precise status of each interesting project. Not trivial. It’s the same pattern for every standards body – getting caught up takes effort.
There are six work groups within SC 37. I’ll be spending most of my time in WG 5 (Biometric Testing and Reporting) and WG 4 (Technical Implementation of Biometric Systems). WG 1 (Harmonized Biometric Vocabulary), WG 2 (Biometric Technical Interfaces), WG 3 (Biometric Data Interchange Formats) and WG 6 (Cross-Jurisdictional and Societal Aspects of Biometrics) will receive attention as and when needed.
Here are some of the projects that look immediately interesting to me. My opinion will certainly change as I get into the work
ISO/IEC 30107-3 — Biometric presentation attack detection — Part 3: Testing and reporting ISO/IEC 30107-4:2020 — Information technology — Biometric presentation attack detection — Part 4: Profile for testing of mobile devices ISO/IEC TS 19795-9:2019 — Information technology — Biometric performance testing and reporting — Part 9: Testing on mobile devices ISO/IEC 20059 — Vulnerability of Biometric Recognition Systems with Respect to Morphing Attacks ISO/IEC 9868 — Remote biometric identification systems — Design, development, and audit ISO/IEC 29794-5 — Face image quality assessment ISO/IEC TR 22116:2021 — A study of the differential impact of demographic factors in biometric recognition system performance ISO/IEC 19795-10 — Quantifying biometric system performance variation across demographic groups ISO/IEC 39794-5:2019 — Information technology — Extensible biometric data interchange formats — Part 5: Face image dataOne observation that I must confirm: SC 37 appears to be oriented to controlled-environment biometric systems: where the operator of the system controls the sensors, hardware and software.
I believe, for now, that there are additional design requirements for the exponential-growth bring-your-own biometric sensor (on your mobile device). And this aspect is very interesting to me. My company’s product does remote ID Proofing and Verification using biometric evaluation for linking, and biometric re-verification (aka “returning user authentication”). We construct 3D face models for proof of liveness and matching. We are concerned with proven liveness and end to end device/sensor security (for mobile devices cameras and webcams).
Performance testing, presentation attack detection/performance, mobile-first and face-first topics are hot now.
I’m about to find out the level of international interest and state of standardization in these areas during the SC 37 plenary at Okayama in January 2024 – wish me luck!.
Realizing that ‘active coal face’ is a dying idiom — but what is the climate-friendly replacement? Maybe the ‘code repo pull requests page’? I invite you to respond! ︎December 22, 2023 was my last day at The New York Times. My tenure was just under two and a half years.
My first 19 months at the company were pretty much everything I had hoped, as I collaborated with Design and Product to design a distributed platform and conceived, designed, and implemented CipherDoc, a service for encrypted data management. I’m incredibly proud of that work!
But alas, plans change. After the company mothballed the project, I refocused my time on glue work: re-platforming services, upgrading runtimes and dependencies, improving logging and observability, and documenting code, architectures, and playbooks. It felt good to reduce the onboarding, maintenance, and on-call overhead for my teams; I hope it helps them to be more productive and fulfilled in their work.
I treasure the terrific people I met at The Times, just super thoughtful, empathetic, and creative co-workers, partners, and colleagues. It’s no wonder they had the energy to form a union, The Times Tech Guild, for which I’m gratified to have helped organize and steward members. The Guild connected me with a far broader range of talented and committed people than I would have otherwise. I will miss them all, and continue to cheer for and support the movement from the outside.
And now, as 2023 winds down, I’ve decided to try something new. More news in the new year!
More about… Personal Work New York TimesIn 2019 I decided to leave independent consulting and join a large corporation – IDEMIA – you might notice their logos at airport security stations. My job was to be embedded in the ISO subcommittee for Mobile Driving License, a.k.a. the ISO 18013-5 mDL standard and to support IDEMIA’s mobile eID/mDL product team. This was a great extension of my existing work on digital credentials in other ISO subcommittees and W3C groups. Being tied to a product team was a new experience for me!
In 2021 I moved to Ping Identity to support the product team for PingOne Neo – ID Verify and Digital Credentials. My focus remained on government-issued digital credentials via ISO mDL and to establish implementations of inter-company digital credentials . I had the privilege of working beside incredibly experienced and generous standards experts who continue to be deeply embedded in OpenID Foundation, IETF, FIDO, Open Wallet Foundation core work. The novelty of Ping was to see how a new product can be engineered to fit into an overarching suite of enterprise products. The interplay of product and corporate priorities on the one hand and the long time scale of standardization on the other led to some “interesting” discussions over my time at Ping.
One constant throughout has been my involvement with Kantara Initiative. This non-profit corporation continues to be a bright light in the world of trustworthy use identity and personal information. I’m currently Chair of the Identity Assurance Work Group and since 2023, Chair of the Board of Directors. Kantara has had great fortune in our Executive Directors over the years – from Joni Brennan to Colin Wallis and now Kay Chopard. The Board is working hard to strengthen our program offerings (as a conformance body for NIST 800-63 and the UK DIATF), expand into new geographies, and sustain innovation in our community work groups – while fiscally prudent and in line with our fiduciary duty to the organization and its members.
At the end of 2023, I have decided to pivot my attention to a big missing piece in the digital credentials story – how to ensure that the recipient of a digital credential is a real human (and the intended one), and that the presenter of a digital credential is in fact authorized to do so. I have joined Facetec to dive deep into the world of liveness-proven biometric verification. Their tech is impressive. The SDK uses the mobile device camera to construct a 3D face model of the person from which it determines whether there’s a real living human present, whether the session is a spoof attempt (using physical artifacts or video playbacks), and whether images are being injected bypassing the camera subsystem. The server SDK completes the liveness analysis and performs highly-accurate matching against a known-good reference. My job? Same-same. Working with international standards bodies (such as ISO SC 37) and testing labs to develop or extend standards for performance evaluation of this new 3D face biometric mode. I’m ingesting big stacks of documents and trying to work out where standardization of user-supplied biometric-enabled devices stands today – in comparison with the well-established border security/public safety modes of operation. This is exciting new ground and appears to be mostly greenfield – and it all ties back to the IDM and digital credentials industry.
Whether it’s the Indieweb or the Fediverse, you should not expect to be able to make a reply or do anything else other than view posts on someone else’s domain (e.g. example.com). If you end up on someone else’s domain and wish to interact with them, in general, you should hop back to your site or app and interact with them from there. It’s like riding a bike and you’ll soon forget it was ever a challenge.
I’m lucky to have two hands, let’s be clear, and the minor struggles I’ve had with them over the years don’t qualify as any kind of real hardship. Yet there have been a lot of small injuries — an ongoing annoyance made worse by being mostly my fault.
Consider the most recent incident. To relieve strain on my left hand, I switched a few months ago from a steel-string to a nylon-string guitar. I knew I wanted the lesser force required to press nylon strings, and the vacation from steel strings has really helped. The wider spacing between the strings is also better for my hands, I realized as I settled in. I’d started on a classical guitar, then hadn’t owned one in decades, it feels good to have one again and I’ve been playing it a lot.
Being the guy who wrote a blog post about an early warning system for RSI not even six months ago, I see the absurdity of my situation. Back in July I was rehabbing an overextended little finger. Now I’m rehabbing a thumb and related muscles insulted by my failure to properly adapt to the new instrument.
You can wrap your thumb around the narrower neck of a steel-string guitar in order to grab the lowest string. You can do that with the wider neck of classical guitar too. But as I probably learned the first time and then forgot, you really shouldn’t. A D major chord with F# in the bass is too much of a stretch for the thumb, at least for my thumb, on a classical guitar. You won’t see classical guitarists do that. Instead they’ll make a tripod with the index, middle, and ring fingers.
So once again I get to rewire my hand posture. Which, again, is a minor hardship, not remotely comparable to the guy I mentioned last time who had to switch sides and learn to fret with his right hand. As I also mentioned there, he found an upside. Now he’s a switch-hitter who can use both halves of his brain directly. In my case, I’m trying to embrace the rewiring as a way to break a habit and form new neural pathways. It’d be nice, though, if that weren’t always a response to self-inflicted injury!
But like I said, it’s a minor hardship. My hands could have been mangled in my dad’s car engine that one time, or in the anchor chain of Ben Smith’s boat that other time: two escapes from disaster that still provoke the occasional nightmare. I’m lucky to have these two hands, and again vow to take better care of them.
Why is it so hard (for me, at least) to detect and avoid injurious hand postures? I guess it’s because whatever you’re projecting — when you write sentences or lines of code, or play notes and chords — has to flow through your hands with minimal conscious attention to your hands. Note to self: pay more attention.
The use of the phrase “critical mass” in this NYTimes story about the enshittification of Goodreads stopped me in my tracks.
Give all of Goodreads’s issues, it might seem easy enough to encourage writers and readers simply to flock to another forum. Sites like The Storygraph and Italic Type have sprung up as promising alternatives, but they’re still far from reaching a critical mass of users.
Nuclear physicists know they are dancing with the devil when they bring fissile material to criticality. They also know that the reaction can be controlled, that it must be, and that the means of control obey well-understood principles.
Social sites typically push toward supercriticality with no such understanding. If Goodreads enshittifies at 125 million users, why would another service expect a different outcome at similar scale?
We can learn from a natural experiment. Not mentioned in the story is a long-running service, LibraryThing, that’s been going strong since 2005. I interviewed its founder and lead developer, Tim Spalding, back in 2008. Listening to that interview again today reminded me that everything I loved about LibraryThing remains important and matters even more now.
LibraryThing was, and remains, a place where you make and share lists of books in order to connect with other people and with books — not primarily via friend relationships but rather book relationships. It’s a small business that’s kept Tim and his crew happily engaged in serving a few million bibliophiles, some of whom pay a membership fee to be super-cataloguers.
I’m not in LibraryThing’s core demographic. Books aren’t as central to my life as they are to members of the service who carefully curate their own lists, tag books and authors, contribute to a common knowledge wiki, and write reviews. But I appreciate their work when I visit the site.
Today I added Ed Yong’s remarkable An Immense World to my list. Among the book’s dozens of reviews on the site, I found a 2000-word essay that usefully highlights many of the strange (to humans) powers of animal perception that Yong describes.
I guess LibraryThing isn’t on the Times’ radar because it hasn’t reached a critical mass of … what, exactly? Tens of millions of people? Hundreds of millions? I’m glad it hasn’t! That’s a recipe for meltdown. LibraryThing has been going strong, for almost two decades, in the Goldilocks zone: neither too little activity nor too much, just the right amount for meaningful experiences at human scale.
I feel the same way about Mastodon. Conventional wisdom says it’s dead in the water: “nobody” goes there, no Mastodon apps rank highly in the app stores. But if critical mass means operating at the scale of Twitter or Facebook, then who wants that? Who benefits from the inevitable enshittification? Not me, and probably not you.
LibraryThing shows that a controlled reaction, at smaller scale, is sustainable over time. Mastodon so far has been successful in the same way, and I see no reason why that can’t continue. Although Mastodon is young, my connections there date back to social networking’s roots in the early blogosphere. It feels like the right amount of critical mass. For me, a billion people on Mastodon is an anti-goal. I would much rather see hundreds or maybe thousands of healthy communities emerge, each operating in its own Goldilocks zone. Many small and safe critical masses, instead of a few huge and dangerous ones, powering small businesses whose proprietors are — like Tim Spalding and his crew — able to maintain real relationships with their customers.
That global conversation we thought we were having on Twitter? We don’t know how to control that reaction and I’m not sure it makes sense to try.
As I have come to see it, the world consists of mounds of information, much of it digital. Our days are spent encountering, reacting, and sifting through it. Our conscious genius pulls together threads from which are crafted uplifting, foreboding, humorous, and tragic stories. We live inside these stories alone or with like-minded people. Most of the time our minds simply tweak and update unfolding dramas of consensus reality or a particular flavor of alternate reality; it’s an efficient, largely automatic process.
Rarely do we toss out whole plot lines and start from scratch. Why? Well, there would be so many subplots left hanging and so many question marks around our relational identities that the uncertainty of getting everything sorted out means it’s generally easier to keep on the established path. Jettisoning a comfortably broken-in outlook is a very messy business; and how often, really, do we feel the need to, or empowered to, rip apart the boxes we inhabit?
Each of us has the ability to craft amazing stories out of fascinating facts and extend invitations to loved ones and complete strangers to come inside and look around, sit a spell, and make themselves at home. There is, however, no way to compel another person to hang out in the story you made. You can’t even force them to cross your threshold. Exhort and cajole all you want, the stories we inhabit remain a personal choice, as they should be. Just as you wouldn’t want to have to live inside someone else’s ill-fitting story, the same goes for everyone else. It’s a hard truth. It can hurt.
Our acceptance of the primacy of the digital world has meant that the velocity and intensity of social physics has changed. My experience of these changes have been dramatic and potentially catastrophic, at least in the short run. The web3 protocol layer system seems intent on launching us all into a digital-twin simulation cloudmind whether we’ve elected to participate or not. Left in the wake of this century-long noetic campaign is the wreckage of once deeply-held relationships, relationships with those who are (or were) close to us in heart – actual physical hearts, not emojis, up-votes, and clicks.
There are too many digital stories, too many doors, too much unease with the masses either searching for answers or working really hard to avoid seeing the holes being torn in the fabric of what we once understood as “reality.” Our story rooms may now be filled with people from far flung corners of the globe. These are people whose heart fields we’ll probably never have the opportunity to feel directly – no mingled auras from warm embraces, conversations over cups of tea, shared meals, card games, porch sitting, bonfires, jam sessions.
In some cases, those whose heart fields we shared in the actual world have wandered off to the digital rooms of sirens, forsaking old connections for greener pastures. The transformation, turbo-charged by the lockdowns, happened with ferocious speed and offered little chance for redress, remedy, or reconciliation. We didn’t know what hit us. Then it was over, and everything had changed.
This is the first Christmas I’ve ever spent alone, totally alone. I was cancelled for breaking out of my box, looking around, asking questions, and imploring people to start having conversations about emerging technology and cybernetics and finance and consciousness and gaming before we go the way of the Lotus Eaters. Maybe I was cancelled for my intensity. I don’t know, the only thing I was told is that it was about “my research.”
I’ve made it through a tearful week. Lots of tears shed during my Christmas Eve read aloud finishing “Momo.” I apologize for the five-month gap, but I know in my heart I was supposed to read it last night. I keep saying time is not what we think it is. In the end of Ende’s story, the hour-lily wielding Momo and the tortoise Cassiopeia save the day and defeat the time bankers. The children return to the amphitheater for free play, grown-ups shelve their Taylorist tendencies, the town’s humanity is restored, and Momo doesn’t have to be alone anymore.
The “Momo” read-aloud playlist here.
I feel we are on Earth, sparks of God’s divine creation, to experience, falter, learn, and feel in the depths of our souls. The holidays are a season of big feelings – even more so when you’ve lost a loved one to the great beyond and other loved ones to the pit-traps of sinister social physics. The afternoon was mild here in Philadelphia, and I took the opportunity to fill my basket with gatherings and head out to the Kelpius Cave in the Wissahickon- alchemy of the soul. What better balm could there be than to lay out a heart-felt intention to close a rocky year?
When I thought that I was going to decamp to Seattle in the summer I took all of my precious nature materials with a friend to Valley Forge and created a magnificent spiral between Mount Misery and Mount Joy. Since I returned, I’ve accumulated a few more items – feathers and quartz from Arkansas, acorns from Laurel Hill cemetery, dried flowers from birthday carnations I bought myself, and gifts from friends across the country. I took them with a candle to the park and lit up the darkness and asked God for guidance to do the next right thing.
Once you’ve ripped up the boxes you really need a divine compass.
The coming year should bring new opportunities in Hot Springs, Arkansas after we sell the family home in the spring – fingers crossed someone wants to pay a good price for a well-loved 1880s row house with many original features. I’m hoping 2024 will be gentler than the clear-the-decks tsunami of the past six months. I met my husband when I was twenty. We grew up together. This divorce is my first break-up. I know that sounds ridiculous, and yet it’s true. I am my own person, and I know my own mind. That said, I’m going to have to craft my own new traditions and ways of doing things as a single person and doing that feels overwhelming right now. Will I ever celebrate Christmas again? It’s hard to say. It’s also been two years since my child has spoken to me. Prayers for reconciliation would be welcome. I still love my family. I am fine with them living in the story of their choice. I think we could create a shared space that bridges our separate boxes.
Despite the waves of difficultly I’ve been navigating since 2019, I know I would die a dysfunctional person had I elected to live the rest of my life inside stories I knew to be fundamentally flawed in their incompleteness and injurious to my spirit. So, I’m just going to put one foot in front of the other and try not to be afraid of a future with a lone heart-field.
I’m Jerry Hawver’s daughter, and Hawvers “git er done.”
Merry Christmas everyone. Thanks for stopping by my story, even if we can’t mingle our actual auras. May the new year bring blessings to you and your loved ones. Our power is in the heart – hugs all around. Hug your people as much as you can while you have them near you. It’s a sad day when you have no one to hug.
A light in the darkness.
Stones and brick, rubble from the floor of the “cave,” outline the heart. A ring of soft pine needles mingled with dried purple carnations and white Alstroemeria sprinkled with green leaves gifted from California and the last of my creosote gifted to me last year by Dru in Tucson for my birthday – it smells like the desert in the rain.
The next ring was an outline of pinecones gathered in the park with ferns and white pine sprigs and gifted dandelion seed heads that lit up the dark like stars.
I used a large stone as a centerpiece to hold many assorted lovely things lit by a candle.
A soft, leathery buckeye casing holding a spiral shell and manzanita berries with some Arkansas quartz below it.
Quartz and a shed snakeskin for sparkly new beginnings.
The last sprig of sage from Standing Rock gifted to me by Jason with a bit of fungus above it and acorns from which mighty things grow.
And an oak leaf for strength and fortitude.
River pebbles – may the rich tapestry of our experiences, both beautiful and challenging, rub us to a polished crystalline smooth symmetry.
May we have the wits to find our way through the worm holes and arrive on the other side.
Smoke from a wrapped mullein leaf gifted to me by a beautiful family in Washington state, thank you kind herbalist and standing farmer.
And this ridiculous figurine was already there when I arrived, in a niche made from where a portion of the wall had fallen away. I have no idea of the meaning behind it, but I suspect the trickster energy was perhaps expecting me. Bittersweet.
Philadelphia has brought me a depth of understanding my life would not have had if I had landed somewhere else. While I am grateful for its many stories, I see it’s time to move on. Goodbye for now Kelpius Cave, ritual anchor for the Nephele era.
I do a lot more cycling in Sonoma County, California than was possible in Cheshire County, New Hampshire. The Mediterranean climate here, which enables me to ride year-round, is a blessing for my mental and physical well-being. And because the topography is even more rugged, I’m doing more climbing that ever.
Yesterday, Luann dropped me at the Coleman Overlook on the coast and I cycled home from there. I’m not a fast climber, I can’t keep up with younger friends when we cycle together, and my rides aren’t extreme by local standards. But my cumulative elevation gain over the course of a year far exceeds what it ever was back east, and I’ve had plenty of time to reflect on climbing strategy.
A better gear ratio would help, but my older road bike won’t accommodate that. So on the steepest pitches I switch to a weaving ascent that eases the grade, which I’ve decided is OK. For a while I resisted shoes with cleats that lock into pedals, but now I use them to gain extra leverage which really helps.
It’s never mainly about the equipment, though, the real challenge is always mental. How do you think about reaching the top of the big hill you’re climbing? One piece of conventional wisdom: don’t look ahead. If you look down at the road you aren’t forced to think about the grade, or your slow progress up it. Instead you see pavement moving beneath you, and feel steady progress.
Of course that defeats the purpose of cycling through spectacular Sonoma County landscapes. Recently a friend suggested a different strategy: look to the side. Of course! There’s little or no traffic on many of these routes, so it’s safe to do that. And the effect is mesmerizing.
I’ve described it like this:
Everything looks different from everywhere. You’re always seeing multiple overlapping planes receding into the distance, like dioramas. And they change dramatically as you move around even slightly. Even just ten paces in any direction, or a slight change in elevation, can alter the sightlines completely and reveal or hide a distant landmark.
So, don’t look ahead to the top of the hill, and don’t look down at the road. Look left and right to see sliding panels of majestic scenery. It really helps!
Yesterday, NPR published a 4-minute interview Number of journalists killed in Gaza since Oct. 7 attacks called unprecedented loss with the president of the nonprofit Committee to Protect Journalists. CPJ maintains a list at Journalist casualties in the Israel-Gaza war.
Until this unprecedented threat to journalists is mitigated, I would expect to continue to hear a whole lot of nonsense.
Ten years ago today the drafts that would be approved as the final OpenID Connect specifications were published, as announced in my post Fourth and possibly last Release Candidates for final OpenID Connect specifications and Notice of 24 hour review period.
The adoption of OpenID Connect has exceeded our wildest expectations. The vast majority of federated signins to sites and applications today use OpenID Connect. Android, AOL, Apple, AT&T, Auth0, Deutsche Telekom, ForgeRock, Google, GrabTaxi, GSMA Mobile Connect, IBM, KDDI, Microsoft, NEC, NRI, NTT, Okta, Oracle, Orange, Ping Identity, Red Hat, Salesforce, Softbank, Symantec, T-Mobile, Telefónica, Verizon, Yahoo, and Yahoo! Japan, all use OpenID Connect, and that’s just the tip of the iceberg. While OpenID Connect is “plumbing” and not a consumer brand, it’s filling a need and doing it well.
It’s fitting that the second set of errata corrections to the OpenID Connect specifications were just approved, as described in the post Second Errata Set for OpenID Connect Specifications Approved. While we are proud of the quality of the final specifications, with 9 3/4 years of thousands of developers using and deploying the specifications, it’s unsurprising that issues would be found that needed clarification and correction.
The updated OpenID Connect specifications have just been submitted to the International Organization for Standardization (ISO) for Publicly Available Submission (PAS) status. Approved PAS submissions are published as ISO specifications. This will foster adoption in jurisdictions that require using standards that are published by organizations with international treaty status.
Celebrations of the tenth anniversary of the approval of OpenID Connect will occur worldwide in 2024. The first will be in Asia at the OpenID Summit Tokyo in January. The second will be in the Americas at Identiverse in May. The third will be in Europe at the European Identity and Cloud Conference in June. Join us at these events for the celebrations!
I can’t wait to see what the next decade brings for OpenID Connect!
Recently I made a post on Veramo and Verified Credentials where I discussed a little bit about Veramo and my findings in trying to use it. This post is about DIDKit from SpruceID. DIDKit is another set of packages for creating DIDs, verifiable credentials, verifiable presentations, etc. I only used the command line interface (CLI) tool in my experimentation with it and was entirely focused on DID creation. This is how it went.
First off, let me say that I tried DIDKit not only because I wanted to see how another tool worked but because when using Veramo there is no way via the CLI to get the private key for a DID I created. I suspect with enough magic it could be retrieved via JavaScript, but maybe not. It’s locked away in a key management system (KMS).
Secondly, I found Create and Post Your Own did:web post to be easier to navigate than the directions on the SpruceID site. I did find somewhere in the DIDKit docs how to create a did:web from their did:key generation command but can’t find it again which only goes to show how difficult it is to find some information in their documentation. (Since initially writing this I looked harder and did find it at “did-web in minutes”)Although SpruceID says it supports did:web it seems that it can resolve did:web but it doesn’t create a did:web fully. You must create a did:key and make some edits on it to make it into a did:web. The edits are minor, but I consider this a weird limitation. I read somewhere along the way that it only supports the ED25519 curve in creating did:key/did:web, but I am not sure if that limitation referred only to creation or resolving as well.
Once I created a JSON Web Key (JWK) using one of their key generation functions, built the did:key from the key, edited it to be a did:web, and published it to manicprogrammer.github.io/.well-known/did.json it resolved fine with their “didkit did-resolve” command.
Unlike the Veramo tool, I found no way through the CLI to add services so I just manually added a DIDComm service to the DID. As with my Veramo post let me say — don’t message me on that DIDComm service as I don’t have nor have I found a mobile device agent/wallet to which I can add an existing DID and mediator endpoints for DIDComm much less the DIDComm v2 endpoint I gave it. So I can’t get the messages. Even blocktrust.dev which has the mediator doesn’t have such a thing. Perhaps I’ll setup a browser based DIDComm messaging app to test it out if I can find a DIDComm V2 set of libraries for the browser. (I’ll have to get proficient with JavaScript though, which I have never found the time to do.) Why do I have the endpoint in the DID then? Just for fun to see that I could add one and it properly resolve. Obviously, I have not been able to test it.
What else did I find in my experience with DIDKit? In the documentation, I couldn’t find what the below ‘key” parameter did. But, following the directions to create a did:key the below command worked:
didkit key-to-did key -k issuer_key.jwkI also found that on its Github it doesn’t have a discussion section.
All in all, the toolkit did a good job from the CLI. I am likely to try out the creation of a VC with it. It has packages for Rust (in which DIDKit is written), C, Java/Android, Python, and Javascript.
A few weeks ago I messed around with the Veramo project for #verifiablecredentials. It’s not ready for prime time nor is it professed to be, but it does get some things done. I focused on DIDs. I was entirely using the CLI as javascript is not a “native” language for me but I may decide to get into that space to mess with this project some. The deficiencies I ran into may be CLI specific and not present when using the APIs directly.
I did get a well-formed DID out of it that I published at:
did:web:manicprogrammer.github.ioDon’t send me a didcomm message via it despite it having a service for that defined as I don’t currently have a personal agent setup to communicate via that service. I know, why publish the service then? For fun. Maybe I’ll remove it.
One issue I hit with the CLI that was especially off-putting to me is that I found no way to get the private key via the CLI for the created DID, even with the did export function (perhaps I am wrong, but I didn’t see it and thus wonder what the value of the export is). If someone finds me wrong let me know. <EDIT>: since writing this I have prompted a question about it and confirmed what I found. See here.</EDIT> It uses by default a local KMS. This means I can only create and send credentials created by Veramo as I don’t have the private key for signing via another method. I suppose I can get to it via some javascript magic and I may try to do that or else I’ll likely create a different did with keys I have generated in other ways.
All in all. It was worthwhile to play with from the CLI. No doubt, the real value is in the Javascript APIs. What’s it good for? I don’t really know yet. It’s good for messing around and becoming more familiar with verifiable credential structure, verifiable presentation structure, DID structure etc.
#decentralizedidentity #verifiablecredentials
There is some major positive good news for the KERI & ACDC ecosystem. The European Banking Authority announced on LinkedIn the EBA Pillar 3 data hub which is built on KERI & ACDC. The Pillar 3 data hub uses the vLEI system developed by GLEIF, the Global Legal Entity Identifier Foundation.
vLEI System Architecture Diagram: What this meansThis means that around 6000 financial institutions in the EU will now be required to use the EBA Pillar 3 data hub, and by extension KERI & ACDC, for their financial reporting. You can read more in their website announcement and the linked report PDF.
The important parts include pages 50-52, specifically the Garner analysis in section 6.3.128:
And section 6.4.130 Conclusion:
“vLEI effectively meets…Pillar 3 reporting requirements…perceived as a low-risk project overall…significant opportunity to enhance integrity of reporting processes”A number of advantages and risks were identified with the paper saying
The automation of identity verification and related processes through the vLEI could also offer numerous potential advantages for both Financial Institutions and other Regulators in the EU financial market
https://www.eba.europa.eu/sites/default/files/2023-12/d5b13b4d-a9dc-4680-8b7c-0a3a4c694fac/Discussion%20paper%20on%20Pillar3%20data%20hub.pdf
Potential Advantages:
For Financial Institutions Non-repudiable identification This means once you say something with KERI you can’t un-say it, as in you can’t change your story without getting caught Operational efficiency: unified digital format Enhanced products and services: trusted information enables risk management, customer service, and enhanced online experience For Supervisors/Regulators Enhanced Trust: simplify both validation of regulatory reports and authorized sign off Comprehensive Entity Overview: transparent, aggregated view of legal entities and hierarchies Standardization of Data Processes: smarter, more cost-effective, reliable data workflowsRisks:
Development of ecosystem Due to ecosystem novelty there is only one QVI right now (Provenant), Support is needed from GLEIF to grow the population of QVIs, incentive structure, and ecosystem orchestration Ensure adequate support to institutions End-user applications bank wallet Wallet for reporting institutions Compatibility with eIDAS 2.0 framework User-friendly applications for digital signatures, key management, and logging services Market’s recognition of benefits offered by vLEI Pillar 3 reporting requirement could trigger a “snowball effect” to catalyze market recognition of vLEI benefits.This is an exciting development in the KERI space that this blog will continue to track.
Interoperability is a grand goal, and a tough problem to crack. After all, what is interoperability other than independent things just working out of the box? In the standards and specifications world, we have to be precise about a lot of things, but none more precise than what we expect to be interoperable with, and how we get to the interop point.
In my experience, there are a few common ways to get there.
ConformanceThe easiest way to achieve interoperability is for there to be no choice from the implementations. If there’s only one option, and implementations are motivated to follow that option, then interoperability is much easier to count on. If a specification has a MUST, and means it, you can realistically rely on that being followed by well-meaning developers.
This zero-level interoperability is not without its confusion, though. In any specification, there are a lot of assumptions that lead up to a MUST being placed. Changes in this context could make that MUST behave differently in between implementations. For example, taking the byte value of a data structure, even a simple one like a string or number, assumes an encoding and order for that data structure. What’s most dangerous about this kind of problem is that it’s easy for multiple developers to make the same assumptions and therefore assure themselves that the MUST as written is sufficient, until someone else comes along with different assumptions and everything breaks in spite of seeming to be conformant.
To give a concrete example, try asking anyone for a USB cable and chances are you’ll get a few different varieties back, from type A, to type C, to micro-B, to power-only varieties. All of them are USB and conform to all aspects of the cabling standard, but “USB” is not sufficient to ensure compatibility on a physical level.
Even with its limitations, it’s still a good idea for specifications to be specific as much as possible. But the world can’t always make a single choice and stick to it. Things start to get more interesting when there’s choice between options, though, so how do we handle that?
DiscoveryIf my system can ask your system which options it supports ahead of time, then ostensibly I should be able to pick one of those options and expect it to work. Many standard internet APIs are based around this concept, with an initial discovery phase that sets the parameters of interoperability for future connections.
This pattern works fairly well, at least for common options and happy paths. If your system supports some or all of what my system wants, then I can probably figure out how to connect to you successfully. If your system doesn’t support what I need, then at least I know I can’t start the process. OpenID Connect usually works from a discovery-based process, where the RP fetches the IdP’s discovery document prior to connecting.
The discovery pattern is predicated on an agreement of how to do discovery in the first place. I need to at least know how to make an initial call in order to figure out what the options are for all future calls. This is expected to be out of band for the rest of the protocol, but is often built on the same underlying assumptions. Does the protocol assume HTTP as a transport? Then discovery can use that, also.
Discovery is generally done without context, though. The existence of something in a discovery step does not guarantee that it will be usable in the context of a real request. A server might support seven different cryptographic algorithms, but might only allow some of them to specific clients or request types. That kind of detail is hard to capture through discovery.
For a physical example, let’s say that before you ask for a USB cable, you can check a list of all the available types that the person you’re asking has available. That way when you ask for a specific cable, you’ll at least know that they had it as an option. But maybe they only had one and already lent it out to someone else, or they only hand out power-only cables to people they haven’t met before, in case the cable goes walkabout.
NegotiationIf we can instead bake the discovery process into the protocol itself, we can end up with a negotiation pattern. One party makes a request that includes the options that they’re capable of, and the other party responds with their own set of options, or chooses from the first set. From there, both parties now know the parameters the need to connect.
This kind of option works well with connection-focused protocols, and it has the distinct advantage of avoiding an additional round trip to do discovery. There’s also no longer a need to specify a separate process for discovery, since it’s baked in to the protocol itself. Content negotiation in HTTP, algorithm selection in TLS, and grant negotiation in GNAP all follow this pattern.
Negotiation falls short when decisions have to be made about the initial connection, much like when there’s a separate discovery call. The protocol can be built to robustly account for those failures, such as a content type being unavailable in HTTP, but the ability to negotiate does not guarantee satisfactory results. Negotiation can also end up with less than ideal results when there’s not a clear preference order, but in such cases it’s possible for a negotiation to continue over several round trips.
If you need a USB cable, you can walk up to someone and say “Hi, can I borrow a USB cable? I need it to be USB-A or USB-C and I need it for a data connection.” The person you’re asking can then see if they have anything that fits your criteria, and choose appropriately from their supply. If they hand you something that’s less than ideal, you can clarify “I’d really prefer USB-C if you have it, but this will work if not”.
ConfigurationOn a simpler level, many developers simply want to choose an option and run with it, and if the other side makes a compatible choice, this can short-circuit any kind of discovery or negotiation process in a positive way. This might seem magical, but it happens way more often than many software architects and standards authors like to admit. It’s not uncommon for two developers to make similar assumptions, or for libraries to influence each others’ implementations such that they end up doing the same thing even without any external agreement to do so.
If a developer codes up something based on an example, and it works, that developer is not likely to look beyond the example. Why would they? The goal is to get something to connect, and if it does, then that job is done and they can move on to more interesting problems. And if it doesn’t work? Chance are they’ll tweak the piece that doesn’t work until it does work.
JSON works this way in practice, with well-formed JSON being the interoperability expectation and anything else being, effectively, schema-by-fiat. While there are schema languages on top of JSON, the practical truth is that applications apply their own internal schema-like expectations to the JSON by looking for a field with a certain name in a certain place with a data value that parses how they expect it to. Anything that runs afoul of that is an error not of JSON but for the application to deal with. This is a far cry from the days of XML, which expected processing of namespaces and schemas to make sense of tags at the parsing level. Was it more robust? Arguably, yes. But it was also far too heavy for an average developer to care about. JSON’s approach lets us get to data exchange simply by letting us get it right by accident most of the time, and ignoring things that don’t make sense.
If you want a USB-C cable but just ask someone for a USB cable, and they hand you a USB-C cable, everyone’s happy. You may have been accidentally interoperable with your request and response, but there’s power in that kind of accident and the frequency with which it happens.
PracticalityAll of these interoperability methods have merit, and most systems are built out of a combination of all of them in one way or another. When we’re defining what interoperability means, we always need to take in the context of what is interoperable, for whom, and when.At the end of the day, practical interoperability means that things connect well enough to get stuff done. We should endeavor to build our standards and systems to allow for robust discovery and negotiation, but always keep in mind that developers will find the best path for them to connect.
Interoperability is a grand goal indeed, and while a lot of the time we stumble backwards into it, there are well-trodden paths for getting there.
How do you survive in a world that is no longer optimized for making your own clothing when you suddenly find that modern conveniences no longer accommodate you? As a textile historian, Morgan has been ruminating for years about women’s contributions to the domestic economy, the massive time investment of producing clothing for a family, and the comparative properties of different textile fibers. These research interests were informed by a lifetime of sewing and other fiber crafts. None of this experience, however, properly prepared her to face the reality of needing to rely on her own hands to provide large portions of her own wardrobe.
Guest co-host Juliana Sims sits down with Morgan to talk about how, in the wake of a recently developed allergy to synthetic fabrics, she now finds herself putting that knowledge of historical textile production to use to produce clothing that she can wear.
Links and other notes:
Morgan presented this as a (much shorter) talk at the Dress Conference 2023 Slides from the presentation Morgan's Dissertation, which we also covered RSI Glove PatternThe quote that Morgan somewhat misremembered about a woman preparing wool before the winter:
"A thrifty countrywoman had a small croft, she and her sturdy spouse. He tilled his own land, whether the work called for the plough, or the curved sickle, or the hoe. She would now sweep the cottage, supported on props; now she would set the eggs to be hatched under the plumage of the brooding hen; or she gathered green mallows or white mushrooms, or warmed the low hearth with welcome fire. And yet she diligently employed her hands at the loom, and armed herself against the threats of winter." -- Ovid, Fasti 4.687-714
I participated in a meeting titled “Meta’s Threads Interoperating in the Fediverse Data Dialogue” at Meta in San Francisco yesterday. It brought together a good number of Meta/Threads people (across engineering, product, policy), some Fediverse entrepreneurs like myself, some people who have been involved in ActivityPub standardization, a good number of industry observers / commentators, at least one journalist, and people from independent organizations whose objective is to improve the state of the net. Altogether about 30 people.
It was conducted under the Chatham House rule, so I am only posting my impressions, and I don’t identify people and what specific people said. (Although most attendees volunteered for a public group photo at the end; I will post a link when I get one. Photo added at the bottom of this post.)
For the purposes of this post, I’m not going to comment about larger questions such as whether Meta is good or bad, should be FediBlock’ed immediately or not; I’m simply writing down some notes about this meeting.
In no particular order:
The Threads team has been doing a number of meetings like this, in various geographies (London was mentioned), and with various stakeholders including the types of people that came to this meeting, as well as Fediverse instance operators, regulators and civil society.
Apparently many (most?) invitees to these meetings were invited because other invitees had been recommending them. I don’t know whether or what kind of future meetings like this they are planning, but I’d be happy to pass along names if we know each other and you get in touch. Thanks to – you know who you are – who passed along my name.
The Threads team comes across as quite competent and thoughtful at what they do.
On some subjects that are “obvious” to those of use who have hung around open federated systems long enough like myself, many attendees seemed strangely underinformed. I didn’t get the impression that they don’t want to know, but simply that integrating with the “there-is-nobody-in-charge” Fediverse is so different from other types of projects they have done in the past, they are still finding their bearings. I heard several: “A year ago, I did not know what the Fediverse was.”
Rolling out a large node – like Threads will be – in a complex, distributed system that’s as decentralized and heterogeneous as the Fediverse is not something anybody really has done before. It’s unclear what can go wrong, so the right approach appears to be to go step-by-step, feature by feature: try it, see how it works in practice, fix what needs fixing, and only then move on to the next feature.
That gradual approach opens them up to suspicions their implementation is one-sided and entirely self-serving. I guess that can’t be avoided until everything is deployed they publicly said they will deploy.
While there are many challenges, I did get the impression the project is proceeding more or less as planned, and there are no major obstacles.
Everybody knows and is aware Meta brings a “trust deficit” to the Fediverse. The best mitigation mentioned was to be as transparent as possible about all aspects of what they plan and do.
I think that’s a good approach, but also that they can do far more on transparency than they have so far. For example, they could publicly share a roadmap and the engineering rationale for why the steps they identified need to be in this sequence.
There are many, many questions on many aspects of the Fediverse, from technical details, to operational best practices, to regulatory constraints and how they apply to a federated system. The group generally did not know, by and large, how to get them answered, but agreed that meetings like this serve as a means to connect with people who might know.
I think this is a problem all across the Fediverse, not specific to Meta. We – the Fediverse – need to figure out a way to make that easier for new developers; certainly my own learning curve to catch up was steeper than I would have liked, too.
Many people did not know about FediForum, our Fediverse unconference, and I suspect a bunch of the meeting attendees will come to the next one (likely in March; we are currently working on picking a date). Many of the discussions at this meeting would have been right at home as FediForum sessions, and while I am clearly biased as FediForum organizer, I would argue that doing meetings like this in an open forum like FediForum could help substantially with the trust deficit mentioned above.
There’s significant interest in the Fediverse Test Suite we just got funding approval for from the EU’s NGI Zero program. There’s general agreement that the Fediverse could work “better”, be more reliable, and more be comprehensible to mainstream users, if we had better the test coverage than the Fediverse has today. This is of interest to all new (and existing) developers.
There was a very interesting side discussion on whether it would be helpful for Fediverse instances (including Threads) to share reputation information with other instances that each instance might maintain on individual ActivityPub actors for its own purposes already. Block lists as they are shared today are a (very primitive) version of this; a more multi-faceted version might be quite helpful across the Fediverse. This came up in a breakout group discussion, and was part of brainstorming; I didn’t hear that anybody actually worked on this.
When we think of privacy risks when Meta connects to the Fediverse, we usually think of what happens to data that moves from today’s Fediverse into Meta. I didn’t realize the opposite is also quite a challenge (personal data posted to Threads, making its way into the Fediverse) for an organization as heavily monitored by regulators around the world as is Meta.
There was very little talk (not none, but little) about the impact on regulation, such as the “continuous and real-time access” provision in the EU’s Digital Markets Act and whether that was a / the driver for Fediverse integration.
There was very little discussion on business models for Threads, and where exactly ads would go. For example, would ads just stay within the Threads app, or would they inject them into ActivityPub feeds, like some companies did with RSS back in the days? Of course, should that happen, as a non-Threads Fediverse user, one can always unfollow; there is no way for them to plaster non-Threads users with ads if they don’t interact with Threads accounts.
I came away convinced that the team working on Threads indeed genuinely wants to make federation happen, and have it happen in a “good” way. I did not get any sense whatsoever that any of the people I interacted were executing any secret agenda, whether embrace-and-extend, favoring Threads in some fashion or anything like that. (Of course, that is a limited data point, but I thought I convey it anyway.)
However, the meeting did not produce a clear answer to the elephant-in-the-room question that was raised repeatedly by several attendees in several versions, which is some version of: “All the openness with Threads, namely integration with the Fediverse, supporting account migration out from Threads etc, is the opposite of what Facebook/Meta has done over its history. What has fundamentally changed so that you now believe openness is the better strategy?” And: “In the past Facebook was a far more open system than it is today, you gradually locked it down. What guarantee is there that your bosses won’t follow the same playbook this time, even if you think they won’t?”
Personally I believe this question needs a better answer than has been given publicly so far, and the answer needs to come from the very top of Meta. The statement must have staying power beyond what any one executive can deliver.
I left the meeting with far more questions than I could get answered; but nobody wanted to stay all night :-)
My gut feel is that it is safe to assume they will do a reasonably fair, responsible job with a gradual rollout of federation for at least the next year or two or such. Beyond that, and in particular if it turns out creators with large follower groups indeed move off Threads at a significant rate (one of the stated reasons why they are implementing Fediverse support as creators have asked for this), I don’t think we know at all what will happen. (I’m not sure that anybody knows at this point.) And of course, none of this addresses the larger issues that Meta has as an organization.
In the hope this summary was useful …
(Source. Thanks tantek.com for initiating this.)
This two-hour talk provides additional context for Washington Sean’s guest post, here, about his experience of the Santa Paula Mountains and Thomas Aquinas College as an energetic gatekeeper. In it I walk through a map I made about a year ago that links Vanguard Corporation and Burroughs Research Lab in Paoli, PA to the Deveraux Foundation’s Campbell Ranch School for special needs children in Goleta, CA. Included are side explorations into Big Tech’s workforce development programs for autistic youth, bio-nano and theoretical physics research at UC Santa Barbara, Theosophy’s Ojai roots, the Bionic Woman, an Arco-affiliated Bauhaus sculptor who retired to Montecito, and IoT “Hollywood” cosmetics.
You can access an interactive version of the map below here.
Ineractive Map: https://embed.kumu.io/c1f287d3928ee50886f46ea7d8e83a37#untitled-map?s=bm9kZS1UNlR5dGYwSw%3D%3D
This open letter and touching memoir was written by my friend Washington Sean after listening to my recent ramblings about Trichotomy and anticipated territorial skirmishes over an imagined inter-dimensional bridge where piles of soul bound tokens might be held in the future, inert digital golems awaiting the spirits of inbound travelers. My birthday is later this week and this offering feels like a special gift. It was a delight to picture myself a bit in that magical place beyond the looming gate of St. Thomas Aquinas. And I didn’t have to carry 40 pounds of gear up a mountain!
I’ve been thinking a lot about multiverses and portals and one’s life work. After I finished the trichotomy stream, Washington Sean and I exchanged a few emails about consciousness and shipping companies – waves, you know. I brought up W.R. Grace and J. Peter Grace Jr. of the Grace Line, devout Catholics with ties to the Trappist Abbey in Spencer, MA outside Worcester. RFK Jr.’s dad Joseph was a fellow-Catholic colleague with the Maritime Commission. I sense that standardized contemplative prayer is a key element in the B-Corp social impact “moral economy,” a game where focused spiritualized attention will be used to fuel the Web3 global brain platform.
Below is the stream, I’ll put a screenshot of that map with a link in case you wish to explore where the Grace empire intersects with the Vatican and Zen Buddhist brownie bakeries staffed by former-felons. My friend is a talented photographer. Enjoy his windows into a time before the fire.
Interactive Map: https://embed.kumu.io/a90ed96b44ccb02e398d6f53f2ad3dbe#untitled-map?s=bm9kZS12QjBlMnNkOQ%3D%3D
Dear Alison,
Ok — so I am having a hard time with this last email you sent me. In the email you addressed the concept of a trichotomy as a means of explaining the differences between the mind/spirit, the body and the soul. And you also mentioned Thomas Aquinas and pointed to J Peter Grace and his role as a past Governor (Board of Directors) for a school called Thomas Aquinas College.
Peter Grace, “whose wife was a key player in Vatican II” was also mentioned in your email. This wasn’t unexpected as we had been discussing shipping magnates Stavros Niarchos and Aristotle Onassis and their connection to the Negroponte brothers (another typical conversation with Alison, right?) and somehow Peter Grace was mixed in, someone I’d never heard of before. And so, with all of these topics thrown into an email is nothing too out of the ordinary, as our correspondences are often filled with different threads and rabbit holes. But unknowingly on your part, you had sent something else that was embedded in your email — something else that caused a peculiar epiphany – a sort of unsettling realization about our research endeavors that led to an interesting reevaluation of my own past – my own lived experience.
Specifically, it was your mention of how Thomas Aquinas College was fitting into this mix. I know I’ve mentioned I grew up in Ventura County, but I’ve never told you about the significance of the College in my own life. Nor would I have ever had a reason to until now… So, this is another ‘bizarre’ coincidence — or perhaps more evidence of our ability to tap into the magical realism of our lived experiences – perhaps sharing a mutual ‘frequency’ or a least being signaled to look at certain topics, certain ideas, within a common bandwidth, listening for concepts and symbols that resonate with me, with us, right now.
Thomas Aquinas College now has two locations – and they did not open the Massachusetts location until 2019. But — and here is why this is weird and personal — the California campus is in Ventura County where I grew up. But not just that — the campus sits at the entrance of a popular trail head where I literally ‘grew up’ — as in — where I slowly turned into a man; and where I formed a relationship with God and nature; where I battled my place in the world and contemplated my existence in the future.
The CA campus is located in Santa Paula canyon along state Highway 150 between the communities of Santa Paula and Ojai. It was near the campus that the ‘Thomas Fire’ of 2017 started. The Thomas Fire was, for a brief period, California’s largest wildfire in total burned acreage, scorching over 280,000 acres, but it’s place at the top of the charts was quickly usurped by another raging firestorm in the following season. Several of my close friends lost their homes in that fire including my two best friends. A great effort was made by the local fire department to protect the college a few days after the east winds event was over and when the winds changed, and the fire began to burn back the way it had come. The college experienced only minor physical damage, but the traumatic impact of the fire still lives on in that part of Ventura County.
Ironically, even though I have called the Pacific Northwest home for many years, I was visiting a friend for his birthday in 2017 (on November 30th — surely just another coincidence that November 30th is the same day you sent this perplexing email) who was battling cancer for the 3rd bout. A couple days later his sister’s home in the Ventura foothills, where the birthday party was hosted, was completely obliterated. I looked online at the drone shots that were available and I could pick their house out – a pile of ashes with only a gun safe standing in what used to be the garage.
I was in town for only two nights, so the day after the birthday party in Ventura I visited my best friend’s home in upper Santa Paula whose house was very close to the origin of the fire that would erupt two days later, and it was among the first to burn down. Of the few things they had time to grab before they left, was the wedding plaque that we had commissioned to be carved in leather commemorating the year of their union (2012). My friend’s wife tells a great story of how she ran back into the house and had to use a stick to knock it off the wall since she could not reach it.
Their home was not far as the crow flies from the campus grounds, and it is odd in fact, that I can still remember driving down Highway 150 after my visit with them as I was on my way back home to my parents’ house where I’d soon bum a ride from my father and get dropped at the Burbank airport and catch the last flight back to Portland. But on that drive home that night I passed by the campus, and I can recall how raw and powerful the winds were already blowing. Call it a premonition, or a hunch, but the thought of fire entered my mind that night, right as I drove by the Thomas Aquinas College campus. Two days later, the winds would grow even more ferocious and would knock down an electrical wire, sparking the fire. By that Tuesday I had learned what had happened. And it was on a Wednesday morning that I would be delivering opening remarks for the small non-profit I was president of at the time. It was our annual awards breakfast and while I kept a cheery face for our sponsors and guests, inside I was solemn and grieving, and I couldn’t help but feel a tad guilty for having a premonition, not having the knowledge or intuitive sense of how to act on it, and then learning how many of my friends were now burned out of their homes, their lives forever altered.
The Thomas Aquinas College campus sits above the Santa Paula creek on a small bluff nestled between hilly terrain at the mouth of Santa Paula canyon. This is not a ‘mighty river’ or a mountain river that one might see or think of as imagined in one of the more pristine national parks of the Sierra Nevada. The Santa Paula creek is a typical river of the Los Padres National Forest. Much of the time, rivers in southern California run at a low flow rate and often they can barely muster a trickle or even dry up completely, running ephemerally for long stretches before entering the myriad of pathways into the greater water table. However, during periods of heavy rain, these same little creeks can turn into flood channels of immense power and geological change – deluges capable of swallowing huge swaths of land and altering the landscape in a matter of days or hours. The clay soils have poor drainage and cannot absorb the water fast enough. And after intense fires, the sage, manzanita, and scrub brush of the chapparal can leave a layer of plant derived oil that coats the surface with a thin, impermeable layer, further exacerbating the drainage issues. Thus, the Los Padres National Forest, and the terrain around the Santa Paula canyon are areas that have been experiencing rapid geological transformation – occurring in faster cycles than more stout or solid mountain ranges.
There is a sulphur spring near the mouth of the Santa Paula canyon. The smell is thick, and the pronounced stench of rotten eggs is hard to miss on the highway, as it just before the main trailhead parking areas. Up until very recently, by grant of an old easement with the Thomas Aquinas College, the National Forest Service, and the other ranch and property owners, the first mile of the trail actually cut through the campus property, ascending a few hundred feet in elevation along an asphalt road. The road crests by the Ferndale Ranch (home to a meandering flock of peacocks for many years) then drops down and heads around a bend. The last point on private property was just past the oil wells where the road ended, and a dirt trail formally began. It was only a few years ago that the campus was finally successful in obtaining new easement rights along the creek directly to the parking lots so that the official trail no longer goes through the campus property at all. But from the time I was a child and even through my 20’s and late 30’s the trail always involved that long, winding road.
My first trip into Santa Paula canyon was in 4th grade – as part of a day long field trip through my public elementary school. In fact, now that I think of it, lots of the public school kids in took day trips to the Ferndale Ranch (located just beyond the College campus) and hiked in to Punch Bowls — about 2 1/2 miles of hiking with a fairly steep and laborious slug up a hill at the end to climb for nine and ten year olds. The cliffs near the punch bowls were really dangerous — and the water could easily kill drown a child or adult during the non-drought years – as a series of cascading pools with increasingly large cliff jumps was more the stuff of adventurers and high school kids, not elementary or middle schoolers. I did not do the jumps until in my early twenties.
The year after I did the ‘outdoor education’ through my public elementary school, it would have been 1992 or 1993, the district discontinued the program as a tragic drowning occurred with another school — a 12-year-old boy passed away when he got sucked over the falls. But even though the schools stopped taking kids there, the popularity of the spot has only grown and my mother kept taking us there through our adolescence. In fact, she used to call it ‘church’ and on more than one occasion she called in sick for us and took us hiking so that we could “go to church.” Raised catholic, but never practicing, my mom considered it more important to be in nature than in a giant building when it came to praising God and his creation.
But it was later in my life that the East Fork trail and Santa Paula Canyon became even more important. One of my best friends, the one who would later lose his home near the origin of the Thomas Fire, was the one who introduced me and another friend to the camp. He had been there as a boy with his father, and he had sort of rediscovered it. Through the Thomas Aquinas college campus, up the Santa Paula Canyon trail and then past the popular ‘Punch Bowls’ (we always called them the moon rocks) hiking spot, was the old and in some spots, quite non-existent trail that went up the East Fork that led to the secret camp. Truth be told, it wasn’t really ‘secret’, and it was more like ‘rediscovered’. The camp, that we endearingly called ‘the land of one hundred waters’, was shown on some older maps, not on newer ones. But Santa Paula Peak, situated prominently above the valley where the camp was located, was listed on the Sierra Clubs’ Top 50 Peaks in California. And other prominent hikers knew of the camp and old trails. So, while it was sort of hard to get to and somewhat forgotten, the idea of it being a true secret was more romanticized than reality. The camp actually use to have a wagon trail to it (supposedly) in the late 19th century, where pioneer families would take extended vacations, but our ascent was always along the worn and mostly faded away and long neglected trail.
After the 2004-2005 floods, most of even the old trail washed away and it became more like boulder scrambling until the very last bit. Despite the technical difficulty and the lack of stable ground, the destination made it worth it. The camp was ‘a secret camp’ partly because it had some improvements that were added by a generation of hikers and campers that came before us. My friend’s father was a member of these hikers, part of a group of friends comprising an informal fraternity of sorts. The camp they built for themselves in the late 70’s and early 80’s included improvements that most camps do not have – especially those that are a full day’s hard walk through arduous terrain. The camp featured a twenty-foot long picnic table built out of a large fir tree that had been cut down and milled on site. There was also an asadero style bbq grill and an assortment of cast iron pots, skillets and other cookware. Nearby springs flowed year-round amidst a flat parcel of ground submerged beneath the deep shade of canyon live oaks that were hundreds of years old. Chumash griding bowls and mortars have been found in the area while exploring off the trails. About another thousand feet in elevation, above the camp, and along another worn and overgrown trail, there was located another camp sitting in huge boulders and cliffs comprised of sandstone and siltstone. The cliffs had this reddish tinge or hue to them that when hit with the last rays of sunlight, they’d turn the most amazing color before our eyes.
When we first started our hikes, the trail began by going through the large green cast iron gates at the entrance to the campus by the highway. On the weekends, the gates were locked and hikers were instructed by a sign to use the non-vehicle entrance. Strict instructions marked on signs all along the part of the road through the campus were hard to miss and the security guard was largely dedicated to making sure the hikers never wandered off the route. It almost felt intentional – the way the trailhead made the route so much longer. Surely, as we had tried on more than one occasion, there was a quicker, more efficient way through the campus. But on the weekends, hundreds, sometimes even over a thousand people on a real hot spell, would use the trailhead in search of the reliable swimming holes further up the canyon.
All in all, I have spent over a year of my life sleeping in this place. Starting at nineteen and through a lot of my twenties, I needed to be there, up the East Fork, at the secret camp, at the headwaters, near the eternal springs, nestled amidst the oaks and old growth firs, above the dominion of the Thomas Aquinas Campus. I wasn’t the only one. I shared this experience with my best friends. Even my wife has spent a few weeks camping up in this place and would know the college if mentioned. I could tell stories for hours of this place.
Five nights here, three or four days there – the days turned into weeks and the weeks piled into months. I soon realized that I was spending much of my free time going to this place. When I wasn’t working or on another adventure, I made my best effort to get my bag packed and get up the mountain. When I was faced with either getting a new roommate or moving into my van to save money, I chose my van. I was already spending so much time at camp – it was an easy choice and it helped me save money even quicker for the big trip to New Zealand I had been planning.
Quickly however, it became apparent that Thomas Aquinas College with its big cast iron gates and zealous security guards was a barrier to our adventures up the mountain. Eventually, even parking on the highway at the trailhead would become too risky as more than one of us had our windows smashed. So, finding a cut-through, or a quicker path to the get to camp was a matter of circumstance, not choice. The impedance of the college and its long and winding road became cumbersome and annoying. For most people, the hike ended at around the 3 ½ mile mark – when they got to moon rocks. But for us, that was only about a quarter of the way, and the biggest nuisance was always going through the gates and walking up the long and winding road around the campus water reclamation pond (fancy for on-site sewage treatment). It took at least thirty or forty-five minutes to walk that first section – getting past the oil wells and onto the rock and dirt track always offered a sigh of relief. A mile is not that far, but it is a noticeable distance that was hard to ignore with 40-50lbs of rations and gear stuffed in a backpack. Clearly, we were hindered and greatly bothered with what seemed extra or unnecessary labor put in front of us by the College.
So, we got bikes. We would use our bikes and ride up the hill along the asphalt path in extra low gear. Still physically difficult, but much quicker. And even better – on the way back we would really shave off the drag and droll of finishing a weekend of backpacking by plopping relentlessly along a hot, stinky asphalt path back to civilization. What better way than to whizz through the campus and down the hill in a mere instant – or about 25 mph. The security guard actually scolded us one time when we were riding our bikes too fast as even the cars were expected to observe a 15 mph speed limit.
Truth be told – there was actually another way to get to our secret camp – from an entirely different direction. So, in a sense, we could escape the dominion and the gates of Thomas Aquinas if we absolutely wanted to. We had found a way to our paradise that circumnavigated the more obvious and conventional path. But the other route featured its own drawbacks. First, it required an access code through a locked gate – so only certain people knew how to acquire the code (another easement battle from a forlorn era). But with the code, we could travel up the mouth of Timber canyon and ascend up the face of Santa Paula Peak. Like the Santa Paula Canyon trail, part of this felt redundant as there was, feasibly, a dirt road that would allow us to start closer to the actual forest (reserved public lands) lands. But we did not have permission to drive on the dirt roads. And this trail, being on an exposed and very sunny south face of a prominent 5,000 foot peak (the peak used to house a fire lookout tower and it was confirmed that a double-wide trail suitable for dirt bike travel used to exist all the way to the summit), was hard to climb during the day, and even in the winter months depending on the weather. We’d typically hike up the peak trail when cooler weather was around. On more than a few occasions we hiked up the peak at night. While better maintained and easier to navigate (up until 2021 washouts on the backside) this trail required more climbing and had a long descent into camp.
Weigh points along the peak trail last comprised of the following spots: ‘the house’ (burned in Thomas Fire but was the old trailhead start), the gate, the oaks (now known as ‘cow shit camp’, Kenny’s hill, the switchbacks, lunch rock, orange peel, soda pop, the peak spur, the saddle, the grassy spot, the last turn.
Weigh points along the canyon trail and the East Fork were last comprised of the following spots: The Gates (start of trail at highway), Ferndale, Noel’s place, the Oil well, the washout (aka ‘hell hill’), big cone, moon rocks, first crossing, log jam, poison oak camp, land of the milky white rocks, the witches portal, the flats, the spring trail, the meadow.
As I already mentioned, most hikers going up the canyon stopped just past big cone or the moon rocks (popularly known as the Punch Bowls), but our journey was always much longer. During the summer months we’d stash extra beers in the creek near the moon rocks for the last stop on our return hike home. Before retrieving our bikes (also stashed nearby) we would drink, swim and eat whatever last rations we had managed to save. Our adventures ended with riding the rest of the trail in a slightly altered state of consciousness, gleefully blowing past the other hikers.
Eventually we learned the cycle of the big weekend hikers and campers could occasionally yield substantial gleanings of food, clothes and an assortment of abandoned camping gear. Descending the trail after a big summer weekend filled with tourists came with the feeling of returning to civilization in phases – the moon rocks and surrounding camping areas functioned as a sort of open-air dumpster or secondhand store. Any valuable gear we found we would stash for our next hike up. But discarded food stuffs such as ultra-processed and flavor enhanced cheese chips or a bag (opened or not it wouldn’t matter) of fortified and enriched chocolate chip cookies was always a welcome addition to our favorite end of hike snack – the ‘tuna boat’ – a green bell pepper stuffed with chunk tuna and chopped onions, cilantro and jalapeno or serrano, and finished with a few dallops of hot sauce from a fast food condiment packet.
Groups of people would come to the moon rocks from the nearby towns of Ventura, Oxnard, Camarillo and Port Hueneme for day hikes. Sometimes even people from Los Angeles County would hike in the canyon near the popular swimming spots. In my lifetime, the canyon acquired great popularity and many young people found it a particularly cool place to ‘camp out and party’. The drinking and revelry often combined with a lack of experience in many of the camping basics –concepts that we took for granted like what to bring and how to stay nourished and hydrated were not taught in the limited curriculum of field trips and ‘outdoor education’ available in elementary school. This resulted in a great number of hang overs and many of the inexperienced campers simply abandoning leftover food, dirty clothes, and even perfectly good gear that just looked too heavy to carry to a nineteen-year-old with a throbbing headache. Their discarded leftovers were always our miraculous discoveries.
So much of my life, my energy field, the imprint of who I am (and who I am still to become) is in relation to that magical place. I have hundreds (probably thousands) of photos of my time in the East Fork canyon and Los Padres National Forest. I hope you can appreciate the significance of the coincidence and the elements of magical realism that present here.
Thomas Aquinas College was always a gatekeeper – not just a ‘way’ point, on our trail, but also, a ‘weigh’ point, a spot on the trail that weighed both our packs and our worthiness as we traveled through its dominion. After passing through the gates, it taxed us appropriately on our travels into higher realms.
The important work that I would do up in the camps and bluffs above the dominion of the college, beyond the realm of the material world, was spiritual work. True, our materialist trappings of fine cuts of meat, malted ales and other liquors, legumes, potatoes and assorted vegetables, they always traveled with us, as they served to literally weigh us down all along the chosen route. But the majority of the work done at camp was always spiritual work. In fact, one of my best friends, (not the one who lived right next to the fire, but who also lost his home(s) to the fire), worked for several years to construct his very own cabin, hidden away from camp up in the bluffs, nestled in its own little nook. It was a beautiful little forest cabin before it burned down in the Thomas Fire. It was comprised of a variety of native stone and hand-hewn lumber, and also some building materials he had carried in. It had taken him months of accumulated time to build it, almost all by his own hand. Before it burned down, he used to refer to it as ‘his life’s work.’
What is this notion of our ‘life’s work’? The time that I craved to be there – and the amount of time I spent there – for some it was perceived as escaping from work or escaping from responsibility – from existence in the material realm. But it never felt like that for me. It was a place of great importance, and learning. Of experiments and mysteries.
I didn’t understand it in this way then – but the important barrier (or bridge) that Thomas Aquinas College served in my travels isn’t just an inconvenience. It was a physical burden to have to pass through the gates of Thomas Aquinas College and walk the extra mile. But now, now I see that it was also a spiritual bridge that they controlled – or, more precisely, closely monitored.
At first, I used to believe that the college was not receptive of the hikers, and that they’d rather not have us there. The stern nature of the signs and security guards keeping everyone on the right path seemed bothersome to the pristine and peaceful environment that was cultivated on the campus grounds. But now, re-thinking the role not as an inconvenience to the college, but instead, as a chosen duty, or a higher calling, a sort of self-endowed guardianship over a gateway and control of the bridge, or the portal, from one dimension to the next. The college watched over my travels as I left material world and prepared to ascend into the spiritual world. They were monitoring my travel into and out of Gods splendor – now I see that it was not an accident that the college situated itself in this manner. The perceived hacks and different techniques my friends and I learned to get around the expectation and presumed relationship with the College as gate keeper and pathway enforcer were beneficial to us, but that did not diminish the college’s dominion over the bridge or the majority of other hikers. In fact, it was only because of our desire to return to the higher realms over and over again that the important role the college served became noticeable. Had I only hiked into the canyon on occasion, it would be easy to overlook. But having made this special place my literal second home, the college was always the first weigh point on the longer journey.
Thomas Aquinas College is not far from several other notable institutions of learning and foundations of spiritual significance. The Ojai Valley has been a center of new thought for well over a century now (and quite longer if considering indigenous cosmology). Just over four miles from the college campus as the crow flies, there is the Ojai Valley School, an alternative education school for children founded in 1911. And not far off, perhaps another mile or so further west is the Thacher School, established even earlier in 1887. Situated close to the Thacher school is the Krishnamurti Foundation for America, another well-known institution. It was in 1922, in Ojai, where Jiddu Krishnamurti is said to have had a “life changing experience.” Directly to the west of Thomas Aquinas College, about five miles away and sitting atop the summit of Sulphur Mountain is Meher Mount – a spiritual retreat center first established in 1946 and dedicated to the Avatar Baba Meher. A little further west and centrally located in the small town of Ojai is the Krotona Theosopical Society – first founded in Hollywood in 1912, and later relocated in Ojai in 1922. Yogi Paramahansa Yogananda was known to visit the area and helped found his spiritual sanctuary in 1950 as part of his self-realization fellowship. No doubt there are more places of spiritual and cultural significance in and around Thomas Aquinas College. The reputation of the area precedes itself.
However, in taking a step back and thinking about the college’s place differently, and in general contemplating what it is about this area that has drawn so many to the area in search of enlightenment, I could not help but wonder – where the energy is coming from? Is it inherent in the land, as if by a magical grid or ley lines? Or is this energy coming in from somewhere else?
Just south of the campus and the sulphur springs, about two miles away, is another site of importance that has reemerged as important – perhaps the most significant and important site of all – that suggests broadening my horizons even further – and asking, or perhaps, doing the work to begin to formulate new questions, better questions, that stretch my understanding of the intelligent forces at work in our world.
COMSAT, a global satellite communications company, established its teleport site in Santa Paula in 1975. The site is shown on the COMSAT webpage under its ‘commercial’ heading. But, as of November 1, 2023, COMSAT, and SatCom Direct, are now part of Goonhilly. The technical components at the site and the capabilities of the site far exceed mere commercial endeavors.
For many years I just assumed that the satellite dishes and arrays that are visible from the highway, just south of the gates at Thomas Aquinas, were nothing special – just more telecommunications equipment for the burgeoning cellular telephone industry. Now I understand that the array there is unique – and possesses capabilities to look farther into space – into a cosmic void where one day we might discover the true meaning of intelligence.
Goonhilly “is one of the world’s premier space communication gateways providing control and uplinks for satellites and deep space missions.” With the recent acquisition of COMSAT Teleports, including the Santa Paula site, Goonhilly is expanding “all communication service offerings from LEO (Low Earth Orbit) right through commercial GEO to lunar and deep space.”
Goonhilly’s first site was located at Goonhilly Downs, near Helston on the Lizard Pennisula in Cornwall, England. Under a 999-year lease from British Telecom (BT Inc.) it was one of the largest stations in the world at its inception and still possesses some of the most advanced capabilities on the planet. Its parabolic dish, nicknamed ‘Arthur,’ was one of the first of its kind. Its current largest dish, called ‘Merlin’ is equally impressive in the modern era.
Now, connected to the Santa Paula site next to the Thomas Aquinas College (and others around the earth), Goonhilly has positioned itself in many ways as its own kind of keeper, or guardian, of our ability to move from one dimension to the next. Goonhilly maintains the flow of information as it is transformed from the physical experience into digitized atoms and bits that are parceled and scattered into the heavens. As the information slowly coalesces and comes back together again, it is Goonhilly that is now positioned to monitor and carefully watch over our access to intelligence and information as we pursue our rightful place in existence and amongst the higher realms.
Space signaling, and earth-based radar as a form of communication and wayfinding from one dimension to the next, is a technologically complex subject that I don’t ever think I will fully understand. Nor should I have to. As long as I am alive, I will keep my eyes (and my heart) open to discovering new ways around the barriers or tolled bridges or locked gates that slow me down and impede my journey. As long as I am alive, I will look for ways to lighten my pack and quicken my journey as I pass by the weigh points.
By Washington Sean – December 2023
It was 11am at the Fort Lauderdale airport, an hour after my non-stop flight to Portland was supposed to have boarded. As I had been watching our estimated departure get pushed back in 15 minute increments, I finally received the dreaded news over the loudspeaker - the flight was cancelled entirely. As hordes of people started lining up to rebook their flights with the gate agent, I found a quiet spot in the corner and opened up my laptop to look at my options.
The other Alaska Airlines flight options were pretty terrible. There was a Fort Lauderdale to Seattle to Portland option that would have me landing at midnight. A flight on a partner airline had a 1-hour connection through Dallas, and there were only middle seats available on both legs. So I started to get creative, and searched for flights from Orlando, about 200 miles north. There was a non-stop on Alaska Airlines at 7pm, with plenty of available seats, so I called up customer service and asked them to change my booking. Since the delay was their fault, there were no change fees even though the flight was leaving from a different airport.
So now it was my responsibility to get myself from Miami to Orlando by 7pm. I could have booked a flight on a budget airline for $150, but it wouldn't have been a very nice experience, and I'd have a lot of time to kill in the Orlando airport. Then I remembered the Brightline train recently opened new service from Miami to Orlando, supposedly taking less time than driving there.
Brightline Station Fort LauderdaleNever having tried to take that train before, I didn't realize they run a shuttle service from the Fort Lauderdale airport to the train station, so I jumped in an Uber headed to the station. On the way there, I booked a ticket on my phone. The price from Miami to Orlando was $144 for Coach, or $229 for Premium class. Since this will probably be the only time I take this train for the foreseeable future, I splurged for the Premium class ticket to see what that experience is like.
Astute readers will have noticed that I mentioned I booked a ticket from Miami rather than Fort Lauderdale. We'll come back to that in a bit. Once I arrived at the station, I began my Brightline experience.
Walking in to the station felt like something between an airport and a car rental center.
There was a small ticket counter in the lobby, but I already had a ticket on my phone so I went up the escalators.
At the top of the escalators was an electronic gate where you scan your QR code to go through. Mine didn't work (again, more on that later), but it was relatively empty and a staff member was able to look at my ticket on my phone and let me through anyway. There was a small X-Ray machine, I tossed my roller bag and backpack onto the belt, but kept my phone and wallet in my pocket, and walked through the security checkpoint.
Once through the minimal security checkpoint, I was up in the waiting area above the platform with a variety of different sections. There was a small bar with drinks and snacks, a couple large seating areas, an automated mini mart, some tall tables...
... and the entrance to the Premium lounge.
Brightline Station Premium LoungeThe Premium Lounge entrance had another electronic gate with a QR code scanner. I tried getting in but it also rejected my boarding pass. My first thought was I booked my ticket just 10 minutes earlier so it hadn't synced up yet, so I went back to the the security checkpoint and asked what was wrong. They looked at my boarding pass and had no idea what was wrong, and let me in to the lounge via the back employee-only entrance instead.
Once inside the lounge, I did a quick loop to see what kind of food and drink options there were. The lounge was entirely un-attended, the only staff I saw were at the security checkpoint, and someone occasionally coming through to take out dirty dishes.
The first thing you're presented with after entering the lounge is the beverage station. There are 6 taps with beer and wine, and you use a touch screen to make your selection and pour what you want.
On the other side of the wall is the food. I arrived at the tail end of the breakfast service, so there were pretty slim pickings by the end.
There were yogurts, granola, a bowl of bacon and egg mix, several kinds of pastries, and a bowl of fruit that nobody seemed to have touched. I don't know if this was just because this was the end of the morning, but if you were vegan or gluten free there was really nothing you could eat there.
There was also a coffee and tea station with some minimal options.
Shortly after I arrived, it rolled over to lunch time, so the staff came out to swap out the food at the food station. The lunch options were also minimal, but there was a bit more selection.
There was a good size meat and cheese spread. I'm not a big fan of when they mix the meat and cheese on the same plate, but there was enough of a cheese island in the middle I was reasonably confident I wasn't eating meat juice off the side of the cheeses. The pasta dish also had meat so I didn't investigate further. Two of the three wraps had meat and I wasn't confident about which were which so I skipped those. There was a pretty good spinach and feta salad, and some hummus as well as artichoke dip, and a variety of crackers. If you like desserts, there was an even better selection of small desserts as well.
At this point I was starting to listen for my train's boarding announcement. There was really barely any staff visible anywhere, but the few people I saw had made it clear they would clearly announce the train over the loudspeakers when it was time. There was also a sign at the escalators to the platform that said boarding opens 10 minutes before the train departs.
The trains run northbound and southbound every 1-2 hours, so it's likely that you'll only hear one announcement for a train other than yours the entire time you're there.
The one train announcement I heard was a good demonstration of how quickly the whole process actually is once the train shows up. The train pulls up, they call everyone down to the platform, and you have ten minutes to get onto the train. Ten minutes isn't much, but you're sitting literally right on top of the train platform so it takes no time to get down there.
Once your train is called, it's time to head down the escalator to the train platform!
Boarding the TrainBut wait, I mentioned my barcode had failed to be scanned a couple times at this point. Let me explain. Apparently, in my haste in the back of the Uber, I had actually booked a ticket from Miami to Orlando, but since I was already at the Fort Lauderdale airport, I had gone to the Fort Lauderdale Brightline station since it was the closest. So the departure time I saw on my ticket didn't match the time the train arrived at Fort Lauderdale, and the ticket gates refused to let me in because the ticket didn't depart from that station. I don't know why none of the employees who looked at my ticket mentioned this ever. It didn't end up being a big deal because thankfully Miami was earlier in the route, so I essentially just got on my scheduled train 2 stops late.
So anyway, I made my way down to the platform to board the train. I should also mention at this point that I was on a conference call from my phone. I had previously connected my phone to the free wifi at the station, and it was plenty good enough for the call. As I went down the escalator to the platform, it broke up a bit in the middle of the escalator, but picked back up once I was on the platform outside.
There were some signs on the platform to indicate "Coach 1", "Coach 2" and "Coach 3" cars. However my ticket was a "Premium" ticket, so I walked to where I assumed the front of the train would be when it pulled up.
I got on the train on the front car marked "SMART" and "3", seats 9-17. It wasn't clear what "SMART" was since I didn't see that option when booking online. My seat was seat 9A, so I wasn't entirely sure I was in the right spot, but I figured better to be on the train than on the platform, so I just went in. We started moving shortly after. As soon as I walked in, I had to walk past the train attendant pushing a beverage cart through the aisles. I made it to seat 9, but it was occupied. I asked the attendant where my seat was, and she said it was in car 1 at the "front", and motioned to the back of the train. I don't know why their cars are in the opposite order you'd expect. So I took my bags back to car 1 where I was finally greeted with the "Premium" sign I was looking for.
I was quickly able to find my seat, which was not in fact occupied. The Premium car was configured with 2 seats on one side and 1 seat on the other side.
The Brightline Premium CarSome of the seats are configured to face each other, so there is a nice variety of seating options. You could all be sitting around a table if you booked a ticket for 4 people, or you could book 2 tickets and sit either next to each other or across from each other.
Since I had booked my ticket so last minute, I had basically the last available seat in the car so I was sitting next to someone. As soon as I sat down, the beverage cart came by with drinks. The cart looked like the same type you'd find on an airplane, and even had some identical warning stickers on it such as the "must be secured for takeoff and landing" sign. The drink options were also similar to what you'd get on a Premium Economy flight service. I opted for a glass of prosecco, and made myself comfortable.
The tray table at the seat had two configurations. You could either drop down a small flap or the whole tray.
The small tray was big enough to hold a drink or an iPad or phone, but not much else. The large tray was big enough for my laptop with a drink next to it as well as an empty glass or bottle behind it.
Under the seat there was a single power outlet for the 2 seats with 120v power as well as two USB-C ports.
Shortly after I had settled in, the crew came back with a snack tray and handed me these four snacks without really giving me the option of refusing any of them.
At this point I wasn't really hungry since I had just eaten at the airport, so I stuffed the snacks in my bag, except for the prosciutto, which I offered to my seat mate but he refused.
By this point we were well on our way to the Boca Raton stop. A few people got off and on there, and we continued on. I should add here that I always feel a bit unsettled when there is that much movement of people getting on and off all the time. These stops were about 20-30 minutes away from each other, which meant the beginning of the ride I never really felt completely settled in. This is the same reason I prefer a 6 hour flight over two 3 hour flights. I like to be able to settle in and just not think about anything until we arrive.
We finally left the last of the South Florida stops, West Palm Beach, and started the rest of the trip to Orlando. A bunch of people got off at West Palm Beach, enough that the Premium cabin was nearly empty at that point. I was able to move to the seat across the aisle which was a window/aisle seat all to myself!
Finally I could settle in for the long haul. Shortly before 3, the crew came by with the lunch cart. The options were either a vegetarian or non-vegetarian option, so that made the choice easy for me.
The vegetarian option was a tomato basil mozzarella sandwich, a side of fruit salad, and some vegetables with hummus. The hummus was surprisingly good, not like the little plastic tubs you get at the airport. The sandwich was okay, but did have a nice pesto spread on it.
After lunch, I opened up my computer to start writing this post and worked on it for most of the rest of the trip.
As the train started making a left turn to head west, the conductor came on the loudspeaker and made an announcement along the lines of "we're about to head west onto the newest tracks that have been built in the US in 100 years. We'll be reaching 120 miles per hour, so feel free to feel smug as we whiz by the cars on the highway." And sure enough, we really picked up the speed on that stretch! While we had reached 100-120mph briefly during the trip north, that last stretch was a solid 120mph sustained for about 20 minutes!
Orlando StationWe finally slowed down and pulled into the Orlando station at the airport.
Disembarking the train was simple enough. This was the last stop of the train so there wasn't quite as much of a rush to get off before the train started again. There's no need to mind the gap as you get off since there's a little platform that extends from the train car.
At the Orlando station there was a short escalator up and then you exit through the automated gates.
I assumed I would have to scan my ticket when exiting but that ended up not being the case. Which actually meant that the only time my ticket was ever checked was when entering the station. I never saw anyone come through to check tickets on the train.
At this point I was already in the airport, and it was a short walk around the corner to the tram that goes directly to the airport security checkpoint.
The whole trip took 176 minutes for 210 miles, which is an average speed of 71 miles per hour. When moving, we were typically moving at anywhere from 80-120 miles per hour.
Summary The whole experience was way nicer than an airplane, I would take this over a short flight from Miami to Orlando any day. It felt similar to a European train, but with service closer to an airline. The service needs to be better timed with the stops when people are boarding. The only ticket check was when entering the station, nobody came to check my ticket or seat on the train, or even when I left the destination station. While the Premium car food and drinks were free, I'm not sure it was worth the $85 extra ticket price over just buying the food you want. Unfortunately the ticket cost was similar to that of budget airlines, I would have preferred the cost to be slightly lower. But even still, I would definitely take this train over a budget airline at the same cost.We need more high speed trains in the US! I go from Portland to Seattle often enough that a train running every 90 minutes that was faster than a car and easier and more comfortable than an airplane would be so nice!
I did an impromptu live today trying to sort through issues around communication of complex ideas, navigating information streams, and collective thought fields. It’s a bit late, so I don’t have the wherewithall to summarize at the moment, but here are the papers referenced if you want to explore them.
My testimony at the Philadelphia School Reform Commission About Ridge Lane 4 minutes: https://wrenchinthegears.com/2018/05/17/yes-i-am-an-advisor-for-ridge-lane-superintendent-hite-may-17-2018/
Ridge Lane LP Credo (Tridentine Creed) – https://www.ridge-lane.com/our-credo
Attilla Grandpierre: The Physics of Collective Consciousness: https://wrenchinthegears.com/wp-content/uploads/2023/11/The-Physics-of-Collective-Consciousness.pdf
Knowledgeworks iLearn Whitepaper: https://wrenchinthegears.com/wp-content/uploads/2023/11/Knowledgeworks-Connections-to-the-Future-iLearn.pdf
Dana Klisanin: Transception: The Dharma of Evolutionary Guidance Media – https://wrenchinthegears.com/wp-content/uploads/2023/11/Transception-The-Dharma-of-Evolutinary-Guidance-Media-Dana-Klisanin.pdf
Automated Human Biofield Assessment and Tuning – https://wrenchinthegears.com/wp-content/uploads/2023/11/Human-Emotion-Recognition-Analysis-and-Transformation-By-Bioenergy-Field-in-Smart-Grid.pdf
Gordana Vitaliano (Nanoengineering / Addiction) – Tapping Into Subconsciousness Processing: https://web.archive.org/web/20021217014453/https://vxm.com/NLPVitaliano.html
Kneoworld Map: https://web.archive.org/web/20210104142455/https://littlesis.org/oligrapher/6018-kneomedia-gamified-edu-tainment
Hal Puthoff , Austin Remote Viewing: https://earthtech.org/team/ https://ciaotest.cc.columbia.edu/olj/sa/sa_jan02srm01.html
Evan Baehr of Learn Capital at the Vatican: https://www.youtube.com/watch?v=NWLy3m5gXH4
Here’s the latest installment in the series on LLM-assisted coding over at The New Stack: Puzzling over the Postgres Query Planner with LLMs.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
11 How to Use LLMs for Dynamic Documentation
Just before Thanksgiving, NPR‘s All Things Considered radio program had a short item on DMARC, a protocol that attempts to control fraudulent use of internet domains by email spammers by asserting that messages coming from those domains are authenticated using DKIM or SPF. Since I have been working in that area, a colleague alerted me to the coverage and I listened to it online.
A couple of people asked me about my opinion of the article, which I thought might be of interest to others as well.
From the introduction:
JENNA MCLAUGHLIN, BYLINE: Cybercriminals love the holiday season. The internet is flooded with ads clamoring for shoppers’ attention, and that makes it easier to slip in a scam. At this point, you probably know to watch out for phishing emails, but it might surprise you to know that there’s a tool that’s been around a long time that could help solve this problem. It’s called DMARC – or the Domain Message Authentication, Reporting and Conformance Protocol – whew. It’s actually pretty simple. It basically helps prove the sender is who they say they are.
Of course it doesn’t help prove the sender is who they say they are at all, it expresses a request for what to do when the sender doesn’t. But I’ll forgive this one since it’s the interviewer’s misunderstanding.
ROBERT HOLMES: DMARC seeks to bring trust and confidence to the visible from address of an email so that when you receive an email from an address at wellsfargo.com or bestbuy.com, you can say with absolute certainty it definitely came from them.
(1) There is no “visible from address”. Most mail user agents (webmail, and programs like Apple Mail) these days leave out the actual email address and only display the “friendly name”, which isn’t verified at all. I get lots of junk email with addresses like:
From: Delta Airlines <win-Eyiuum8@Eyiuum8-DeltaAirlines.com>This of course isn’t going to be affected by Delta’s DMARC policy (which only applies to email with a From address of @delta.com), but a lot of recipients are going to only see “Delta Airlines.” Even if the domain was visible, it’s not clear how much attention the public pays to the domain, compounded by the fact that this one is deceptively constructed.
(2) There is no absolute certainty. Even with a DKIM signature in many cases a bogus Authentication-Results header field could be added, or the selector record in DNS could be spoofed by cache poisoning.
HOLMES: So the thing about good security – it should be invisible to Joe Public.
This seems to imply that the public doesn’t need to be vigilant as long as the companies implement DMARC. Not a good message to send. And of course p=none, which for many domains is the only safe policy to use, isn’t going to change things at all, other than to improve deliverability to Yahoo and Gmail.
HOLMES: I think the consequences of getting this wrong are severe. Legitimate email gets blocked.
Inappropriate DMARC policies cause a lot of legitimate email blockage as well.
When we embarked on this authentication policy thing (back when we were doing ADSP), I hoped that it would cause domains to separate their transactional and advertising mail, use different domains or subdomains for those, and publish appropriate policies for those domains. It’s still not perfect, since some receive-side forwarders (e.g., alumni addresses) break DKIM signatures. But what has happened instead is a lot of blanket requirements to publish restrictive DMARC policies regardless of the usage of the domain, such as the CISA requirement on federal agencies. And of course there has been a big marketing push from DMARC proponents that, in my opinion, encourages domains to publish policies that are in conflict with how their domains are used.
Going back to my earlier comment, I really wonder if domain-based policy mechanisms like DMARC provide significant benefit when the domain isn’t visible. On the other hand, DMARC does cause definite breakage, notably to mailing lists.
Some riddles are so complex that people just stop contemplating, and concede. Here on the VRM dev list, over many years, the struggle to explain structural concepts in motion has fought for words in a social context that disavows progress by design. There is only one place where "self-induced Sovereignty" is approachable in accurate human terms, and conversing the methods elsewhere, like Canada, Europe, China, or via the modern Administrative State, etc... is a failure at inception, and the process of grabbing the word to apply policy obfuscations on accurate use is intentional. As has been discussed plenty of times, the State-defined "Sovereign citizen movement" is the most dangerous ideology in existence, and the people who live under its definition are the most dangerous people in existence. I mean, have you seen them all out there doing what they do, acting all Sovereign unto themselves? Scary stuff, right?
"Human Rights" is another great example, used pervasively in Government administration, and by identity entrepreneurs in aggressively collating the un-identified/ un-banked/ un-civilized. Powerful words and concepts are always open to perversion by intent, and require courageous stewardship to hold accountable to the actual meaning conferred by utterance. Most western societies operate under the belief that human rights exist, and their society protects them. Yet, in defining the human rights for and unto the human population being administered, the order of operations is strictly accurate, where words documenting process fall well-short.I've been trying to be intentional about using generative AI for more and more tasks in my life. For example, the image above is generated by DALL-E. I think generative AI is going to upend almost everything we do online, and I'm not alone. One of the places it will have the greatest impact is its uses in personal agents whether or not these agents enable people to lead effective online lives.
Jamie Smith recently wrote a great article in Customer Futures about the kind of AI-enabled personal agents we should be building. As Jamie points out: "Digital identity [is how we] prove who we are to others". This statement is particularly resonant as we consider not just the role of digital identities in enhancing personal agents, but also their crucial function in asserting ownership of our creations in an AI-dominated landscape.
Personal agents, empowered by AI, will be integral to our digital interactions, managing tasks and providing personalized experiences. As Bill Gates says, AI is about to completely change how you use computers. The key to the effectiveness of these personal agents lies in the robust digital identities they leverage. These identities are not just tools for authentication; they're pivotal in distinguishing our human-generated creations from those produced by AI.
In creative fields, for instance, the ability to prove ownership of one's work becomes increasingly vital as AI-generated content proliferates. A strong digital identity enables creators to unequivocally claim their work, ensuring that the nuances of human creativity are not lost in the tide of AI efficiency. Moreover, in sectors like healthcare and finance, where personal agents are entrusted with sensitive tasks, a trustworthy, robust, self-sovereign identity ensures that these agents act in harmony with our real-world selves, maintaining the integrity and privacy of our personal data.
In this AI-centric era, proving authorship through digital identity becomes not just a matter of pride but a shield against the rising tide of AI-generated fakes. As artificial intelligence becomes more adept at creating content—from written articles to artwork—the line between human-generated and AI-generated creations blurs. A robust, owner-controlled digital identity acts as a bastion, enabling creators to assert their authorship and differentiate their genuine work from AI-generated counterparts. This is crucial in combating the proliferation of deepfakes and other AI-generated misinformation, ensuring the authenticity of content and safeguarding the integrity of our digital interactions. In essence, our digital identity becomes a critical tool in maintaining the authenticity and trustworthiness of the digital ecosystem, protecting not just our intellectual property but the very fabric of truth in our digital world.
As we embrace this new digital frontier, the focus must not only be on the convenience and capabilities of AI-driven agents but also on fortifying our digital identities so that your personal agent is controlled by you. Jamie ends his post with five key questions about personal agents that we shouldn't lose sight of:
Who does the digital assistant belong to?
How will our personal agents be funded?
What will personal agents do tomorrow, that we can’t already do today?
Will my personal agent do things WITH me and FOR me, or TO me?
Which brands will be trusted to offer personal agents?
Your digital identity is your anchor in the digital realm, asserting our ownership, preserving our uniqueness, and fostering trust in an increasingly automated world, helping you operationalize your digital relationships. The future beckons with the promise of AI, but it's our digital identity that will define our place in it.
It was really nice of Elizabeth Garber to acknowledge me in the whitepaper that she co-authored with Mark Haine titled “Human-Centric Digital Identity: for Government Officials”. I recommend everyone read it, even if you aren’t in government, as it is a very strong and considerate effort to try and tackle a broad, complicated, but important topic. It reminded me that I was overdue to publish the talk I gave at Identiverse 2023, since it was her being in the audience that led us to have some nice conversations about our shared viewpoint on how Value-Sensitive Design and Human-Centric Design are key cogs in building ethical and inclusive digital identity systems. So, below is a re-recording of my talk, “Collision Course: Ethics vs Human-Centered Design in the New Perimeter”.
This talk was a challenging one for me to put together, because the subject matter is something I’ve been struggling to come to grips with. With digital identity becoming a critical component of a more digital-centric world, it seems clear that success and sustainability hinges on placing people and their societal values at the center of the architecture. But in doing so as part of my day-to-day work, I sometimes find the principles of human-centered design, inclusion, privacy-by-design, and ethics coming into conflict with each other. How are we, as identity practitioners, supposed to resolve these conflicts, navigating the challenge of building for the world that people actually live in, and not the one we wished they lived in? I increasingly found that there was no blueprint, no guide, already existing that I could tap into.
Well, I’ve often found that nothing brings things into better focus than being forced to develop a talk around it, so that’s what I set out to do. Can’t say I have the answers, but I did try to lay out some approaches and ideas that I found helpful when faced with these questions in the global projects I’m involved in. As always, I would love to hear people’s thoughts and experiences as they relate to this topic.
LinksIn the talk, I refer to a few resources that folks can use to learn more about Value Sensitive Design and Ethic in Tech. Below are links to the same:
Introduction to Value Sensitive Design Value Sensitive Design and Information Systems Translating Values into Design Requirements Ethics for the Digital Age: Where Are the Moral Specs? (Value Sensitive Design and Responsible Innovation) An Ethical Framework for Evaluating Experimental Technology Ethics-by-Design:Project SHERPA Value sensitive design as a formative frameworkOther links from the talk:
It’s getting easier to make an account on Mastodon An Introduction to the GDPR (v3), IDPro Body of Knowledge 1(5). Impact of GDPR on Identity and Access Management, IDPro Body of Knowledge 1(1) Code of Conduct: the Human Impact of Identity Exclusion by Women in IdentityTowards the end of 2019, I was invited to deliver a keynote at the OpenID Foundation Summit in Japan. At a very personal level, the January 2020 Summit was an opportunity to spend time with dear friends from around the world. It would be the last time I saw Kim Cameron in person. It would include a dinner with the late Vittorio Bertocci. And it was my last “big” trip before the COVID lock down.
At the Summit, I was asked to talk about the “Future of Identity.” It was a bit of a daunting topic since I am no real futurist and haven’t been an industry analyst for a long time. So I set about writing what I thought the next 10 years would look like from the view of a practitioner. You can read what I wrote as well as see a version of me presenting this.
A concept I put forward in that talk was one of “counselors”: software agents that act on one’s behalf to make introductions of the individual to a service and vice versa, perform recognition of these services and associated credentials, and prevent or at least inhibit risky behavior, such as dodgy data sharing. I provide an overview of these concepts in my Future of Identity talk at approximately minute 20.
Why even talk about counselorsThat’s a reasonable question. I have noticed that there is a tendency in the digital identity space (and I am sure in others too) to marvel at problems. Too many pages spent talking about how something is a fantastically hard problem to solve and why we must do so… with scant pages of follow up on how we do so. Additionally, there’s another tendency to marvel at very technical products and services that “solve the problem.” Except they don’t. They solve a part of the problem or they are one of many tools needed to solve the problem. The challenges of digital identity management are legion and they manifest themselves in different ways to different industry sectors in different geographies. One can argue that while we have used magnificent tools to solve account management problems, we really haven’t begun to solve identity management ones. Counselors are a way to both humanize online interactions and make meaningful (as in meaningful and valuable to the individual) progress on solving the challenges of digital identity management.
Counselors in the Modern EraSitting through the sessions at Authenticate 2023, and being surrounded by a ton of super smart people, I realized that the tools to make counselors real are very much within our grasp. Among these tools, 4 are the keys to success:
Interface layer powered by generative AI and LLMs Bilateral recognition tokens powered by passkeys Potentially verifiable data powered by Verified Credentials Safe browsing hints Interface layer powered by generative AI and LLMsAt their core, counselors are active clients that run on a person’s device. Today we can think of these akin to personal digital assistants, password managers, and digital wallets. What is missing is a user interface layer that is more than a Teddy Ruxpin clone that only knows a few key phrases and actions accompanied by zero contextual awareness. What is needed is a meaningful conversational interface that is contextually aware. Generative AI and large language models (LLMs) are showing promise that they can power that layer. And these models are now running on form factors that could easily be mobile, wearable, and eventually implantable. This would enable the counselor to understand requests such as “Find me the best price for 2 premium economy seats to Tokyo for these dates in January” and “know” that I’ll be flying out of D.C. and am a Star Alliance flier.
Recognition tokens powered by passkeysWe have got to get out of the authentication business. It fails dramatically and spectacularly and seemingly on a daily basis. We have to move to the business of enabling service providers and consumers to recognise each other. Both the original talk and my more recent Ceremonies talk speak to this need. A crucial puzzle piece for recognition is the use of cryptography. Right now the easiest way a normal human being can use cryptography to “prove” who they are is WebAuthn and, more generally, passkeys. Armed with passkeys, a counselor can ensure that a service recognizes the person and that the counselor recognizes the service. To be clear, today, passkeys and the ceremonies and experiences surrounding them are in the early stages of global adoption… but it is amazing to see the progress that happened in the prior year and it bodes well for the future.
One thing to note is that passkeys as they work today provide a form of cryptographic proof that the thing interacting with a service is the same one you saw the day before, and notional there is the same human associated with the thing. There is no real reason why, in theory, this couldn’t be flipped around such that the service has to provide a form of cryptographic proof that the service is the same one with which the thing interacted the day before. A counselor could broker these kinds of flows to ensure that the service recognizes the person that the counselor is working on behalf of and the counselor can recognize the service.
Potentially verifiable data powered by verifiable credentialsOne thing a counselor needs to do is to share data, on behalf of the individual, with a service. This data could be credit card information, home address, passport number, etc. Some of the data they would need to share are pieces of information about the individual from 3rd party authorities such as a local department of motor vehicles or employer. Ideally, the service would like a means to verify such information, and the individual, in some cases, would like the issuer of the information not to know where the information is shared. Here verified credentials (VCs) could play a role. Additionally, the service may want information about an individual that the individual provides and acts as the authority/issuer. Here too verified credentials could play a role. Standardized request and presentation patterns and technologies are crucially important and my hope is the VCs will provide them.
So why include the word “potentially” in the title of this section? There are many scenarios in which the service neither needs nor cares to verify information provided by the individual. Said differently, not every use case is a high assurance use case (nor should it be) and not every use case is rooted in a regulated sector. Hopefully VCs will provide a standardized (or at least standardizable) means for data presentation that can span both use cases that require verification and those that do not. If not, we’ll always have CSV.
Safe interaction hintsWhile one’s street sense might warn you that walking down that dark alley or getting money from that shifty looking ATM isn’t a good idea, an online version of that same street sense isn’t as easily cultivated. Here we need to turn to outside sources. Signals such as suspect certificates, questionable privacy policies, and known malware drop sites can all be combined to inform the individual everything from “This isn’t the site you actually are looking for” to “I suggest you do not sign up on this service… here are 3 alternatives” to “I’ll generate a one-time credit card number for you here.” One can imagine multiple sources for such hints and services. From browser makers to government entities to privacy-oriented product companies and well beyond. This is where real differentiation and competition can and should occur. And this is where counselors move from being reasonably inert cold storage layers for secrets and data to real valuable tools for an online world.
The missing something: privacyAt this point, I felt like I had identified the critical ingredients for a counselor: interface layer, recognition tokens, potentially verifiable data, and safe browsing hints… and then I mentioned this to Nat Sakimura. Nat has a way of appearing at the critical moment, say 10 words, and disrupt your way of thinking. I joke that he’s from the future here to tell us what not to do in order to avoid catastrophe. And I have been lucky and privileged enough to have Nat appear from time to time.
This time he appeared to tell me that the four things I had identified were insufficient. There was something missing. Having safe browsing hints is not enough… what is missing are clear, processable and actionable statements about privacy and data use. A counselor can “read” these statements from a site or service, interpret them into something understandable for the individual, better informing them on how the service will behave, or at least how it ought to behave. Couple this with things like consent receipts, which the counselor can manage, and the individual has records of what the service provider said they would do and to what the individual agreed to. There is an opportunity here for counselors to focus the individual’s attention on what is material for the individual and learn their preferences, such as knowing the individual will not accept tracking cookies.
From where will these counselors comeOne can easily imagine a variety of sources of counselors. The mobile operating system vendors are best-placed to extend their existing so-called smart assistants to become counselors by leveraging their existing abilities to manage passwords, passkeys, debit and credit cards, and along with other forms of credentials. 3rd parties also could build counselors much like we see with digital assistants, password managers, and digital wallets. I expect that there is a marketplace for services, especially safe browsing hints. Here, organizations from government entities to civil society organizations to privacy-oriented product companies could build modules, for lack of a better word, that would be leveraged by the counselor to enhance its value to the individual.
Regardless of where counselors originate, observability and auditability is key. An individual needs a means to examine the actions the automated counselor took and the reasons for the actions. They need a way to revoke past data sharing decisions and consents granted. And they need a means to “fire” their counselor and switch to a new one whilst retaining access, control, and history.
In conclusionWe, as an industry, have been working on identity management for quite some time. But, from some perspectives, we haven’t made progress. Pam Dingle once said something to me to the effect of, “We’ve built a lot of tools but we haven’t solved the problems. We are just at the point where we have the tools we need to do so.” We have solved many of the problems of user account management, but we have yet to solve the problems of identity management to the same extent. The magical future where I can put the supercomputer on my wrist to work in a way that delivers real value and not just interesting insights and alerts feels both disappointingly far away yet tantalizingly within our grasp. I believe that counselors are what is needed to extend our reach to that magical, and very achievable, future.
To do this I believe there are five things required:
Ubiquitous devices, available to all regardless of geography and socio economic condition, in all manner of form factors, which can run privacy-preserving LLMs and thus the interface layer for counselors Maturation of passkey patterns including recovery use cases such that the era of shared secrets can be enshrined in history Standardization of request and presentation processes of potentially verifiable data, along with standardized data structures Trustable sources of safe interaction signals with known rules and standardized data formats Machine-readable and interpretable privacy and data use notices coupled with consent receiptsThe tools we need to unlock the magical future are real… or certainly real enough for proof of concept purposes. Combining those five ingredients makes the magical future and much more magical present… and this is the present in which I want to be.
[I am indebted to Andi Hindle for his help with this post. Always have a proper English speaker check your work — IG 11/15/2023]
It is a testament to his enduring spirit that we all continue to find ways to cope with the absence of Vittorio from our identity community and from our lives. On the day that I learnt of the news, I poured out my immediate feelings in words. But as Ian put it so eloquently (like only he can), we continued to look for ways to operationalize our sadness. In his case, he and Allan Foster set out to honor Vittorio’s legacy by setting up the Vittorio Bertocci Award under the auspices of the Digital Identity Advancement Foundation, which hopefully will succeed in becoming a lasting tribute to the intellect and compassion that Vittorio shared with the identity community. My personal attempt was to try and remember the (often twisted yet somehow endearingly innocent) sense of humor that Vittorio imbued into our personal interactions. And so, prodded by the inquiries from many about the fun (and funny) t-shirt I had designed just to get a good laugh from him at Identiverse, I created a fundraiser for the Pancreatic Cancer Action Network in his honor.
Thanks to all of you Vittorio stans and your incredible generosity, we raised $3,867.38 through t-shirt orders ($917.63) and donations ($2,949.75). I am obviously gratified by the support you gave my little attempt at operationalizing my sadness. But the bonus I wasn’t expecting was the messages people left on the fundraiser site – remembering Vittorio, what he meant to them, and in some cases how cancer affected their lives personally. In these troubling times, it was heartwarming to read these small signals of our shared humanity.
Thank you all once again. And love you Vittorio, always.
Here’s the latest installment in the series on LLM-assisted coding over at The New Stack: Techniques for Using LLMs to Improve SQL Queries.
The join was failing because the two network_interfaces columns contained JSONB objects with differing shapes; Postgres’ JSONB containment operator, @>, couldn’t match them. Since the JSONB objects are arrays, and since the desired match was a key/value pair common to both arrays, it made sense to explode the array and iterate through its elements looking to match that key/value pair.
Initial solutions from ChatGPT, Copilot Chat, and newcomer Unblocked implemented that strategy using various flavors of cross joins involving Postgres’ jsonb_array_elements function.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
10 How LLMs Helped Me Build an ODBC Plugin for Steampipe
I recently read Igor Shadurin's article Dive Into dApps. In it, he defines a dApp (or decentralized application):
The commonly accepted definition of a dApp is, in short, an application that can operate autonomously using a distributed ledger system.
From Dive Into dApps
Referenced 2023-11-12T15:39:42-0500
I think that definition is too specific to blockchains. Blockchains are an implementation choice and there are other ways to solve the problem. That said, if you're looking to create a dApp with a smart contract, then Igor's article is a nice place to start.
Let's start with the goal and work backwards from there. The goal of a dApp is to give people control over their apps and the data in them. This is not how the internet works today. As I wrote in The CompuServe of Things, the web and mobile apps are almost exclusively built on a model of intervening administrative authorities. As the operators of hosted apps and controllers of the identity systems upon which they're founded, the administrators can, for any reason whatsoever, revoke your rights to the application and any data it contains. Worse, most use your data for their own purposes, often in ways that are not in your best interest.
dApps, in contrast, give you control of the data and merely operate against it. Since they don't host the data, they can run locally, at the edge. Using smart contracts on a blockchain is one way to do this, but there are others, including peer-to-peer networks and InterPlanetary File System (IPFS). The point is, to achieve their goal, dApps need a way to store data that the application can reliably and securely reference, but that a person, rather than the app provider, controls. The core requirement for achieving control is that the data service be run by a provider who is not an intermediary and that the data model be substitutable. Control requires meaningful choice among a group of interoperable providers who are substitutable and compete for the trust of their customers.
I started writing about this idea back in 2012 and called it Personal Cloud Application Architecture. At the time the idea of personal clouds had a lot of traction and a number of supporters. We built a demonstration app called Forever and later, I based the Fuse connected car application on this idea: let people control and use the data from their cars without an intermediary. Fuse's technical success showed the efficacy of the idea at scale. Fuse had a mobile app and felt like any other connected car application, but underneath the covers, the architecture gave control of the data to the car's owner. Dave Winer has also developed applications that use a substitutable backend storage based on Node.
Regular readers will wonder how I made it this far without mentioning picos. Forever and Fuse were both based on picos. Picos are designed to be self-hosted or hosted by providers who are substitutable. I've got a couple of projects tee'd up for two groups of students this winter that will further extend the suitability for picos as backends for dApps:
Support for Hosting Picos—the root pico in any instance of the pico engine is the ancestor of all picos in that engine and thus has ultimate control over them. To date, we've used the ability to stand up a new engine and control access to it as the means of providing control for the owner. This project will allow a hosting provider to easily stand up new instance of the engine and its root pico. For this to be viable, we'll use the support for peer DIDs my students built into the engine last year to give owners a peer DID connection to their root pico on their instance of the engine and thus give them control over the root pico and all its decedents.
Support for Solid Pods—at IIW this past October, we had a few sessions on how picos could be linked to Solid pods. This project will marry a pod to each pico that gets created and link their lifecycles. This, combined with their support for peer DIDs, makes the pico and its data movable between engines, supporting substitutability.
If I thought I had the bandwidth to support a third group, I'd have them work on building dApps and an App Store to run on top of this. Making that work has a few other fun technical challenges. We've done this before. As I said Forever and Fuse were both essentially dApps. Manifold, a re-creation of SquareTag is a large dApp for the Internet of Things that supports dApplets (is that a thing?) for each thing you store in it. What makes it a dApp is that the data is all in picos that could be hosted anywhere...at least in theory. Making that less theoretical is the next big step. Bruce Conrad has some ideas around that he calls the Pico Labs Affiliate Network.
I think the work of supporting dApps and personal control of our data is vitally important. As I wrote in 2014:
On the Net today we face a choice between freedom and captivity, independence and dependence. How we build the Internet of Things has far-reaching consequences for the humans who will use—or be used by—it. Will we push forward, connecting things using forests of silos that are reminiscent the online services of the 1980’s, or will we learn the lessons of the Internet and build a true Internet of Things?
From The CompuServe of Things
Referenced 2023-11-12T17:15:48-0500
The choice is ours. We can build the world we want to live in.
Vittorio Bertocci, much like many others in the identity space, had an important impact on my professional life. You can imagine how I felt when, a month following his tragic passing, I saw another blog post produced by the GOAT of creating understandable technical content about identity. Further, the subject of the post is my deepest area of knowledge: verifiable credential adoption (which was the topic of conversation for almost all my time spent with Vittorio).
Vittorio’s sage perspective on verifiable credentials is important for the IDtech community to understand. In this post, I want to outline how Vittorio influenced our direction at Trinsic and highlight a few important points from the recent post.
In 2017 I was a fresh face in the identity industry, pumped full of slogans and claims from the infinitely optimistic self-sovereign identity (SSI) evangelists who I surrounded myself with at the time. Having only seen one perspective, I fully believed that consumers could “own” their identity, that “data breaches would be a thing of the past”, and that verifiable credentials would usher in a new era of privacy maximalism.
The world needs idealists — but it also needs pragmatists. Vittorio became an archetype of the pragmatic energy that eventually worked its way into the culture and products at the company I cofounded in 2019, Trinsic. His directed questions and healthy skepticism of marvelous SSI claims came not from a Luddite spirit, but from deep experience. In a blog post about his involvement in the CardSpace project at Microsoft, he said, “When the user centric identity effort substantially failed to gain traction in actual products, with the identity industry incorporating some important innovations (hello, claims) but generally rejecting many of the key tenets I held so dear, something broke inside me. I became disillusioned with pure principled views, and moved toward a stricter Job to be done, user cases driven stance.”
For the last four years as a reusable identity infrastructure company, our developer tools for integrating verifiable credentials, identity wallets, and policy/governance tools have become quite popular. Thousands of developers have created dozens of applications that have acquired hundreds of thousands of end-users and issued close to a million credentials in production. This experience has given us a unique vantage point on patterns and methods for successfully deploying verifiable credentials in production. We’ve also spoken to many of these customers and other partners on our podcast and in private to understand these patterns more deeply.
I state all of this so that I can say the following with some credibility: Vitorrio’s perspectives (and by extension Auth0’s) are a must-read for anyone working on user-centric identity. I’ll double click on a few of what I view to be the most important points below.
What do we need to do to make a classic OpenID Connect flow behave more like the drivers license for buying wine scenario in offline life?
The two main discrepancies we identified were: Ability to use the token with multiple RPs and Ability to transact with an RP without IdP knowing anything about time and parties involved in the transaction
The first point I want to highlight is that Vittorio introduces verifiable credentials (VCs) by relating them to something his audience is familiar with — OIDC. This is not only a helpful practice for pitching products in general, but it embeds an important point for IDtech people: VCs are not a fundamental transformation of identity. VCs are an incremental improvement on previous generations of identity technology. (But one that I believe can enable exponentially better product experiences when done right.)
VCs will be adopted when they are applied to use cases that existing solutions fail to accommodate. It’s key for VC-powered products to demonstrate how VCs enable a problem to be solved in a new way — otherwise, buyers will opt for the safer federated solutions over VCs.
A classic example to illustrate my point is “passwordless login”. I’ve been hearing about it for 6 years, and yet never actually seen verifiable credentials be adopted for passwordless authentication. I believe the reason for this is that the two points above (ability to use the token with multiple RPs, IdP not knowing about the transaction) aren’t important enough for this use case, and that other, lighter-weight solutions can do it better.
We might say that there are too many cooks in the kitchen… I dare say this space is overspecified… A lot of work will need to happen in the marketplace, as production implementations with working use cases feel the pain points from these specs and run into a few walls for some of VCs to fully come to life.
Vittorio taught me about the history of OAuth, OpenID, OAuth2, and OpenID Connect. I learned about early, nonstandard iterations of “login with” buttons that had millions of active users. I learned about the market forces that led these divergent applications to eventually standardize.
Standardization is essential for adoption. But adoption is essential for knowing what to standardize (there’s nothing worse than standardizing the wrong thing)! Prematurely standardizing before adoption is a classic “cart before the horse” scenario. My conversations with Vittorio led me to write this catch-22 of interoperability post.
IDtech builders need to focus on building a good, adoptable product first. Then make it interoperable/compatible with other products second. This is a key design principle baked into Trinsic’s platform (e.g. whatever you build will inherit interoperability when it’s needed, but you won’t waste time figuring it out in the meantime).
[A misconception:] Centralized DBs will disappear… and in turn this would prevent some of the massive data leaks that we have seen in recent history. It’s unclear how that would work.
Vittorio correctly identified this as a misconception. Centralized databases indeed won’t disappear anytime soon. The notion that companies “won’t need to hold my data”, if it ever happens, will be far in the future.
The near-term disruption that will happen, however, is something I pointed out in a conversation with Vittorio that started on Twitter and moved offline. Service providers who don’t originate data themselves, but aggregate or intermediate between parties in a transaction, are at risk of disruption from verifiable credentials.
The example I use in the post linked above is Work Number. Employers give Work Number information about their employees to avoid fielding background screening calls. If employers gave that information directly to employees in a verifiable credential, however, Work Number’s role would need to change dramatically. Because of this threat, identity verification, student attestations, background screening, and other of these kinds of companies are among the first to adopt verifiable credentials.
Unless users decide to not present more data than necessary for particular operations, it is possible that they will end up disclosing more/all credential data just for usability sake.
This dynamic is Jevons paradox applied to identity — VCs counterintuitively risk creating worse privacy conditions, even with things like data minimization, because of the frequency of use. Nobody has a crystal ball, so it’s impossible to know whether this risk will materialize. Governance is the best tool at our disposal to reduce this risk and enable better privacy for people. I talk about this a fair bit in this webinar and plan to write a blog post about it in the future.
Users will typically already have credentials in their wallets and verifiers will simply need to verify them, in a (mostly) stateless fashion… However, we do have a multi-parties cold start problem. To have viable VCs we need effective, dependable and ubiquitous wallets. To have good wallets, we need relying parties implementing flows that require them, and creating real, concrete requirements for actual use. To incentivize RPs to implement and explore new flows, we need high value, ubiquitous credentials that make good business sense to leverage. But to get natural IdPs to create the infrastructure to issue such credentials, you need all of the above… plus a business case.
The chicken-and-egg problem (or, “cold start” problem) is a trick for almost all IDtech products. While there will always be exceptions to the rule, I have seen enough failure and success to feel confident in a somewhat concrete recipe for overcoming this obstacle.
Remove the wallet as a dependency. If a user needs to be redirected to an app store, download an app, step through onboarding steps, see an empty app, go scan QR codes to get credentials, all before it can actually be used… it’s extremely unlikely to be adopted. Instead, give users invisible “wallets” for their credentials. This is the #1 unlock that led to several of Trinsic’s customers scaling to hundreds of thousands of users. If your entity can play the role of issuer (or IdP) then you’re in a great position. If you’re not, obtain your own data so that you can be. Dig in with one or more companies and partner closely to build something very specific first with existing data. Sell to use cases that aren’t well-served by existing OIDC or similar technologies. Expand the markets you’re selling to by going into the long tail. Focus on either low-frequency, high-value use cases or high-frequency, low-value applications. Make it easy to integrate.Shamefully, it took me 5 years of pattern matching to land at the conclusions that Vittorio and others saw much sooner. These are the same points that led to adoption of OAuth/OIDC. And frankly, when you look at it, are pretty obvious.
The main one is being able to disclose our identity/claims without issuers knowing. It is a civil liberty; it is a right. As more of our life moves online, we should be able to express our identity like we do it offline.
Privacy is an important point. This requirement, in particular, is a requirement for most governments to be involved. It’s also a biggie for any sensitive/”vice” industry (gambling, adult content, controlled substances, etc.) which historically is a driver of new technology due to having broad appeal and high frequency.
Once [the adoption flywheel] happens, it will likely happen all of a sudden… which is why it is really a good idea to stay up-to-date and experiment with VCs TODAY
This “slow… then all at once” dynamic is a critical insight, and very true. We’ve seen this over the last year in the identity verification segment. My first conversations with identity verification companies were at Sovrin in 2018. Despite consistently following along, there was no movement from anybody for years. Suddenly, after Onfido acquired Airside in May, Plaid, Persona, ClearMe, Instnt, Au10tix, and more have jumped into the fray with their own “Reusable ID” solutions.
Auth0 posits that governments will be the critical unlock for verifiable credentials. While I don’t think that’s wrong, we are seeing increased bottoms-up adoption from the private sector, both from IDtech companies and verification providers of all kinds. Governments will play an important role, ideally anchoring wallets with high-assurance legal identity credentials and leading with standards that will produce to interoperable solutions.
If you haven’t already, I encourage you to read the whole post. I’m grateful for the Auth0 team for shipping the post after Vittorio’s passing, so the world can benefit from his knowledge. You can also continue to learn from Vittorio through his podcast, which I’ve found to be a tremendous resource over the years.
If this topic interests you, check out the podcast I host, The Future of Identity. And if you have any feedback on this post, find me on X or LinkedIn — I’m always trying to get smarter and would love to know if I’m wrong about anything. 😊
OpenID Federation draft 31 has been published at https://openid.net/specs/openid-federation-1_0-31.html and https://openid.net/specs/openid-federation-1_0.html. It’s the result of concerted efforts to make the specification straightforward to read, understand, and implement for developers. Many sections have been rewritten and simplified. Some content has been reorganized to make its structure and relationships more approachable. Many inconsistencies were addressed.
Some inconsistencies fixed resulted in a small number of breaking changes. For instance, the name “trust_mark_owners
” is now consistently used throughout, whereas an alternate spelling was formerly also used. The editors tried to make all known such changes in this version, so hopefully this will be the last set of breaking changes. We published draft 31 now in part to get these changes out to implementers. See the history entries at https://openid.net/specs/openid-federation-1_0-31.html#name-document-history for a detailed description of the changes made.
A comprehensive review of the specification is still ongoing. Expect more improvements in the exposition in draft 32. With any luck, -32 will be the basis of the next proposed Implementer’s Draft.
We’re definitely grateful for all the useful feedback we’re receiving from developers. Developer feedback is gold!
Here’s number 12 in the series on LLM-assisted coding over at The New Stack: Let’s Talk: Conversational Software Development
I keep coming back to the theme of the first article in this series: When the rubber duck talks back. Thinking out loud always helps. Ideally, you get to do that with a human partner. A rubber duck, though a poor substitute, is far better than nothing.
Conversing with LLMs isn’t like either of these options, it’s something else entirely; and we’re all in the midst of figuring out how it can work. Asking an LLM to write code, and having it magically appear? That’s an obvious life-changer. Talking with an LLM about the code you’re partnering with it to write? I think that’s a less obvious but equally profound life-changer.
The rest of the series:
1 When the rubber duck talks back
2 Radical just-in-time learning
3 Why LLM-assisted table transformation is a big deal
4 Using LLM-Assisted Coding to Write a Custom Template Function
5 Elevating the Conversation with LLM Assistants
6 How Large Language Models Assisted a Website Makeover
7 Should LLMs Write Marketing Copy?
8 Test-Driven Development with LLMs: Never Trust, Always Verify
9 Learning While Coding: How LLMs Teach You Implicitly
In a recent post, Clive Thompson speaks of the humble cassette tape as a medium that had a a weirdly Internet-like vibe. Clive is focusing on how the cassette tape unlocked creativity, but in doing so he describes its properties in a way that is helpful to discussions about online relationships in general.
Clive doesn't speak about cassette tapes being decentralized. In fact, I chuckle as I write that down. Instead he's focused on some core properties. Two I found the most interesting were that cassette tapes allowed one-to-one exchange of music and that they were permissionless. He says:
If you wanted to record a cassette, you didn’t need anyone’s permission.
This was a quietly radical thing, back when cassette recorders first emerged. Many other forms of audio or moving-image media required a lot of capital infrastructure: If you wanted to broadcast a TV show, you needed a studio and broadcasting equipment; the same goes for a radio show or film, or producing and distributing an album. And your audience needed an entirely different set of technologies (televisions, radios, projectors, record players) to receive your messages.
From The Empowering Style of Cassette Tapes
Referenced 2023-11-02T08:01:46-0400
The thing that struck me on reading this was the idea that symmetric technology democratizes speech. The web is based on assymetric technology: client-server. In theory everyone can have a server, but they don't for a lot of reasons including cost, difficulty, and friction. Consequently, the web is dominated by a few large players who act as intervening administrative authorities. They decide what happens online and who can participate. The web is not one-to-one and it is decidedly not permissionless.
In contrast, the DIDComm protocol is symmetric and so it fosters one-to-one interactions that provide meaningful, life-like online relationships. DIDComm supports autonomic identity systems that provide a foundation for one-to-one, permissionless interactons. Like the cassette tape, DIDComm is a democratizing technology.
Photo Credit: Mix Tape from Andreanna Moya Photography (CC BY-NC-ND 2.0 DEED)
Thanks for reading Phil Windley's Technometria! Subscribe for free to receive new posts and support my work.
The new “Use of Hybrid Public-Key Encryption (HPKE) with Javascript Object Signing and Encryption (JOSE)” specification has been published. Its abstract is:
This specification defines Hybrid public-key encryption (HPKE) for use with Javascript Object Signing and Encryption (JOSE). HPKE offers a variant of public-key encryption of arbitrary-sized plaintexts for a recipient public key.
HPKE works for any combination of an asymmetric key encapsulation mechanism (KEM), key derivation function (KDF), and authenticated encryption with additional data (AEAD) function. Authentication for HPKE in JOSE is provided by JOSE-native security mechanisms or by one of the authenticated variants of HPKE.
This document defines the use of the HPKE with JOSE.
Hybrid Public Key Encryption (HPKE) is defined by RFC 9180. There’s a whole new generation of specifications using it for encryption. The Messaging Layer Security (MLS) Protocol [RFC 9420] uses it. TLS Encrypted Client Hello uses it. Use of Hybrid Public-Key Encryption (HPKE) with CBOR Object Signing and Encryption (COSE) brings it to COSE. And this specification brings it to JOSE.
One of our goals for the JOSE HPKE specification is to keep it closely aligned with the COSE HPKE specification. That should be facilitated by having multiple authors in common, with Hannes Tschofenig and Orie Steele being authors of both, and me being a COSE co-chair.
Aritra Banerjee will be presenting the draft to the JOSE working group at IETF 118 in Prague. I’m hoping to see many of you there!
The specification is available at:
https://www.ietf.org/archive/id/draft-rha-jose-hpke-encrypt-01.htmlThe initial OpenID Connect specifications became final on February 25, 2014. While the working group is rightfully proud of the quality of the work and the widespread adoption it has attained, specification writing is a human endeavor and mistakes will inevitably be made. That’s why the OpenID Foundation has a process for publishing Errata corrections to specifications.
Eight issues were identified and corrected that year, with the first set of errata corrections being published on November 8, 2014. Since that time, suggestions for improvements have continued to trickle in, but with a 9+ year trickle, a total of 95 errata issues have been filed! They range from the nearly trivial, such as an instance of http
that should have been https
, to the more consequential, such as language that could be interpreted in different ways.
I’m pleased to report that, with a substantial investment by the working group, I’ve managed to work through all the 87 additional errata issues filed since the first errata set and incorporate corrections for them into published specification drafts. They are currently undergoing OpenID Foundation-wide review in preparation for a vote to approve the second set of errata corrections.
As a bonus, the OpenID Foundation plans to submit the newly minted corrected drafts for publication by ISO as Publicly Available Specifications. This should foster even broader adoption of OpenID Connect by enabling deployments in some jurisdictions around the world that have legal requirements to use specifications from standards bodies recognized by international treaties, of which ISO is one. Just in time for OpenID Connect’s 10th anniversary!
I’m pleased to bring your attention to the upcoming OpenID Summit Tokyo 2024, which will be held on Friday, January 19, 2024. Join us there for a stellar line-up of speakers and consequential conversations!
This builds on the successes of past summits organized by the OpenID Foundation Japan. For instance, I found the OpenID Summit Tokyo 2020 and associated activities and discussions both very useful and very enjoyable.
A special feature of the 2024 summit will be celebrating the 10th anniversary of the OpenID Connect specifications, which were approved on February 25, 2014. Speakers who were there for its creation, interop testing, and early deployments will share their experiences and lessons learned, including several key participants from Japan. As I recounted at EIC 2023, building ecosystems is hard. And yet we achieved that for OpenID Connect! We are working to create new identity ecosystems as we speak. I believe that the lessons learned from OpenID Connect are very applicable today. Come join the conversation!
Finally, as a teaser, I’m also helping the OpenID Foundation to plan two additional 10th anniversary celebrations at prominent 2024 identity events – one in Europe and one in the Americas. Watch this space for further news about these as it develops!
Doc Searls sent me a link to this piece from Chris Anderson on cloudless computing. Like the term zero data that I wrote about a few weeks ago, cloudless computing is a great name that captures an idea that is profound.
Cloudless computing uses cryptographic identifiers, verifiable data, and location-independent compute1 to move apps to the data wherever it lives, to perform whatever computation needs to be done, at the edge. The genius of of the name cloudless computing is that it gets us out of the trenches of dapps, web3, blockchain, and other specific implementations and speaks to an idea or concept. The abstractions can make it difficult get a firm hold on the ideas, but it's important to getting past the how so we can speak to the what and why.
You be rightly skeptical that any of this can happen. Why will companies move from the proven cloud model to something else? In this talk, Peter Levine talks specifically to that question.
One of the core arguments for why more and more computing will move to the edge is the sheer size of modern computing problems. Consider one example: Tesla Full Self Driving (FSD). I happen to be a Tesla owner and I bought FSD. At first it was just because I am very curious about it and couldn't stand to not have first-hand experience with it. But now, I like it so much I use it all the time and can't imagine driving without an AI assist. But that's beside the point. To understand why that drives computing to the edge, consider that the round trip time to get an answer from the cloud is just too great. The car needs to make decisions onboard for this to work. Essentially, to put this in the cloudless perspective, the computation has to move to where the data from the sensors is. You move the compute to the data, not the other way around.2
And that's just one example. Levine makes the point, as I and others have done, that the Internet of Things leads to trillions of nodes on the Internet. This is a difference in scale that has real impact on how we architect computer systems. While today's CompuServe of Things still relies largely on the cloud and centralized servers, that model can't last in a true Internet of Things.
The future world will be more decentralized than the current one. Not because of some grand ideal (although those certainly exist) but simply because the problems will force it to happen. We're using computers in more dynamic environments than the more static ones (like web applications) of the past. The data is too large to move and the required latency too low. Cloudless computing is the future.
NotesAnderson calls this deterministic computer. He uses that name to describe computation that is consistent and predictable regardless of how the application gets to the data, but I'm not sure that's the core idea. Location independence feels better to me.
An interesting point is that training the AI that drives the car is still done in the cloud somewhere. But once the model is built, it operates close to the data. I think this will be true for a lot of AI models.
Photo Credit: Cloudless Sunset from Dorothy Finley (CC BY 2.0 DEED - cropped)
Thanks for reading Phil Windley's Technometria! Subscribe for free to receive new posts and support my work.